2018/4/5
51单片机
1.说在前面:要和几个朋友做一个项目 ,其中要用到DS18B20,简单介绍下它的特点吧
2.简介:
1.电压范围:3.0到5.5v
2.温度范围:-55度到125度
3.可编程分辨率为9-12位,对应分辨温度为0.5度,0.25度,0.125度。0.0625度
4.特点:采用了单线接口的方式,通过一根线实现和MCU之间的单向通讯
3.内部结构
4.温度和数据之间的关系
数据位一共是16位,高字节前5位是符号位(全0位为正,全1位负),后面为数据位,从2^6开始向后,如果为正值,直接乘以0.0625得标准值。如果为负值,则减一再取反
5.在DS18b20中对ROM和RAM进行配置,达到初始化的效果
6.控制八段数码管显示温度代码:
//预编译文件
#ifndef _temp_H
#define _temp_H
#include<reg52.h>
//定义无符号字符型
#ifndef uchar
#define uchar unsigned char
#endif
//定义无符号字符型
#ifndef uint
#define uint unsigned int
#endif
sbit DSPORT=P3^7;
#endif
#include"temp.h"
//延时1ms
void Delay1ms(uint y)
{
uint x;
for(;y>0;y--)
{
for(x=110;x>0;x--);
}
}
//DS18B20 初始化函数
//1.确定返回值 1:存在 0:不存在
uchar Ds18b20Init()
{
uchar i=0;
DSPORT=0; //拉低
i=70;
while(i--);//延时642us
DSPORT=1; //拉高
i=0;
while(DSPORT)//已读取低电平
{
Delay1ms(1);//延时1ms
i++;
if(i>5)
{
return 0;//初始化失败
}
}
return 1;//初始化成功
}
//写入8位数值
void DsWriteByte(uchar dat)
{
uchar i,j;
for(j=0;j<8;j++)
{
DSPORT=0; //拉低
DSPORT=dat&0x01;//判断最低位是否为1
i=6;
while(i--);//延时68us
DSPORT=1; //拉高
dat>>=1;//右移符号,保证循环的每一次都是0X01
}
}
uchar DsReadByte()
{
uint i,j;
uchar bi,byte;
for(j=8;j>0;j--)
{
DSPORT=0; //拉低
i++; //延时1ms
DSPORT=1; //拉高
i++;
i++;
bi=DSPORT; //0
byte=(byte>>1)|(bi<<7);//移位 1000 0000->0100 0000 | 0
i=4;
while(i--);
}
return byte;//读取
}
void DSchangetemp()//对所给数值进行转换
{
Ds18b20Init();
Delay1ms(1);
DsWriteByte(0XCC);//发送指令,开启温度传感器
DsWriteByte(0x44);//发送指令,温度传感器为12位
}
void DSreadtempcom()//发送温度读取指令
{
Ds18b20Init();
Delay1ms(1);
DsWriteByte(0XCC);//发送指令,开启温度传感器
DsWriteByte(0xbe);//发送指令,温度传感器为12位
}
int DSreadtemp()//温度读取
{
int temp=0;
uchar tmh,tml;
DSchangetemp();
DSreadtempcom();
tml=DsReadByte(); //读取低字节
tmh=DsReadByte(); //读取高字节
temp= tmh;
temp<<=8;//左移八位,所以成为了低八位
temp|=tml;//获得了低八位数据
return temp;
}
#include "reg52.h" //此文件中定义了单片机的一些特殊功能寄存器
#include"temp.h"
typedef unsigned int u16; //对数据类型进行声明定义
typedef unsigned char u8;
sbit LSA=P2^2;
sbit LSB=P2^3;
sbit LSC=P2^4;
char num=0;
u8 Dispdata[8];
u8 code smgduan[10]={0x3f,0x06,0x5b,0x4f,0x66,0x6d,0x7d,0x07,0x7f,0x6f};
//延时函数
void delay(u16 i)
{
while(i--);
}
//数据处理函数
void datapros(int temp)
{
float tp;
if(temp<0)
{
Dispdata[0]=0x40;//代表负号
temp=temp-1;
temp=~temp;
tp=temp;
temp=tp*0.0625*100+0.5;//得到标准温度
}
else
{
Dispdata[0]=0x00;
tp=temp;
temp=tp*0.0625*100+0.5;//得到标准温度
}
Dispdata[1]=smgduan[temp/10000];// 百位
Dispdata[2]=smgduan[temp%10000/1000];//十位
Dispdata[3]=smgduan[temp%10000%1000/100]|0x80 ;//个位,显示小数点
Dispdata[4]=smgduan[temp%100/10];//十分位
Dispdata[5]=smgduan[temp%100%10];//十分位
}
void DigDisplay()
{
u8 i;
for(i=0;i<4;i++)
{
switch(i) //位选,选择点亮的数码管,
{
case(0):
LSA=0;LSB=0;LSC=0; break;//显示第0位
case(1):
LSA=1;LSB=0;LSC=0; break;//显示第1位
case(2):
LSA=0;LSB=1;LSC=0; break;//显示第2位
case(3):
LSA=1;LSB=1;LSC=0; break;//显示第3位
case(4):
LSA=0;LSB=0;LSC=1; break;//显示第4位
case(5):
LSA=1;LSB=0;LSC=1; break;//显示第5位
}
P0=Dispdata[5-i];//发送段码
delay(100); //间隔一段时间扫描
P0=0x00;//消隐
}
}
void main()
{
while(1)
{
datapros(DSreadtemp()); //数据处理函数
DigDisplay();//数码管显示函数
}
}
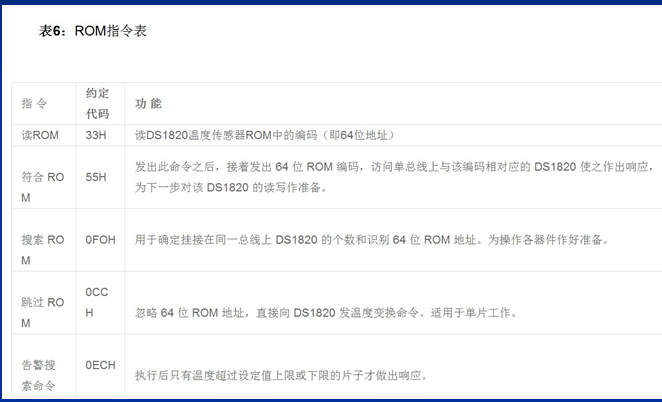
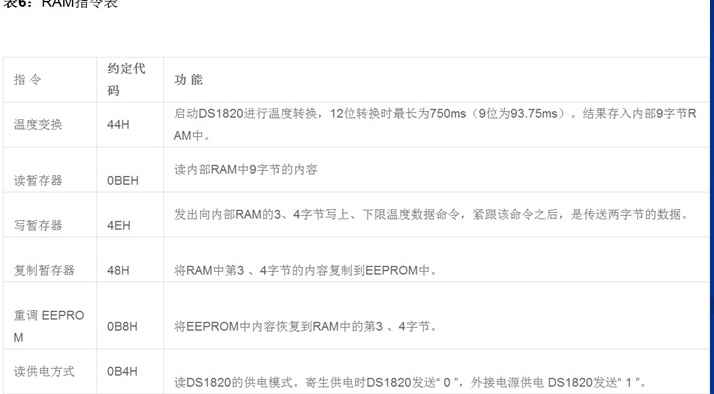