接下来。装逼开始....
安装第三方库
安装请求库
pip install requests
更新请求库
pip install -U requests
安装或者更新完成以后,首先要导入requests库
import requests
发送请求
发送get请求:
# 部分源码
def get(url, params=None, **kwargs):
r"""Sends a GET request.
:param url: URL for the new :class:`Request` object.
:param params: (optional) Dictionary, list of tuples or bytes to send
in the query string for the :class:`Request`.
:param \*\*kwargs: Optional arguments that ``request`` takes.
:return: :class:`Response <Response>` object
:rtype: requests.Response
"""
kwargs.setdefault('allow_redirects', True)
return request('get', url, params=params, **kwargs)
如果得到请求没有参数,直接发送url分类:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule")
如果需要传值,params负责接收参数:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule", params={"key": "values"})
params接收的值就是该url的:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule", params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.url)
使用r.url获得url信息:
http://apis.juhe.cn/fapig/euro2020/schedule?key=9d0dfd9dbaf51de283ee8a88e58e218b
Process finished with exit code 0
其他 HTTP 请求类型:DELETE、HEAD 和 OPTIONS 都类似获取请求:
r = requests.delete("http://apis.juhe.cn/fapig/euro2020/schedule")
r = requests.head("http://apis.juhe.cn/fapig/euro2020/schedule")
r = requests.options("http://apis.juhe.cn/fapig/euro2020/schedule")
发送post请求:
# 部份源码
def post(url, data=None, json=None, **kwargs):
r"""Sends a POST request.
:param url: URL for the new :class:`Request` object.
:param data: (optional) Dictionary, list of tuples, bytes, or file-like
object to send in the body of the :class:`Request`.
:param json: (optional) json data to send in the body of the :class:`Request`.
:param \*\*kwargs: Optional arguments that ``request`` takes.
:return: :class:`Response <Response>` object
:rtype: requests.Response
"""
return request('post', url, data=data, json=json, **kwargs)
如果是来自表单的数据,也就是头信息时content-type=application/x-www-form-urlencoded时,就数据传值:
r = requests.post("http://apis.juhe.cn/fapig/euro2020/schedule", data={"key": "values"})
头信息是content-type = application/json时,就json传值:
r = requests.post("http://apis.juhe.cn/fapig/euro2020/schedule", json={"key": "values"})
发送put请求:
# 部分源码
def put(url, data=None, **kwargs):
r"""Sends a PUT request.
:param url: URL for the new :class:`Request` object.
:param data: (optional) Dictionary, list of tuples, bytes, or file-like
object to send in the body of the :class:`Request`.
:param json: (optional) json data to send in the body of the :class:`Request`.
:param \*\*kwargs: Optional arguments that ``request`` takes.
:return: :class:`Response <Response>` object
:rtype: requests.Response
"""
return request('put', url, data=data, **kwargs)
同贴请求参数类似,如果是来自表单,数据传值:
r = requests.put("http://apis.juhe.cn/fapig/euro2020/schedule", data={"key": "values"})
如果是json格式,也是直接json传值:
r = requests.put("http://apis.juhe.cn/fapig/euro2020/schedule", json={"key": "values"})
可能很疑惑,每个方法里面都有**kwargs,它是专门接收自定义字典参数的;因为这为什么可以解释了请求中没有位置参数,为什么直接传值;这是也有一个动态参数**kwargs,它负责的动态接收参数...
响应数据
上面每个请求都赋值给了r,r负责接收一个响应对象,也就是接收一个返回
返回文本信息:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule", params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.text)
{"reason":"查询成功!","result":{"data":[{"schedule_date":"2021-06-12","schedule_date_format":"06月12日","schedule_week":"周六"...
Process finished with exit code 0
返回编码格式:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule", params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.encoding)
utf-8
Process finished with exit code 0
返回二进制数据,用于处理图像的操作,更多说明,因为接口中不属于常用部分:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule", params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.content)
b'{"reason":"\xe6\x9f\xa5\xe8\xaf\xa2\xe6\x88\x90\xe5\x8a\x9f","result":{"data":[{"schedule_date":"2021-06-12","schedule_date_format":"06\xe6\x9c\x8812\xe6\x97\xa5","schedule_week...
Process finished with exit code 0
返回json格式信息:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule", params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.json())
{'reason': '查询成功', 'result': {'data': [{'schedule_date': '2021-06-12', 'schedule_date_format': '06月12日', 'schedule_week': '周六', ...
Process finished with exit code 0
返回原始字节流信息,流信息多用于写入文件中保存:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule",
params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"},
stream=True)
print(r.raw)
print(r.raw.read(100))
<urllib3.response.HTTPResponse object at 0x7fb4874f8490>
b'{"reason":"\xe6\x9f\xa5\xe8\xaf\xa2\xe6\x88\x90\xe5\x8a\x9f!","result":{"data":[{"schedule_date":"2021-06-12","schedule_date_format":"06'
Process finished with exit code 0
返回网址信息:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule",
params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.url)
http://apis.juhe.cn/fapig/euro2020/schedule?key=9d0dfd9dbaf51de283ee8a88e58e218b
Process finished with exit code 0
返回头信息:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule",
params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.headers)
{'Date': 'Sat, 12 Jun 2021 10:00:26 GMT', 'Content-Type': 'application/json;charset=utf-8', 'Transfer-Encoding': 'chunked', 'Connection': 'keep-alive'...
Process finished with exit code 0
返回cookies信息:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule",
params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.cookies)
<RequestsCookieJar[<Cookie aliyungf_tc=f7bf130436d7c08e9f74057699ee53ed61c20cd1349efaaae343e170ad9fcace for apis.juhe.cn/>]>
Process finished with exit code 0
返回http状态码:
r = requests.get("http://apis.juhe.cn/fapig/euro2020/schedule",
params={"key": "9d0dfd9dbaf51de283ee8a88e58e218b"})
print(r.status_code)
200
Process finished with exit code 0
至此,请求库的基本使用完毕...
以上总结或许能帮助到你,或许帮助不到你,但还是希望能帮助到你,如有疑问、歧义,直接私信留言会及时修正发布;感觉还不错记得点赞呦,谢谢!
未完,待续…
一直都在努力,希望您也是!
微信搜索公众号:就用python
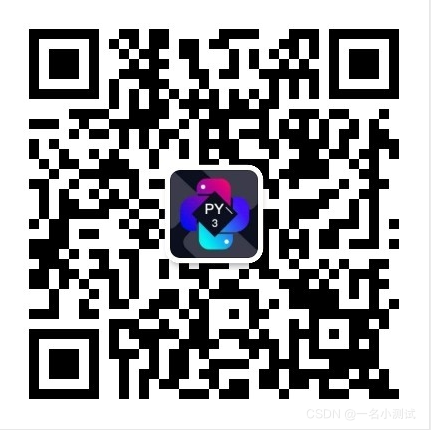