1、思维导图
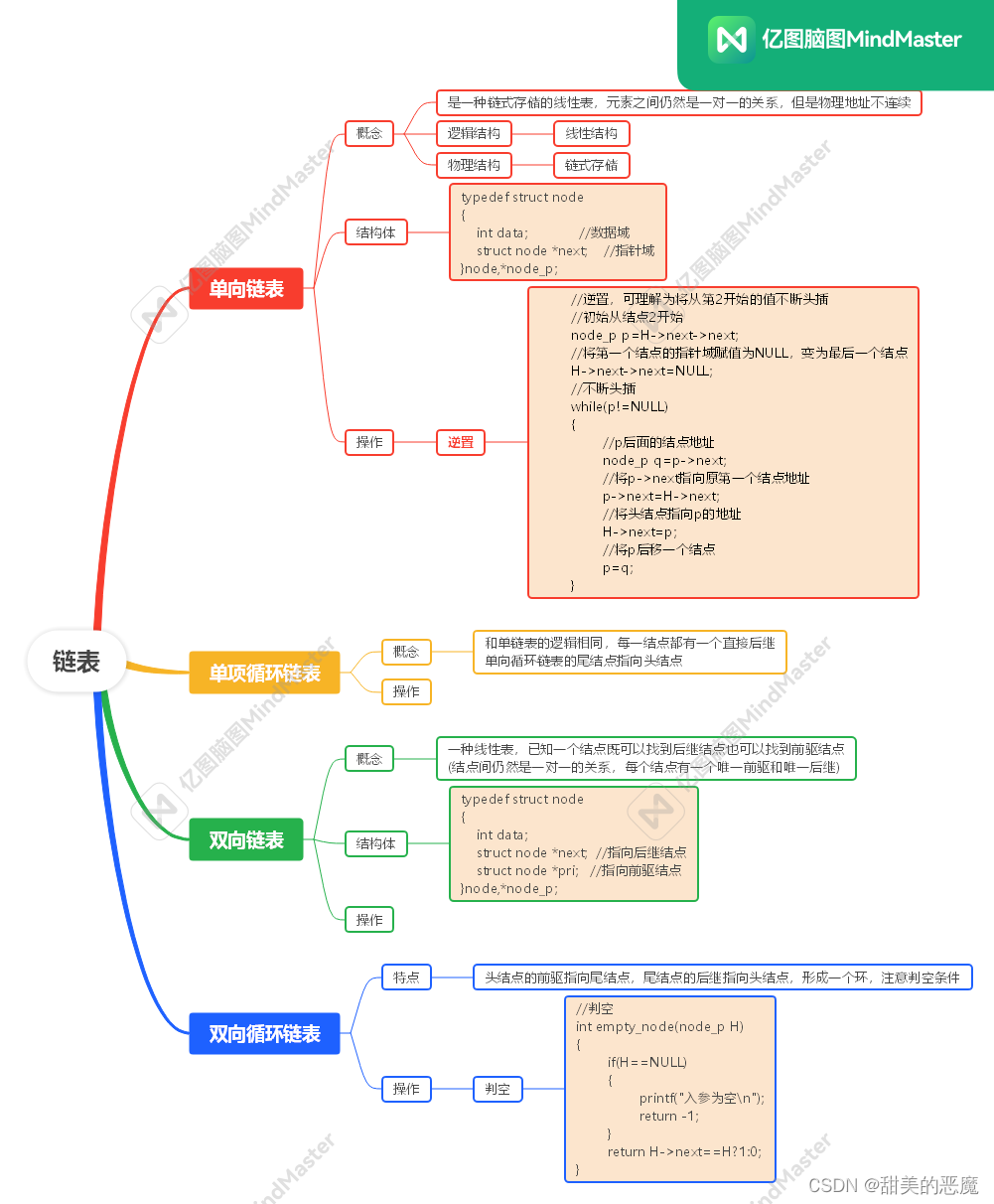
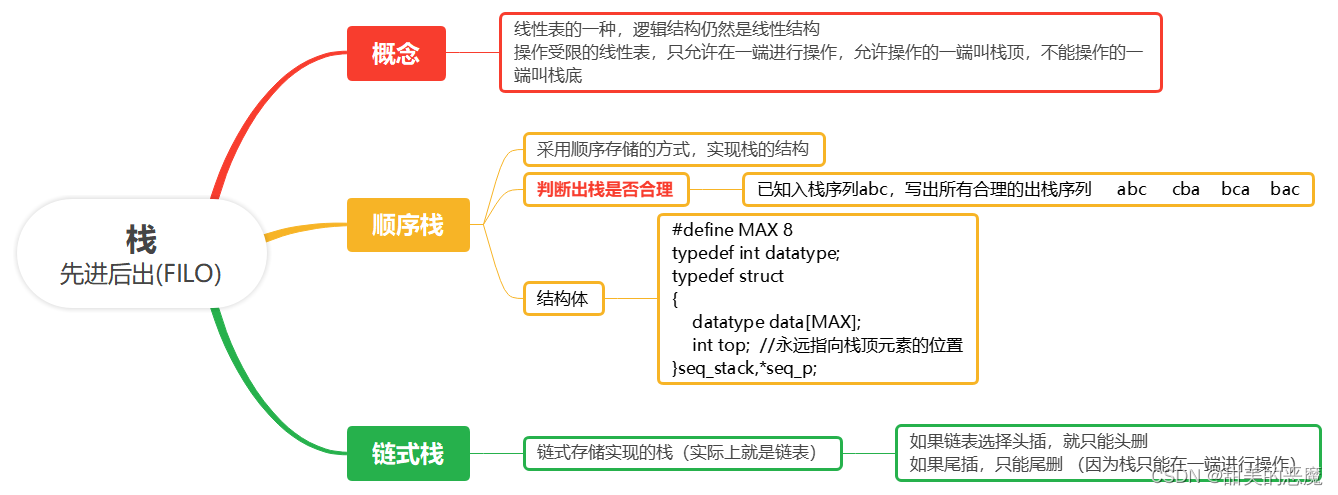
2、顺序栈
seq_stack.h头文件代码:
#ifndef __SEQ_STACK_H__
#define __SEQ_STACK_H__
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define MAX 9
typedef int datatype;
typedef struct
{
datatype data[MAX];
int top;
}seq_stack,*seq_p;
//创建顺序栈表
//申请空间判空
//头栈data和top初始化
seq_p create_stack();
//判空
int empty_stack(seq_p S);
//判满
int full_stack(seq_p S);
//入栈/压栈
void push_stack(seq_p S,datatype data);
//出栈/弹栈
void pop_stack(seq_p S);
//输出,输出顺序和栈操作的顺序一致
void show_stack(seq_p S);
//清空栈表
void clear_stack(seq_p S);
//释放栈表
void free_stack(seq_p *S);
#endif
seq_stack.c自定义函数代码:
//创建顺序栈表
//申请空间判空
//申请成功后,data和top初始化
seq_p create_stack()
{
seq_p S=(seq_p)malloc(sizeof(seq_stack));
if(S==NULL)
{
printf("空间申请失败\n");
return NULL;
}
S->top=-1;//无元素时,top=-1
return S;
}
//判空
int empty_stack(seq_p S)
{
//入参合理性检查
if(S==NULL)
{
printf("入参不合理\n");
return -1;
}
return S->top==-1?1:0;
}
//判满
int full_stack(seq_p S)
{
//入参合理性检查
if(S==NULL)
{
printf("入参不合理\n");
return -1;
}
return S->top==(MAX-1)?1:0;
}
//入栈/压栈
void push_stack(seq_p S,datatype data)
{
//入参合理性检查
if(S==NULL)
{
printf("入参不合理\n");
return;
}
//判满
if(full_stack(S))
{
printf("空间已满\n");
return;
}
//先将top自增
S->top++;
//在将数据入栈
S->data[S->top]=data;
}
//出栈/弹栈
void pop_stack(seq_p S)
{
//入参合理性检查
if(S==NULL)
{
printf("入参不合理\n");
return;
}
//判空
if(empty_stack(S))
{
printf("栈表为空\n");
return;
}
printf("出栈值:%d\n",S->data[S->top]);
S->top--;
return;
}
//输出,输出顺序和栈操作的顺序一致
void show_stack(seq_p S)
{
//入参合理性检查
if(S==NULL)
{
printf("入参不合理\n");
return;
}
//判空
if(empty_stack(S))
{
printf("栈表为空\n");
return;
}
for(int i=S->top;i>=0;i--)
printf("%d ",S->data[i]);
putchar(10);
}
//清空栈表
void clear_stack(seq_p S)
{
//入参合理性检查
if(S==NULL)
{
printf("入参不合理\n");
return;
}
//判空
if(empty_stack(S))
{
printf("栈表为空\n");
return;
}
S->top=-1;
}
//释放栈表
//释放栈表指针对应的地址
void free_stack(seq_p *S)
{
//入参合理性检查
//*S指向栈表地址
//S为二级指针,指向*S指针地址&(*S)
//先检查指针对应的地址是否为NULL
//再检查指针指向的值是否为NULL
if(S==NULL || *S==NULL)
{
printf("入参不合理\n");
return;
}
free(*S);
*S=NULL;
}
main.c主函数代码:
#include "seq_stack.h"
int main()
{
seq_p S=create_stack();
printf("判空结果:%d\n",empty_stack(S));
push_stack(S,10);
push_stack(S,11);
push_stack(S,12);
show_stack(S);
pop_stack(S);
show_stack(S);
free_stack(&S);
printf("%p\n",S);
return 0;
}
运行结果:
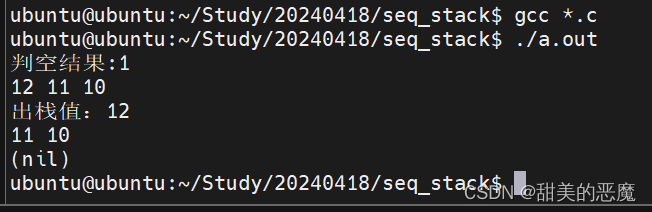
3、链栈
link_stack.h头文件代码:
#include <stdlib.h>
#include <string.h>
//定义结构体
typedef struct node
{
int data;
struct node *next;
}node,*node_p;
//判空
int empty_stack(node_p T);
//创栈结点,申请空间
node_p create_node(int data);
//入栈
void push_stack(node_p *T,int data);
//出栈
void pop_stack(node_p *T);
//输出栈
void show_stack(node_p T);
#endif
link_stack.c自定义函数代码:
#include "link_stack.h"
//创建栈结点,申请空间
node_p create_node(int data)
{
node_p new=(node_p)malloc(sizeof(node));
if(new==NULL)
{
printf("空间申请失败\n");
return NULL;
}
new->data=data;
return new;
}
//判空
int empty_stack(node_p T)
{
//不再需要,入参的判空
//因为T就在栈区
return T==NULL?1:0;
}
//入栈
void push_stack(node_p *T,int data)
{
node_p new=create_node(data);
new->next=*T;//让新结点指向原来的栈顶元素
*T=new; //让栈底指针指向新结点
}
//出栈
void pop_stack(node_p *T)
{
//判空
if(empty_stack(*T))
{
printf("栈链表为空\n");
return;
}
//保存要出栈的结点
node_p pop=*T;
printf("出栈的值为%d\n",pop->data);
*T=(*T)->next;
free(pop);
return;
}
//输出栈
void show_stack(node_p T)
{
//判空
if(empty_stack(T))
{
printf("栈链表为空\n");
return;
}
node_p p=T;
while(p!=NULL)
{
printf("%d->",p->data);
p=p->next;
}
printf("NULL\n");
}
main.c主函数代码:
#include "link_stack.h"
int main()
{
//申请栈顶指针
node_p T=NULL; //此时栈中没有数据
push_stack(&T,11);
push_stack(&T,12);
push_stack(&T,13);
push_stack(&T,14);
push_stack(&T,15);
show_stack(T);
pop_stack(&T);
show_stack(T);
return 0;
}
运行结果:
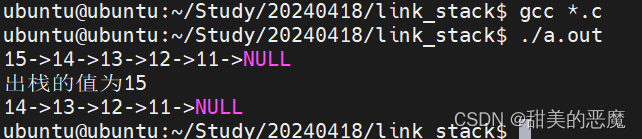