一. 单组数据的Table View
1. 创建工程后,直接把Table View拖到View Controller上
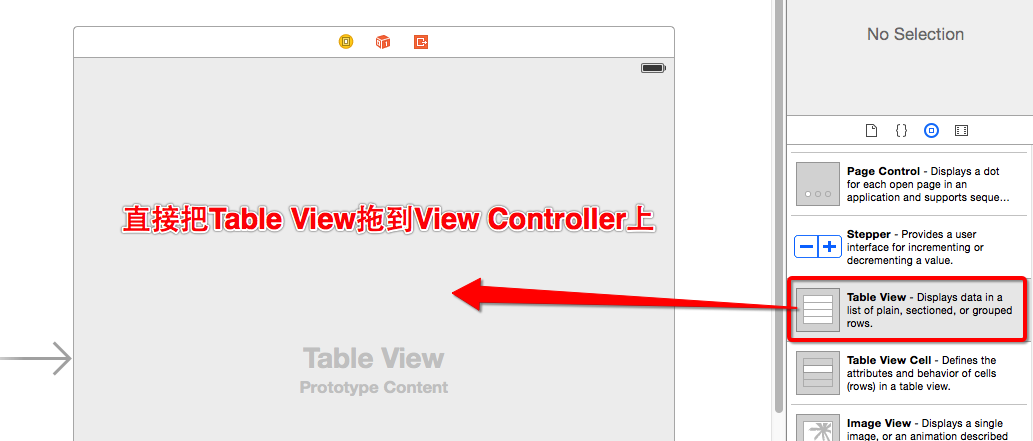
2. 拖线设置数据源
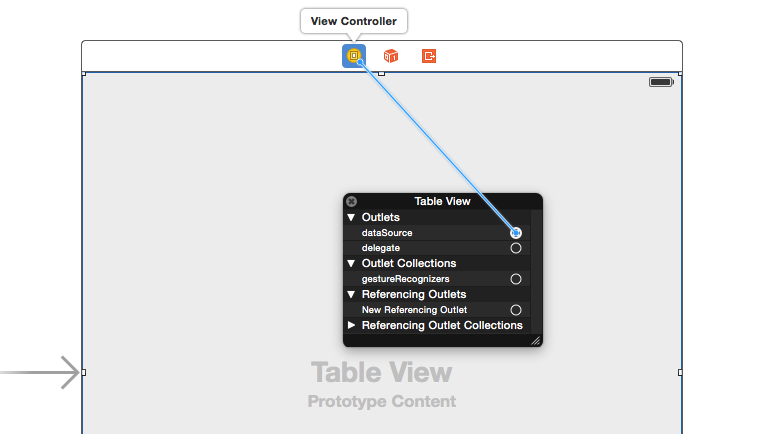
3. 创建数据模型
#import <Foundation/Foundation.h>
@interface SSData : NSObject
@property (nonatomic,strong) NSString *icon;
@property (nonatomic,strong) NSString *intro;
@property (nonatomic,strong) NSString *name;
- (instancetype)initWithDic:(NSDictionary *)dic;
+ (instancetype)dataWithDic:(NSDictionary *)dic;
@end
—————————————————————————————————————————————
#import "SSData.h"
@implementation SSData
- (instancetype)initWithDic:(NSDictionary *)dic {
if (self = [super init]) {
_icon = dic[@"icon"];
_name = dic[@"name"];
_intro = dic[@"intro"];
}
return self;
}
+ (instancetype)dataWithDic:(NSDictionary *)dic {
return [[self alloc] initWithDic:dic];
}
@end
4. 懒加载数据
(1). 在ViewController的扩展类中添加属性
@property (nonatomic,strong) NSArray *dataArray;
(2). 重写get方法实现懒加载
#pragma mark - table数据懒加载
- (NSArray *)dataArray {
if (_dataArray == nil) {
NSBundle *bundle = [NSBundle mainBundle];
NSString *path = [bundle pathForResource:@"heroes.plist" ofType:nil];
NSArray *dataArray = [NSArray arrayWithContentsOfFile:path];
NSMutableArray *dataM = [NSMutableArray array];
for (NSDictionary *dic in dataArray) {
SSData *data = [SSData dataWithDic:dic];
[dataM addObject:data];
}
_dataArray = dataM;
}
return _dataArray;
}
5. 实现数据源方法
#pragma mark - <UITableViewDataSource>实现
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
return 1;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
return self.dataArray.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [[UITableViewCell alloc] initWithStyle:UITableViewCellStyleSubtitle reuseIdentifier:nil];
SSData *data = self.dataArray[indexPath.row];
cell.textLabel.text = data.name;
cell.detailTextLabel.text = data.intro;
cell.imageView.image = [UIImage imageNamed:data.icon];
return cell;
}
- (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section {
return @"LOL英雄名称";
}
- (NSString *)tableView:(UITableView *)tableView titleForFooterInSection:(NSInteger)section {
return @"直到二十年前,符文之地才从战乱中解脱。这片大陆上的人民自远古以来就习惯结群而斗,用战争解决纷争。而不论何时,战争的工具始终都是魔法。";
}
二. 多组数据的Table View
1. 创建工程后,直接把Table View拖到View Controller上,同单组类型
2. 拖线设置数据源,同单组类型
3. 设置分组的类型,不设置默认为单组
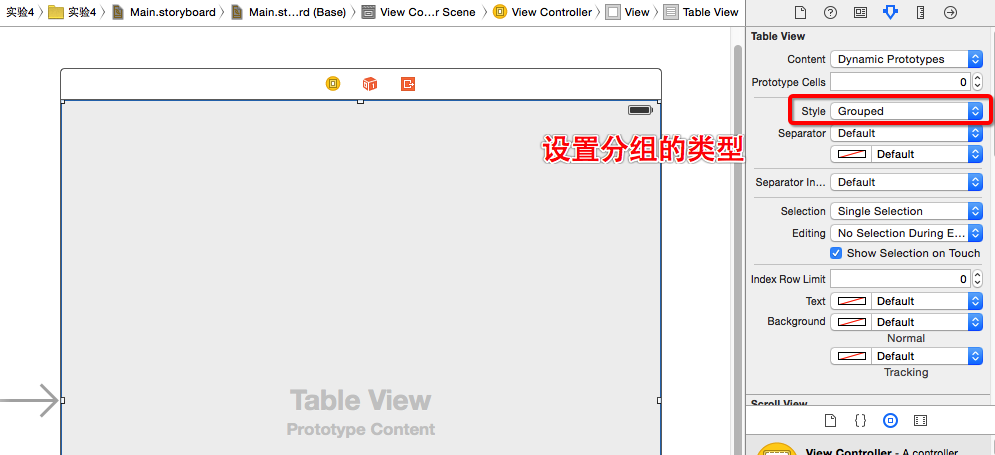
4. 创建数据模型
@interface SSData : NSObject
@property (nonatomic,strong) NSString *imageName;
@property (nonatomic,strong) NSString *carNmae;
+ (SSData *)dataWithDic:(NSDictionary *)dic;
@end
@implementation SSData
+ (SSData *)dataWithDic:(NSDictionary *)dic {
SSData *data = [[SSData alloc] init];
data.imageName = dic[@"imageName"];
data.carNmae = dic[@"carNmae"];
return data;
}
@end
5. 创建组模型
@interface SSGroup : NSObject
@property (nonatomic,strong) NSString *header;
@property (nonatomic,strong) NSString *footer;
@property (nonatomic,strong) NSArray *dataGroup;
@end
implementation SSGroup
@end
6. 懒加载数据
(1). 在ViewController的扩展类中添加属性
@property (nonatomic,strong) NSArray *dataGrup;
(2). 重写get方法实现懒加载
#pragma mark - 懒加载
- (NSArray *)dataGrup {
if (_dataGrup == nil) {
NSString *path = [[NSBundle mainBundle] pathForResource:@"cardata.plist" ofType:nil];
NSArray *arrData = [NSArray arrayWithContentsOfFile:path];
NSMutableArray *arrM1 = [NSMutableArray array];
NSMutableArray *arrM2 = [NSMutableArray array];
NSMutableArray *arrM3 = [NSMutableArray array];
for (NSDictionary *dic in arrData) {
SSData *data = [SSData dataWithDic:dic];
NSString *str = [data.imageName substringToIndex:1];
if ([str isEqualToString:@"A"]) {
[arrM1 addObject:data];
}
if ([str isEqualToString:@"B"]) {
[arrM2 addObject:data];
}
if ([str isEqualToString:@"C"]) {
[arrM3 addObject:data];
}
}
SSGroup *group1 = [[SSGroup alloc] init];
group1.header = @"欧美汽车";
group1.footer = @"世界一流";
group1.dataGroup = arrM1;
SSGroup *group2 = [[SSGroup alloc] init];
group2.header = @"日韩汽车";
group2.footer = @"无言以对";
group2.dataGroup = arrM2;
SSGroup *group3 = [[SSGroup alloc] init];
group3.header = @"天朝汽车";
group3.footer = @"无人能敌";
group3.dataGroup = arrM3;
_dataGrup = @[group1,group2,group3];
}
return _dataGrup;
}
5. 实现数据源方法
#pragma mark - <UITableViewDataSource>
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
return self.dataGrup.count;
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
SSGroup *group = self.dataGrup[section];
return group.dataGroup.count;
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [[UITableViewCell alloc] init];
SSGroup *group = self.dataGrup[indexPath.section];
SSData *data = group.dataGroup[indexPath.row];
cell.textLabel.text = data.carNmae;
cell.imageView.image = [UIImage imageNamed:data.imageName];
return cell;
}
- (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section {
SSGroup *group = self.dataGrup[section];
return group.header;
}
- (NSString *)tableView:(UITableView *)tableView titleForFooterInSection:(NSInteger)section {
NSLog(@"%@",@"E"); SSGroup *group = self.dataGrup[section];
return group.footer;
}
@end