HSSFWorkbook wb = new HSSFWorkbook();
Sheet sheet = wb.createSheet("好家伙表");
sheet.setColumnWidth(0,3766);
sheet.setColumnWidth(1,3766);
sheet.setColumnWidth(2,3766);
sheet.setColumnWidth(3,3766);
sheet.setColumnWidth(4,3766);
sheet.setColumnWidth(5,3766);
sheet.setColumnWidth(6,3766);
sheet.setColumnWidth(7,3766);
sheet.setColumnWidth(8,3766);
sheet.setColumnWidth(9,3766);
sheet.setColumnWidth(10,3766);
sheet.setColumnWidth(11,3766);
sheet.setColumnWidth(12,3766);
Row titleRowOne = sheet.createRow(0);
titleRowOne.createCell(0).setCellValue("好家伙");
HSSFCellStyle style = wb.createCellStyle();
HSSFFont font = wb.createFont();
font.setFontName("宋体");
font.setFontHeightInPoints((short) 11);
font.setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);
style.setFont(font);
style.setFillForegroundColor(IndexedColors.LIGHT_YELLOW.index);
wb.getCustomPalette().setColorAtIndex(IndexedColors.LIGHT_YELLOW.index,(byte) 255,(byte) 255,(byte) 0);
style.setFillPattern(HSSFCellStyle.SOLID_FOREGROUND);
style.setBorderRight(BorderStyle.THIN);
style.setRightBorderColor(IndexedColors.BLACK.index);
titleRowOne.getCell(0).setCellStyle(style);
Row titleRowTwo = sheet.createRow(1);
CellRangeAddress regionOne = new CellRangeAddress(1,1,0,5);
sheet.addMergedRegion(regionOne);
HSSFCellStyle style1 = wb.createCellStyle();
HSSFFont font1 = wb.createFont();
font1.setFontName("宋体");
font1.setFontHeightInPoints((short) 11);
style1.setFont(font1);
style1.setAlignment(HSSFCellStyle.ALIGN_CENTER);
style1.setAlignment(HSSFCellStyle.VERTICAL_CENTER);
style1.setFillPattern(FillPatternType.SOLID_FOREGROUND);
style1.setFillForegroundColor(IndexedColors.CORNFLOWER_BLUE.index);
wb.getCustomPalette().setColorAtIndex(IndexedColors.CORNFLOWER_BLUE.index,(byte) 83,(byte) 141,(byte) 213);
style1.setBorderTop(BorderStyle.THIN);
style1.setTopBorderColor(IndexedColors.BLACK.index);
style1.setBorderBottom(BorderStyle.THIN);
style1.setBottomBorderColor(IndexedColors.BLACK.index);
style1.setBorderRight(BorderStyle.THIN);
style1.setRightBorderColor(IndexedColors.BLACK.index);
style1.getFont(wb).setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);
titleRowTwo.createCell(0).setCellValue("XXXX信息1");
titleRowTwo.getCell(0).setCellStyle(style1);
titleRowTwo.createCell(1).setCellStyle(style1);
titleRowTwo.createCell(2).setCellStyle(style1);
titleRowTwo.createCell(3).setCellStyle(style1);
titleRowTwo.createCell(4).setCellStyle(style1);
titleRowTwo.createCell(5).setCellStyle(style1);
CellRangeAddress regionTwo = new CellRangeAddress(1,1,6,11);
sheet.addMergedRegion(regionTwo);
HSSFCellStyle style2 = wb.createCellStyle();
HSSFFont font2 = wb.createFont();
font2.setFontName("宋体");
font2.setFontHeightInPoints((short) 11);
style2.setFont(font2);
style2.setAlignment(HSSFCellStyle.ALIGN_CENTER);
style2.setAlignment(HSSFCellStyle.VERTICAL_CENTER);
style2.setFillPattern(FillPatternType.SOLID_FOREGROUND);
style2.setFillForegroundColor(IndexedColors.GREEN.index);
wb.getCustomPalette().setColorAtIndex(IndexedColors.GREEN.index,(byte) 146,(byte) 208,(byte) 80);
style2.setBorderTop(BorderStyle.THIN);
style2.setTopBorderColor(IndexedColors.BLACK.index);
style2.setBorderBottom(BorderStyle.THIN);
style2.setBottomBorderColor(IndexedColors.BLACK.index);
style2.setBorderRight(BorderStyle.THIN);
style2.setRightBorderColor(IndexedColors.BLACK.index);
style2.getFont(wb).setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);
titleRowTwo.createCell(6).setCellValue("XXXX信息2");
titleRowTwo.getCell(6).setCellStyle(style2);
titleRowTwo.createCell(7).setCellStyle(style2);
titleRowTwo.createCell(8).setCellStyle(style2);
titleRowTwo.createCell(9).setCellStyle(style2);
titleRowTwo.createCell(10).setCellStyle(style2);
titleRowTwo.createCell(11).setCellStyle(style2);
CellRangeAddress regionThree = new CellRangeAddress(1,1,12,17);
sheet.addMergedRegion(regionThree);
HSSFCellStyle style3 = wb.createCellStyle();
HSSFFont font3 = wb.createFont();
font3.setFontName("宋体");
font3.setFontHeightInPoints((short) 11);
style3.setFont(font3);
style3.setAlignment(HSSFCellStyle.ALIGN_CENTER);
style3.setAlignment(HSSFCellStyle.VERTICAL_CENTER);
style3.setFillPattern(FillPatternType.SOLID_FOREGROUND);
style3.setFillForegroundColor(IndexedColors.ORANGE.index);
wb.getCustomPalette().setColorAtIndex(IndexedColors.ORANGE.index,(byte) 255,(byte) 192,(byte) 0);
style3.setBorderTop(BorderStyle.THIN);
style3.setTopBorderColor(IndexedColors.BLACK.index);
style3.setBorderBottom(BorderStyle.THIN);
style3.setBottomBorderColor(IndexedColors.BLACK.index);
style3.setBorderRight(BorderStyle.THIN);
style3.setRightBorderColor(IndexedColors.BLACK.index);
style3.getFont(wb).setBoldweight(HSSFFont.BOLDWEIGHT_BOLD);
titleRowTwo.createCell(12).setCellValue("XXXX信息3");
titleRowTwo.getCell(12).setCellStyle(style3);
titleRowTwo.createCell(13).setCellStyle(style3);
titleRowTwo.createCell(14).setCellStyle(style3);
titleRowTwo.createCell(15).setCellStyle(style3);
titleRowTwo.createCell(16).setCellStyle(style3);
titleRowTwo.createCell(17).setCellStyle(style3);
Row titleRowThree = sheet.createRow(2);
HSSFCellStyle style4 = wb.createCellStyle();
HSSFFont font4 = wb.createFont();
font4.setFontName("宋体");
font4.setFontHeightInPoints((short) 11);
style4.setFont(font4);
style4.setAlignment(HSSFCellStyle.ALIGN_CENTER);
style4.setAlignment(HSSFCellStyle.VERTICAL_CENTER);
style4.setBorderBottom(BorderStyle.THIN);
style4.setBottomBorderColor(IndexedColors.BLACK.index);
style4.setBorderRight(BorderStyle.THIN);
style4.setRightBorderColor(IndexedColors.BLACK.index);
titleRowThree.createCell(0).setCellValue("XXX0");
titleRowThree.getCell(0).setCellStyle(style4);
titleRowThree.createCell(1).setCellValue("XXX1");
titleRowThree.getCell(1).setCellStyle(style4);
titleRowThree.createCell(2).setCellValue("XXX2");
titleRowThree.getCell(2).setCellStyle(style4);
titleRowThree.createCell(3).setCellValue("XXX3");
titleRowThree.getCell(3).setCellStyle(style4);
titleRowThree.createCell(4).setCellValue("XXX4");
titleRowThree.getCell(4).setCellStyle(style4);
titleRowThree.createCell(5).setCellValue("XXX5");
titleRowThree.getCell(5).setCellStyle(style4);
titleRowThree.createCell(6).setCellValue("XXX6");
titleRowThree.getCell(6).setCellStyle(style4);
titleRowThree.createCell(7).setCellValue("XXX7");
titleRowThree.getCell(7).setCellStyle(style4);
titleRowThree.createCell(8).setCellValue("XXX8");
titleRowThree.getCell(8).setCellStyle(style4);
titleRowThree.createCell(9).setCellValue("XXX9");
titleRowThree.getCell(9).setCellStyle(style4);
titleRowThree.createCell(10).setCellValue("XXX10");
titleRowThree.getCell(10).setCellStyle(style4);
titleRowThree.createCell(11).setCellValue("XXX11");
titleRowThree.getCell(11).setCellStyle(style4);
titleRowThree.createCell(12).setCellValue("XXX12");
titleRowThree.getCell(12).setCellStyle(style4);
titleRowThree.createCell(13).setCellValue("XXX13");
titleRowThree.getCell(13).setCellStyle(style4);
titleRowThree.createCell(14).setCellValue("XXX14");
titleRowThree.getCell(14).setCellStyle(style4);
titleRowThree.createCell(15).setCellValue("XXX15");
titleRowThree.getCell(15).setCellStyle(style4);
titleRowThree.createCell(16).setCellValue("XXX16");
titleRowThree.getCell(16).setCellStyle(style4);
titleRowThree.createCell(17).setCellValue("XXX17");
titleRowThree.getCell(17).setCellStyle(style4);
效果图
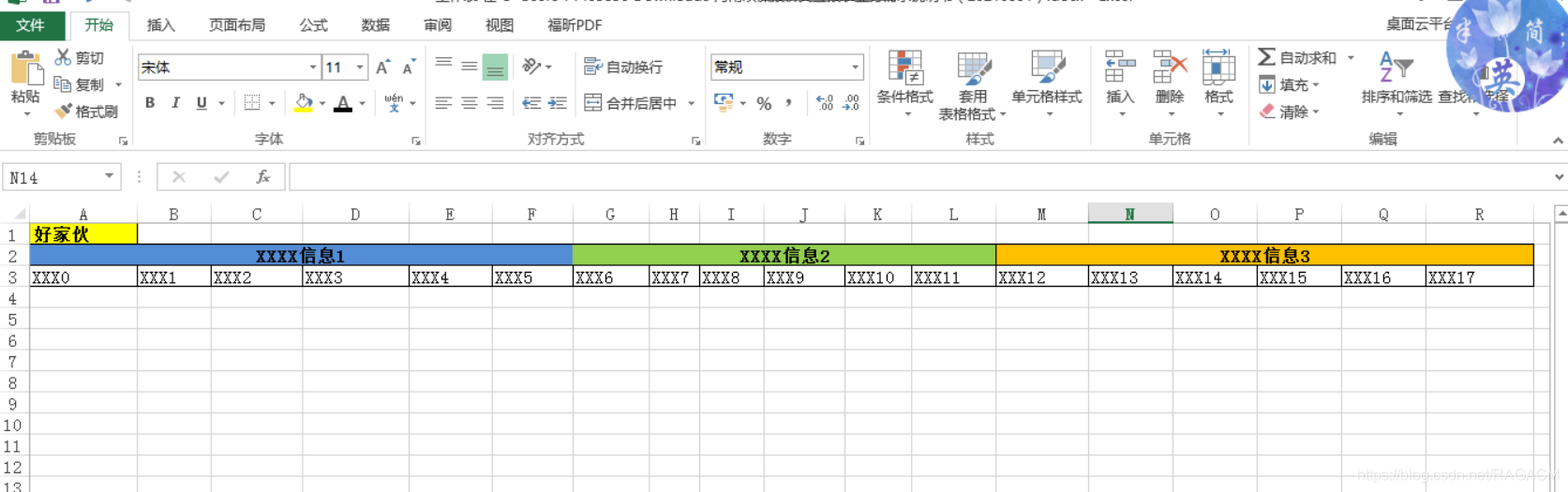