//主布局
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity" >
<TextView
android:id="@+id/myTextView"
android:layout_width="50dp"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:ellipsize="marquee"
android:focusable="true"
android:focusableInTouchMode="true"
android:marqueeRepeatLimit="marquee_forever"
android:singleLine="true"
android:text="testf;dsafjieoafjesla;fjdioa;"
android:textColor="@android:color/black" >
</TextView>
<com.example.test.HorizontalScorllTextView
android:id="@+id/scrollTextView"
android:layout_width="200dp"
android:layout_centerHorizontal="true"
android:layout_below="@+id/myTextView"
android:layout_height="wrap_content"
android:scrollbars="none"
></com.example.test.HorizontalScorllTextView>
<com.example.test.MarqueeTextView
android:id="@+id/marqueeTextView"
android:layout_width="200dp"
android:layout_height="wrap_content"
android:singleLine="true"
android:text="tesja;eao;ffjidaofjieloafj.afiaeofjidk.afjie"
android:layout_centerHorizontal="true"
android:layout_below="@+id/scrollTextView"
/>
</RelativeLayout>
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:orientation="horizontal" >
<TextView
android:id="@+id/tv_video_name"
android:layout_height="wrap_content"
android:layout_width="wrap_content"
android:singleLine="true"
android:textColor="#000000"
android:textSize="15sp"
android:layout_gravity="center_horizontal"
android:layout_marginLeft="10px"
android:layout_marginRight="10px"
/>
</LinearLayout>
package com.example.test;
import android.os.Bundle;
import android.app.Activity;
import android.view.Menu;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
HorizontalScorllTextView scrollView = (HorizontalScorllTextView) findViewById(R.id.scrollTextView);
scrollView.setText("fdajffjdka;lsfieafja;fjisdaofjeifjekalfjdioafjieafj;dsafaefeaf;ejifda;fjie");
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
}
package com.example.test;
import android.content.Context;
import android.util.AttributeSet;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.FrameLayout;
import android.widget.HorizontalScrollView;
import android.widget.TextView;
import com.example.test.R;
public class HorizontalScorllTextView extends HorizontalScrollView implements Runnable{
int currentScrollX = 0;
TextView tv;
public HorizontalScorllTextView(Context context) {
super(context);
initView(context);
}
public HorizontalScorllTextView(Context context, AttributeSet attrs) {
super(context, attrs);
initView(context);
}
public HorizontalScorllTextView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
initView(context);
}
void initView(Context context){
View v = LayoutInflater.from(context).inflate(R.layout.scroll_layout, null);
tv = (TextView)v.findViewById(R.id.tv_video_name);
this.addView(v);
}
public void setText(String text){
tv.setText(text);
startScroll();
}
private void startScroll(){
this.removeCallbacks(this);
post(this);
}
@Override
public void run() {
currentScrollX ++;
scrollTo(currentScrollX, 0);
if (currentScrollX >= tv.getWidth()) {
scrollTo(0, 0);
currentScrollX = 0;
}
postDelayed(this, 50);
}
}
package com.example.test;
import android.content.Context;
import android.graphics.Canvas;
import android.graphics.Paint;
import android.util.AttributeSet;
import android.widget.TextView;
public class MarqueeTextView extends TextView implements Runnable {
private int currentScrollX;
private boolean isStop = false;
private int textWidth;
private boolean isMeasure = false;
public MarqueeTextView(Context context) {
super(context);
}
public MarqueeTextView(Context context, AttributeSet attrs) {
super(context, attrs);
}
public MarqueeTextView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
if (!isMeasure) {
getTextWidth();
isMeasure = true;
}
}
/**
* 获取文字宽度
*/
private void getTextWidth() {
Paint paint = this.getPaint();
String str = this.getText().toString();
textWidth = (int) paint.measureText(str);
}
@Override
public void run() {
currentScrollX -= 1;
scrollTo(currentScrollX, 0);
if (isStop) {
return;
}
if (getScrollX() <= -(this.getWidth())) {
scrollTo(textWidth, 0);
currentScrollX = textWidth;
}
postDelayed(this, 10);
}
public void startScroll() {
isStop = false;
this.removeCallbacks(this);
post(this);
}
public void stopScroll() {
isStop = true;
}
public void startFor0() {
currentScrollX = 0;
startScroll();
}
@Override
public void setText(CharSequence text, BufferType type) {
super.setText(text, type);
startScroll();
}
@Override
public void destroyDrawingCache() {
super.destroyDrawingCache();
}
}
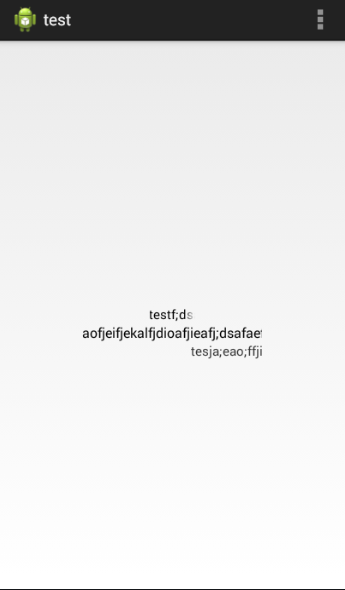