文章目录
一、属性动画
(一)、定义
官网对于属性动画的定义如下:
组件的某些通用属性变化时,可以通过属性动画实现渐变过渡效果,提升用户体验。支持的属性包括width、height、backgroundColor、opacity、scale、rotate、translate等
个人理解:属性动画针对的是同一个组件的属性变化情况,如在同一个页面中触发了组件的属性变化,这个变化需要以过渡的方式体现,这就需要属性动画来承接这个过渡过程,不然变化就会显得十分突兀与生硬。因此,只要组件的指定属性有变化,那么这个变化就会通过属性动画来体现。
(二)、使用方式
使用属性动画,通过animation
属性来为每个组件定义该组件对应的属性动画,如下所示:
animation(
value: {
duration?: number,
tempo?: number,
curve?: string | Curve | ICurve,
delay?:number,
iterations: number,
playMode?: PlayMode,
onFinish?: () => void
}
)
其中的每个参数都会改变属性动画的展现情况,
- duration:设置动画时长,默认值为1000ms,如果设置了浮点值则向下取整;
- tempo:动画播放速度,默认值为1,数值越大,动画播放速度越快。值为0时,表示不存在动画;
- curve:设置动画的播放曲线,作为小白我粗略地理解为动画不同阶段的播放快慢,可以通过Curve来指定动画曲线,如动画快速进入慢速退出,动画快速进入快速退出;
- delay:设置动画延迟执行的时长,默认值为0;
- iterations:设置播放次数,默认为1,设置为-1时表示无限次播放;
- playMode:设置动画的播放模式,如动画正常播放,反向播放,也可以在奇偶次数进行不同的正反向播放;
- onFinish:回调函数,在动画播放完成时出发。当iterations设置为-1时,动画效果无限循环不会停止,所以不会触发此回调;
(三)、使用案例
1、点击按钮变大(width/height)
如果没有加属性动画,那么在点击按钮时就会很生硬地变动,如下所示:
Button('change size')
.onClick(() => {
if (this.flag) {
this.widthSize = 150
this.heightSize = 60
} else {
this.widthSize = 250
this.heightSize = 100
}
this.flag = !this.flag
})
.margin(30)
.width(this.widthSize)
.height(this.heightSize)
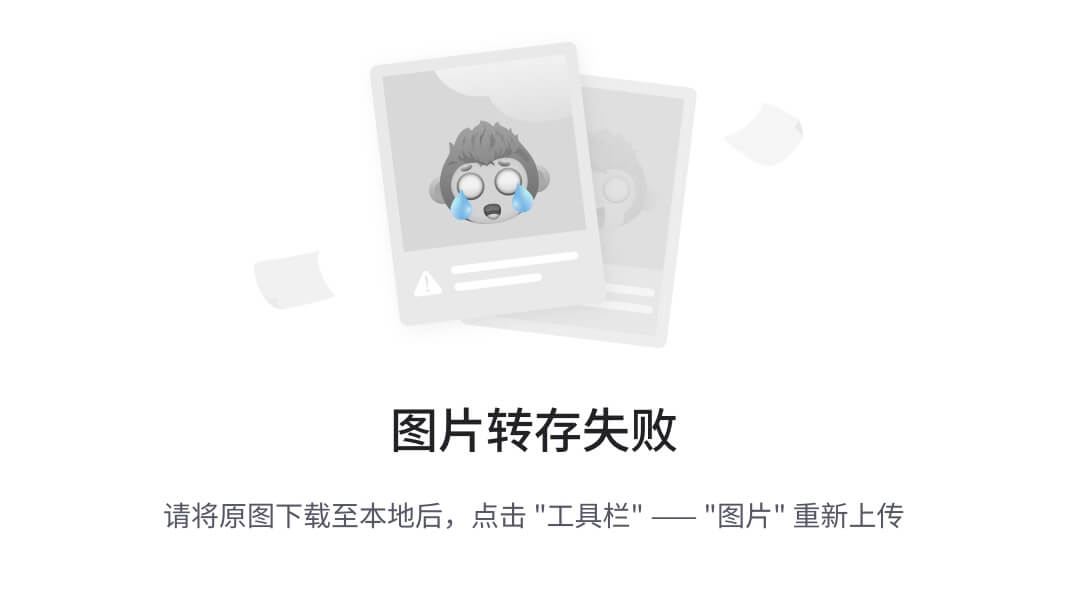
使用属性动画后,点击按钮后按钮变大产生了动画效果,更加顺滑:
Button('change size')
.onClick(() => {
if (this.flag) {
this.widthSize = 150
this.heightSize = 60
} else {
this.widthSize = 250
this.heightSize = 100
}
this.flag = !this.flag
})
.margin(30)
.width(this.widthSize)
.height(this.heightSize)
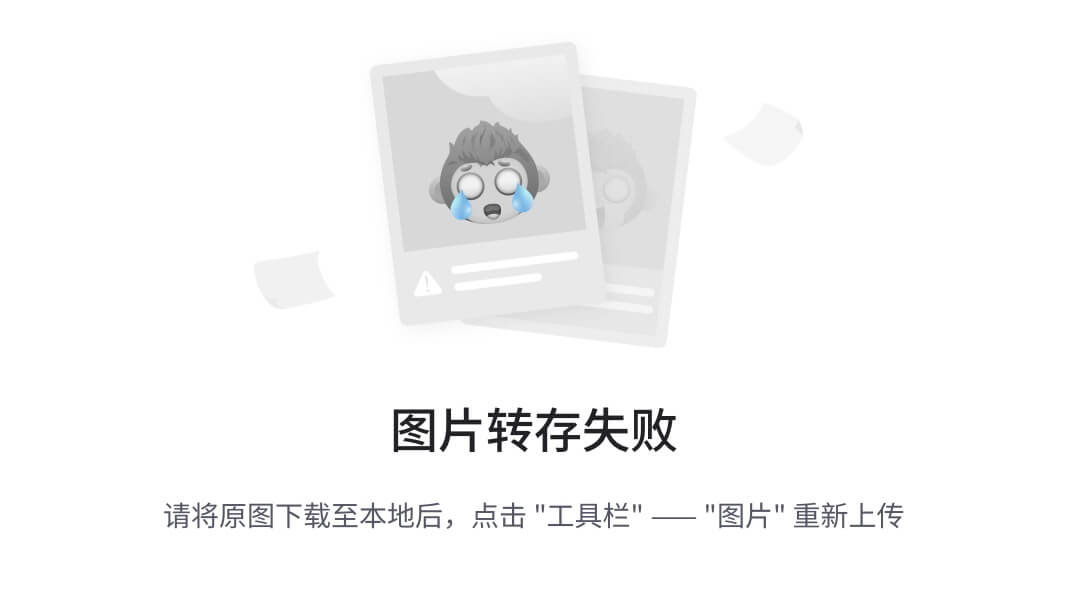
我们可以通过调整animation的属性来调整动画效果。
2、点击按钮调整背景色(backgroundColor)
backgroundColor
属性也可以通过属性动画进行调节,如下所示:
Button('change size')
.onClick(() => {
if (this.flag) {
this.widthSize = 150
this.heightSize = 60
this.bgColor = Color.Blue
} else {
this.widthSize = 250
this.heightSize = 100
this.bgColor = Color.Black
}
this.flag = !this.flag
})
.margin(30)
.backgroundColor(this.bgColor)
.width(this.widthSize)
.height(this.heightSize)
.animation({
duration: 500,
curve: Curve.EaseOut,
iterations: 1,
playMode: PlayMode.Normal
})
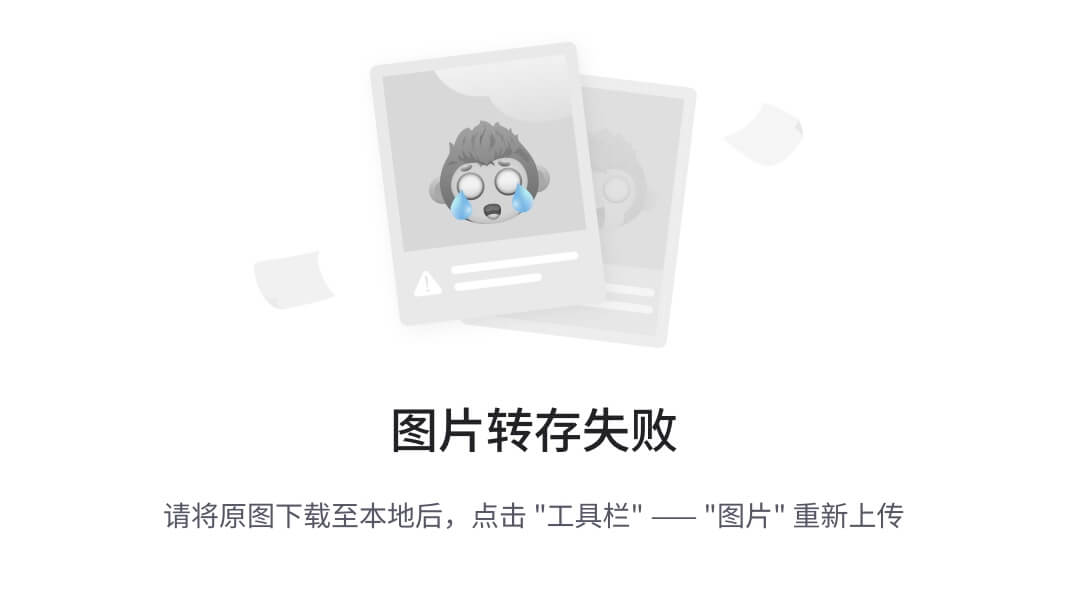
3、点击按钮旋转(rotate)
rotate
属性也可以根据属性动画进行变动,如下所示:
Button('change rotate angle')
.onClick(() => {
if(this.flag){
this.rotateAngle = 90
}
else{
this.rotateAngle = 180
}
this.flag = !this.flag
})
.margin(50)
.rotate({ angle: this.rotateAngle })
.animation({
duration: 1200,
curve: Curve.Friction,
delay: 500,
iterations: 1, // 设置-1表示动画无限循环
playMode: PlayMode.Alternate
})
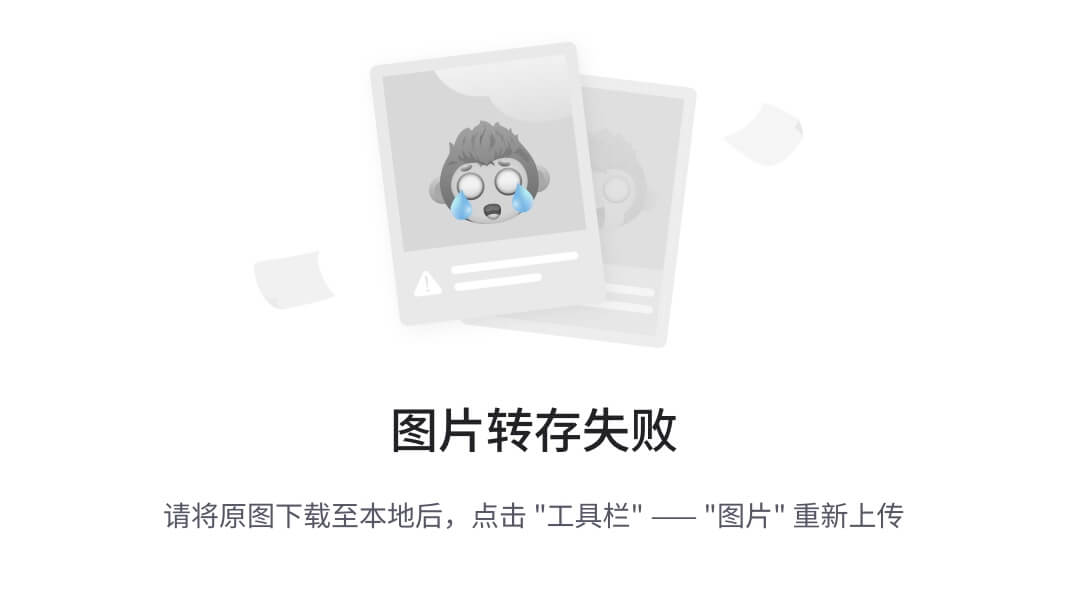
4、动画结束时替换文字(onFinish)
通过onFinish
属性,在动画播放完成后替换文字:
Button(this.btnText)
.onClick(() => {
if (this.flag) {
this.widthSize = 150
this.heightSize = 60
this.bgColor = Color.Blue
this.opa = 0.8
} else {
this.widthSize = 250
this.heightSize = 100
this.bgColor = Color.Black
this.opa = 0.5
}
this.flag = !this.flag
})
.opacity(this.opa)
.margin(30)
.backgroundColor(this.bgColor)
.width(this.widthSize)
.height(this.heightSize)
.animation({
duration: 500,
curve: Curve.EaseOut,
iterations: 1,
playMode: PlayMode.Normal,
onFinish: () => {
if(this.flag){
this.btnText = "button clicked"
}else{
this.btnText = "button"
}
}
})
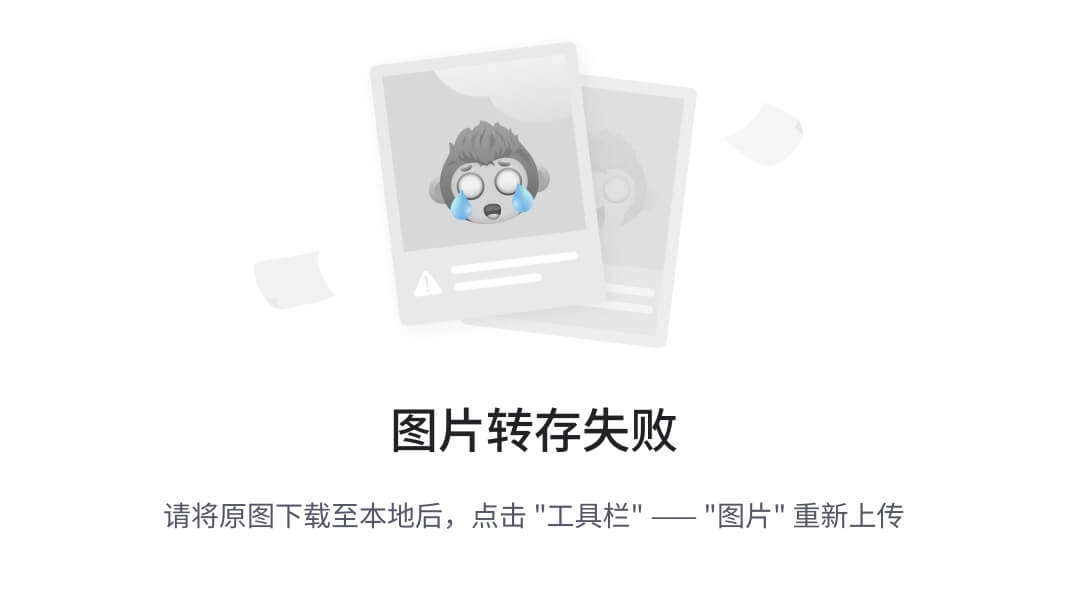
我们可以通过函数来替换onFinish
属性对应的函数,如下所示:
private onBtnAnimationFinish(){
if(this.flag){
this.btnText = "button clicked"
}else{
this.btnText = "button"
}
}
build() {
Column() {
Button(this.btnText)
.onClick(() => {
if (this.flag) {
this.widthSize = 150
this.heightSize = 60
this.bgColor = Color.Blue
this.opa = 0.8
} else {
this.widthSize = 250
this.heightSize = 100
this.bgColor = Color.Black
this.opa = 0.5
}
this.flag = !this.flag
})
.opacity(this.opa)
.margin(30)
.backgroundColor(this.bgColor)
.width(this.widthSize)
.height(this.heightSize)
.animation({
duration: 500,
curve: Curve.EaseOut,
iterations: 1,
playMode: PlayMode.Normal,
onFinish: this.onBtnAnimationFinish
})
}.width('100%').margin({ top: 20 })
}
二、显式动画
(一)、定义
官网的定义如下:
提供全局animateTo显式动画接口来指定由于闭包代码导致的状态变化插入过渡动效。
个人理解:animationTo
其实与属性动画的效果是一样的,只是使用的方式不太一样,属性动画是在组件上直接使用的,而animationTo
是在以全局函数的形式使用的,第二个参数为函数闭包,在该函数闭包中如果存在状态变量的变动,那么就是通过第一个参数对应的动画数据展示动画效果。
(二)、使用方式
animateTo(
value: AnimateParam,
event: () => void
): void
animateTo
以函数的形式出现,第二个参数为函数闭包,在该函数闭包中如果存在状态变量的变动,那么就是通过第一个参数对应的动画数据展示动画效果。
(三)、使用案例
animateTo
函数其实与属性动画效果是一致的,只不过使用方式不一样,可以针对指定的闭包函数效果来执行不同的动画效果。对于属性动画来说,只有一种动画变动效果,只要对应的属性存在变动,则会使用该动画进行过渡。而animateTo
函数则可以针对一个组件进行多种动画效果展示,针对不同的闭包函数可以执行不同的动画效果。
如下所示,我们针对flag
的属性值,执行两种不同的闭包函数,调用两种不同的动画效果:
Button(this.btnText)
.onClick(() => {
if (this.flag) {
animateTo({
duration: 500,
curve: Curve.EaseOut,
iterations: 1,
playMode: PlayMode.Normal,
onFinish: this.onBtnAnimationFinish
}, () =>{
this.widthSize = 150
this.heightSize = 60
this.bgColor = Color.Blue
this.opa = 0.8
})
} else {
animateTo({
duration: 500,
curve: Curve.EaseOut,
iterations: 1,
playMode: PlayMode.Normal,
onFinish: this.onBtnAnimationFinish
}, () =>{
this.widthSize = 250
this.heightSize = 100
this.bgColor = Color.Black
this.opa = 0.5
})
}
this.flag = !this.flag
})
.opacity(this.opa)
.margin(30)
.backgroundColor(this.bgColor)
.width(this.widthSize)
.height(this.heightSize)