一、流程分析
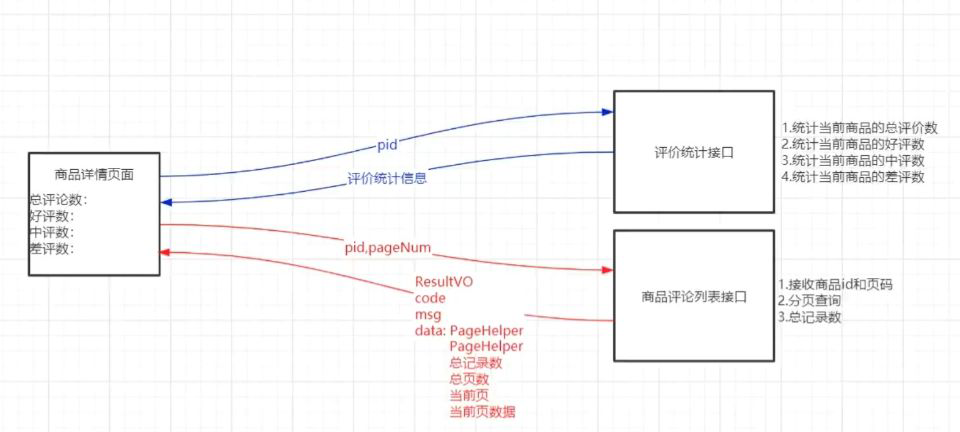
二、接口开发:改造商品评论列表接口(分页查询)
1.定义PageHelper:
@Data
@NoArgsConstructor
@AllArgsConstructor
public class PageHelper<T> {
//总记录数
private int count;
//总页数
private int pageCount;
//分页数据
private List<T> list;
}
2.改造数据库操作
ProductCommentsMapper :
@Repository
public interface ProductCommentsMapper extends GeneralDAO<ProductComments> {
//根据商品id分页查询评论信息
public List<ProductCommentsVO> selectCommontsByProductId(@Param("productId") String productId,
@Param("start") int start,
@Param("limit") int limit);
}
映射文件:
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.qfedu.fmmall.dao.ProductCommentsMapper">
<resultMap id="BaseResultMap" type="com.qfedu.fmmall.entity.ProductComments">
<id column="comm_id" jdbcType="VARCHAR" property="commId" />
<result column="product_id" jdbcType="VARCHAR" property="productId" />
<result column="product_name" jdbcType="VARCHAR" property="productName" />
<result column="order_item_id" jdbcType="VARCHAR" property="orderItemId" />
<result column="user_id" jdbcType="VARCHAR" property="userId" />
<result column="is_anonymous" jdbcType="INTEGER" property="isAnonymous" />
<result column="comm_type" jdbcType="INTEGER" property="commType" />
<result column="comm_level" jdbcType="INTEGER" property="commLevel" />
<result column="comm_content" jdbcType="VARCHAR" property="commContent" />
<result column="comm_imgs" jdbcType="VARCHAR" property="commImgs" />
<result column="sepc_name" jdbcType="TIMESTAMP" property="sepcName" />
<result column="reply_status" jdbcType="INTEGER" property="replyStatus" />
<result column="reply_content" jdbcType="VARCHAR" property="replyContent" />
<result column="reply_time" jdbcType="TIMESTAMP" property="replyTime" />
<result column="is_show" jdbcType="INTEGER" property="isShow" />
</resultMap>
<resultMap id="ProductCommentsVOMap" type="com.qfedu.fmmall.entity.ProductCommentsVO">
<id column="comm_id" jdbcType="VARCHAR" property="commId" />
<result column="product_id" jdbcType="VARCHAR" property="productId" />
<result column="product_name" jdbcType="VARCHAR" property="productName" />
<result column="order_item_id" jdbcType="VARCHAR" property="orderItemId" />
<result column="is_anonymous" jdbcType="INTEGER" property="isAnonymous" />
<result column="comm_type" jdbcType="INTEGER" property="commType" />
<result column="comm_level" jdbcType="INTEGER" property="commLevel" />
<result column="comm_content" jdbcType="VARCHAR" property="commContent" />
<result column="comm_imgs" jdbcType="VARCHAR" property="commImgs" />
<result column="sepc_name" jdbcType="TIMESTAMP" property="sepcName" />
<result column="reply_status" jdbcType="INTEGER" property="replyStatus" />
<result column="reply_content" jdbcType="VARCHAR" property="replyContent" />
<result column="reply_time" jdbcType="TIMESTAMP" property="replyTime" />
<result column="is_show" jdbcType="INTEGER" property="isShow" />
<result column="user_id" jdbcType="VARCHAR" property="userId" />
<result column="username" jdbcType="VARCHAR" property="username" />
<result column="nickname" jdbcType="VARCHAR" property="nickname" />
<result column="user_img" jdbcType="VARCHAR" property="userImg" />
</resultMap>
<select id="selectCommontsByProductId" resultMap="ProductCommentsVOMap">
SELECT u.nickname,
u.user_img,
u.username,
c.comm_id,
c.product_id,
c.product_name,
c.order_item_id,
c.user_id,
c.is_anonymous,
c.comm_type,
c.comm_level,
c.comm_content,
c.comm_imgs,
c.sepc_name,
c.reply_status,
c.reply_content,
c.reply_time,
c.is_show
FROM product_comments c
INNER JOIN users u
ON u.user_id = c.user_id
WHERE c.product_id=#{productId}
limit #{start},#{limit};
</select>
</mapper>
3.改造业务逻辑层(将查询所有评论的业务改造为分页查询)
ProductCommentsService:
public interface ProductCommentsService {
//根据商品id实现评论的分页查询
//productId:商品id pageNum:页码 limit:每页显示条数
public ResultVO listCommentsByProductId(String productId,int pageNum,int limit);
}
ProductCommentsServiceImpl :
@Service
public class ProductCommentsServiceImpl implements ProductCommentsService {
@Autowired
private ProductCommentsMapper productCommentsMapper;
public ResultVO listCommentsByProductId(String productId,int pageNum,int limit) {
// List<ProductCommentsVO> productCommentsVOS = productCommentsMapper.selectCommontsByProductId(productId);
//
// ResultVO resultVO = new ResultVO(ResStatus.OK, "success", productCommentsVOS);
//分页查询
//1.根据商品id查询总记录数
Example example = new Example(ProductComments.class);
Example.Criteria criteria = example.createCriteria();
criteria.andEqualTo("productId",productId);
int count = productCommentsMapper.selectCountByExample(example);
//2.计算出总页数(必须确定每页显示多少条 pageSize=limit)
int pageCount = count%limit==0? count/limit : count/limit+1;
//3.查询当前页的数据(因为评论中需要用户信息,因此需要联表查询---自定义)
int start = (pageNum-1)*limit;
List<ProductCommentsVO> list = productCommentsMapper.selectCommontsByProductId(productId, start, limit);
ResultVO resultVO = new ResultVO(ResStatus.OK, "success", new PageHelper<ProductCommentsVO>(count,pageCount,list));
return resultVO;
}
}
4.改造控制层:
@RestController
@CrossOrigin
@RequestMapping("/product")
@Api(value = "提供商品信息相关的接口",tags = "商品管理")
public class ProductController {
@Autowired
private ProductService productService;
@Autowired
private ProductCommentsService productCommentsService;
@ApiOperation("商品基本信息查询接口")
@GetMapping("/detail-info/{pid}")
public ResultVO getProductBasicInfo(@PathVariable("pid") String pid){
return productService.getProductBasicInfo(pid);
}
@ApiOperation("商品参数信息查询接口")
@GetMapping("/detail-params/{pid}")
public ResultVO getProductParams(@PathVariable("pid") String pid){
return productService.getProductParamsById(pid);
}
@ApiOperation("商品评论信息查询接口")
@GetMapping("/detail-commonts/{pid}")
@ApiImplicitParams({
@ApiImplicitParam(dataType = "int",name = "pageNum",value = "当前页码",required = true),
@ApiImplicitParam(dataType = "int",name = "limit",value = "每页显示条数",required = true)
})
public ResultVO getProductCommonts(@PathVariable("pid") String pid,int pageNum,int limit){
return productCommentsService.listCommentsByProductId(pid,pageNum,limit);
}
}
测试: