using System;
using System.Collections.Generic;
using System.Text;
using System.Net;
using System.Net.Sockets;
using System.Collections;
using System.IO;
using System.Text.RegularExpressions;
using RE = System.Text.RegularExpressions.Regex;
using System.Security.Cryptography.X509Certificates;
/***************************************************************************************************************************************************
* *文件名:HttpProc.cs
* *创建人:kenter
* *日 期:2010.02.23 修改
* *描 述:实现HTTP协议中的GET、POST请求
* *使 用:HttpProc.WebClient client = new HttpProc.WebClient();
client.Encoding = System.Text.Encoding.Default;//默认编码方式,根据需要设置其他类型
client.OpenRead("http://www.baidu.com");//普通get请求
MessageBox.Show(client.RespHtml);//获取返回的网页源代码
client.DownloadFile("http://www.codepub.com/upload/163album.rar",@"C:\163album.rar");//下载文件
client.OpenRead("http://passport.baidu.com/?login","username=zhangsan&password=123456");//提交表单,此处是登录百度的示例
client.UploadFile("http://hiup.baidu.com/zhangsan/upload", @"file1=D:\1.mp3");//上传文件
client.UploadFile("http://hiup.baidu.com/zhangsan/upload", "folder=myfolder&size=4003550",@"file1=D:\1.mp3");//提交含文本域和文件域的表单
*****************************************************************************************************************************************************/
namespace HttpProc
{
///<summary>
///上传事件委托
///</summary>
///<param name="sender"></param>
///<param name="e"></param>
public delegate void WebClientUploadEvent(object sender, HttpProc.UploadEventArgs e);
///<summary>
///下载事件委托
///</summary>
///<param name="sender"></param>
///<param name="e"></param>
public delegate void WebClientDownloadEvent(object sender, HttpProc.DownloadEventArgs e);
///<summary>
///上传事件参数
///</summary>
public struct UploadEventArgs
{
///<summary>
///上传数据总大小
///</summary>
public long totalBytes;
///<summary>
///已发数据大小
///</summary>
public long bytesSent;
///<summary>
///发送进度(0-1)
///</summary>
public double sendProgress;
///<summary>
///发送速度Bytes/s
///</summary>
public double sendSpeed;
}
///<summary>
///下载事件参数
///</summary>
public struct DownloadEventArgs
{
///<summary>
///下载数据总大小
///</summary>
public long totalBytes;
///<summary>
///已接收数据大小
///</summary>
public long bytesReceived;
///<summary>
///接收数据进度(0-1)
///</summary>
public double ReceiveProgress;
///<summary>
///当前缓冲区数据
///</summary>
public byte[] receivedBuffer;
///<summary>
///接收速度Bytes/s
///</summary>
public double receiveSpeed;
}
///<summary>
///实现向WEB服务器发送和接收数据
///</summary>
public class WebClient
{
private WebHeaderCollection requestHeaders, responseHeaders;
private TcpClient clientSocket;
private MemoryStream postStream;
private Encoding encoding = Encoding.Default;
private const string BOUNDARY = "--HEDAODE--";
private const int SEND_BUFFER_SIZE = 10245;
private const int RECEIVE_BUFFER_SIZE = 10245;
private string cookie = "";
private string respHtml = "";
private string strRequestHeaders = "";
private string strResponseHeaders = "";
private int statusCode = 0;
private bool isCanceled = false;
public event WebClientUploadEvent UploadProgressChanged;
public event WebClientDownloadEvent DownloadProgressChanged;
///<summary>
///初始化WebClient类
///</summary>
public WebClient()
{
responseHeaders = new WebHeaderCollection();
requestHeaders = new WebHeaderCollection();
}
/// <summary>
/// 获得字符串中开始和结束字符串中间得值
/// </summary>
/// <param name="str"></param>
/// <param name="s">开始</param>
/// <param name="e">结束</param>
/// <returns></returns>
public string gethtmlContent(string str, string s, string e)
{
Regex rg = new Regex("(?<=(" + s + "))[.\\s\\S]*?(?=(" + e + "))", RegexOptions.Multiline | RegexOptions.Singleline);
return rg.Match(str).Value;
}
/// <summary>
/// 过滤HTML字符
/// </summary>
/// <param name="source"></param>
/// <returns></returns>
public string htmlConvert(string source)
{
string result;
//remove line breaks,tabs
result = source.Replace("\r", " ");
result = result.Replace("\n", " ");
result = result.Replace("\t", " ");
//remove the header
result = Regex.Replace(result, "(<head>).*(</head>)", string.Empty, RegexOptions.IgnoreCase);
result = Regex.Replace(result, @"<( )*script([^>])*>", "<script>", RegexOptions.IgnoreCase);
result = Regex.Replace(result, @"(<script>).*(</script>)", string.Empty, RegexOptions.IgnoreCase);
//remove all styles
result = Regex.Replace(result, @"<( )*style([^>])*>", "<style>", RegexOptions.IgnoreCase); //clearing attributes
result = Regex.Replace(result, "(<style>).*(</style>)", string.Empty, RegexOptions.IgnoreCase);
//insert tabs in spaces of <td> tags
result = Regex.Replace(result, @"<( )*td([^>])*>", " ", RegexOptions.IgnoreCase);
//insert line breaks in places of <br> and <li> tags
result = Regex.Replace(result, @"<( )*br( )*>", "\r", RegexOptions.IgnoreCase);
result = Regex.Replace(result, @"<( )*li( )*>", "\r", RegexOptions.IgnoreCase);
//insert line paragraphs in places of <tr> and <p> tags
result = Regex.Replace(result, @"<( )*tr([^>])*>", "\r\r", RegexOptions.IgnoreCase);
result = Regex.Replace(result, @"<( )*p([^>])*>", "\r\r", RegexOptions.IgnoreCase);
//remove anything thats enclosed inside < >
result = Regex.Replace(result, @"<[^>]*>", string.Empty, RegexOptions.IgnoreCase);
//replace special characters:
result = Regex.Replace(result, @"&", "&", RegexOptions.IgnoreCase);
result = Regex.Replace(result, @" ", " ", RegexOptions.IgnoreCase);
result = Regex.Replace(result, @"<", "<", RegexOptions.IgnoreCase);
result = Regex.Replace(result, @">", ">", RegexOptions.IgnoreCase);
result = Regex.Replace(result, @"&
C#、.NET网络请求总结(WebClient和WebRequest)
最新推荐文章于 2023-12-03 20:23:10 发布
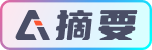