Rula is the Human Resources Manager at Mixed Dimensions Inc. She has some very delightful puzzles for the employees to enjoy their free time at the office. One of these puzzles is the Worm Puzzle.
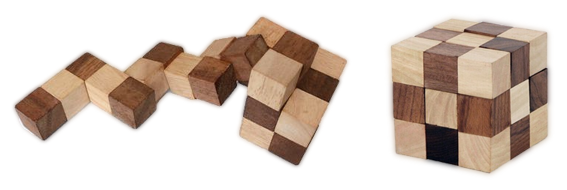
The puzzle consists of 27 cubic pieces of several types. The pieces are connected by a rope that runs through holes in the pieces, and allows the pieces to rotate. In order to solve the puzzle, you need to build a3x3x3 cube.
Each piece has one of the following three types:
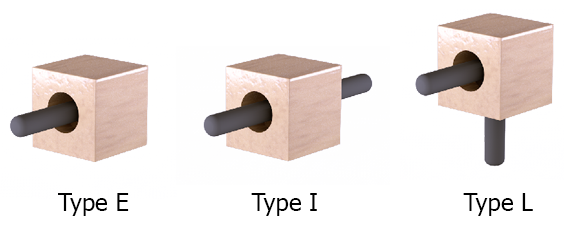
- Type E represents an end cube, it has a hole at one face only. This piece is always connected to only one other piece.
- Type I represents a cube which has two holes on opposite faces, the rope goes straight into one hole and out through the other.
- Type L represents a cube which has two holes in two adjacent faces, the rope enters through one face, makes a 90 degree turn, and exits through the other face.
Well, Hasan has cut the rope of the puzzle by mistake, of course he didn’t want to tell Rula about it, so he bought a new rope and linked the pieces together to make a new Worm Puzzle, but he wasn't sure whether he linked them in their original order or not.
Now Rula has been trying to solve the puzzle for two days, but with no success, although she used to solve it in 30 seconds. She started to suspect that something might be wrong with the puzzle.
She asked one of the engineers at Mixed Dimensions to verify whether the puzzle is solvable or not. Unfortunately, that engineer was Hasan, and of course, the question is difficult for him to answer.
Given the type of each piece, and the order in which Hasan linked the pieces together, can you help him by finding whether the given puzzle is solvable or not?
The input consists of a string of 27 letters, each letter will be either anE, I, orL, which represents the type of that piece.
The first and last letters are always E.
Print YES if the given puzzle is solvable, otherwise printNO.
EILILLLLLLILILLLLLLILILLLLE
YES
EILLLILLILLLILILLLLILILILIE
YES
EILLILLLILLILLLILLLILLLLILE
NO
题意:给出二十七个方块,问你能否组合成一股立方体,字母的意思看上图
题解:枚举起点,然后进行dfs,对于每一个字母的操作模拟一下相应的操作,注意方向即可
然后就是简单判断一下是否在限制条件内
注意:当时我做的时候,在字母E的时候也枚举了六个方向,导致超时了
因为刚开始已经枚举了起点了,只需要随意选择一个方向即可,这样不会导致枚举相同起点方向
#include<stdio.h>
#include<string.h>
#include<algorithm>
using namespace std;
char a[50];
int visit[5][5][5];
int dx[]={1,0,0,-1,0,0};
int dy[]={0,1,0,0,-1,0};
int dz[]={0,0,1,0,0,-1};
bool dfs(int x,int y,int z,int pos,int d)
{
if(pos>=27)
return true;
if(x>3||y>3||z>3||x<1||y<1||z<1)
return false;
if(visit[x][y][z])
return false;
visit[x][y][z]=1;
if(a[pos]=='E'&&pos!=0&&pos!=26)
return false;
if(a[pos]=='E'&&pos==26)
return true;
int flag=0;
if(a[pos]=='E'){
if(dfs(x+dx[0],y+dy[0],z+dz[0],pos+1,0)){
flag=1;
}
}
else if(a[pos]=='I')
flag=dfs(x+dx[d],y+dy[d],z+dz[d],pos+1,d);
else{
for(int i=0;i<6;i++){
if((i%3!=d%3)&&dfs(x+dx[i],y+dy[i],z+dz[i],pos+1,i)){
flag=1;
break;
}
}
}
visit[x][y][z]=0;
return flag;
}
bool deal()
{
memset(visit,0,sizeof(visit));
for(int i=1;i<=3;i++){
for(int j=1;j<=3;j++){
for(int k=1;k<=3;k++){
if(dfs(i,j,k,0,0)){
return 1;
}}}}
return 0;
}
int main()
{
//freopen("in.txt","r",stdin);
while(scanf("%s",a)!=EOF)
{
if(deal())
puts("YES");
else
puts("NO");
}
return 0;
}
Mixed Dimensions Onsite Round will host N contestants this year.
Moath and Saif have been preparing the contest hall, Moath needs X minutes to set up a computer for the contest, while Saif needs Y minutes to set up a computer. Each one of them works separately on one computer at a time.
Hasan is concerned they won't finish setting up the PCs in time, can you help Hasan figure out the minimum time required by Moath and Saif to set up all N PCs for the contest?
The input contains 3 integers, N, X, and Y (1 ≤ N, X, Y ≤ 109), the number of PCs to set up, the amount of minutes Moath needs to set up a computer, and the amount of minutes Saif needs to set up a computer, respectively.
On a single line, print one integer, the minimum number of minutes required by Moath and Saif to set up all the PCs for the contest.
5 3 4
9
100 10 1
91
题意:
一共n台电脑,A装机需要x分钟,B装机需要y分钟,问至少需要几分钟
题解:
二分时间,下界是 0 ,上界是 max(x,y)*n
#include<stdio.h>
#include<string.h>
#include<algorithm>
using namespace std;
int main()
{
long long n,x,y;
//freopen("in.txt","r",stdin);
while(scanf("%lld%lld%lld",&n,&x,&y)!=EOF)
{
if(x<y)
swap(x,y);
long long left=0,right=x*n,mid;
while(left<right)
{
mid=(left+right)>>1;
if((mid/x+mid/y)<n)
left=mid+1;
else
right=mid;
}
printf("%lld\n",right);
}
return 0;
}
Hamza is a new intern at Mixed Dimensions, and he's learning the basics about 3D models. Hasan gave him an easy task on his first day, he has to count the number of boundary edges in a 3D mesh.
A boundary edge in a 3D mesh is an edge that is a part of only one triangle. Hamza got so confused, and asked Hasan to simplify the problem. Hasan decided to give him only two triangles. But he still can’t figure it out. Given the names of the vertices that make up the two triangles, can you help Hamza find the number of boundary edges in the two triangles?
The input consists of two lines, each line describes one triangle, and contains 3 distinct integers from 1 to 6 which represent the names of the vertices of each triangle. It is guaranteed that the two triangles are different by at least one vertex.
Print one integer, the number of boundary edges in the two given triangles.
3 5 2 3 1 2
4
6 1 4 5 2 3
6
The first sample results in the following 2 triangles with 4 boundary edges (in green):
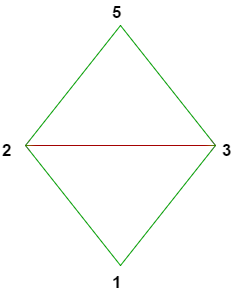
水题
题意:给出六个点,至多有两个点重合,求图中有几条边
题解:只有两种情况,一个是四条边(如上图),一个是两条边(也就是至多重合一个点的情况)
代码太简单,就不贴了
After Hasan ruined the Worm puzzle, Rula brought a new game to the office, the Card game.
The game has a group of cards, each card has an integer value Vi written on it.
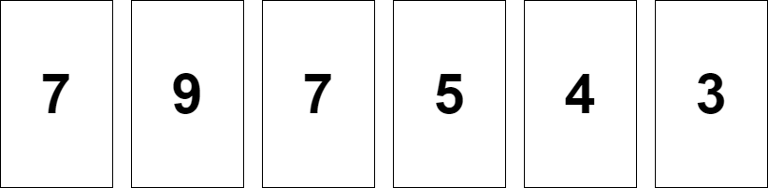
Initially, N cards are placed side by side in a straight line (refer to the picture above). In each turn you will pick two adjacent card sets (a single card is also considered a card set) and merge the cards of the two sets together to form one new set.
After each turn, you will add the maximum card value in each card set to your score. The game is over when no more merges could be performed.
What is the maximum score you can get at the end of the game, given that your initial score is 0?
The first line of input contains a single integer N (2 ≤ N ≤ 105), the number of cards in the game.
The second line contains N space-separated integers, each integer is Vi (1 ≤ Vi ≤ 5000), which represents the number written on the ith card.
On a single line, print the maximum score you can get.
5 1 2 3 2 1
23
6 7 9 7 5 4 3
108
One of the possible solutions for the first sample is as follows:
- Initially, each set will contain a single card: {1} {2} {3} {2} {1}.
- Merge {1} with {2}, the resulting sets are: {1 2} {3} {2} {1}, which will add (2 + 3 + 2 + 1 = 8) to your score.
- Merge {2} with {1}, the resulting sets are: {1 2} {3} {2 1}, which will add (2 + 3 + 2 = 7) to your score.
- Merge {1 2} with {3}, the resulting sets are: {1 2 3} {2 1}, which will add (3 + 2 = 5) to your score.
- Merge {1 2 3} with {2 1}, the resulting sets are: {1 2 3 2 1}, which will add 3 to your score.
Final score is 8 + 7 + 5 + 3 = 23.
水题:
题意:
给出一系列数字,每个数字看做一共集合,每次和并两个集合,直到最后只有一共集合
每次合并后计算每个集合最大值的和,求解这个和的最大值
题解:
最大的数出现n-1次,第二大的数出现n-2次,,,,,最小的数一次都没有出现在总和中
排序一下,然后O(n)计算一下即可
代码太简单就不贴了
Round 16,777,216 of Codeforce-is has just finished, and all the contestants were able to pass the pretests in problem A.
Maike, the founder of Codeforce-is wants to measure how weak the tests for problem A were, so he became interested in finding the amount of changes that will occur on the contest’s scoreboard after the system tests.
Given the score for problem A, the score of each contestant before the system tests, and the probability of failing the system tests for each contestant’s solution, can you help Maike find the expected number of pairs of contestants that will swap their relative order after the system tests? That is, the number of pairs of contestants i, j such that the scorei > scorej before the system tests, but scorei < scorej after the system tests.
The first line of input consists of two integers, N (2 ≤ N ≤ 105), and SA (1 ≤ SA ≤ 105), the number of contestants in the round, and the score the contestants achieved for passing the pretests of problem A.
The second line contains N space-separated integers, the Kth integer is TK (SA ≤ TK ≤ 105) which represents the total score in the contest for the Kth contestant before the system tests, Ti ≥ Ti + 1 for (1 ≤ i < N).
The third and final line contains N space-separated numbers, the Kth number is PK (0.01 ≤ PK ≤ 1.00) which represents the probability that the Kth contestant's solution will fail the system tests. Numbers are given with exactly two digits after the decimal point.
On a single line, output the expected number of pairs of contestants that will swap their relative order after the system tests are over.
2 10 25 20 0.50 0.50
0.250000000
4 10 25 20 15 15 1.00 0.50 1.00 0.50
0.750000000
题意:
给出原本n个人的分数,和这些人解A题解出来的概率,解出来就可以得到分数S,求解所有情况下可以反超的概率(后者分数比前者分数多)
题解:
枚举每一个人可以反超前面某些人的概率,这个人要是可以反超前者,就必须是前者一直停留在这里的概率乘以本身可以到达前面(超过)的概率
使用STL 中的upper_bound()函数和lower_bound( )函数,理解题意后自行看代码即懂
#include<stdio.h>
#include<string.h>
#include<algorithm>
using namespace std;
#define MAXN 100005
int a[MAXN];
double p[MAXN],sum[MAXN];
bool cmp(const int &a,const int &b)
{
return a>b;
}
int main()
{
int n,s;
freopen("in.txt","r",stdin);
while(scanf("%d%d",&n,&s)!=EOF)
{
sum[0]=0;
for(int i=1;i<=n;i++)
scanf("%d",&a[i]);
for(int i=1;i<=n;i++){
scanf("%lf",&p[i]);
sum[i]=sum[i-1]+p[i];
}
double ans=0;
int left,right;
for(int i=1;i<=n;i++){
left=upper_bound(a+1,a+i,a[i]+s,cmp)-a;
right=lower_bound(a+1,a+i,a[i],cmp)-a;
ans+=(sum[right-1]-sum[left-1])*(1-p[i]);
}
printf("%0.9lf\n",ans);
}
return 0;
}