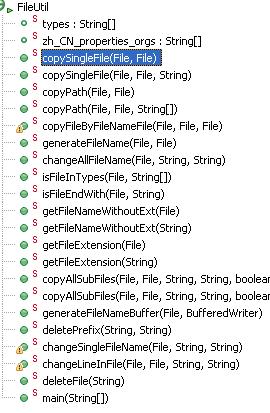
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.nio.channels.FileChannel;
import java.util.ArrayList;
import java.util.Hashtable;
import java.util.List;
/**
* Author: zhusheng3@126.com
* Date 2007-11-28 08:36:45
* NMS, UTStarcom, Shenzhen
*/
public class FileUtil
{
public static String[] types = new String[] { ".txt", ".sql", ".xml",
".properties.org", ".properties", ".conf",".csv" ,".parsers"};
public static String[] zh_CN_properties_orgs = new String[] { "zh_CN.properties.org"};
public static void copySingleFile(File in, File out) throws IOException
{
if (true)
{
if (!out.exists())
{
out.getParentFile().mkdirs();
}
FileChannel inChannel = new FileInputStream(in).getChannel();
FileChannel outChannel = new FileOutputStream(out).getChannel();
try
{
// System.out.println("File Coppied from:" + in);
inChannel.transferTo(0, inChannel.size(), outChannel);
}
catch (IOException e)
{
e.printStackTrace();
throw e;
}
finally
{
if (inChannel != null) inChannel.close();
if (outChannel != null) outChannel.close();
}
}
else
{
System.out.println("File Can't Write:" + out);
}
}
public static void copySingleFile(File in, File out, String fileType)
throws IOException
{
if (FileUtil.isFileEndWith(in, fileType))
{
copySingleFile(in, out);
}
}
public static void copyPath(File sourceRoot, File targetRoot)
{
copyAllSubFiles(sourceRoot, targetRoot, null, null, true);
}
public static void copyPath(File sourceRoot, File targetRoot, String[] types)
{
copyAllSubFiles(sourceRoot, targetRoot, null, null, true, types);
}
public static void copyFileByFileNameFile(File fileNameListFile,
File fromPath,File toPath) throws IOException
{
BufferedReader br = null;
try
{
br = new BufferedReader(new FileReader(fileNameListFile));
String line = br.readLine();
String sourcePrefix = line.substring(0, line.indexOf("//"));
while (line != null && (!line.trim().equalsIgnoreCase("")))
{
// source file
String srcName =line;
String srcPrefix =fromPath.getAbsolutePath();
File srcFile = new File(line);
// target file
String tgtPrefix =toPath.getAbsolutePath()+"//";
String tgtName =tgtPrefix+srcName.substring(srcName.indexOf(srcPrefix)+srcPrefix.length());
File tgtFile = new File(tgtName);
// copy each file
copySingleFile(srcFile, tgtFile);
line = br.readLine();
}
}
catch (Exception e)
{
e.printStackTrace();
}
finally
{
if (br != null)
{
try
{
br.close();
}
catch (IOException e)
{
e.printStackTrace();
}
}
}
}
public static void generateFileName(File sourcePath, File outPutFile)
{
BufferedWriter bw = null;
try
{
bw = new BufferedWriter(new FileWriter(outPutFile));
generateFileNameBuffer(sourcePath, bw);
}
catch (IOException e)
{
e.printStackTrace();
}
finally
{
if (bw != null)
{
try
{
bw.close();
}
catch (IOException e)
{
e.printStackTrace();
}
}
}
}
public static void changeAllFileName(File inputFile, String fromString,
String toString)
{
// if input parameter is a Single File
if (inputFile.isFile())
{
changeSingleFileName(inputFile, fromString, toString);
}
// if input parameter is a Directory
else if (inputFile.isDirectory())
{
File[] subFiles = inputFile.listFiles();
for (int i = 0; i < subFiles.length; i++)
{
File tempFile = subFiles[i];
if (tempFile.isFile())
{
changeSingleFileName(tempFile, fromString, toString);
}
else if (tempFile.isDirectory())
{
// 锟捷癸拷recursion the directory
changeAllFileName(tempFile, fromString, toString);
}
else
{
System.out.println("this file Name can not parse:"
+ tempFile.getAbsolutePath());
}
}
}
else
{
System.out.println("this file Name can not parse:"
+ inputFile.getAbsolutePath());
}
}
public static boolean isFileInTypes(File file, String[] types)
{
for (int i = 0; i < types.length; i++)
{
if (isFileEndWith(file, types[i]))
{
return true;
}
}
return false;
}
public static boolean isFileEndWith(File file, String endWithString)
{
if (file.isDirectory()) return false;
// endWithString is null or empty string return true
if (endWithString == null || endWithString.equalsIgnoreCase(""))
{
return true;
}
else
{
String fileName = file.getName();
if (fileName.length() > endWithString.length())
{
String fileend = fileName.substring(fileName.length()
- endWithString.length(), fileName.length());
if (endWithString.equalsIgnoreCase(fileend))
{
return true;
}
else
{
return false;
}
}
return false;
}
}
public static String getFileNameWithoutExt(File file)
{
if (file.isDirectory()) return null;
String fileName = file.getAbsolutePath();
int pointPostion = fileName.lastIndexOf(".");
return fileName.substring(0,pointPostion);
}
public static String getFileNameWithoutExt(String filename)
{
File file = new File(filename);
if (file.isDirectory()) return null;
String fileName = file.getAbsolutePath();
int pointPostion = fileName.lastIndexOf(".");
return fileName.substring(0,pointPostion);
}
public static String getFileExtension(File file)
{
if (file.isDirectory()) return null;
String fileName = file.getName();
int pointPostion = fileName.lastIndexOf(".");
return fileName.substring(pointPostion, fileName.length());
}
public static String getFileExtension(String fileName)
{
try
{
File file = new File(fileName);
if (file.isDirectory()) return null;
int pointPostion = fileName.lastIndexOf(".");
return fileName.substring(pointPostion, fileName.length());
}
catch (Exception e)
{
e.printStackTrace();
}
return null;
}
public static void copyAllSubFiles(File sourceRoot, File targetRoot,
String sourcePrefix, String targetPrefix, boolean isFirstTime)
{
if (isFirstTime)
{
sourcePrefix = sourceRoot.getAbsolutePath();
targetPrefix = targetRoot.getAbsolutePath();
}
try
{
String targetFilePath = "";
if (sourceRoot.isFile())
{
String targetPath = targetRoot.getAbsolutePath();
if (!targetPath.endsWith("//"))
{
targetPath = targetPath + "//";
}
targetFilePath = targetPath + sourceRoot.getName();
copySingleFile(sourceRoot, new File(targetFilePath));
}
else if (sourceRoot.isDirectory())
{
File[] files = sourceRoot.listFiles();
for (int i = 0; i < files.length; i++)
{
String srcName = "";
String tgtName = "";
File tgtFile = null;
if (files[i].isFile())
{
srcName = files[i].getAbsolutePath();
tgtName = targetPrefix
+ srcName.substring(srcName
.indexOf(sourcePrefix)
+ sourcePrefix.length());
tgtFile = new File(tgtName);
copySingleFile(files[i], tgtFile);
}
else if (files[i].isDirectory())
{
srcName = files[i].getAbsolutePath();
tgtName = targetPrefix
+ srcName.substring(srcName
.indexOf(sourcePrefix)
+ sourcePrefix.length());
tgtFile = new File(tgtName);
if (!tgtFile.exists())
{
tgtFile.mkdirs();
}
// System.out.println("srcName:"+srcName);
// System.out.println("tgtName:"+tgtName);
copyAllSubFiles(files[i], tgtFile, sourcePrefix,
targetPrefix, false);
}
}
}
}
catch (IOException e)
{
e.printStackTrace();
}
}
public static void copyAllSubFiles(File sourceRoot, File targetRoot,
String sourcePrefix, String targetPrefix, boolean isFirstTime,
String[] types)
{
if (isFirstTime)
{
sourcePrefix = sourceRoot.getAbsolutePath();
targetPrefix = targetRoot.getAbsolutePath();
}
try
{
String targetFilePath = "";
if (sourceRoot.isFile())
{
String targetPath = targetRoot.getAbsolutePath();
if (!targetPath.endsWith("//"))
{
targetPath = targetPath + "//";
}
targetFilePath = targetPath + sourceRoot.getName();
if (isFileInTypes(sourceRoot, types))
{
copySingleFile(sourceRoot, new File(targetFilePath));
}
}
else if (sourceRoot.isDirectory())
{
File[] files = sourceRoot.listFiles();
for (int i = 0; i < files.length; i++)
{
String srcName = "";
String tgtName = "";
File tgtFile = null;
if (files[i].isFile())
{
srcName = files[i].getAbsolutePath();
tgtName = targetPrefix
+ srcName.substring(srcName
.indexOf(sourcePrefix)
+ sourcePrefix.length());
tgtFile = new File(tgtName);
if (isFileInTypes(files[i], types))
{
copySingleFile(files[i], tgtFile);
}
}
else if (files[i].isDirectory())
{
srcName = files[i].getAbsolutePath();
tgtName = targetPrefix
+ srcName.substring(srcName
.indexOf(sourcePrefix)
+ sourcePrefix.length());
tgtFile = new File(tgtName);
// if (!tgtFile.exists())
// {
// tgtFile.mkdirs();
// }
// System.out.println("srcName:"+srcName);
// System.out.println("tgtName:"+tgtName);
copyAllSubFiles(files[i], tgtFile, sourcePrefix,
targetPrefix, false, types);
}
}
}
}
catch (IOException e)
{
e.printStackTrace();
}
}
public static void generateFileNameBuffer(File sourcePath, BufferedWriter bw)
{
try
{
if (sourcePath.isFile())
{
bw.write(sourcePath.getAbsolutePath());
bw.newLine();
System.out.println(sourcePath.getAbsolutePath());
}
else if (sourcePath.isDirectory())
{
File[] files = sourcePath.listFiles();
for (int i = 0; i < files.length; i++)
{
if (files[i].isFile())
{
bw.write(files[i].getAbsolutePath());
bw.newLine();
System.out.println(files[i].getAbsolutePath());
}
else if (files[i].isDirectory())
{
generateFileNameBuffer(files[i], bw);
}
}
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
public static String deletePrefix(String inputString, String prefix)
{
String result = inputString.substring(inputString.indexOf(prefix)
+ prefix.length() );
return result;
}
public static void changeSingleFileName(File inputFile, String fromString,
String toString)
{
String oldFileName = inputFile.getAbsolutePath();
String newFileName = "";
// System.out.println("oldFileName:" + oldFileName);
if (oldFileName.indexOf(fromString) >= 0)
{
newFileName = oldFileName.replaceAll(fromString, toString);
File newFile = new File(newFileName);
inputFile.renameTo(new File(newFileName));
// System.out.println("newFileName:" + newFileName);
}
else
{
System.out.println("no match String:" + fromString + "->"
+ toString + " |in| " + inputFile.getName());
}
}
public static void changeLineInFile(File inputFile, File outputFile,
String fromPrefix, String toPrefix)
{
if (outputFile.exists())
{
// outputFile.delete();
}
BufferedReader br = null;
BufferedWriter bw = null;
int beginPos = 0;
int endPos = 0;
try
{
br = new BufferedReader(new FileReader(inputFile));
bw = new BufferedWriter(new FileWriter(outputFile));
String line = br.readLine();
boolean isend = false;
int begin = 0;
while (line != null)
{
// System.out.println("from:" + fromPrefix);
System.out.println(" old:" + line);
String newline = toPrefix + deletePrefix(line, fromPrefix);
//System.out.println(" to:" + toPrefix);
System.out.println("new:" + newline);
bw.write(newline);
bw.newLine();
line = br.readLine();
}
}
catch (Exception e)
{
System.out.println("--" + beginPos + ":" + endPos);
e.printStackTrace();
}
finally
{
if (br != null)
{
try
{
br.close();
}
catch (IOException e)
{
e.printStackTrace();
}
}
if (bw != null)
{
try
{
bw.close();
}
catch (IOException e)
{
e.printStackTrace();
}
}
}
}
public static boolean deleteFile(String deletePath) {
try {
File file = new File(deletePath);
if (!file.isDirectory()) {
file.delete();
} else if (file.isDirectory()) {
String[] filelist = file.list();
for (int i = 0; i < filelist.length; i++)
{
File delfile = new File(deletePath + "//" + filelist[i]);
if (!delfile.isDirectory())
delfile.delete();
else if (delfile.isDirectory())
deleteFile(deletePath + "//" + filelist[i]);
}
file.delete();
}
} catch (Exception e) {
e.printStackTrace();
}
return true;
}
public static void main(String[] args)
{
}
}