栈和队列是限定插入和删除操作的只能在表的 “端点” 进行的特殊的线性表。
一、栈
操作原则:先进后出(后进先出)
栈的应用:
引入:
可以利用一个栈结构保存每个出现的左括号,当遇到右括号时,从栈中弹出左括号,检验匹配情况。
检验过程中,遇到以下情况之一可得出括号不匹配的结论:
(1)当遇到某一个右括号时,栈已空,说明到目前为止,右括号多于左括号。
(2)从栈中弹出的左括号与当前检验的右括号类型不同,说明出现了括号交叉情况。
(3)算术表达式输入完毕,但栈中还有没有匹配的左括号,说明左括号多于右括号。
1.栈的抽象数据类型
具体操作 :
2.栈的顺序存储结构及实现
操作:
1.初始化栈
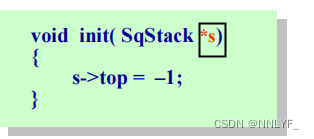
typedef struct {
int top;
} SqStack;
void init(SqStack s) {
s.top = -1;
}
int main() {
SqStack stack;
stack.top = 0;
init(stack);
printf("%d\n", stack.top); // 输出结果为0,未被修改
return 0;
}
正确方法:
void init(SqStack *s) {
s->top = -1;
}
int main() {
SqStack stack;
init(&stack);
printf("%d\n", stack.top); // 输出结果为-1,已经被初始化
return 0;
}
2.测试栈是否为空、是否已满(不需要修改原始数据)
3.入栈和出栈
#include <stdio.h>
#define M (1000+5)
typedef char ElemType;
typedef struct {
ElemType data[M];
int top;
} SqStack;
void push(SqStack* S, ElemType x) {//入栈操作
if (S->top < M - 1) {
S->top++;
S->data[S->top] = x;
}
else {
printf("overflow!");
}
}
ElemType pop(SqStack* S) {//出栈操作
if (S->top == -1) {
printf("Underflow");
return -1;
}
else {
ElemType x = S->data[S->top];
S->top--;
return x;
}
}
int main() {
SqStack stack;
stack.top = -1;//初始化栈
push(&stack, 'a');
push(&stack, 'b');
push(&stack, 'c');
for (int i = stack.top; i >= 0; i--) {
printf("%c ", stack.data[i]);
}
printf("\n");
while (stack.top != -1) {
ElemType x = pop(&stack);
printf("Pop: %c\n", x);
}
return 0;
}
如果Top初始值为1
要注意出栈和入栈时,是指针先移动还是元素先动!!
bool Push (Stack S,ElementType x)
{
if(S->Top==S->MaxSize){
printf("Stack Full\n");
return false;
}
else{
S->Data[S->Top]=x;
S->Top++;
return true;
}
}
ElementType Pop(Stack S)
{
if(S->Top==0){
printf("Stack Empty\n");
return ERROR;
}
else{
S->Top--;
ElementType x=S->Data[S->Top];
return x;
}
}
例题:利用顺序栈实现十进制转r进制。输入2个自然数n和r,输出转换后的r进制数。测试数据有多组,处理到输入结束。
#include <stdio.h>
#define M (1000+5)
typedef char ElemType;
typedef struct {
ElemType data[M];
int top;
} SqStack;
void push(SqStack* S, ElemType x) {//入栈操作
if (S->top < M - 1) {
S->top++;
S->data[S->top] = x;
}
else {
printf("overflow!");
}
}
ElemType pop(SqStack* S) {//出栈操作
if (S->top == -1) {
printf("Underflow");
return -1;
}
else {
ElemType x = S->data[S->top];
S->top--;
return x;
}
}
void decimalToR(int n, int r) {
SqStack stack;
stack.top = -1;//栈的初始化
while (n > 0) {
int remainder = n % r;
if (remainder < 10) {
push(&stack, '0' + remainder);
}
else {
push(&stack, 'A' + remainder - 10);
}
n =n / r;
}
while (stack.top != -1) {
printf("%c", pop(&stack));
}
printf("\n");
}
int main() {
int n, r;
while (scanf("%d %d", &n, &r) != EOF) {
decimalToR(n, r);
}
return 0;
}
类似的题: