pytest
使用规则:
1、测试文件名必须以“test_”开头或者"_test"结尾(如:test_ab.py)
2、测试方法必须以“test_”开头。
3、测试类命名以"Test"开头。
运行方法:
在控制台直接输入pytest,会测试所有符合规矩的测试文件
在控制台中输入pytest\文件夹名,会执行该文件中所有的测试用例;在文件夹名后再加上文档名,可以执行该测试文档
进行pytest.ini的配置
[pytest]
testpaths=./文件夹名#用于执行该文件夹中的所有测试用例
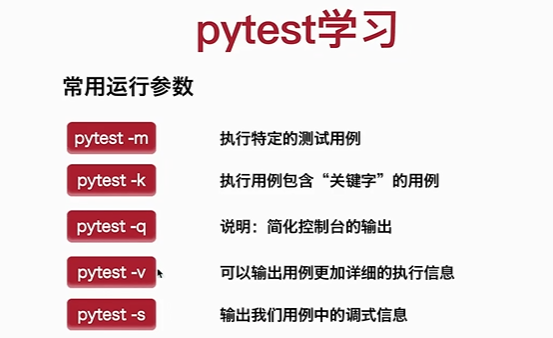
使用标签
@pytest.mark.标签名
在配置环境中
[pytest]
testpaths=./文件夹名#用于执行该文件夹中的所有测试用例
markers=
p0:最高优先级
test:测试环境
pro:生产环境
pytest -m '标签名'
pytest -k '关键词' 根据关键词来匹配测试
pytest.ini配置
addopts配置参数addopts=-q/-v与图片上的作用相同
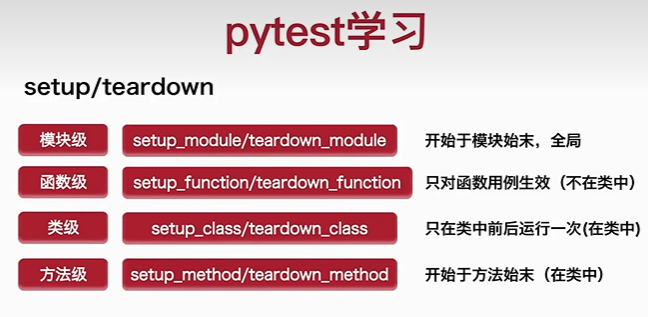

模块级的可以自主定义,也可以在主函数中使用
函数级是每个测试函数都会执行一遍
类级是在每个类的前后出现一次
方法级时每个方法都会出现
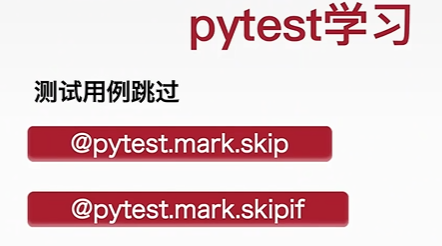
skipif可以加判断语句,判断条件为Ture就可以跳过
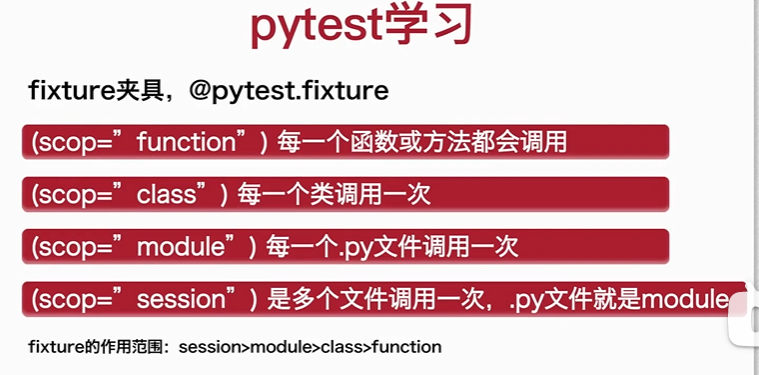
yield返回迭代对象,前面都是前置语句,后面都是后置。
YAML学习
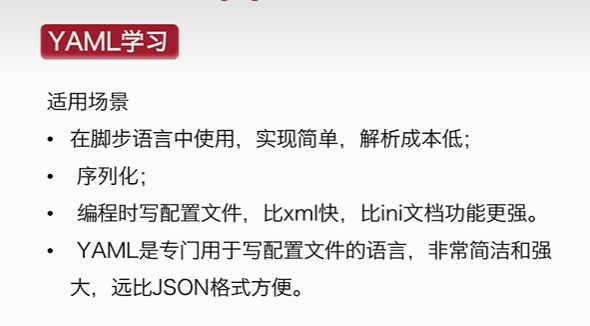
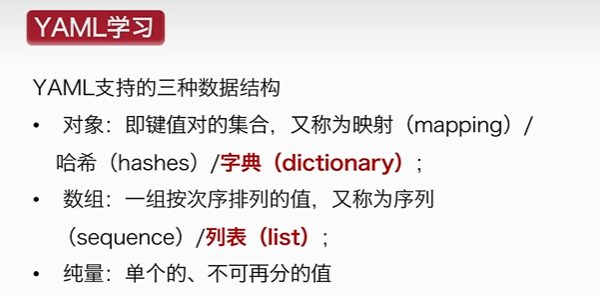
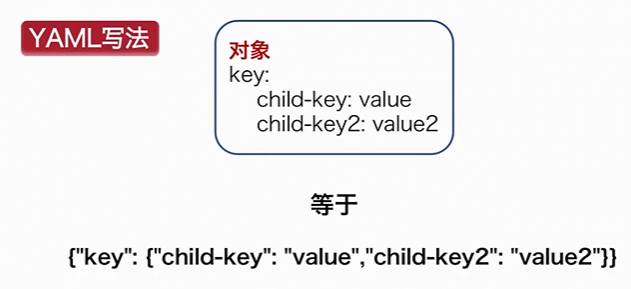
hero:
name: 安琪拉
word: 火焰是我最喜欢的玩具
Hp: 445.5
#字典形式,与上面的相同
hero:{ name: 安琪拉, word: 火焰是我最喜欢的玩具, Hp: 445.5 }
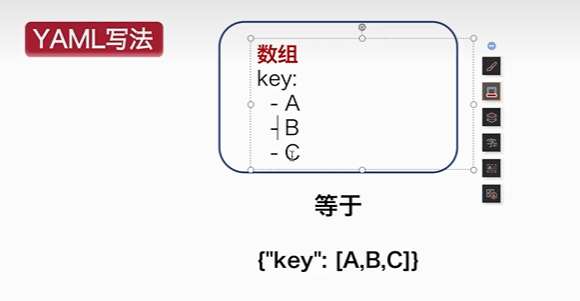
heros_name:
- 安琪拉
- 黄忠
- 小乔
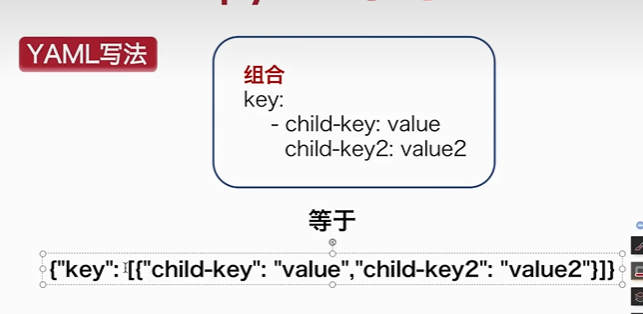
heros:
- name: 黄忠
word: 周日被我射熄火了
Hp: 440
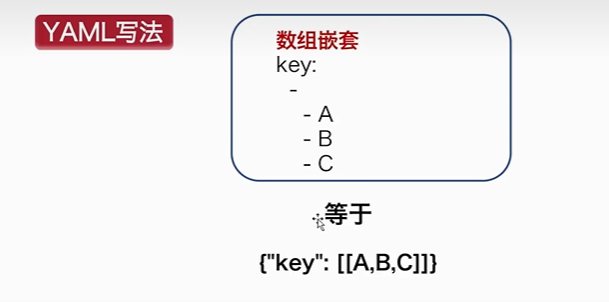
heros_name_list:
- - 安琪拉
- 黄忠
- 小乔
import yaml
f=open("../config/data.yaml",encoding="utf-8")
# 读取文件
data=yaml.safe_load(f)
print(data['hero'])
print(data['heros_name'])
print(data['heros'])
print(data['heros_name_list'])
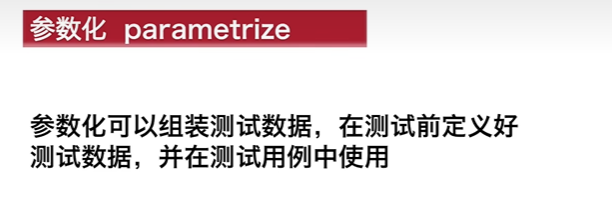
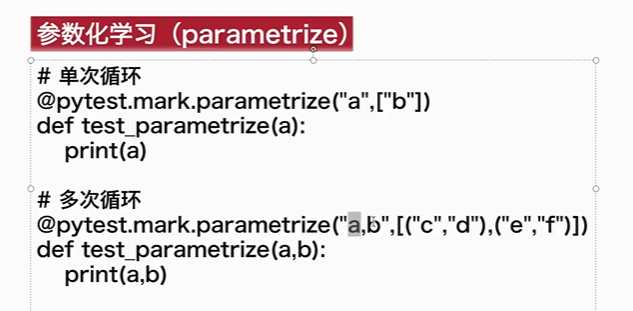
import pytest
#单次循环
# @pytest.mark.parametrize(key,values)
# value要用数组
@pytest.mark.parametrize("key",["value"])
def test_parametriza_01(key):
# 其实是获取key后的值
print("我是"+key)
# 数组中有三项会运行三次,一个参数多个值,测试用例会把每个值赋给参数,进行测试用例执行
@pytest.mark.parametrize("key",["value","nihao","lizhi"])
def test_parametriza_01(key):
assert key=="value"
import pytest
# 多次循环
# 数组
@pytest.mark.parametrize("name,word",[["安琪拉","火焰是我最喜欢的玩具"],["黄忠","周日被我射熄火了"]])
def test_parametrize_02(name,word):
print(name)
print(word)
print(f'{name}的台词是{word}')
# 元组
@pytest.mark.parametrize("name,word",[("安琪拉","火焰是我最喜欢的玩具"),("黄忠","周日被我射熄火了")])
def test_parametrize_02(name,word):
print(name)
print(word)
print(f'{name}的台词是{word}')
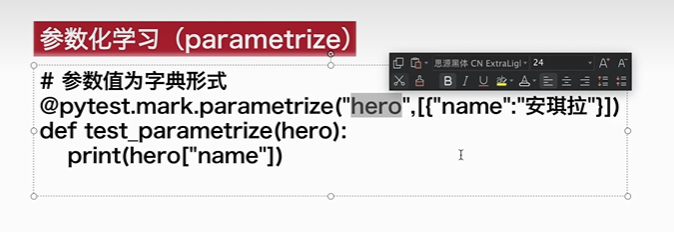
#字典形式,参数值为字典
@pytest.mark.parametrize("hero",[{"name":"安琪拉","word":"火焰是我最喜欢的玩具"},{"name":"黄忠","word":"周日被我射熄火了"}])
def test_parametrize_02(hero):
print(hero["name"])
print(hero["word"])
data.yaml
mobile_belong: {shouji: 13689028881, appkey: 0c818521d38759e1}
mobile_belong_post:
- - 13689028881
- 0c818521d38759e1
mobile_belong_get:
- [13689028881,0c818521d38759e1]
- [13000000000,0c818521d38759e1]
read_data.py
import yaml
def read_data():
f = open("D:\pycharm\pythonproject\ApiTest\config\data.yaml", encoding="utf-8")
data = yaml.safe_load(f)
print(data["mobile_belong_post"])
return data
get_data=read_data()
test_mobile_yaml.py
import requests
import pytest
from utils.read_data import get_data
# 方法一
# def test_mobile():
# param = get_data["mobile_belong"]
# print(param)
# # 将requests和pytest一起使用
# r = requests.get("http://sellshop.5istudy.online/sell/shouji/query",
# params={"shouji": param["shouji"], "appkey": param["appkey"]})
# assert r.status_code == 200
# result = r.json()
# assert result["status"] == 0
# assert result["result"]["province"] == "北京"
# 方法二
# @pytest.mark.parametrize("mobile,appkey",get_data["mobile_belong_post"])
# def test_mobile_02(mobile,appkey):
# # param = get_data["mobile_belong"]
# # print(param)
# # 将requests和pytest一起使用
# r = requests.get("http://sellshop.5istudy.online/sell/shouji/query",
# params={"shouji": mobile, "appkey": appkey})
# assert r.status_code == 200
# result = r.json()
# assert result["status"] == 0
# assert result["result"]["province"] == "北京"
#方法三,验证不同手机号
@pytest.mark.parametrize("mobile,appkey",get_data["mobile_belong_get"])
def test_mobile_02(mobile,appkey):
# param = get_data["mobile_belong"]
# print(param)
# 将requests和pytest一起使用
r = requests.get("http://sellshop.5istudy.online/sell/shouji/query",
params={"shouji": mobile, "appkey": appkey})
assert r.status_code == 200
result = r.json()
assert result["status"] == 0
assert result["result"]["province"] == "北京"
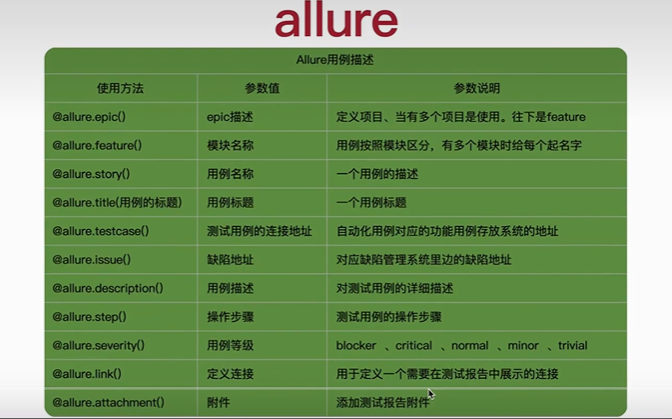