Java容器类类库的用途是"保存对象",并将其划分为两个不同的概念
Collection:一个独立元素的序列,这些元素都服从一条或多条规则。
List必须按照插入的顺序保存元素,而Set不能有重复元素。
Queue按照排队规则来确定对象产生的顺序(通常与它们被插入的顺序相同)
.......
Map:一组成对的"键值对"对象,允许你使用键来查找值。
ArrayList允许你使用数字(下标)来查找值,因此在某种意义上,它将数字与对象关联在一起了。
映射表允许我们使用另一个对象来查找某个对象,也被称为"关联数组",因为它将某些对象与另外一些对象关联在了一起。
或者被称为"字典",因为你可以使用键对象来查找值对象。
Java集合框架主要结构图
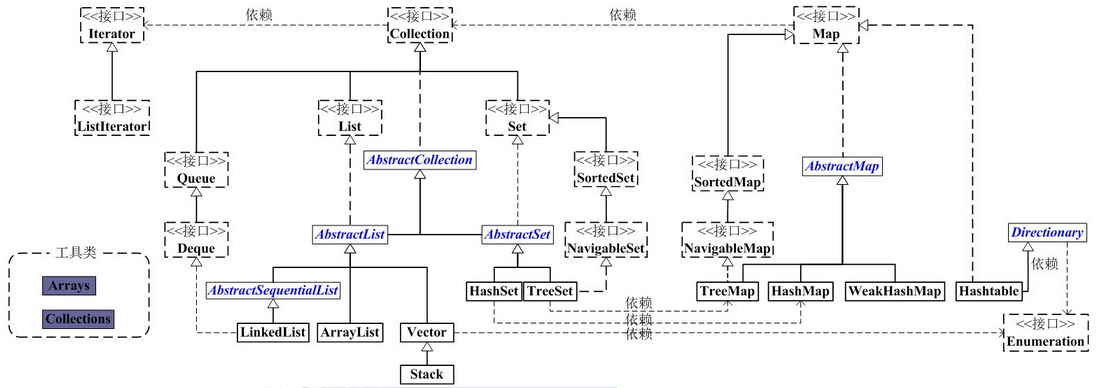
JDK源码注释
The root interface in the collection hierarchy. A collection represents a group of objects, known as its elements. Some collections allow duplicate elements and others do not. Some are ordered and others unordered. The JDK does not provide any direct implementations of this interface: it provides implementations of more specific subinterfaces like Set and List. This interface is typically used to pass collections around and manipulate them where maximum generality is desired.
Bags or multisets (unordered collections that may contain duplicate elements) should implement this interface directly.
All general-purpose Collection implementation classes (which typically implement Collection indirectly through one of its subinterfaces) should provide two "standard" constructors: a void (no arguments) constructor, which creates an empty collection, and a constructor with a single argument of type Collection, which creates a new collection with the same elements as its argument. In effect, the latter constructor allows the user to copy any collection, producing an equivalent collection of the desired implementation type. There is no way to enforce this convention (as interfaces cannot contain constructors) but all of the general-purpose Collection implementations in the Java platform libraries comply.
The "destructive" methods contained in this interface, that is, the methods that modify the collection on which they operate, are specified to throw UnsupportedOperationException if this collection does not support the operation. If this is the case, these methods may, but are not required to, throw an UnsupportedOperationException if the invocation would have no effect on the collection. For example, invoking the addAll(Collection) method on an unmodifiable collection may, but is not required to, throw the exception if the collection to be added is empty.
Some collection implementations have restrictions on the elements that they may contain. For example, some implementations prohibit null elements, and some have restrictions on the types of their elements. Attempting to add an ineligible element throws an unchecked exception, typically NullPointerException or ClassCastException. Attempting to query the presence of an ineligible element may throw an exception, or it may simply return false; some implementations will exhibit the former behavior and some will exhibit the latter. More generally, attempting an operation on an ineligible element whose completion would not result in the insertion of an ineligible element into the collection may throw an exception or it may succeed, at the option of the implementation. Such exceptions are marked as "optional" in the specification for this interface.
It is up to each collection to determine its own synchronization policy. In the absence of a stronger guarantee by the implementation, undefined behavior may result from the invocation of any method on a collection that is being mutated by another thread; this includes direct invocations, passing the collection to a method that might perform invocations, and using an existing iterator to examine the collection.
Many methods in Collections Framework interfaces are defined in terms of the equals method. For example, the specification for the contains(Object o) method says: "returns true if and only if this collection contains at least one element e such that (o==null ? e==null : o.equals(e))." This specification should not be construed to imply that invoking Collection.contains with a non-null argument o will cause o.equals(e) to be invoked for any element e. Implementations are free to implement optimizations whereby the equals invocation is avoided, for example, by first comparing the hash codes of the two elements. (The Object.hashCode() specification guarantees that two objects with unequal hash codes cannot be equal.) More generally, implementations of the various Collections Framework interfaces are free to take advantage of the specified behavior of underlying Object methods wherever the implementor deems it appropriate.
Some collection operations which perform recursive traversal of the collection may fail with an exception for self-referential instances where the collection directly or indirectly contains itself. This includes the clone(), equals(), hashCode() and toString() methods. Implementations may optionally handle the self-referential scenario, however most current implementations do not do so.
集合层次结构中的根接口。一个集合表示一组对象,称为其元素。有些集合允许重复元素,而其他集合不允许。有些是有序的,有些是无序的。JDK不提供这个接口的任何直接实现:它提供了更多特定子接口的实现,比如Set和List。这个接口通常用于传递集合并在需要最大普遍性的情况下处理它们。
包或多重包(可能包含重复元素的无序集合)应直接实现此接口。
所有通用的Collection实现类(通常通过其一个子接口间接实现Collection)应该提供两个“标准”构造函数:一个void(无参数)构造函数,它创建一个空集合,以及一个具有单类型参数的构造函数集合,它创建一个与它的参数具有相同元素的新集合。实际上,后者的构造函数允许用户复制任何集合,生成所需实现类型的等效集合。没有办法强制执行这个约定(因为接口不能包含构造函数),但是Java平台库中的所有通用Collection实现都符合。
如果此集合不支持该操作,则指定此接口中包含的“破坏性”方法,即修改它们在其上运行的集合的方法,以引发UnsupportedOperationException。如果是这种情况,那么这些方法可能(但不是必需)抛出一个UnsupportedOperationException,如果调用对集合没有影响的话。例如,如果要添加的集合为空,则在不可修改集合上调用addAll(Collection)方法可能(但不是必需)抛出异常。
一些集合实现对它们可能包含的元素有限制。例如,有些实现禁止使用空元素,有些实现对元素类型有限制。尝试添加不合格的元素会引发未检查的异常,通常为NullPointerException或ClassCastException。尝试查询不合格元素的存在可能会引发异常,或者它可能仅返回false;一些实现将展现前一种行为,一些将展现后者。更一般地说,尝试对不合格元素进行操作,其完成不会导致将不合格元素插入到集合中可能会引发异常,或者可能会成功执行。这种例外在该接口的规范中被标记为“可选”。
由每个集合决定其自己的同步策略。在没有实现的更强保证的情况下,未定义的行为可能是由另一个线程正在改变的集合上的任何方法的调用引起的;这包括直接调用,将集合传递给可能执行调用的方法,并使用现有的迭代器来检查集合。
集合框架接口中的许多方法是根据equals方法定义的。例如,contains(Object o)方法的规范说:“当且仅当此集合包含至少一个元素e以使得(o == null?e == null:o.equals(e))时,返回true “。本规范不应被解释为暗示使用非空参数调用Collection.contains o将导致任何元素e被调用o.equals(e)。实现可以自由地实现优化,从而避免了equals调用,例如,首先比较两个元素的哈希代码。 (Object.hashCode()规范保证两个哈希代码不相等的对象不能相等)。更一般地说,不同的集合框架接口的实现可以自由地利用底层Object方法的指定行为,只要实现者认为它适合。
执行集合的递归遍历的某些集合操作可能会失败,并且集合直接或间接包含其自身的自引用实例的异常。这包括clone(),equals(),hashCode()和toString()方法。实现可以选择处理自引用场景,但是大多数当前的实现不这样做。 |
Collection接口基本方法
• size()
获取元素个数
• isEmpty()
集合是否为空
• contains(Object)
集合中是否包含指定元素
• iterator()
获取迭代器
• toArray()
将集合转换为数组
• toArray(T[])
将集合转换为数组,并根据传入参数类型指定数组的类型
• add(E)
向集合中添加一个元素
• remove(Object)
删除集合中的一个元素
• containsAll(Collection<?>)
是否包含指定集合的全部元素
• addAll(Collection<? extends E>)
将某个集合中所有元素添加到本集合中
• removeAll(Collection<?>)
删除本集合中和某集合一致的元素
• clear()
删除集合中所有元素
JDK 1.8 中Controller 还新增了几个方法,这里暂时放着,和Stream一起看。