本文翻译自SUN官方文档
“Ruby on Rails” Principles
“Ruby on Rails”的准测
Convention over configuration
约定更重于配置
Why punish the common cases?
Encourages standard practices
鼓励标准化
Everything simpler and smaller
每件事情更加简单而小型化
Don’t Repeat Yourself (DRY)
Framework written around minimizing repetition
这个框架减少重复劳动
Repetitive code harmful to adaptability
重复性代码有害适用性
Agile development environment
No recompile, deploy, restart cycles
没有编译,部署,重启环节
Simple tools to generate code quickly
简单的工具高效产生代码
Testing built into the framework
由测试驱动
Step By Step Process of Building “Hello World” Rails Application
一步一步搭建“Hello World”的Rails应用
Steps to Follow:
Demo: Building “Hello World” Rails Application Step by Step.
1. Create “Ruby on Rails” Project
创建“Ruby on Rails”工程
The directory structure along with boilerplate files of the application is created
目录结构被自动创建
Directory Structure of a Rails Application
When you ask NetBeans to create a Rails project - internally NetBeans uses the rails' helper script -, it creates the entire directory structure for your application.
netbeans会自动创建Rails的目录结构
The boiler plate files are also created
The names of the directories and files are the same for all Rails projects
Rails knows where to find things it needs within this structure, so you don't have to tell it explicitly.
Rails知道如何从这种结构里找到它需要的东西,你不必明确指明。
Directory Structure of a Rails Application
app: Holds all the code that's specific to this particular application.
app:控制这个项目专属的代码
config:控制Rails环境的配置文件
config/environments
config/initializers
boot.rb
database.yml
environment.rb
routes.rb
Learning Point: Environments
What is an Environment?
Rails provides the concept of environments - development, test, production
Rails提供环境的概念 - 开发,测试,产品
As a default, different database is going to be used for different environment.
默认情况下,不同的数据将被用于不用的环境、
Therefore each environment has its own database connection settings.
因此,不同的环境有自己独立数据联接设置
It is easy to add custom environments
添加环境是很方便的
For example, staging server environment
例如,添加server环境
Rails always runs in only one environment
Rails只能在一个环境里运行
Dictated by ENV['RAILS_ENV'] (same as RAILS_ENV)
config/database.yml
2. Create Database using “Rake”
Creating Database
Creating and dropping of databases are done using “Rake” 用Rake创建和删除数据库
After create rake task is performed, <project_name>_development database, for example, helloworld_development is created
Learning Point: What is Rake?
What is “Rake”?
db:migrate
db:sessions:create
doc:app
doc:rails
log:clear
rails:freeze:gems
rails:freeze:edge
rails:update
:test
:stats
3. Create a Model through “Generator”
What is a Model?
In the context of MVC pattern, a Model represents domain objects such as message, school, product, etc. A model has attributes and methods.
The attributes represents the characteristics of the domain object, for example, a message model might have length, creator as attributes.
The methods in a model contains some business logic.
Most models have corresponding database tables. For example, a message model will have messages table.
Most model classes are ActiveRecord type
Creating a Model using Generator
Files That Are Created
app/models/message.rb (Model file)
Model Class Example
Message mode in messages.rb file
Learning Point: What is Generator?
What is “Generator”?
You can often avoid writing boilerplate code by using the built-in generator scripts of Rails to create it for you.
模板的代码可以由Rails脚本完成
This leaves you with more time to concentrate on the code that really matters--your business logic.
这可以使你将更多的精力放在那些真正有意义的业务逻辑代码上
Leaning Point:What is Rails Console?
The Rails console gives you access to your Rails Environment, for example, you can interact with the domain models of your application as if the application is actually running.
Things you can do include performing find operations or creating a new active record object and then saving it to the database.
A great tool for impromptu testing
NetBeans runs a script to start Rails Console
Leaning Point: What is Rails Script?
Script
NetBeans runs Rails Script internally
You can run the Script at the commandline
Useful scripts
console
generate
plugin
server
4. Create Database Tables using Migration
You are going to create a database table (in a previously created database) through migration
You also use migration for any change you are going to make in the schema - adding a new column, for example
When you create a Model, the first version of the migration file is automatically created
db/migrate/migrate/001_create_messages.rb, which defines initial structure of the table
Performing Migration
Leaning Point: What is Migration?
Migrations can manage the evolution of a schema.
It's a solution to the common problem of adding a field to make a new feature work in your local database, but being unsure of how to push that change to other developers and to the production server.
With migrations, you can describe the transformations in self-contained classes that can be checked into version control systems and executed against another database that might be one, two, or five versions behind.
5. Create a Controller
Example: HelloController
Controller contains actions, which are defined with def
6. Write a View
What is a View?
7. Set URL Routing
URL Routing
The Rails routing facility is pure Ruby code that even allows you to use regular expressions.
Because Rails does not use the web server's URL mapping, your custom URL mapping will work the same on every web server.
configuration/routes.rb file contains the routing setting
routes.rb
What is and Why Ruby on Rails (RoR)?
What Is “Ruby on Rails”?
A full-stack MVC web development framework
一个MVC的web开发框架
Written in Ruby
Ruby编写
First released in 2004 by David Heinemeier Hansson
由David Heinemeier Hansson在2004年发布第一个版本
Gaining popularity
更加流行
“Ruby on Rails” MVC
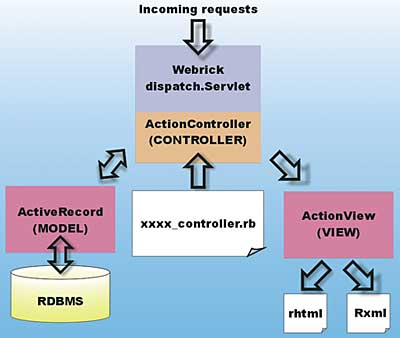
“Ruby on Rails” Principles
“Ruby on Rails”的准测
Convention over configuration
约定更重于配置
Why punish the common cases?
Encourages standard practices
鼓励标准化
Everything simpler and smaller
每件事情更加简单而小型化
Don’t Repeat Yourself (DRY)
Framework written around minimizing repetition
这个框架减少重复劳动
Repetitive code harmful to adaptability
重复性代码有害适用性
Agile development environment
No recompile, deploy, restart cycles
没有编译,部署,重启环节
Simple tools to generate code quickly
简单的工具高效产生代码
Testing built into the framework
由测试驱动
Step By Step Process of Building “Hello World” Rails Application
一步一步搭建“Hello World”的Rails应用
Steps to Follow:
- Create “Ruby on Rails” project - Rails generates directory structure Rails自动产生目录结构
- Create Database (using Rake) 自动创建数据库
- Create Models (through Rails Generator) 自动创建模型
- Create Database Tables (using Migration) 自动创建数据表
- Create Controllers (through Rails Generator) 自动创建控制器
- Create Views 创建视图
- Set URL Routing - Map URL to controller and action 映射URL到控制器和action
Demo: Building “Hello World” Rails Application Step by Step.
1. Create “Ruby on Rails” Project
创建“Ruby on Rails”工程
The directory structure along with boilerplate files of the application is created
目录结构被自动创建
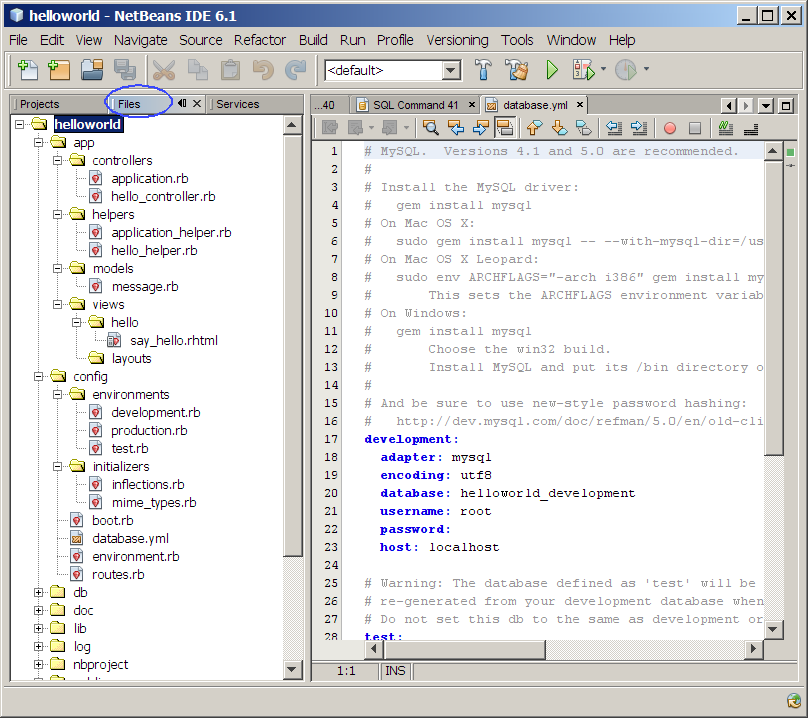
Directory Structure of a Rails Application
When you ask NetBeans to create a Rails project - internally NetBeans uses the rails' helper script -, it creates the entire directory structure for your application.
netbeans会自动创建Rails的目录结构
The boiler plate files are also created
The names of the directories and files are the same for all Rails projects
Rails knows where to find things it needs within this structure, so you don't have to tell it explicitly.
Rails知道如何从这种结构里找到它需要的东西,你不必明确指明。
Directory Structure of a Rails Application
app: Holds all the code that's specific to this particular application.
app:控制这个项目专属的代码
- app/controllers: Holds controllers that should be named like hello_controller.rb for automated URL mapping. All controllers should descend from ApplicationController which itself descends from ActionController::Base. app/controllers:控制器应该被命名如hello_controller.rb,这样就能自动映射URL。
- app/models: Holds models that should be named like message.rb. Most models will descend from ActiveRecord::Base. app/models:模型应该被命名为message.rb。
- app/views: Holds the template files for the view that should be named like hello/say_hello.rhtml for the HelloController#say_hello action. app/views:视图的模板文件应该被命名为hello/say_hello.rhtml
- app/views/layouts: Holds the template files for layouts to be used with views. This models the common header/footer method of wrapping views. In your views, define a layout using the <tt>layout :default</tt> and create a file named default.rhtml. Inside default.rhtml, call <% yield %> to render the view using this layout.
- app/helpers: Holds view helpers that should be named like hello_helper.rb. These are generated for you automatically when using script/generate (Generator) for controllers. Helpers can be used to wrap functionality for your views into methods.
config:控制Rails环境的配置文件
config/environments
config/initializers
boot.rb
database.yml
environment.rb
routes.rb
Learning Point: Environments
What is an Environment?
Rails provides the concept of environments - development, test, production
Rails提供环境的概念 - 开发,测试,产品
As a default, different database is going to be used for different environment.
默认情况下,不同的数据将被用于不用的环境、
Therefore each environment has its own database connection settings.
因此,不同的环境有自己独立数据联接设置
It is easy to add custom environments
添加环境是很方便的
For example, staging server environment
例如,添加server环境
Rails always runs in only one environment
Rails只能在一个环境里运行
Dictated by ENV['RAILS_ENV'] (same as RAILS_ENV)
config/database.yml
- development:
- adapter: mysql
- encoding: utf8
- database: helloname_development
- username: root
- password:
- host: localhost
- test:
- adapter: mysql
- encoding: utf8
- database: helloname_test
- username: root
- password:
- host: localhost
2. Create Database using “Rake”
Creating Database
Creating and dropping of databases are done using “Rake” 用Rake创建和删除数据库
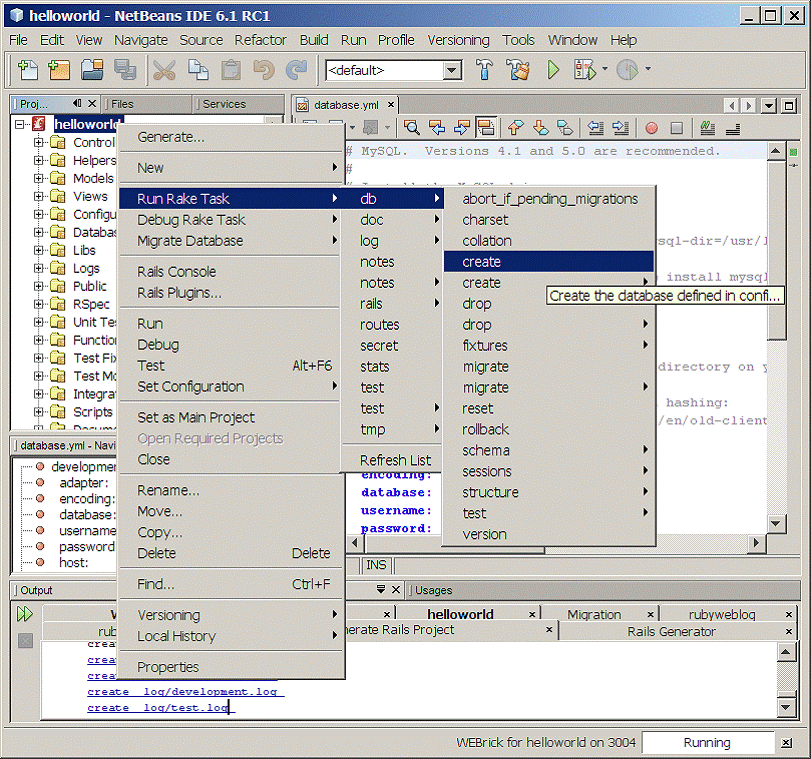
After create rake task is performed, <project_name>_development database, for example, helloworld_development is created
Learning Point: What is Rake?
What is “Rake”?
- Rake is a build language for Ruby.
- Rails uses Rake to automate several tasks such as creating and dropping databases, running tests, and updating Rails support files.
- Rake lets you define a dependency tree of tasks to be executed
- Rake tasks are loaded from the file Rakefile
- Rails rake tasks are under <project-name>/lib/tasks
- You can put your custom tasks under lib/tasks
db:migrate
db:sessions:create
doc:app
doc:rails
log:clear
rails:freeze:gems
rails:freeze:edge
rails:update
:test
:stats
3. Create a Model through “Generator”
What is a Model?
In the context of MVC pattern, a Model represents domain objects such as message, school, product, etc. A model has attributes and methods.
The attributes represents the characteristics of the domain object, for example, a message model might have length, creator as attributes.
The methods in a model contains some business logic.
Most models have corresponding database tables. For example, a message model will have messages table.
Most model classes are ActiveRecord type
Creating a Model using Generator
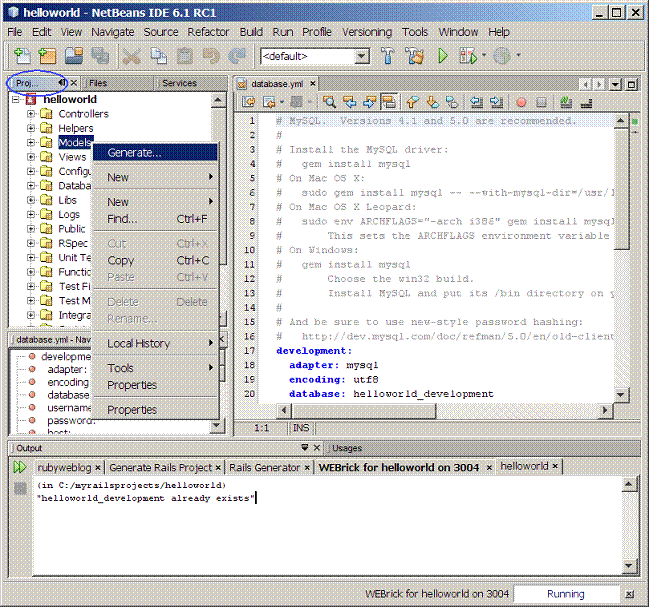
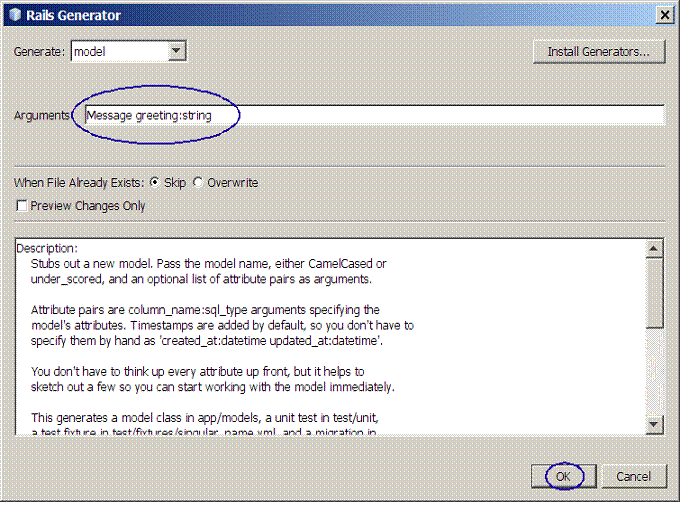
Files That Are Created
app/models/message.rb (Model file)
- Models/messages.rb in logical view
- A file that holds the methods for the Message model.
- Unit Tests/message_test.rb in logical view
- A unit test for checking the Message model.
- Test Fixtures/messages.yml in logical view
- A test fixture for populating the model.
- Database Migrations/migrate/001_create_messages.rb in logical view
- A migration file for defining the initial structure of the database.
Model Class Example
Message mode in messages.rb file
- class Message < ActiveRecord::Base
- end
Learning Point: What is Generator?
What is “Generator”?
You can often avoid writing boilerplate code by using the built-in generator scripts of Rails to create it for you.
模板的代码可以由Rails脚本完成
This leaves you with more time to concentrate on the code that really matters--your business logic.
这可以使你将更多的精力放在那些真正有意义的业务逻辑代码上
Leaning Point:What is Rails Console?
The Rails console gives you access to your Rails Environment, for example, you can interact with the domain models of your application as if the application is actually running.
Things you can do include performing find operations or creating a new active record object and then saving it to the database.
A great tool for impromptu testing
NetBeans runs a script to start Rails Console
Leaning Point: What is Rails Script?
Script
NetBeans runs Rails Script internally
You can run the Script at the commandline
Useful scripts
console
generate
plugin
server
4. Create Database Tables using Migration
You are going to create a database table (in a previously created database) through migration
You also use migration for any change you are going to make in the schema - adding a new column, for example
When you create a Model, the first version of the migration file is automatically created
db/migrate/migrate/001_create_messages.rb, which defines initial structure of the table
- class CreateMessages < ActiveRecord::Migration
- def self.up
- create_table :messages do |t|
- t.string :greeting
- t.timestamps
- end
- end
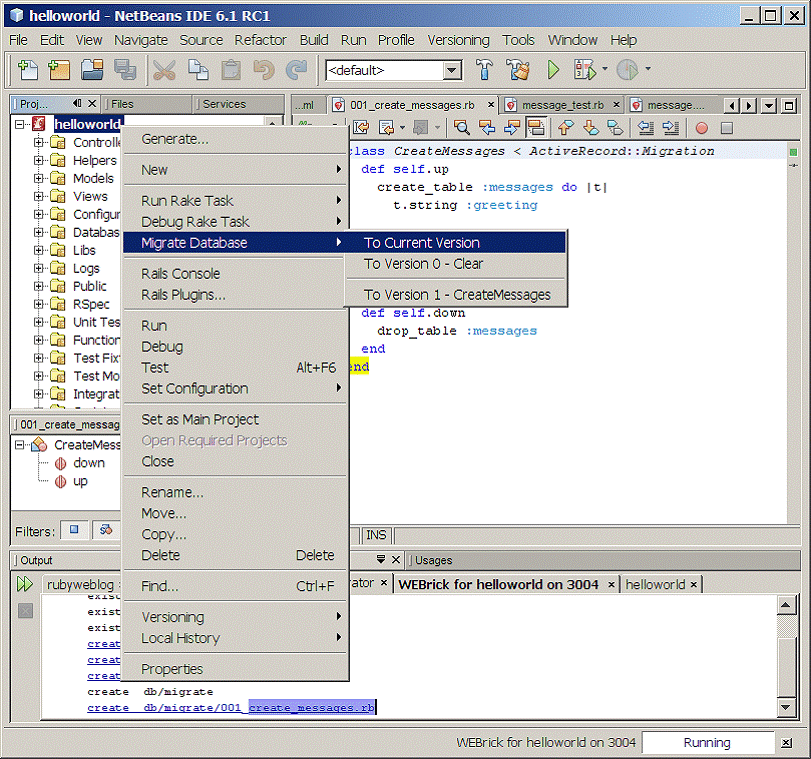
Leaning Point: What is Migration?
Migrations can manage the evolution of a schema.
It's a solution to the common problem of adding a field to make a new feature work in your local database, but being unsure of how to push that change to other developers and to the production server.
With migrations, you can describe the transformations in self-contained classes that can be checked into version control systems and executed against another database that might be one, two, or five versions behind.
5. Create a Controller
- Action Controllers are the core of a web request in Rails.
- They are made up of one or more actions that are executed on request and then either render a template or redirect to another action.
- An action is defined as a public method on the controller, which will automatically be made accessible to the web-server through Rails Routes.
- You are going to create a controller using Generator
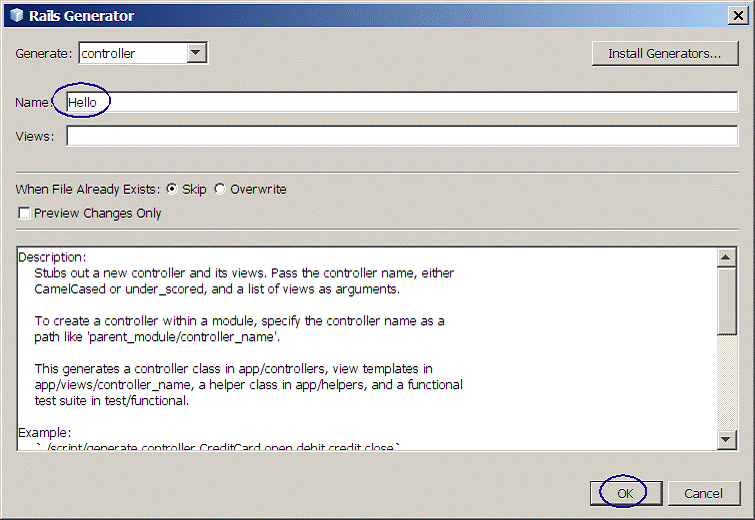
Example: HelloController
Controller contains actions, which are defined with def
- class HelloController < ApplicationController
- def say_hello
- @hello = Message.new(:greeting => "Hello World!")
- end
- end
6. Write a View
What is a View?
- View is represented by a set of templates that get displayed.
- Templates share data with controllers through mutually accessible variables.
- A template can be either in the form of *.rhtml or *.erb file. - The *.erb file is searched first by Rails. If there is no *.erb file, then *.rhtml file is used.
- RHTML file is created under the directory of /app/views/<controller>
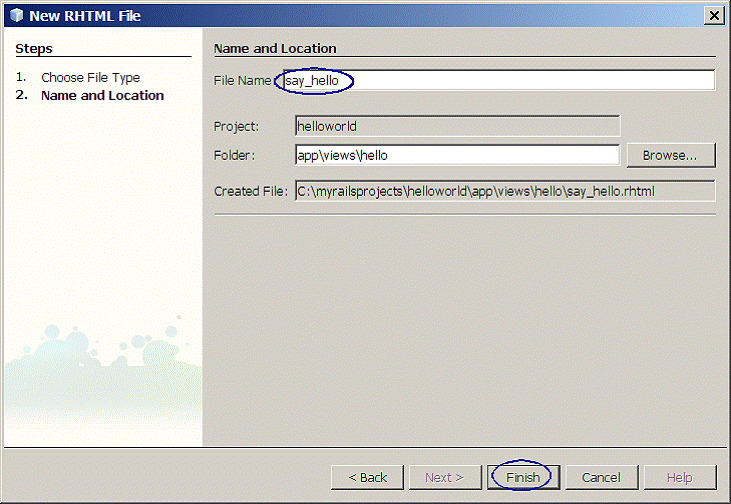
- say_hello.rhtml
- My greeting message is <%= @hello.greeting %>
- <br/>
- The current time is <%= Time.now %>
7. Set URL Routing
URL Routing
The Rails routing facility is pure Ruby code that even allows you to use regular expressions.
Because Rails does not use the web server's URL mapping, your custom URL mapping will work the same on every web server.
configuration/routes.rb file contains the routing setting
routes.rb
- ActionController::Routing::Routes.draw do |map|
- map.root :controller => "hello"
- # Install the default routes as the lowest priority.
- map.connect ':controller/:action/:id'
- map.connect ':controller/:action/:id.:format'
- end