fixture标志传参
阅读目录:
特点
示例
特点:
-
采用pytest.mark.XXX(参数)标志所需要的参数,然后在fixture中可以做一些逻辑处理 -
fixture采用reuqest获取参数 -
传参的个数可以是多个,类型可以为简单类型或复杂对象
示例
简单类型
#! usr/bin/env python
# _*_ coding: utf-8 _*_
# @Author: zcs
# @wx: M_Haynes
# @Blog:
# 备注: pytest_框架(6) -- fixture作用域 scope 详解
import pytest
@pytest.fixture()
def setup_down(request):
marker = request.node.get_closest_marker("canshu")
if marker is None:
data = None
else:
data = marker.args[0] + 1
return data
# 有 canshu 标记的情况:
@pytest.mark.canshu(1)
def test_data(setup_down):
print("setup_down={}".format(setup_down))
# 无 canshu 标记的情况:
def test_data2(setup_down):
print("setup_down={}".format(setup_down))
if __name__ == '__main__':
pytest.main(['-vs'])
结果: 实行test用例时,调用@pytest.mark.canshu
并传入参数,实现fixture
复杂类型
也可以是其他类型, 比如列表
## 参数为复杂的类型 -- 列表
@pytest.fixture()
def setup_down_list(request):
lists = request.node.get_closest_marker("list")
if lists is None:
data = None
else:
print("data的值是:", lists.args[0])
data = lists.args[0]
data[-1] -= 1
print("data1={}".format(data))
return data
# 有list的情况
@pytest.mark.list([1,2,3,4,5]) ## 注意:这个参数传进的是元组包含着列表 --- ([1,2,3,4,5],)
def test_list1(setup_down_list):
print("setup_down_list={}".format(setup_down_list))
# 没有list的情况
def test_list2(setup_down_list):
print("setup_down_list={}".format(setup_down_list))
if __name__ == '__main__':
pytest.main(['-vs'])
结果: 传入的参数是以元组包含列表的形式传入进行,所以参数操作时,需要注意解析
传入参数是字典
## 参数为复杂的类型 -- 字典
@pytest.fixture()
def setup_down_dict(request):
dicts = request.node.get_closest_marker("dict")
if dicts is None:
data = None
else:
data = dicts.args[0]
print("类型是:{}".format(type(data)))
print(" data_value:{}".format(data['key']))
data['key'] -= 1
print("data1={}".format(data))
return data
# 有list的情况
@pytest.mark.dict({"key": 1, "value": 2}) ## 注意:这个参数传进的是元组包含着字典 -- ({"key": 1, "value": 2},)
def test_list1(setup_down_dict):
print("setup_down_list={}".format(setup_down_dict))
# 没有list的情况
def test_list2(setup_down_dict):
print("setup_down_list={}".format(setup_down_dict))
if __name__ == '__main__':
pytest.main(['-vs'])
结果:
可以传多个
## 参数为复杂的类型 -- 多个
@pytest.fixture()
def setup_down_more(request):
more_request_1 = request.node.get_closest_marker('list')
more_request_2 = request.node.get_closest_marker('dict')
if more_request_1 is None:
more_request_1 = None
else:
more_request_1 = more_request_1.args[0]
more_request_1[-1] = 999
if more_request_2 is None:
more_request_2 = None
else:
more_request_2 = more_request_2.args[0]
more_request_2['key'] = '修改后的参数'
return more_request_1 , more_request_2
## 有多个参数传入的情况下
@pytest.mark.list([1.2,3])
@pytest.mark.dict({'key':1, 'value': '2'})
def test_more(setup_down_more):
print("setup_down_more:{}".format(setup_down_more))
def test_more2(setup_down_more):
print("setup_down_more2:{}".format(setup_down_more))
结果:
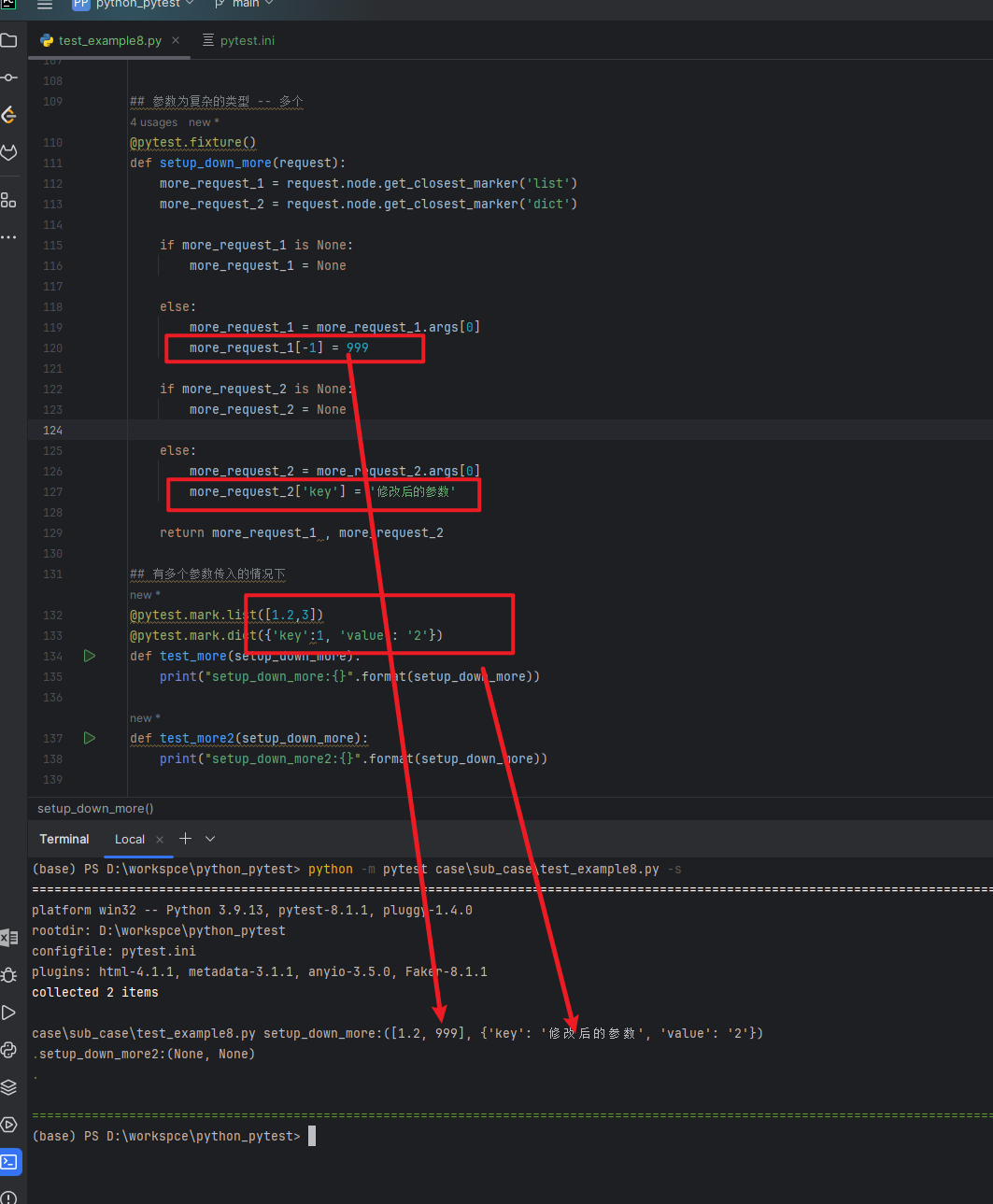
补充:
命令执行, 需要在pytest.ini文件中加配置:
[pytest]
markers =
canshu
list
dict
结果:
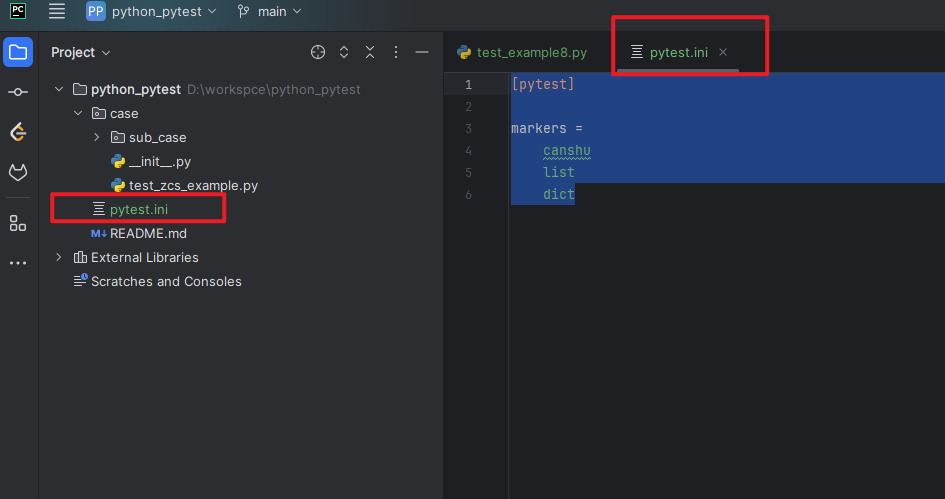
括展: pytest.ini文件
介绍:
pytest.ini 文件时用于配置pytest测试框架的一个配置文件,通过这个文件,可以自定义和控制pytest的行为, 设置各种选项,扩展和插件等 (创建时,在根目录下 创建pytest.ini 文件, 文本格式类型
基本结构:
[pytest]
# 配置选项在这里写
常用配置选项
-
addopts:
是一个常用的选项,可以在这里添加默认的命令行选项,如: 想要默认启用详细模式-v
:
[pytest]
addopts = -v
-
testpaths
: 指定默认测试路径,若你的测试文件都放在 tests 目录下,可以这样写:
[pytest]
testpaths = tests
-
norecursedirs
: 指定pytest不要递归查找测试的目录,如 不想测试venv
和build
目录
[pytest]
norecursedirs = venv build
-
pytest_files
: 指定那些文件名模式的文件应该被认为是测试文件, 如:
[pytest]
python_files = test_*py *_test.py
-
pytest_classes
: 指定哪些类名模式的类应该被认为是测试类,如:
[pytest]
python_classes = Tests* *Tests
-
python_functions
: 指定哪些函数名模式的函数应该被认为是测试函数,如:
[pytest]
python_function = test_* *_test
使用插件
pytest 提供了很多的插件,可以通过pytest.ini
文件进行配置,如: 使用pytest - cov
插件来生成测试覆盖率报告:
[pytest]
addopts = -- cov = my_package --cov-report=html
禁用警告
可以通过配置文件来禁用某些警告,如:
[pytest]
filterwarnings =
ignore:: DeprecationWarning
示例:
有一个 Python 项目,目录结构如下:
my_project/
│
├── my_package/
│ └── __init__.py
│ └── module.py
│
├── tests/
│ └── test_module.py
│
├── pytest.ini
└── requirements.txt
pytest.ini
文件内容如下:
[pytest]
addopts = -v --maxfail=3
testpaths = tests
norecursedirs = venv build
python_files = test_*.py *_test.py
python_classes = Test* *Tests
python_functions = test_* *_test
** 解释示例配置:**
addopts = -v --maxfail=3:pytest 将以详细模式运行,并且在失败3次测试后停止测试。
testpaths = tests:pytest 将在 tests 目录下查找测试。
norecursedirs = venv build:pytest 不会在 venv 和 build 目录下递归查找测试。
python_files = test_*.py *_test.py:pytest 将识别 test_*.py 和 *_test.py 格式的文件作为测试文件。
python_classes = Test* *Tests:pytest 将识别以 Test 开头或以 Tests 结尾的类作为测试类。
python_functions = test_* *_test:pytest 将识别以 test_ 开头或以 _test 结尾的函数作为测试函数。
本文由 mdnice 多平台发布