这周我学习了MySQL以及JDBC,所以就以javafx为媒介,写了个学生管理系统,全程独立写完,毫无保留供大家参考,如果有什么不解的地方可以私信我,尽量帮大家解答
一.作品展示
1.登录界面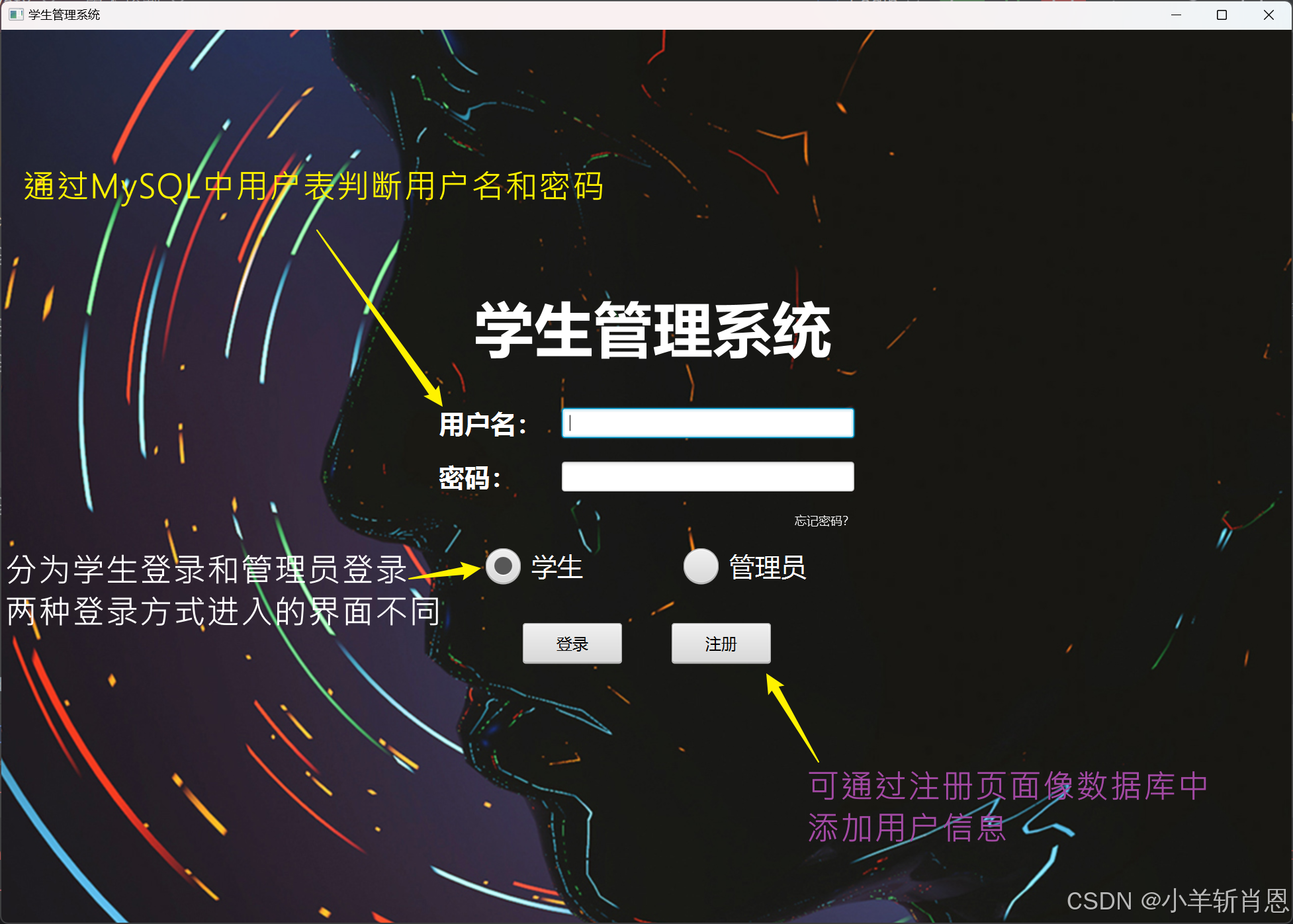
2.注册界面: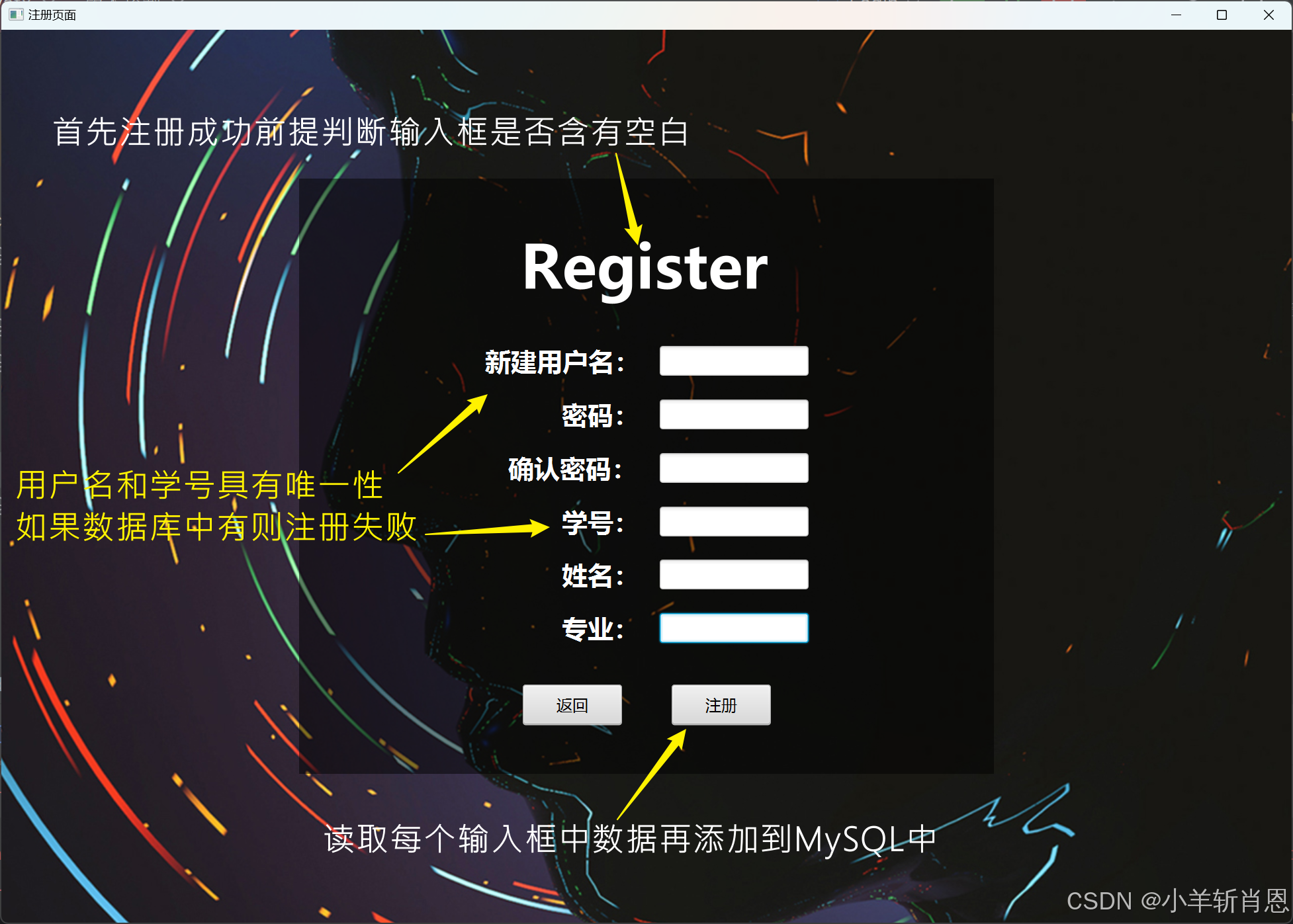
3.学生页面: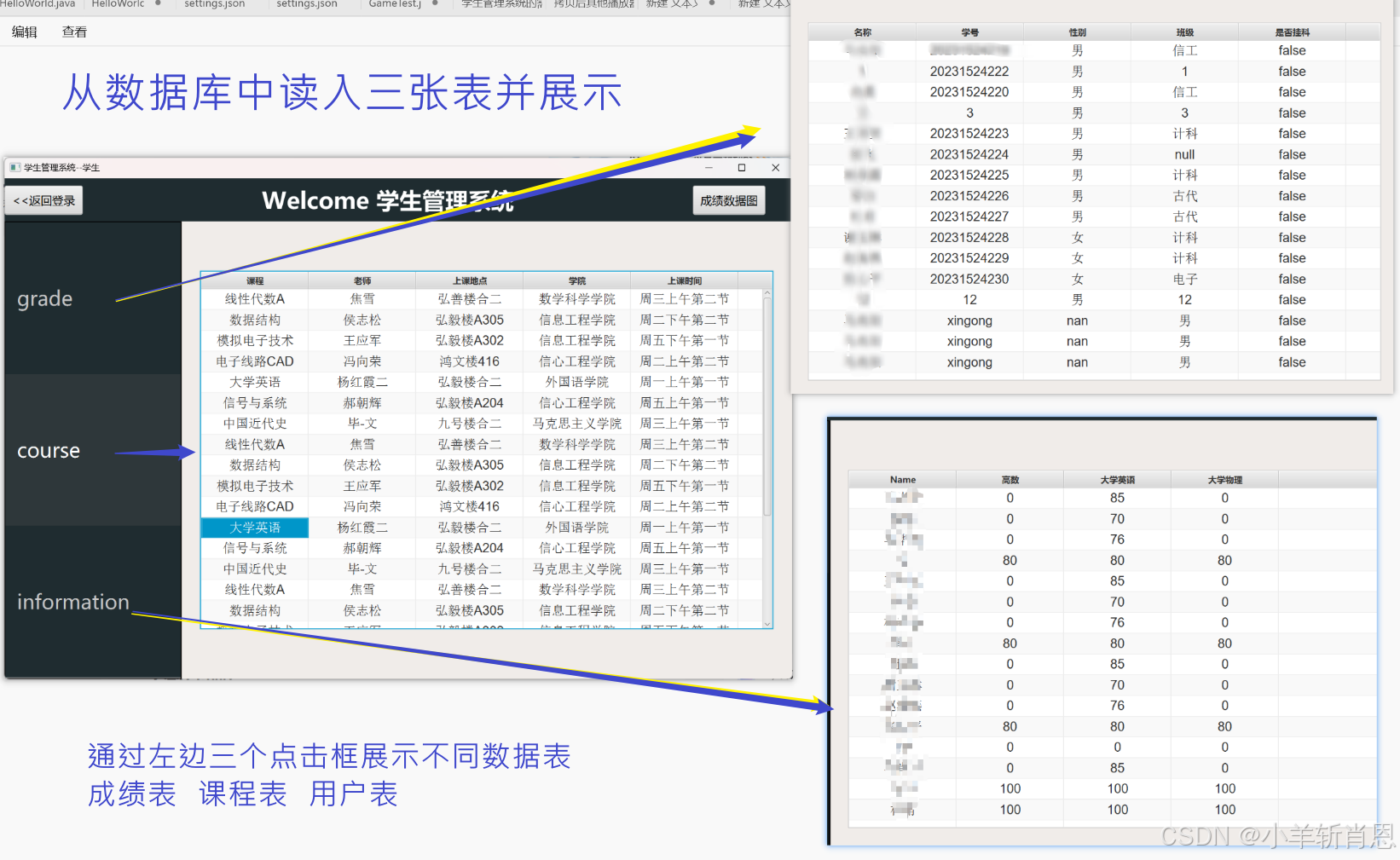
这里注意右上角有个成绩数据图按钮,让我们来看看
二.管理员页面:
那么,接下来我来给大家展示我的源码和一些小思路
二.E-R图 + 系统结构图
mysql表中对应关系:
系统结构图:
三.代码show
1.框架: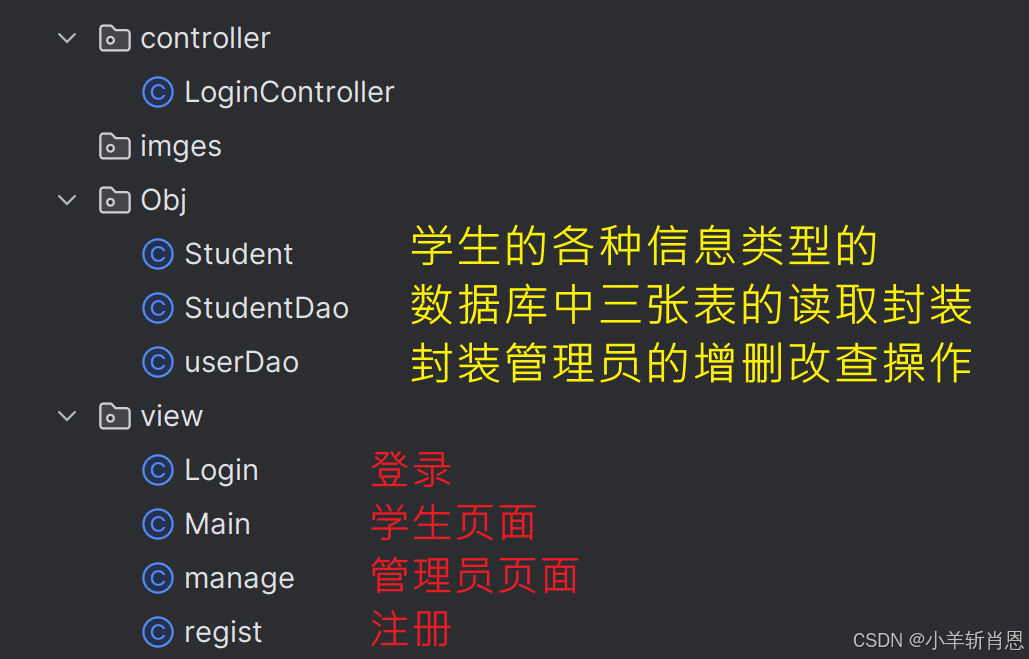
2.登录代码:
import javafx.application.Application;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.image.Image;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import java.sql.*;
public class Login extends Application {
//4.获取执行sql的对象 Statement
public static Connection conn;
public PreparedStatement stmt;
@Override
public void start(Stage stage) throws Exception {
stage.setTitle("学生管理系统");
GridPane grid=new GridPane();
grid.setAlignment(Pos.CENTER);
//水平和垂直间距
grid.setHgap(20);
grid.setVgap(20);
//距离上下左右外框
grid.setPadding(new Insets(25,25,25,25));
Text scenetitle =new Text(" 学生管理系统");
scenetitle.setFont(Font.font("Thoma", FontWeight.BOLD,60));
scenetitle.setFill(Color.WHITE);
grid.add(scenetitle,0,0,2,1);
Label userName =new Label("用户名:");
userName.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
userName.setTextFill(Color.WHITE);
grid.add(userName,0,2);
TextField userTextFild =new TextField();
userTextFild.setPrefWidth(100); // 设置文本框宽度为200像素
userTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(userTextFild,1,2);
Label pw =new Label("密码:");
pw.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
pw.setTextFill(Color.WHITE);
grid.add(pw,0,3);
PasswordField pwBox =new PasswordField();
pwBox.setPrefWidth(100);
pwBox.setPrefHeight(30);
grid.add(pwBox,1,3);
final ToggleGroup tg = new ToggleGroup();
RadioButton rb1 = new RadioButton("学生");
rb1.setToggleGroup(tg);
rb1.setUserData("A");
rb1.setSelected(true);
rb1.requestFocus();
RadioButton rb2 = new RadioButton("管理员");
rb2.setToggleGroup(tg);
rb2.setUserData("B");
rb1.setStyle("-fx-text-fill: white;-fx-font-size: 26;");
rb2.setStyle("-fx-text-fill: white;-fx-font-size: 26;");
final boolean[] flag = {false};
tg.selectedToggleProperty().addListener(new ChangeListener<Toggle>() {
public void changed(ObservableValue<? extends Toggle> ov, Toggle old_toggle, Toggle new_toggle) {
if (tg.getSelectedToggle() != null) {
System.out.println(tg.getSelectedToggle().getUserData().toString().equals("B"));
if(tg.getSelectedToggle().getUserData().toString().equals("B")) flag[0] =true;
else flag[0] =false;
}
}
});
HBox hbtg=new HBox(100);
hbtg.setAlignment(Pos.CENTER);
hbtg.getChildren().addAll(rb1,rb2);
grid.add(hbtg,0,5,2,1);
Text wang =new Text(" 忘记密码?");
wang.setFont(Font.font("Thoma", FontWeight.NORMAL,12));
wang.setFill(Color.WHITE);
grid.add(wang,1,4);
Button btn1 =new Button("登录");
Button btn2 =new Button("注册");
btn1.setMinWidth(100); // 设置按钮最小宽度为100像素
btn1.setMinHeight(40); // 设置按钮最小高度为40像素
btn1.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
btn2.setStyle("-fx-text-fill: black;-fx-font-size: 16px;");
btn2.setMinWidth(100); // 设置按钮最小宽度为100像素
btn2.setMinHeight(40); // 设置按钮最小高度为40像素
HBox hbBtn=new HBox(50);
hbBtn.setAlignment(Pos.CENTER);
hbBtn.getChildren().addAll(btn1,btn2);
grid.add(hbBtn,0,7,2,1);
/*Image bg=new Image("file:///D:/作品/bilibili.xiazai/表单/image/dark1.jpg");
ImageView imageView =new ImageView(bg);
root.getChildren().add(imageView);*/
//注册
btn2.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent actionEvent) {
regist regist=new regist(stage);
stage.close();
}
});
//登录校验
btn1.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent actionEvent) {
String usename=userTextFild.getText();
String pwd=pwBox.getText();
System.out.println(usename+" "+pwd);
if (usename.equals("")||pwd.equals("")) showLoginFailureAlert("登录失败", "用户名或密码不能为空");
else {
String sql ="select * from users;";
ResultSet rs= null;
boolean into=false;
try {
stmt= conn.prepareStatement(sql);
rs = stmt.executeQuery(sql);
while(rs.next()){
String username=rs.getNString(1);
String password=rs.getNString(2);
if (usename.equals(username)&&pwd.equals(password)) into =true;
}
} catch (SQLException e) {
throw new RuntimeException(e);
}
if (into) {
showLoginSuccessAlert("登录成功","Welcome back!");
if (!flag[0]) {
Main main = new Main(stage);
}else {
manage manage = new manage(stage);
}
// Main main = new Main(stage);
//manage manage=new manage(stage);
stage.close();
}else {
showLoginFailureAlert("登录失败", "用户名或密码错误");
}
}
}
});
// 创建 BackgroundImage 对象
Image bgimg=new Image("file:///D:/作品/bilibili.xiazai/表单/image/dark1.jpg");
BackgroundImage background = new BackgroundImage(bgimg,
BackgroundRepeat.NO_REPEAT,
BackgroundRepeat.NO_REPEAT,
BackgroundPosition.CENTER,
BackgroundSize.DEFAULT);
// 创建 Background 对象并设置到根节点
grid.setBackground(new Background(background));
// 创建场景并设置到舞台
Scene scene = new Scene(grid, 1300, 900);
stage.setScene(scene);
stage.show();
}
//初始化,在star()执行之前执行,一般可以再这里面新建一个线程去连接数据库
//(这样可以保证和显示窗口同步进行)
@Override
public void init()throws Exception{
super.init();
// 1.注册驱动
Class.forName("com.mysql.jdbc.Driver");
//2.获取连接
String url="jdbc:mysql://127.0.0.1:3306/test";
String username="root";
String password="123456";
conn= DriverManager.getConnection(url,username,password);
}
//关闭窗口的时候可以在这里做一些清理数据库或者资源链接的操作
@Override
public void stop() throws Exception {
super.stop();
System.out.println("stop()...");
}
public static void main(String[] args) {
launch();
}
public static void showLoginFailureAlert(String title, String message) {
Alert alert = new Alert(Alert.AlertType.ERROR);
alert.setTitle(title);
alert.setHeaderText(null);
alert.setX(650);
alert.setY(400);
alert.getDialogPane().setMinHeight(200);
alert.getDialogPane().setMinWidth(200);
alert.setContentText(message);
alert.showAndWait();
}
public static void showLoginSuccessAlert(String title,String message) {
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle(title);
alert.setHeaderText(null);
alert.setX(650);
alert.setY(400);
alert.getDialogPane().setMinHeight(200);
alert.getDialogPane().setMinWidth(200);
alert.setContentText(message);
alert.showAndWait();
}
}
上方代码主要就是通过javafx的布局场景来实现的,都是些基础活,比较具有逻辑性的就是登录和注册按钮的监听事件来判断登录,以及对两个单选按钮就行数据获取
还有就是在init()方法初始化连接数据库的代码,之后直接实用sql语句就可以了
下面这两个方法设为了静态方法,每个类都可以调用,这俩是操作成功弹窗和失败弹窗
非常的实用,只用传进去两个文字参数就可以喽
public static void showLoginFailureAlert(String title, String message) {
Alert alert = new Alert(Alert.AlertType.ERROR);
alert.setTitle(title);
alert.setHeaderText(null);
alert.setX(650);
alert.setY(400);
alert.getDialogPane().setMinHeight(200);
alert.getDialogPane().setMinWidth(200);
alert.setContentText(message);
alert.showAndWait();
}
public static void showLoginSuccessAlert(String title,String message) {
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle(title);
alert.setHeaderText(null);
alert.setX(650);
alert.setY(400);
alert.getDialogPane().setMinHeight(200);
alert.getDialogPane().setMinWidth(200);
alert.setContentText(message);
alert.showAndWait();
}
3.注册代码:
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.PasswordField;
import javafx.scene.control.TextField;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class regist {
private final Stage stage =new Stage();
public regist(Stage stage1) {
GridPane grid=new GridPane();
grid.setAlignment(Pos.CENTER);
// 创建 BackgroundImage 对象
Image bgimg=new Image("file:///D:/作品/bilibili.xiazai/表单/image/dark1.jpg");
ImageView bgimageView =new ImageView(bgimg);
grid.setHgap(20);
grid.setVgap(20);
//距离上下左右外框
grid.setPadding(new Insets(25,25,25,25));
Text scenetitle =new Text(" Register");
scenetitle.setFont(Font.font("Thoma", FontWeight.BOLD,60));
scenetitle.setFill(Color.WHITE);
grid.add(scenetitle,0,0,2,1);
Label userName =new Label("新建用户名:");
userName.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
userName.setTextFill(Color.WHITE);
grid.add(userName,0,2);
TextField userTextFild =new TextField();
userTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
userTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(userTextFild,1,2);
Label pw =new Label(" 密码:");
pw.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
pw.setTextFill(Color.WHITE);
grid.add(pw,0,3);
PasswordField pwBox =new PasswordField();
pwBox.setPrefWidth(150);
pwBox.setPrefHeight(30);
grid.add(pwBox,1,3);
Label npw =new Label(" 确认密码:");
npw.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
npw.setTextFill(Color.WHITE);
grid.add(npw,0,4);
PasswordField npwBox =new PasswordField();
npwBox.setPrefWidth(150);
npwBox.setPrefHeight(30);
grid.add(npwBox,1,4);
//-------------------------------------------
Label numberL =new Label(" 学号:");
numberL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
numberL.setTextFill(Color.WHITE);
grid.add(numberL,0,5);
TextField numberTextFild =new TextField();
numberTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
numberTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(numberTextFild,1,5);
//------------------------
Label nameL =new Label(" 姓名:");
nameL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
nameL.setTextFill(Color.WHITE);
grid.add(nameL,0,6);
TextField nameTextFild =new TextField();
nameTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
nameTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(nameTextFild,1,6);
Label majorL =new Label(" 专业:");
majorL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
majorL.setTextFill(Color.WHITE);
grid.add(majorL,0,7);
TextField majorTextFild =new TextField();
majorTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
majorTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(majorTextFild,1,7);
Button btn =new Button("注册");
btn.setMinWidth(100); // 设置按钮最小宽度为100像素
btn.setMinHeight(40); // 设置按钮最小高度为40像素
btn.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
Button back =new Button("返回");
back.setMinWidth(100); // 设置按钮最小宽度为100像素
back.setMinHeight(40); // 设置按钮最小高度为40像素
back.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
HBox hbBtn=new HBox(50);
hbBtn.setAlignment(Pos.CENTER);
hbBtn.getChildren().addAll(back,btn);
grid.add(hbBtn,0,9,2,1);
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent actionEvent) {
String username=userTextFild.getText();
String pwd=pwBox.getText();
String npwd=npwBox.getText();
String number=numberTextFild.getText();
String name=nameTextFild.getText();
String major= majorTextFild.getText();
if (username.isEmpty()||pwd.isEmpty()||npwd.isEmpty()||number.isEmpty()||name.isEmpty()|| major.isEmpty()){
Login.showLoginFailureAlert("注册失败","输入框存在空白");
}else {
if (!pwd.equals(npwd)){
Login.showLoginFailureAlert("注册失败","两次密码输入的不相同");
}else {
String sql="INSERT INTO grade (number,name,math,english,physics,username) values (?,?,?,?,?,?)";
try {
PreparedStatement stmt=Login.conn.prepareStatement(sql);
stmt.setString(1, number);
stmt.setString(2, name);
stmt.setInt(3,0);
stmt.setInt(4,0);
stmt.setInt(5,0);
stmt.setString(6, username);
int rs = stmt.executeUpdate();
} catch (SQLException e) {
if (e.getErrorCode() == 1062) { // 1062 错误码表示违反了唯一约束
// 处理重复 username 的情况
Login.showLoginFailureAlert("注册失败", "该学号或用户名已经存在,请检查后再注册哦~");
} else {
Login.showLoginFailureAlert("注册失败", "未知错误,请稍后再尝试,grande表出现异常");
}
throw new RuntimeException(e);
}
sql = "INSERT INTO users (username, password, member, name, gender, major) VALUES (?, ?, ?, ?, ?, ?)";
try {
PreparedStatement stmt=Login.conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, pwd);
stmt.setString(3, number);
stmt.setString(4, name);
stmt.setString(5, "男");
stmt.setString(6, major);
int rs = stmt.executeUpdate();
Login.showLoginSuccessAlert("注册成功", "请牢记你的账号和密码");
stage.close();
stage1.show();
} catch (Exception e) {
Login.showLoginFailureAlert("注册失败","未知错误,请稍后再尝试");
throw new RuntimeException(e);
}
}
}
}
});
back.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
stage1.show();
stage.close();
}
});
// 创建 StackPane 作为最外层容器
StackPane root = new StackPane();
StackPane mid = new StackPane();
//半透明背景
Rectangle backgroundRect = new Rectangle(300,150,700,600);
backgroundRect.setFill(Color.valueOf("#0000007F"));
mid.getChildren().add(backgroundRect);
// 设置背景图片
root.getChildren().add(0, bgimageView);
// 添加 Group 布局
mid.getChildren().add(grid);
root.getChildren().add(mid);
Scene scene = new Scene(root,1300,900);
stage.setScene(scene);
stage.setTitle("注册页面");
stage.show();
}
}
上方同登录页面,一些布局的基本操作,关键逻辑代码在注册按钮的事件监听事件中,当输入框中为空会出现什么弹框,当注册的用户名或学号在数据库中已经存在会跳出什么弹框,以及登录成功后如何把数据存到数据库中
try {
、、、、
} catch (SQLException e) {
if (e.getErrorCode() == 1062) { // 1062 错误码表示违反了唯一约束
// 处理重复 username 的情况
Login.showLoginFailureAlert("注册失败", "该学号或用户名已经存在,请检查后再注册哦~");
}
当在try-catch块中异常代码为1062就表示向数据库中添加某个唯一性的字段已存在的报错代码
4.学生页面代码:
import com.javafx01.Obj.Student;
import com.javafx01.Obj.StudentDao;
import demo.bing;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Pos;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import java.sql.Statement;
import java.util.List;
public class Main {
private final Stage stage =new Stage();
private ListView<String> listView;
private TableView<Student> tableView;
private StudentDao studentDao;
public Main(Stage loginStage) {
studentDao= new StudentDao();
// 左侧列表 ListView
listView = new ListView<>();
ObservableList<String> categories = FXCollections.observableArrayList("grade", "course", "information");
listView.setItems(categories);
listView.setFixedCellSize(212);
// 设置 ListView 背景颜色为黑色
listView.setStyle("-fx-background-color: black;");
listView.setCellFactory(param -> {
ListCell<String> cell = new ListCell<>() {
@Override
protected void updateItem(String item, boolean empty) {
super.updateItem(item, empty);
if (empty || item == null) {
setText(null);
setGraphic(null);
} else {
setText(item.toString());
setMinWidth(200);
setStyle("-fx-font-size: 28px;-fx-background-color: #1f2c30;-fx-text-fill: #C8C8C8FF;"); // 设置每一行的字体大小为28像素
if (isSelected()) {
setStyle("-fx-font-size: 28px; -fx-background-color: #2e373a; -fx-text-fill: #FFFFFF;");
}
}
}
};
return cell;});
// 右侧表格 TableView
tableView = new TableView<>();
tableView.setPrefWidth(800); // 设置 TableView 宽度
tableView.setPrefHeight(500); // 设置 TableView 宽度
tableView.getSelectionModel().setCellSelectionEnabled(true);//只能选中单元格
// 监听列表选择事件
listView.getSelectionModel().selectedItemProperty().addListener((observable, oldValue, newValue) -> {
if (newValue != null) {
tableView.getColumns().clear();
if (newValue.equals("grade")) {
TableColumn<Student, String> nameColumn = new TableColumn<>("Name");
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
TableColumn<Student, Integer> mathColumn = new TableColumn<>("高数");
mathColumn.setCellValueFactory(new PropertyValueFactory<>("math"));
TableColumn<Student, Integer> englishColumn = new TableColumn<>("大学英语");
englishColumn.setCellValueFactory(new PropertyValueFactory<>("english"));
TableColumn<Student, Integer> physicsColumn = new TableColumn<>("大学物理");
physicsColumn.setCellValueFactory(new PropertyValueFactory<>("physics"));
tableView.getColumns().addAll(nameColumn, mathColumn, englishColumn, physicsColumn);
}else if (newValue.equals("course")){
tableView.getColumns().clear();
TableColumn<Student, String> nameColumn = new TableColumn<>("课程");
nameColumn.setCellValueFactory(new PropertyValueFactory<>("lesson"));
TableColumn<Student, String> mathColumn = new TableColumn<>("老师");
mathColumn.setCellValueFactory(new PropertyValueFactory<>("teacher"));
TableColumn<Student, String > englishColumn = new TableColumn<>("上课地点");
englishColumn.setCellValueFactory(new PropertyValueFactory<>("location"));
TableColumn<Student, String> physicsColumn = new TableColumn<>("学院");
physicsColumn.setCellValueFactory(new PropertyValueFactory<>("major"));
TableColumn<Student, String> timeColumn = new TableColumn<>("上课时间");
timeColumn.setCellValueFactory(new PropertyValueFactory<>("time"));
tableView.getColumns().addAll(nameColumn, mathColumn, englishColumn, physicsColumn,timeColumn);
}else if (newValue.equals("information")){
tableView.getColumns().clear();
TableColumn<Student, String> nameColumn = new TableColumn<>("名称");
nameColumn.setCellValueFactory(new PropertyValueFactory<>("name"));
TableColumn<Student, String> mathColumn = new TableColumn<>("学号");
mathColumn.setCellValueFactory(new PropertyValueFactory<>("number"));
TableColumn<Student, String> genderColumn = new TableColumn<>("性别");
genderColumn.setCellValueFactory(new PropertyValueFactory<>("gender"));
TableColumn<Student, String > englishColumn = new TableColumn<>("班级");
englishColumn.setCellValueFactory(new PropertyValueFactory<>("major"));
TableColumn<Student, Boolean> physicsColumn = new TableColumn<>("是否挂科");
physicsColumn.setCellValueFactory(new PropertyValueFactory<>("fail"));
tableView.getColumns().addAll(nameColumn, mathColumn,genderColumn, englishColumn, physicsColumn);
}loadItems(newValue);//光标在哪一行的名字
// 获取所有的 TableColumn
ObservableList<TableColumn<Student, ?>> columns = tableView.getColumns();
// 遍历所有列并设置列宽
for (TableColumn<Student, ?> column1 : columns) {
column1.setPrefWidth(150); // 将列宽设置为 200 像素
column1.setCellFactory(column->new TableCellWithStyle<>());
}
}
});
HBox top = new HBox(250);
top.setBackground(Background.fill(Color.web("#1f2c30")));
top.setMinHeight(60);
Text text = new Text("Welcome 学生管理系统");
text.setFont(Font.font("宋体", FontWeight.BOLD,32));
text.setFill(Color.WHITE);
Button back =new Button("<<返回登录");
back.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
loginStage.show();
stage.close();
}
});
back.setMinWidth(100); // 设置按钮最小宽度为100像素
back.setMinHeight(40); // 设置按钮最小高度为40像素
back.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
Button tu =new Button("成绩数据图");
tu.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
bing bing=new bing();
bing.bing();
}
});
tu.setMinWidth(100); // 设置按钮最小宽度为100像素
tu.setMinHeight(40); // 设置按钮最小高度为40像素
tu.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
top.setAlignment(Pos.CENTER_LEFT);
// top.setAlignment(Pos.CENTER);
top.getChildren().addAll(back,text,tu);
Group center = new Group();
// center.setBackground(Background.fill(Color.web("#1f2c30")));
// center.setAlignment(Pos.CENTER);
tableView.setLayoutX(50);
tableView.setLayoutY(50);
center.getChildren().add(tableView);
// 默认加载第一个类别的数据
listView.getSelectionModel().select(0);
// HBox root = new HBox(listView, tableView);
// root.setSpacing(100);
BorderPane borderPane = new BorderPane();
borderPane.setBackground(Background.fill(Color.web("#1f2c30")));
borderPane.setBackground(Background.fill(Color.web("#f0ece9")));
borderPane.setLeft(listView);
borderPane.setCenter(center);
borderPane.setTop(top);
Scene scene = new Scene(borderPane, 1100, 699);
stage.setTitle("学生管理系统--学生");
stage.setScene(scene);
stage.show();
}
private void loadItems(String category) {
List<Student> items = studentDao.getStudentBy(category);
tableView.setItems(FXCollections.observableArrayList(items));
}
public static class TableCellWithStyle<T> extends javafx.scene.control.TableCell<Student, T> {
@Override
protected void updateItem(T item, boolean empty) {
super.updateItem(item, empty);
if (item != null) {
setText(item.toString());
setAlignment(Pos.CENTER); // 居中显示
setFont(Font.font("Arial", 18)); // 设置字体大小
} else {
setText(null);
}
}
}
}
这里面的难点就是如何从数据库中的数据加载到tableview这张表上,为什么点击左边不同的listview会显示不同的表:这里面就是给每个listview绑定事件,获取获得焦点的值,然后重新渲染tableview
接下来是这里面调用的studentDao类和饼图类
studentDao:
import com.javafx01.view.Login;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
public class StudentDao {
static String sql;
static ResultSet rs;
public List<Student> getStudentBy(String category) {
// Simulate data fetching from a database or other data source
List<Student> studentDao = new ArrayList<>();
switch (category) {
case "grade":
sql="select * from grade";
rs=null;
try {
PreparedStatement stmt= Login.conn.prepareStatement(sql);
rs=stmt.executeQuery();
while(rs.next()){
String name=rs.getNString(2);
int math=rs.getInt(3);
int english=rs.getInt(4);
int physics=rs.getInt(5);
studentDao.add(new Student(name,math,english,physics));
}
}catch (Exception e) {
throw new RuntimeException(e);
}
studentDao.add(new Student("礼拜天", 0,85,0));
studentDao.add(new Student("李白", 100,100,100));
studentDao.add(new Student("杜甫", 100,100,100));
break;
case "course":
sql="select * from course";
rs=null;
try {
PreparedStatement stmt= Login.conn.prepareStatement(sql);
rs=stmt.executeQuery();
while(rs.next()){
String name=rs.getNString(1);
String teacher=rs.getNString(2);
String location=rs.getNString(3);
String major=rs.getNString(4);
String time =rs.getNString(5);
studentDao.add(new Student(name,teacher,location,major,time));
}
}catch (Exception e) {
throw new RuntimeException(e);
}
studentDao.add(new Student("math","李老师","3号楼","信工","1" ));
studentDao.add(new Student("english","王老师","4号楼","信工","1" ));
studentDao.add(new Student("physics","马老师","5号楼","信工","1" ));
break;
case "information":
sql="select * from users;";
rs=null;
try {
PreparedStatement stmt= Login.conn.prepareStatement(sql);
rs=stmt.executeQuery(sql);
while(rs.next()){
String number=rs.getNString(3);
String name=rs.getNString(4);
String gender =rs.getNString(5);
String major=rs.getNString(6);
studentDao.add(new Student(name,number,gender,major,false));
}
}catch (Exception e) {
System.out.println(1111111111111111L);
throw new RuntimeException(e);
}
studentDao.add(new Student("马离谱","xingong","nan","男",false));
studentDao.add(new Student("马离谱","xingong","nan","男",false));
studentDao.add(new Student("马离谱","xingong","nan","男",false));
break;
}
return studentDao;
}
}
Student:
public class Student {
private String Name;
private String username;
private String password;
private int math;
private int english;
private int physics;
public Student(String name, String username, String password, int math, int english, int physics, String major, String number, String gender) {
Name = name;
this.username = username;
this.password = password;
this.math = math;
this.english = english;
this.physics = physics;
this.major = major;
Number = number;
this.gender = gender;
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
private String lesson;
private String teacher;
private String location;
private String major;
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
public String getTime() {
return time;
}
public void setTime(String time) {
this.time = time;
}
private String Number;
public Student(String name, String number, String gender, String major, boolean fail) {
Name = name;
Number = number;
this.gender = gender;
this.major = major;
this.fail = fail;
}
private String gender;
public Student(String lesson, String teacher, String location, String major, String time) {
this.lesson = lesson;
this.teacher = teacher;
this.location = location;
this.major = major;
this.time = time;
}
private String classly;
private String time;
public String getClassly() {
return classly;
}
public void setClassly(String classly) {
this.classly = classly;
}
private boolean fail;
public Student(String name, int math, int english, int physics) {
Name = name;
this.math = math;
this.english = english;
this.physics = physics;
}
public String getName() {
return Name;
}
public void setName(String name) {
Name = name;
}
public int getMath() {
return math;
}
public void setMath(int math) {
this.math = math;
}
public int getEnglish() {
return english;
}
public void setEnglish(int english) {
this.english = english;
}
public int getPhysics() {
return physics;
}
public void setPhysics(int physics) {
this.physics = physics;
}
public String getLesson() {
return lesson;
}
public void setLesson(String lesson) {
this.lesson = lesson;
}
public String getTeacher() {
return teacher;
}
public void setTeacher(String teacher) {
this.teacher = teacher;
}
public String getLocation() {
return location;
}
public void setLocation(String location) {
this.location = location;
}
public String getMajor() {
return major;
}
public void setMajor(String major) {
this.major = major;
}
public String getNumber() {
return Number;
}
public void setNumber(String number) {
Number = number;
}
public boolean isFail() {
return fail;
}
public void setFail(boolean fail) {
this.fail = fail;
}
}
饼状图:
import com.javafx01.view.Login;
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.EventHandler;
import javafx.scene.Scene;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.scene.chart.*;
import javafx.scene.Group;
import javafx.scene.control.Label;
import javafx.scene.input.MouseEvent;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
public class bing {
static int a=0;
static int b=0;
static int c=0;
static int d=0;
static int e=0;
static int number=0;
public void bing() {
Stage stage =new Stage();
Scene scene = new Scene(new Group());
stage.setTitle("全体学生成绩分析");
stage.setWidth(500);
stage.setHeight(500);
String sql ="SELECT math + english + physics AS total_score FROM grade;";
try {
PreparedStatement stmt= Login.conn.prepareStatement(sql);
ResultSet rs=stmt.executeQuery();
while(rs.next()){
number++;
int temp=rs.getInt(1);
if (temp>250) a++;
else if (temp>220) b++;
else if (temp>180) c++;
else if (temp>90) d++;
else e++;
}
System.out.println(number);
}catch (Exception e) {
throw new RuntimeException(e);
}
ObservableList<PieChart.Data> pieChartData =
FXCollections.observableArrayList(
new PieChart.Data("优秀(250~)", ((double) a /number)*100),
new PieChart.Data("良好(220~250)", ((double) b /number)*100),
new PieChart.Data("及格(180~220)", ((double) c /number)*100),
new PieChart.Data("不及格(90~180)", ((double) d /number)*100),
new PieChart.Data("较差(~90)", ((double) e /number)*100));
final PieChart chart = new PieChart(pieChartData);
chart.setTitle("学生成绩表分析");
final Label caption = new Label("返回");
caption.setTextFill(Color.DARKORANGE);
caption.setStyle("-fx-font: 24 arial;");
for (final PieChart.Data data : chart.getData()) {
data.getNode().addEventHandler(MouseEvent.MOUSE_PRESSED, new EventHandler<MouseEvent>() {
@Override public void handle(MouseEvent e) {
caption.setTranslateX(e.getSceneX());
caption.setTranslateY(e.getSceneY());
caption.setText((String.valueOf(data.getPieValue())) + "%");
caption.setStyle("-fx-font: 32 arial;");
}
});
}
((Group) scene.getRoot()).getChildren().addAll(chart, caption);
stage.setScene(scene);
stage.show();
}
}
5.管理员页面代码
import com.javafx01.Obj.Student;
import com.javafx01.Obj.userDao;
import com.javafx01.view.Login;
import com.javafx01.view.Main;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.control.cell.PropertyValueFactory;
import javafx.scene.control.cell.TextFieldTableCell;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.scene.text.Text;
import javafx.stage.Stage;
import java.util.List;
public class manage {
// private final Stage stage =new Stage();
private ListView<String> listView;
private userDao userDao=new userDao();
private Pagination pagination;
private TableView<Student> table;
private final Stage stage =new Stage();
public manage( Stage LoginStage) {
pagination = new Pagination(10, 0);
pagination.setStyle("-fx-border-color:red;");
pagination.setPageFactory((Integer pageIndex) -> createPage(pageIndex));
AnchorPane anchor = new AnchorPane();
//在anchor布局的四周添加pagination
//使用 AnchorPane 布局,将 Pagination 控件锚定在四个边距上,并设置边距为 10 像素。
AnchorPane.setTopAnchor(pagination, 60.0);
AnchorPane.setRightAnchor(pagination, 60.0);
AnchorPane.setBottomAnchor(pagination, 60.0);
AnchorPane.setLeftAnchor(pagination, 60.0);
anchor.getChildren().addAll(pagination);
HBox boottom=new HBox(80);
boottom.setAlignment(Pos.CENTER);
Button add =new Button("添加");
add.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
userDao.add();
table.refresh();
}
});
Button delete =new Button("删除");
add.setMinWidth(100); // 设置按钮最小宽度为100像素
add.setMinHeight(40); // 设置按钮最小高度为40像素
delete.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
userDao.delete();
table.refresh();
}
});
add.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
delete.setStyle("-fx-text-fill: black;-fx-font-size: 16px;");
delete.setMinWidth(100); // 设置按钮最小宽度为100像素
delete.setMinHeight(40); // 设置按钮最小高度为40像素
Button alter =new Button("修改信息");
alter.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
userDao.alter();
table.refresh();
}
});
Button serch =new Button("查询");
alter.setMinWidth(100); // 设置按钮最小宽度为100像素
alter.setMinHeight(40); // 设置按钮最小高度为40像素
alter.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
Button alterGrand =new Button("修改成绩");
alterGrand.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
userDao.altergrade();
table.refresh();
}
});
alterGrand.setMinWidth(100); // 设置按钮最小宽度为100像素
alterGrand.setMinHeight(40); // 设置按钮最小高度为40像素
alterGrand.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
serch.setStyle("-fx-text-fill: black;-fx-font-size: 16px;");
serch.setMinWidth(100); // 设置按钮最小宽度为100像素
serch.setMinHeight(40); // 设置按钮最小高度为40像素
serch.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
userDao.serch();
table.refresh();
}
});
Button back =new Button("退出登录");
back.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
Login.showLoginSuccessAlert("退出成功","欢迎下次光临哦~~~");
LoginStage.show();
stage.close();
}
});
back.setMinWidth(100); // 设置按钮最小宽度为100像素
back.setMinHeight(40); // 设置按钮最小高度为40像素
back.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
boottom.getChildren().addAll(add,delete,alter,alterGrand,serch,back);
HBox top = new HBox();
top.setBackground(Background.fill(Color.web("#1f2c30")));
boottom.setBackground(Background.fill(Color.web("#1f2c30")));
boottom.setMinHeight(100);
top.setMinHeight(100);
Text text = new Text("Welcome 学生管理系统");
text.setFont(Font.font("宋体", FontWeight.BOLD,32));
text.setFill(Color.WHITE);
top.setAlignment(Pos.CENTER);
top.getChildren().add(text);
BorderPane borderPane = new BorderPane();
borderPane.setBackground(Background.fill(Color.web("#f0ece9")));
borderPane.setTop(top);
borderPane.setCenter(anchor);
borderPane.setBottom(boottom);
Scene scene = new Scene(borderPane, 1100, 699);
stage.setTitle("学生管理系统--管理员");
stage.setScene(scene);
stage.show();
}
public VBox createPage(int pageIndex) {
VBox box = new VBox(5);
table = new TableView<>();
/* ObservableList<Student> data = FXCollections.observableArrayList(
//添加数据
);
table.setItems(data);*/
loadItems( pageIndex);
table.setPlaceholder(new Button("没有数据"));
table.getSelectionModel().setCellSelectionEnabled(true);//只能选中单元格
table.setRowFactory(tv -> {
TableRow<Student> row = new TableRow<>();
row.setMinHeight(50);
return row;
});
TableColumn <Student, String>userNameCol = new TableColumn<>("用户名");
userNameCol.setCellValueFactory(new PropertyValueFactory<>("username"));
TableColumn <Student, String>passwordCol = new TableColumn<>("密码");
passwordCol.setCellValueFactory(new PropertyValueFactory<>("password"));
TableColumn <Student, String>numberCol = new TableColumn<>("学号");
numberCol.setCellValueFactory(new PropertyValueFactory<>("number"));
TableColumn <Student, String>nameCol = new TableColumn<>("姓名");
nameCol.setCellValueFactory(new PropertyValueFactory<>("name"));
TableColumn <Student, String>genderCol = new TableColumn<>("性别");
genderCol.setCellValueFactory(new PropertyValueFactory<>("gender"));
TableColumn<Student, String> majorCol = new TableColumn<>("专业");
majorCol.setCellValueFactory(new PropertyValueFactory<>("major"));
TableColumn <Student, Integer>mathCol = new TableColumn<>("高等数学");
mathCol.setCellValueFactory(new PropertyValueFactory<>("math"));
TableColumn <Student, Integer>englishCol = new TableColumn<>("大学英语");
englishCol.setCellValueFactory(new PropertyValueFactory<>("english"));
TableColumn <Student, Integer>physicsCol = new TableColumn<>("大学物理");
physicsCol.setCellValueFactory(new PropertyValueFactory<>("physics"));
//这个是给每一列设置可以修改数据
userNameCol.setCellFactory(TextFieldTableCell.forTableColumn());
table.getColumns().addAll(userNameCol,passwordCol,numberCol,nameCol,genderCol,majorCol,mathCol,englishCol,physicsCol);
ObservableList<TableColumn<Student, ?>> columns = table.getColumns();
for (TableColumn<Student, ?> column1 : columns) {
column1.setPrefWidth(110); // 将列宽设置为 200 像素
column1.setCellFactory(column->new Main.TableCellWithStyle<>());
// column1.setCellFactory(TextFieldTableCell.forTableColumn());
}
// 降序 firstNameCol.setSortType(TableColumn.SortType.DESCENDING);
// 不可排序 lastNameCol.setSortable(false);
box.setSpacing(5);
box.setPadding(new Insets(10, 0, 0, 10));
box.getChildren().addAll(table);
return box;
}
private void loadItems(int pageIndex) {
List<Student> items = userDao.getStudentBy();
int fromIndex = pageIndex * 4;
int toIndex = Math.min(fromIndex +4, items.size());
table.setItems(FXCollections.observableArrayList(items.subList(fromIndex,toIndex)));
}
}
这个代码主要难点实现就是如何对表视图就行分页操作,以及下面按钮的增删改查操作,下面是这个类调用的类
userDao:
import com.javafx01.view.Login;
import javafx.collections.FXCollections;
import javafx.collections.ObservableList;
import javafx.event.ActionEvent;
import javafx.event.EventHandler;
import javafx.geometry.Insets;
import javafx.geometry.Pos;
import javafx.scene.Group;
import javafx.scene.Scene;
import javafx.scene.control.*;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.*;
import javafx.scene.paint.Color;
import javafx.scene.text.Font;
import javafx.scene.text.FontWeight;
import javafx.stage.Stage;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
public class userDao {
static String sql;
static ResultSet rs;
Stage stage;
Scene scene;
Image bgimg=new Image("file:///D:/作品/bilibili.xiazai/表单/image/req.jpg");
ImageView bgimageView =new ImageView(bgimg);
StackPane root;
public List<Student> getStudentBy() {
// Simulate data fetching from a database or other data source
List<Student> userdao = new ArrayList<>();
//多表查询
sql="select u.* , g.math,g.english,g.physics from users u left outer join grade g on u.username=g.username;";
rs=null;
try {
PreparedStatement stmt= Login.conn.prepareStatement(sql);
rs=stmt.executeQuery();
while(rs.next()){
String name=rs.getNString(4);
String username=rs.getNString(1);
String password=rs.getNString(2);
int math=rs.getInt(7);
int english=rs.getInt(8);
int physics=rs.getInt(9);
String major=rs.getNString(6);
String number=rs.getNString(3);
String gender=rs.getNString(5);
userdao.add(new Student(name,username,password,math,english,physics,major,number,gender));
}
}catch (Exception e) {
throw new RuntimeException(e);
}
return userdao;
}
public void add(){
stage=new Stage();
root=new StackPane();
root.getChildren().add(0, bgimageView);
stage.setTitle("学生管理系统--管理员操作");
GridPane grid=new GridPane();
grid.setAlignment(Pos.CENTER);
Label userName =new Label("新建用户名:");
userName.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
userName.setTextFill(Color.WHITE);
grid.add(userName,0,2);
TextField userTextFild =new TextField();
userTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
userTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(userTextFild,1,2);
Label pw =new Label(" 密码:");
pw.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
pw.setTextFill(Color.WHITE);
grid.add(pw,0,3);
PasswordField pwBox =new PasswordField();
pwBox.setPrefWidth(150);
pwBox.setPrefHeight(30);
grid.add(pwBox,1,3);
Label npw =new Label(" 确认密码:");
npw.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
npw.setTextFill(Color.WHITE);
grid.add(npw,0,4);
PasswordField npwBox =new PasswordField();
npwBox.setPrefWidth(150);
npwBox.setPrefHeight(30);
grid.add(npwBox,1,4);
//-------------------------------------------
Label numberL =new Label(" 学号:");
numberL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
numberL.setTextFill(Color.WHITE);
grid.add(numberL,0,5);
TextField numberTextFild =new TextField();
numberTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
numberTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(numberTextFild,1,5);
//------------------------
Label nameL =new Label(" 姓名:");
nameL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
nameL.setTextFill(Color.WHITE);
grid.add(nameL,0,6);
TextField nameTextFild =new TextField();
nameTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
nameTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(nameTextFild,1,6);
Label majorL =new Label(" 专业:");
majorL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
majorL.setTextFill(Color.WHITE);
grid.add(majorL,0,7);
TextField majorTextFild =new TextField();
majorTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
majorTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(majorTextFild,1,7);
Button btn =new Button("添加");
btn.setMinWidth(100); // 设置按钮最小宽度为100像素
btn.setMinHeight(40); // 设置按钮最小高度为40像素
btn.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent actionEvent) {
String username=userTextFild.getText();
String pwd=pwBox.getText();
String npwd=npwBox.getText();
String number=numberTextFild.getText();
String name=nameTextFild.getText();
String major= majorTextFild.getText();
if (username.isEmpty()||pwd.isEmpty()||npwd.isEmpty()||number.isEmpty()||name.isEmpty()|| major.isEmpty()){
Login.showLoginFailureAlert("注册失败","输入框存在空白");
}else {
if (!pwd.equals(npwd)){
Login.showLoginFailureAlert("注册失败","两次密码输入的不相同");
}else {
sql="INSERT INTO grade (number,name,math,english,physics,username) values (?,?,?,?,?,?)";
try {
PreparedStatement stmt=Login.conn.prepareStatement(sql);
stmt.setString(1, number);
stmt.setString(2, name);
stmt.setInt(3,0);
stmt.setInt(4,0);
stmt.setInt(5,0);
stmt.setString(6, username);
int t = stmt.executeUpdate();
} catch (SQLException e) {
if (e.getErrorCode() == 1062) { // 1062 错误码表示违反了唯一约束
// 处理重复 username 的情况
Login.showLoginFailureAlert("添加失败", "该学号或用户名已经存在,请检查后再注册哦~");
} else {
Login.showLoginFailureAlert("添加失败", "未知错误,请稍后再尝试,grande表出现异常");
}
throw new RuntimeException(e);
}
sql = "INSERT INTO users (username, password, member, name, gender, major) VALUES (?, ?, ?, ?, ?, ?)";
try {
PreparedStatement stmt=Login.conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, pwd);
stmt.setString(3, number);
stmt.setString(4, name);
stmt.setString(5, "男");
stmt.setString(6, major);
int rs = stmt.executeUpdate();
Login.showLoginSuccessAlert("添加成功", "请牢记添加用户的账号和密码");
stage.close();
// stage1.show();
} catch (Exception e) {
Login.showLoginFailureAlert("注册失败","未知错误,请稍后再尝试");
throw new RuntimeException(e);
}
}
}
}
});
HBox hbBtn=new HBox(50);
hbBtn.setAlignment(Pos.CENTER);
hbBtn.getChildren().addAll(btn);
grid.add(hbBtn,0,9,2,1);
grid.setHgap(20);
grid.setVgap(20);
root.getChildren().add(1,grid);
Scene scene = new Scene(root,800,500);
stage.setScene(scene);
stage.show();
}
public void delete(){
stage=new Stage();
root=new StackPane();
root.getChildren().add(0, bgimageView);
stage.setTitle("学生管理系统--管理员操作");
GridPane grid=new GridPane();
grid.setAlignment(Pos.CENTER);
Label userName =new Label("请输入你要删除的用户名");
userName.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
userName.setTextFill(Color.WHITE);
grid.add(userName,0,2);
TextField userTextFild =new TextField();
userTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
userTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(userTextFild,1,2);
Label numberL =new Label(" 请输入你要删除的学号");
numberL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
numberL.setTextFill(Color.WHITE);
grid.add(numberL,0,5);
TextField numberTextFild =new TextField();
numberTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
numberTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(numberTextFild,1,5);
Button btn =new Button("删除");
btn.setMinWidth(100); // 设置按钮最小宽度为100像素
btn.setMinHeight(40); // 设置按钮最小高度为40像素
btn.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
HBox hbBtn=new HBox(50);
hbBtn.setAlignment(Pos.CENTER);
hbBtn.getChildren().addAll(btn);
grid.add(hbBtn,0,9,2,1);
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
String username=userTextFild.getText();
String number=numberTextFild.getText();
if (username.isEmpty()||number.isEmpty()) Login.showLoginFailureAlert("修改失败","输入框存在空白");
else {
sql="delete from users where username=? and member=?";
try {
PreparedStatement stmt=Login.conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, number);
int rs = stmt.executeUpdate();
} catch (Exception e) {
Login.showLoginFailureAlert("删除失败","未知错误,请稍后再尝试,表出现异常");
throw new RuntimeException(e);
}
sql="delete from grade where username=? and number=?";
try {
PreparedStatement stmt=Login.conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, number);
int rs = stmt.executeUpdate();
if(rs==0){
Login.showLoginFailureAlert("删除失败","请检查你输入的用户名和学号,没有查询到该用户");
}else {
Login.showLoginSuccessAlert("删除成功", "请通知被 t 出去的学生");
stage.close();
}
} catch (Exception e) {
Login.showLoginFailureAlert("删除失败","未知错误,请稍后再尝试,表出现异常");
throw new RuntimeException(e);
}
}
}
});
grid.setHgap(20);
grid.setVgap(20);
root.getChildren().add(1,grid);
Scene scene = new Scene(root,800,500);
stage.setScene(scene);
stage.show();
}
public void alter(){
stage=new Stage();
root=new StackPane();
root.getChildren().add(0, bgimageView);
stage.setTitle("学生管理系统--管理员操作");
GridPane grid=new GridPane();
grid.setAlignment(Pos.CENTER);
Label userName =new Label("请输入你要修改的用户名");
userName.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
userName.setTextFill(Color.WHITE);
grid.add(userName,0,2);
TextField userTextFild =new TextField();
userTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
userTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(userTextFild,1,2);
Label pw =new Label(" 修改为新密码:");
pw.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
pw.setTextFill(Color.WHITE);
grid.add(pw,0,3);
PasswordField pwBox =new PasswordField();
pwBox.setPrefWidth(150);
pwBox.setPrefHeight(30);
grid.add(pwBox,1,3);
Label nameL =new Label(" 修改的新姓名:");
nameL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
nameL.setTextFill(Color.WHITE);
grid.add(nameL,0,6);
TextField nameTextFild =new TextField();
nameTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
nameTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(nameTextFild,1,6);
Label majorL =new Label(" 修改的新专业:");
majorL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
majorL.setTextFill(Color.WHITE);
grid.add(majorL,0,7);
TextField majorTextFild =new TextField();
majorTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
majorTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(majorTextFild,1,7);
Button btn =new Button("修改");
btn.setMinWidth(100); // 设置按钮最小宽度为100像素
btn.setMinHeight(40); // 设置按钮最小高度为40像素
btn.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
HBox hbBtn=new HBox(50);
hbBtn.setAlignment(Pos.CENTER);
hbBtn.getChildren().addAll(btn);
grid.add(hbBtn,0,9,2,1);
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
String username =userTextFild.getText();
String pwd=pwBox.getText();
String name=nameTextFild.getText();
String major= majorTextFild.getText();
if (username.isEmpty()||pwd.isEmpty()||name.isEmpty()|| major.isEmpty()){
Login.showLoginFailureAlert("修改失败","输入框存在空白");
}else {
sql = "update users set password=?,name=?,major=? where username=?;";
try {
PreparedStatement stmt=Login.conn.prepareStatement(sql);
stmt.setString(1, pwd);
stmt.setString(2, name);
stmt.setString(3, major);
stmt.setString(4, username);
int rs = stmt.executeUpdate();
if (rs==0){
Login.showLoginFailureAlert("修改失败","不存在该用户,请不要胡闹呢");
}else {
Login.showLoginSuccessAlert("修改成功", "请通知学生哦~~~");
stage.close();
}
} catch (Exception e) {
Login.showLoginFailureAlert("修改失败","未知错误,请稍后再尝试");
throw new RuntimeException(e);
}
}
}
});
grid.setHgap(20);
grid.setVgap(20);
root.getChildren().add(1,grid);
Scene scene = new Scene(root,800,500);
stage.setScene(scene);
stage.show();
}
public void altergrade(){
stage=new Stage();
root=new StackPane();
root.getChildren().add(0, bgimageView);
stage.setTitle("学生管理系统--管理员操作");
GridPane grid=new GridPane();
grid.setAlignment(Pos.CENTER);
Label userName =new Label("请输入你要修改的用户名");
userName.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
userName.setTextFill(Color.WHITE);
grid.add(userName,0,2);
TextField userTextFild =new TextField();
userTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
userTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(userTextFild,1,2);
Label mathL =new Label(" 修改高数成绩为:");
mathL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
mathL.setTextFill(Color.WHITE);
grid.add(mathL,0,3);
TextField mathTextFild =new TextField();
mathTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
mathTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(mathTextFild,1,3);
Label englishL =new Label(" 修改大英成绩为:");
englishL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
englishL.setTextFill(Color.WHITE);
grid.add(englishL,0,4);
TextField englishTextFild =new TextField();
englishTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
englishTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(englishTextFild,1,4);
Label physicsL =new Label(" 修改大物成绩为:");
physicsL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
physicsL.setTextFill(Color.WHITE);
grid.add(physicsL,0,5);
TextField physicsTextFild =new TextField();
physicsTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
physicsTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(physicsTextFild,1,5);
Button btn =new Button("修改");
btn.setMinWidth(100); // 设置按钮最小宽度为100像素
btn.setMinHeight(40); // 设置按钮最小高度为40像素
btn.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
HBox hbBtn=new HBox(50);
hbBtn.setAlignment(Pos.CENTER);
hbBtn.getChildren().addAll(btn);
grid.add(hbBtn,0,9,2,1);
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
String username =userTextFild.getText();
try {
int math=Integer.parseInt(mathTextFild.getText());
int english=Integer.parseInt(mathTextFild.getText());
int physics=Integer.parseInt(mathTextFild.getText());
// 继续进行操作
if (username.isEmpty()||mathTextFild.getText().isEmpty()||englishTextFild.getText().isEmpty()||physicsTextFild.getText().isEmpty()){
Login.showLoginFailureAlert("修改失败","输入框存在空白");
}else {
sql = "update grade set math=?,english=?,physics=? where username=?;";
try {
PreparedStatement stmt=Login.conn.prepareStatement(sql);
stmt.setInt(1, math);
stmt.setInt(2, english);
stmt.setInt(3, physics);
stmt.setString(4, username);
int rs = stmt.executeUpdate();
if (rs==0){
Login.showLoginFailureAlert("修改失败","不存在该用户,请不要胡闹呢");
}else {
Login.showLoginSuccessAlert("修改成功", "请提醒学生查看有没有挂科哦~~~");
stage.close();
}
} catch (Exception e) {
Login.showLoginFailureAlert("修改失败","未知错误,请稍后再尝试");
throw new RuntimeException(e);
}
}
} catch (NumberFormatException e) {
Login.showLoginFailureAlert("修改失败","请输入合法的数字");
}
}
});
grid.setHgap(20);
grid.setVgap(20);
root.getChildren().add(1,grid);
Scene scene = new Scene(root,800,500);
stage.setScene(scene);
stage.show();
}
public void serch(){
stage=new Stage();
root=new StackPane();
root.getChildren().add(0, bgimageView);
stage.setTitle("学生管理系统--管理员操作");
GridPane grid=new GridPane();
grid.setAlignment(Pos.CENTER);
Label userName =new Label("请输入你要查询的用户名");
userName.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
userName.setTextFill(Color.WHITE);
grid.add(userName,0,2);
TextField userTextFild =new TextField();
userTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
userTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(userTextFild,1,2);
Label numberL =new Label(" 请输入你要查询的学号");
numberL.setFont(Font.font("Thoma", FontWeight.BOLD, 26)); // 设置字体大小为18, 加粗
numberL.setTextFill(Color.WHITE);
grid.add(numberL,0,5);
TextField numberTextFild =new TextField();
numberTextFild.setPrefWidth(150); // 设置文本框宽度为200像素
numberTextFild.setPrefHeight(30); // 设置文本框高度为30像素
grid.add(numberTextFild,1,5);
Button btn =new Button("查询");
btn.setMinWidth(100); // 设置按钮最小宽度为100像素
btn.setMinHeight(40); // 设置按钮最小高度为40像素
btn.setStyle(" -fx-text-fill: black;-fx-font-size: 16px;");
HBox hbBtn=new HBox(50);
hbBtn.setAlignment(Pos.CENTER);
hbBtn.getChildren().addAll(btn);
grid.add(hbBtn,0,9,2,1);
btn.setOnAction(new EventHandler<ActionEvent>() {
@Override
public void handle(ActionEvent event) {
String username=userTextFild.getText();
String number=numberTextFild.getText();
if (username.isEmpty()||number.isEmpty()) Login.showLoginFailureAlert("修改失败","输入框存在空白");
else {
sql="select u.* , g.math,g.english,g.physics from users u left outer join grade g on u.username=g.username where u.username=? and u.member=? ";
try {
PreparedStatement stmt = Login.conn.prepareStatement(sql);
stmt.setString(1, username);
stmt.setString(2, number);
rs = stmt.executeQuery();
System.out.println(rs.getFetchSize());
Stage qstage = new Stage();
StackPane qroot = new StackPane();
qroot.getChildren().add(0, bgimageView);
stage.setTitle("学生管理系统--查询结果展示");
Group group = new Group();
ObservableList<String> data = FXCollections.observableArrayList();
ListView<String> listView = new ListView<>();
listView.setPrefSize(300, 460);
System.out.println(rs.getFetchSize());
boolean flag=false;
while (rs.next()) {
flag=true;
String name = rs.getNString(4);
username = rs.getNString(1);
String password = rs.getNString(2);
int math = rs.getInt(7);
int english = rs.getInt(8);
int physics = rs.getInt(9);
String major = rs.getNString(6);
number = rs.getNString(3);
String gender = rs.getNString(5);
data.addAll("用户名: " + username, "密码: " + password, "学号是: " + number, "姓名是: " + name, "性别是: " + gender, "专业是: " + major, "高数成绩: " + String.valueOf(math), "大学英语成绩: " + String.valueOf(english), "大学物理成绩: " + String.valueOf(physics));
}
if (flag) {
listView.setItems(data);
listView.setCellFactory(param -> new ListCell<>() {
@Override
protected void updateItem(String item, boolean empty) {
super.updateItem(item, empty);
if (empty || item == null) {
setText(null);
setGraphic(null);
} else {
setText(item);
setFont(Font.font("Arial", FontWeight.BOLD, 22));
setAlignment(Pos.CENTER);
setBackground(new Background(new BackgroundFill(Color.YELLOW, CornerRadii.EMPTY, Insets.EMPTY)));
setPrefHeight(50); // 设置每一行的高度为 50 像素
}
}
});
listView.setLayoutX(30);
listView.setLayoutY(50);
group.getChildren().add(listView);
qroot.getChildren().add(1, group);
Scene qscene = new Scene(qroot, 800, 500);
qstage.setScene(qscene);
qstage.show();
// Login.showLoginSuccessAlert("查询成功", "请通知被 t 出去的学生");
stage.close();
}else {
stage.close();
Login.showLoginFailureAlert("查询失败","不存在该用户,请检查后再查询");
}
} catch (Exception e) {
System.out.println(2222);
Login.showLoginFailureAlert("查询失败","未知错误,请稍后再尝试,表出现异常");
throw new RuntimeException(e);
}
}
}
});
grid.setHgap(20);
grid.setVgap(20);
root.getChildren().add(1,grid);
Scene scene = new Scene(root,800,500);
stage.setScene(scene);
stage.show();
}
}
这写代码就是对增删改查操作进行封装,都会新建一个场景,不管从哪个类调用都可以的,如果以后进行大项目操作可以直接调用,比较省事
四.数据库中的表:
以上就是我的学生管理系统全部部分了,希望对大家在成为程序员的道路上有所帮助