介绍:
用户在浏览器端注册账号,注册信息会传到数据库(不允许重复的用户名)。然后用户可根据已经注册的账号进行登录
效果图: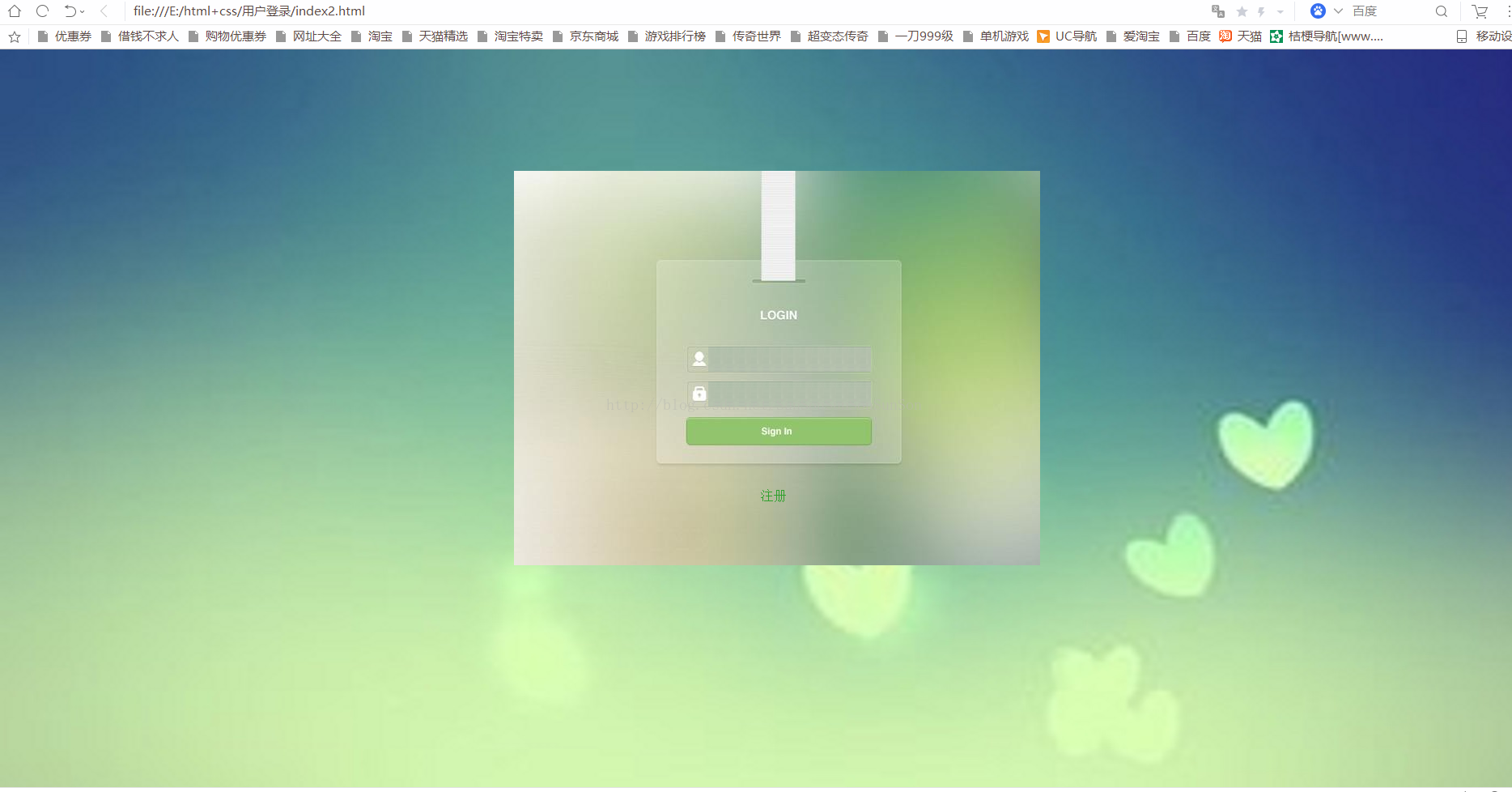
源码:
用户注册html+css:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="css/style.css">
<title>用户登录</title>
</head>
<body style="background:url('images/bg.jpg');">
<div class="bg">
<form method="get" action="http://localhost:8887/index.html">
<div class="name"><input type="text" name="name" value=" "></div>
<div class="password"><input type="password" name="password" value=""></div>
<div class="enter"><input type="submit" value=""></div>
<html lang="en">
<head>
<meta charset="UTF-8">
<link rel="stylesheet" href="css/style.css">
<title>用户登录</title>
</head>
<body style="background:url('images/bg.jpg');">
<div class="bg">
<form method="get" action="http://localhost:8887/index.html">
<div class="name"><input type="text" name="name" value=" "></div>
<div class="password"><input type="password" name="password" value=""></div>
<div class="enter"><input type="submit" value=""></div>
</form>
<div><a href="file:///E:/html+css/数据库模拟用户注册/index.html" target="block">注册</a></div>
</div>
</body>
</html>
*{margin:0;padding:0;}
.bg{
background:url("../images/ending.jpg");
height:487px;
width:650px;
margin:150px auto;
}
.bg .name{
background:url("../images/input.jpg") repeat-x;
width:201px;
height:31px;
position:relative;
top:217px;
left:240px;
}
.bg .name input{
width:201px;
height:31px;
background:none;
border:0;
outline:none;
}
.bg .password{
background:url("../images/input.jpg") repeat-x;
width:201px;
height:31px;
position:relative;
top:229px;
left:240px;
}
.bg .password input{
width:201px;
height:31px;
background:none;
border:0;
outline:none;
}
.bg .enter{
width:228px;
height:33px;
position:relative;
top:243px;
left:213px;
}
.bg .enter input{
width:228px;
height:33px;
background:none;
border:0;
outline:none;
}
.bg div{
width:40px;
height:20px;
position:relative;
top:297px;
left:305px;
}
.bg div a{
color:#2EA02C;
text-decoration:none;
}
.bg div a:hover{
color:#F50303;
}
<div><a href="file:///E:/html+css/数据库模拟用户注册/index.html" target="block">注册</a></div>
</div>
</body>
</html>
*{margin:0;padding:0;}
.bg{
background:url("../images/ending.jpg");
height:487px;
width:650px;
margin:150px auto;
}
.bg .name{
background:url("../images/input.jpg") repeat-x;
width:201px;
height:31px;
position:relative;
top:217px;
left:240px;
}
.bg .name input{
width:201px;
height:31px;
background:none;
border:0;
outline:none;
}
.bg .password{
background:url("../images/input.jpg") repeat-x;
width:201px;
height:31px;
position:relative;
top:229px;
left:240px;
}
.bg .password input{
width:201px;
height:31px;
background:none;
border:0;
outline:none;
}
.bg .enter{
width:228px;
height:33px;
position:relative;
top:243px;
left:213px;
}
.bg .enter input{
width:228px;
height:33px;
background:none;
border:0;
outline:none;
}
.bg div{
width:40px;
height:20px;
position:relative;
top:297px;
left:305px;
}
.bg div a{
color:#2EA02C;
text-decoration:none;
}
.bg div a:hover{
color:#F50303;
}
用户注册java服务器端代码:
import java.io.IOException;
import java.net.ServerSocket;
/**
* 服务器程序
* @author 27895
*
*/
public class Server {
private ServerSocket ser;
private boolean Runnable = true;
public static void main(String[] args) {
Server server = new Server();
server.start();
}
/**
* 启动程序方法
* @param port
*/
public void start(int port){
try {
ser = new ServerSocket(port);
//接收客户端的消息
this.receive();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 重写start()方法
*/
public void start(){
start(8887);
}
/**
* 接收来自客户端的消息
*/
public void receive(){
while(Runnable){
try {
new Thread(new Execute(ser.accept())).start();
} catch (IOException e) {
//e.printStackTrace();
stop();
}
}
}
public void stop(){
Runnable = false;
}
}
import java.net.ServerSocket;
/**
* 服务器程序
* @author 27895
*
*/
public class Server {
private ServerSocket ser;
private boolean Runnable = true;
public static void main(String[] args) {
Server server = new Server();
server.start();
}
/**
* 启动程序方法
* @param port
*/
public void start(int port){
try {
ser = new ServerSocket(port);
//接收客户端的消息
this.receive();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 重写start()方法
*/
public void start(){
start(8887);
}
/**
* 接收来自客户端的消息
*/
public void receive(){
while(Runnable){
try {
new Thread(new Execute(ser.accept())).start();
} catch (IOException e) {
//e.printStackTrace();
stop();
}
}
}
public void stop(){
Runnable = false;
}
}
/**
* 接收客户端的消息
* @author 27895
*
*/
public class Receive {
private InputStream is;
private Map<String,String> map;
/**
* 写构造器
* @param client
*/
public Receive(Socket client){
map = new HashMap<String,String>();
try {
is = client.getInputStream();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 获取客户端的信息
*/
public String Get(int length){
try {
byte[] date = new byte[length];
int len = is.read(date);
String msg = decode(new String(date,0,len),"utf-8");
return msg;
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
/**
* 解决编码问题
* @param value
* @param code
* @return
*/
public String decode(String value,String code){
try {
return java.net.URLDecoder.decode(value,code);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
return null;
}
/**\
* 分析客户端的请求
* @param msg
* @return
*/
public Map<String,String> analysis(String msg){
//获取首行的字符串 包含姓名 密码
String str = msg.substring((msg.indexOf("?")+1),(msg.indexOf("HTTP/"))-1);
//截取姓名和密码
String[] arr = str.split("&");
//将姓名和密码存入Map集合中
for(int i=0;i<arr.length;i++){
String[] arrs = arr[i].split("=");
map.put(arrs[0], arrs[1]);
}
return map;
}
/**
* 重写annalysis方法
*/
public Map<String,String> analysis(){
Map<String,String> maps = analysis(Get(20480));
return maps;
}
}
* 接收客户端的消息
* @author 27895
*
*/
public class Receive {
private InputStream is;
private Map<String,String> map;
/**
* 写构造器
* @param client
*/
public Receive(Socket client){
map = new HashMap<String,String>();
try {
is = client.getInputStream();
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 获取客户端的信息
*/
public String Get(int length){
try {
byte[] date = new byte[length];
int len = is.read(date);
String msg = decode(new String(date,0,len),"utf-8");
return msg;
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
/**
* 解决编码问题
* @param value
* @param code
* @return
*/
public String decode(String value,String code){
try {
return java.net.URLDecoder.decode(value,code);
} catch (UnsupportedEncodingException e) {
e.printStackTrace();
}
return null;
}
/**\
* 分析客户端的请求
* @param msg
* @return
*/
public Map<String,String> analysis(String msg){
//获取首行的字符串 包含姓名 密码
String str = msg.substring((msg.indexOf("?")+1),(msg.indexOf("HTTP/"))-1);
//截取姓名和密码
String[] arr = str.split("&");
//将姓名和密码存入Map集合中
for(int i=0;i<arr.length;i++){
String[] arrs = arr[i].split("=");
map.put(arrs[0], arrs[1]);
}
return map;
}
/**
* 重写annalysis方法
*/
public Map<String,String> analysis(){
Map<String,String> maps = analysis(Get(20480));
return maps;
}
}
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Map;
/**
* 将用户名、密码和数据库做对比
* @author 27895
*
*/
public class Datebass {
private Map<String,String> map;
/**
* 写构造器
* @param map
*/
public Datebass(Map<String,String> map){
this.map = map;
}
/**
* 加载数据库
*/
public Connection loading(){
try {
//加载类驱动
Class.forName("com.mysql.jdbc.Driver");
//建立连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/register","root","wydfo8888.");
return conn;
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
return null;
}
/**
* 将用户名和数据库做对比
*/
public void constrast(){
Connection conn = loading();
try {
String exist = "select * from register_user where username=?";
PreparedStatement ps = conn.prepareStatement(exist);
ps.setObject(1,map.get("name").trim());
ResultSet rs = ps.executeQuery();
//判断用户名是否正确
if(rs.next()){
//用户名存在
//从数据库取出该用户
//String name = rs.getString(1);
String password = rs.getString(4);
//将密码做对比
if(password.equals(map.get("password").trim())){
Execute.name = true;
Execute.password = true;
}else{
Execute.name = true;
Execute.password = false;
}
}else{
Execute.name = false;
Execute.password = true;
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Map;
/**
* 将用户名、密码和数据库做对比
* @author 27895
*
*/
public class Datebass {
private Map<String,String> map;
/**
* 写构造器
* @param map
*/
public Datebass(Map<String,String> map){
this.map = map;
}
/**
* 加载数据库
*/
public Connection loading(){
try {
//加载类驱动
Class.forName("com.mysql.jdbc.Driver");
//建立连接
Connection conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/register","root","wydfo8888.");
return conn;
} catch (ClassNotFoundException | SQLException e) {
e.printStackTrace();
}
return null;
}
/**
* 将用户名和数据库做对比
*/
public void constrast(){
Connection conn = loading();
try {
String exist = "select * from register_user where username=?";
PreparedStatement ps = conn.prepareStatement(exist);
ps.setObject(1,map.get("name").trim());
ResultSet rs = ps.executeQuery();
//判断用户名是否正确
if(rs.next()){
//用户名存在
//从数据库取出该用户
//String name = rs.getString(1);
String password = rs.getString(4);
//将密码做对比
if(password.equals(map.get("password").trim())){
Execute.name = true;
Execute.password = true;
}else{
Execute.name = true;
Execute.password = false;
}
}else{
Execute.name = false;
Execute.password = true;
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
/**
* 服务器应答程序
* @author 27895
*
*/
public class Response {
private BufferedWriter bw;
private StringBuilder sb;
private int code = 0;
private String info = "";
private int len = 0;
//定义两个常量
public static final String CRLF = "\r\n";
public static final String BLANK = " ";
public Response(Socket client,int code,String info){
this.code = code;
this.info = info;
sb = new StringBuilder();
try {
bw = new BufferedWriter(new OutputStreamWriter(client.getOutputStream()));
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 封装头信息
*/
public void Headinfo(){
//1.HTTP协议版本、状态代码、描述
sb.append("HTTP/1.1").append(BLANK).append(code).append(BLANK);
switch(code){
case 200:
sb.append("OK");
break;
case 404:
sb.append("NOT FOUND");
break;
case 505:
sb.append("SEVER ERROR");
break;
default:
break;
}
sb.append(CRLF);
//2.响应头(Response Head)
sb.append("Server:Apache").append(BLANK).append("Tomcat/6.0.12").append(CRLF);
sb.append("Data:").append(new Date()).append(CRLF);
sb.append("Content-type:text/html;charset=GBK").append(CRLF);
//正文长度:字节长度
sb.append("Content-Length:").append(len).append(CRLF).append(CRLF);
}
/**
* 构建正文
* @param info
* @return
*/
public StringBuilder content(String info){
StringBuilder content = new StringBuilder();
len += info.getBytes().length;
content.append(info);
return content;
}
/**
* 推送信息到客户端
*/
public void PushToClient(){
//构建正文 计算正文的长度
StringBuilder msg = content(info);
//构建头信息
Headinfo();
//将正文加入到信息中
sb.append(msg);
//通过流推送到客户端
try {
bw.write(sb.toString());
bw.flush();
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
* 服务器应答程序
* @author 27895
*
*/
public class Response {
private BufferedWriter bw;
private StringBuilder sb;
private int code = 0;
private String info = "";
private int len = 0;
//定义两个常量
public static final String CRLF = "\r\n";
public static final String BLANK = " ";
public Response(Socket client,int code,String info){
this.code = code;
this.info = info;
sb = new StringBuilder();
try {
bw = new BufferedWriter(new OutputStreamWriter(client.getOutputStream()));
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* 封装头信息
*/
public void Headinfo(){
//1.HTTP协议版本、状态代码、描述
sb.append("HTTP/1.1").append(BLANK).append(code).append(BLANK);
switch(code){
case 200:
sb.append("OK");
break;
case 404:
sb.append("NOT FOUND");
break;
case 505:
sb.append("SEVER ERROR");
break;
default:
break;
}
sb.append(CRLF);
//2.响应头(Response Head)
sb.append("Server:Apache").append(BLANK).append("Tomcat/6.0.12").append(CRLF);
sb.append("Data:").append(new Date()).append(CRLF);
sb.append("Content-type:text/html;charset=GBK").append(CRLF);
//正文长度:字节长度
sb.append("Content-Length:").append(len).append(CRLF).append(CRLF);
}
/**
* 构建正文
* @param info
* @return
*/
public StringBuilder content(String info){
StringBuilder content = new StringBuilder();
len += info.getBytes().length;
content.append(info);
return content;
}
/**
* 推送信息到客户端
*/
public void PushToClient(){
//构建正文 计算正文的长度
StringBuilder msg = content(info);
//构建头信息
Headinfo();
//将正文加入到信息中
sb.append(msg);
//通过流推送到客户端
try {
bw.write(sb.toString());
bw.flush();
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
import java.net.Socket;
import java.util.HashMap;
import java.util.Map;
/**
* 通过加入线程进行操作
* @author 27895
*
*/
public class Execute implements Runnable{
private Receive receive;
private Response response;
private String info;
private int code = 200;
private Map<String,String> map;
public static boolean name = true;
public static boolean password = true;
private Socket client;
public Execute(Socket client){
map = new HashMap<String,String>();
receive = new Receive(client);
info = new String();
this.client = client;
}
/**
* 用户名错误
*/
public void usernameerr(){
info = "<html><head><title>返回信息</title><style>*{margin:0;padding:0;}"
+ ".img{margin:319px 700px;}body{background:pink;}"
+ "h1{color:#03A9F4;text-align:center;line-height:400px;}"
+ ".dd{background:red;height:400px;width:400px;margin: 200px 768px;}"
+ "</style></head><body><div class=\"dd\"><h1>登录失败 用户不存在!!!</h1>"
+ "</div></body></html>";
code = 404;
}
/**
* 密码错误
*/
public void passworderr(){
info = "<html><head><title>返回信息</title><style>*{margin:0;padding:0;}"
+ ".img{margin:319px 700px;}body{background:pink;}"
+ "h1{color:#03A9F4;text-align:center;line-height:400px;}"
+ ".dd{background:red;height:400px;width:400px;margin: 200px 768px;}"
+ "</style></head><body><div class=\"dd\"><h1>登录失败 密码不正确!!!</h1>"
+ "</div></body></html>";
code = 404;
}
/**
* 账户正确
*/
public void success(){
info = "<html><head><title>返回信息</title><style>*{margin:0;padding:0;}"
+ ".img{margin:319px 700px;}body{background:pink;}"
+ "h1{position:relative;top:334px;left:900px;color:#03A9F4;}"
+ ".d a{color:blue;text-decoration:none;}.d a:hover{color:red;}"
+ ".d{position:relative;top:375px;left:961px;}</style></head><body>"
+ "<h1>登录成功!!!</h1><div class=\"d\"><strong>"
+ "<a href=\"file:///E:/html+css/我的网页制作/index.html\" target=\"block\">"
+ "点击跳转</a></strong></div></body></html>";
code = 200;
}
public void run(){
//接收客户端的消息
map = receive.analysis();
//将数据传到数据库
Datebass db = new Datebass(map);
//进行校验
db.constrast();
//推送到客户端
if(!name){
usernameerr();
}else if(name && !password){
passworderr();
}else{
success();
}
response = new Response(client,code,info);
//推送
response.PushToClient();
//清空
name = true;
password = true;
info = "";
}
}
import java.util.HashMap;
import java.util.Map;
/**
* 通过加入线程进行操作
* @author 27895
*
*/
public class Execute implements Runnable{
private Receive receive;
private Response response;
private String info;
private int code = 200;
private Map<String,String> map;
public static boolean name = true;
public static boolean password = true;
private Socket client;
public Execute(Socket client){
map = new HashMap<String,String>();
receive = new Receive(client);
info = new String();
this.client = client;
}
/**
* 用户名错误
*/
public void usernameerr(){
info = "<html><head><title>返回信息</title><style>*{margin:0;padding:0;}"
+ ".img{margin:319px 700px;}body{background:pink;}"
+ "h1{color:#03A9F4;text-align:center;line-height:400px;}"
+ ".dd{background:red;height:400px;width:400px;margin: 200px 768px;}"
+ "</style></head><body><div class=\"dd\"><h1>登录失败 用户不存在!!!</h1>"
+ "</div></body></html>";
code = 404;
}
/**
* 密码错误
*/
public void passworderr(){
info = "<html><head><title>返回信息</title><style>*{margin:0;padding:0;}"
+ ".img{margin:319px 700px;}body{background:pink;}"
+ "h1{color:#03A9F4;text-align:center;line-height:400px;}"
+ ".dd{background:red;height:400px;width:400px;margin: 200px 768px;}"
+ "</style></head><body><div class=\"dd\"><h1>登录失败 密码不正确!!!</h1>"
+ "</div></body></html>";
code = 404;
}
/**
* 账户正确
*/
public void success(){
info = "<html><head><title>返回信息</title><style>*{margin:0;padding:0;}"
+ ".img{margin:319px 700px;}body{background:pink;}"
+ "h1{position:relative;top:334px;left:900px;color:#03A9F4;}"
+ ".d a{color:blue;text-decoration:none;}.d a:hover{color:red;}"
+ ".d{position:relative;top:375px;left:961px;}</style></head><body>"
+ "<h1>登录成功!!!</h1><div class=\"d\"><strong>"
+ "<a href=\"file:///E:/html+css/我的网页制作/index.html\" target=\"block\">"
+ "点击跳转</a></strong></div></body></html>";
code = 200;
}
public void run(){
//接收客户端的消息
map = receive.analysis();
//将数据传到数据库
Datebass db = new Datebass(map);
//进行校验
db.constrast();
//推送到客户端
if(!name){
usernameerr();
}else if(name && !password){
passworderr();
}else{
success();
}
response = new Response(client,code,info);
//推送
response.PushToClient();
//清空
name = true;
password = true;
info = "";
}
}
用户注册html+css代码:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>模拟用户注册</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body style="background:url('images/bg.jpg');">
<div class="yanzheng">
<div class="music">
<img src="images/music.jpg" alt="无图片" class="img">
<input type="button" value="" id="play">
</div>
<form method="get" action="http://localhost:8888/index.html">
<div class="bg">
<div class="logo"></div>
<div class="name">
<div class="qian"></div>
<div class="zhong"><span><input type="text" value="" name="name"></span></div>
<div class="hou"></div>
</div>
<div class="email">
<div class="qian"></div>
<div class="hou"><span><input type="text" value=" " name="email"></span></div>
</div>
<div class="password">
<div class="qian"></div>
<div class="zhong"><span><input type="password" value="" name="password"></span></div>
<div class="hou"></div>
</div>
<div class="enter">
<input type="submit" value="">
</div>
</div>
</form>
</div>
<audio src="music/1.mp3" id="music" loop="loop"></audio>
<script src="js/jquery.js"></script>
<script src="js/tz_mini.js"></script>
<script>
//当前页面加载完后执行这个方法
$(document).ready(function(){
//开启拖拽
$(".yanzheng").draggable();
/*
$('#music').get(0).addEventListener("timeupdate",function(){
//获取当前时间
var time = parseInt(this.currentTime);
if(time === 270){
$("#music").get(0).play();
}
});*/
//点击按钮播放音乐
var mark = true;
$("#play").click(function(){
if(mark){
//开始播放音乐
$("#music").get(0).play();
$(".img").addClass("rotateZ");
mark=false;
}else{
//停止音乐
$("#music").get(0).pause();
$(".img").removeClass("rotateZ");
mark=true;
}
});
});
</script>
</body>
</html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>模拟用户注册</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body style="background:url('images/bg.jpg');">
<div class="yanzheng">
<div class="music">
<img src="images/music.jpg" alt="无图片" class="img">
<input type="button" value="" id="play">
</div>
<form method="get" action="http://localhost:8888/index.html">
<div class="bg">
<div class="logo"></div>
<div class="name">
<div class="qian"></div>
<div class="zhong"><span><input type="text" value="" name="name"></span></div>
<div class="hou"></div>
</div>
<div class="email">
<div class="qian"></div>
<div class="hou"><span><input type="text" value=" " name="email"></span></div>
</div>
<div class="password">
<div class="qian"></div>
<div class="zhong"><span><input type="password" value="" name="password"></span></div>
<div class="hou"></div>
</div>
<div class="enter">
<input type="submit" value="">
</div>
</div>
</form>
</div>
<audio src="music/1.mp3" id="music" loop="loop"></audio>
<script src="js/jquery.js"></script>
<script src="js/tz_mini.js"></script>
<script>
//当前页面加载完后执行这个方法
$(document).ready(function(){
//开启拖拽
$(".yanzheng").draggable();
/*
$('#music').get(0).addEventListener("timeupdate",function(){
//获取当前时间
var time = parseInt(this.currentTime);
if(time === 270){
$("#music").get(0).play();
}
});*/
//点击按钮播放音乐
var mark = true;
$("#play").click(function(){
if(mark){
//开始播放音乐
$("#music").get(0).play();
$(".img").addClass("rotateZ");
mark=false;
}else{
//停止音乐
$("#music").get(0).pause();
$(".img").removeClass("rotateZ");
mark=true;
}
});
});
</script>
</body>
</html>
*{margin:0;padding:0;}
.bg{
background:url("../images/捕获.PNG");
width:810px;
height:702px;
margin:100px auto;
}
.bg .logo{
background:url("../images/logo.jpg");
width:214px;
height:71px;
position:relative;
top:95px;
left:305px;
}
.bg .name{
height:77px;
width:472px;
position:relative;
top:142px;
left:170px;
}
.bg .name .qian{
background:url("../images/nameqian.jpg");
height:77px;
width:62px;
float:left;
}
.bg .name .zhong{
background:url("../images/namezhong.jpg") repeat-x;
height:77px;
width:355px;
float:left;
}
.bg .name .hou{
background:url("../images/namehou.jpg");
height:77px;
width:55px;
float:right;
}
.bg .name .zhong span{
display:block;
height:77px;
width:100%;
}
.bg .name .zhong span input{
background:none;
height:77px;
width:100%;
outline:none;
border:0;
font-size:40px;
line-height:40px;
}
.bg .email{
height:73px;
width:472px;
position:relative;
top:144px;
left:170px;
}
.bg .email .qian{
background:url("../images/emailqian.jpg");
height:73px;
width:57px;
float:left;
}
.bg .email .hou{
background:url("../images/emailhou.jpg") repeat-x;
height:73px;
width:415px;
float:left;
}
.bg .email .hou span{
display:block;
height:73px;
width:100%;
}
.bg .email .hou span input{
background:none;
height:73px;
width:100%;
outline:none;
border:0;
font-size:40px;
line-height:40px;
}
.bg .password{
height:77px;
width:473px;
position:relative;
top:145px;
left:169px;
}
.bg .password .qian{
background:url("../images/passwordqian.jpg");
height:77px;
width:58px;
float:left;
}
.bg .password .zhong{
background:url("../images/passwordzhong.jpg") repeat-x;
height:77px;
width:363px;
float:left;
}
.bg .password .hou{
background:url("../images/passwordhou.jpg");
height:77px;
width:52px;
float:right;
}
.bg .password .zhong span{
display:block;
height:77px;
width:100%;
}
.bg .password .zhong span input{
background:none;
height:77px;
width:100%;
outline:none;
border:0;
font-size:40px;
line-height:40px;
}
.enter{
height:85px;
width:473px;
position:relative;
top:222px;
left:170px;
}
.enter input{
background:none;
height:85px;
width:473px;
border:0;
outline:none;
}
.music img{
box-shadow:0 0 6px #fff;
border-radius:50%;
}
.music{
height:30px;
width:30px;
position:relative;
top:160px;
left:1300px;
}
.rotateZ{animation:run 15s linear infinite}
/*定义一个关键帧*/
@keyframes run{
from{transform:rotate(0deg)}
to{transform:rotate(360deg)}
}
.music input{
background:none;
border:0;
outline:none;
width:22px;
height:22px;
position:relative;
top:-31px;
left:4px;
}
.bg{
background:url("../images/捕获.PNG");
width:810px;
height:702px;
margin:100px auto;
}
.bg .logo{
background:url("../images/logo.jpg");
width:214px;
height:71px;
position:relative;
top:95px;
left:305px;
}
.bg .name{
height:77px;
width:472px;
position:relative;
top:142px;
left:170px;
}
.bg .name .qian{
background:url("../images/nameqian.jpg");
height:77px;
width:62px;
float:left;
}
.bg .name .zhong{
background:url("../images/namezhong.jpg") repeat-x;
height:77px;
width:355px;
float:left;
}
.bg .name .hou{
background:url("../images/namehou.jpg");
height:77px;
width:55px;
float:right;
}
.bg .name .zhong span{
display:block;
height:77px;
width:100%;
}
.bg .name .zhong span input{
background:none;
height:77px;
width:100%;
outline:none;
border:0;
font-size:40px;
line-height:40px;
}
.bg .email{
height:73px;
width:472px;
position:relative;
top:144px;
left:170px;
}
.bg .email .qian{
background:url("../images/emailqian.jpg");
height:73px;
width:57px;
float:left;
}
.bg .email .hou{
background:url("../images/emailhou.jpg") repeat-x;
height:73px;
width:415px;
float:left;
}
.bg .email .hou span{
display:block;
height:73px;
width:100%;
}
.bg .email .hou span input{
background:none;
height:73px;
width:100%;
outline:none;
border:0;
font-size:40px;
line-height:40px;
}
.bg .password{
height:77px;
width:473px;
position:relative;
top:145px;
left:169px;
}
.bg .password .qian{
background:url("../images/passwordqian.jpg");
height:77px;
width:58px;
float:left;
}
.bg .password .zhong{
background:url("../images/passwordzhong.jpg") repeat-x;
height:77px;
width:363px;
float:left;
}
.bg .password .hou{
background:url("../images/passwordhou.jpg");
height:77px;
width:52px;
float:right;
}
.bg .password .zhong span{
display:block;
height:77px;
width:100%;
}
.bg .password .zhong span input{
background:none;
height:77px;
width:100%;
outline:none;
border:0;
font-size:40px;
line-height:40px;
}
.enter{
height:85px;
width:473px;
position:relative;
top:222px;
left:170px;
}
.enter input{
background:none;
height:85px;
width:473px;
border:0;
outline:none;
}
.music img{
box-shadow:0 0 6px #fff;
border-radius:50%;
}
.music{
height:30px;
width:30px;
position:relative;
top:160px;
left:1300px;
}
.rotateZ{animation:run 15s linear infinite}
/*定义一个关键帧*/
@keyframes run{
from{transform:rotate(0deg)}
to{transform:rotate(360deg)}
}
.music input{
background:none;
border:0;
outline:none;
width:22px;
height:22px;
position:relative;
top:-31px;
left:4px;
}