我的原则:先会用再说,内部慢慢来。
学以致用,根据场景学源码
一、架构
1.1 UML 图
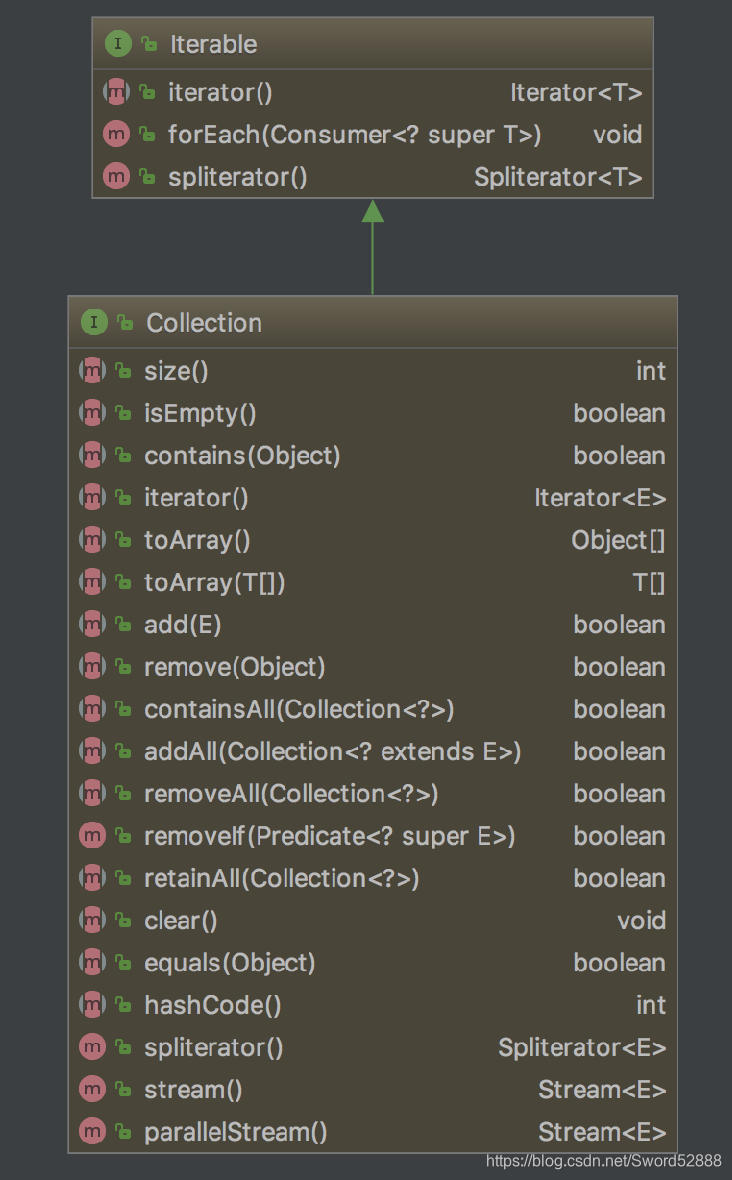
=== 点击查看top目录 ===
1.2 子类UML图
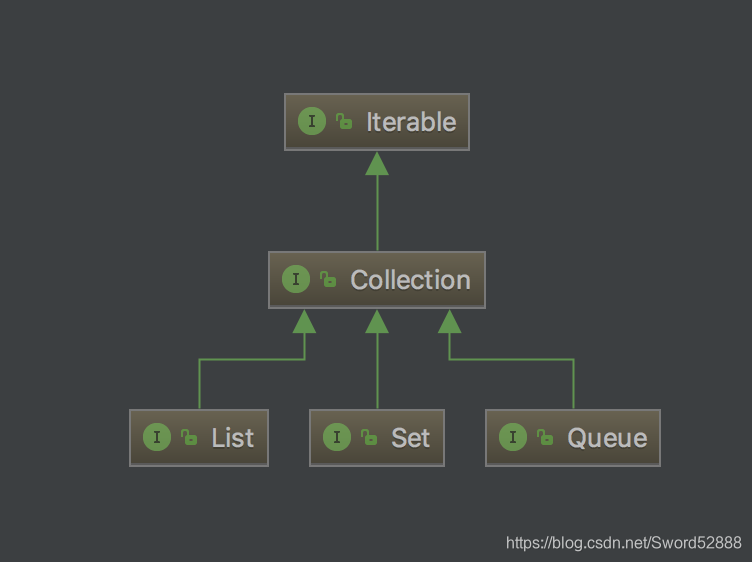
1.3 常用子类UML图
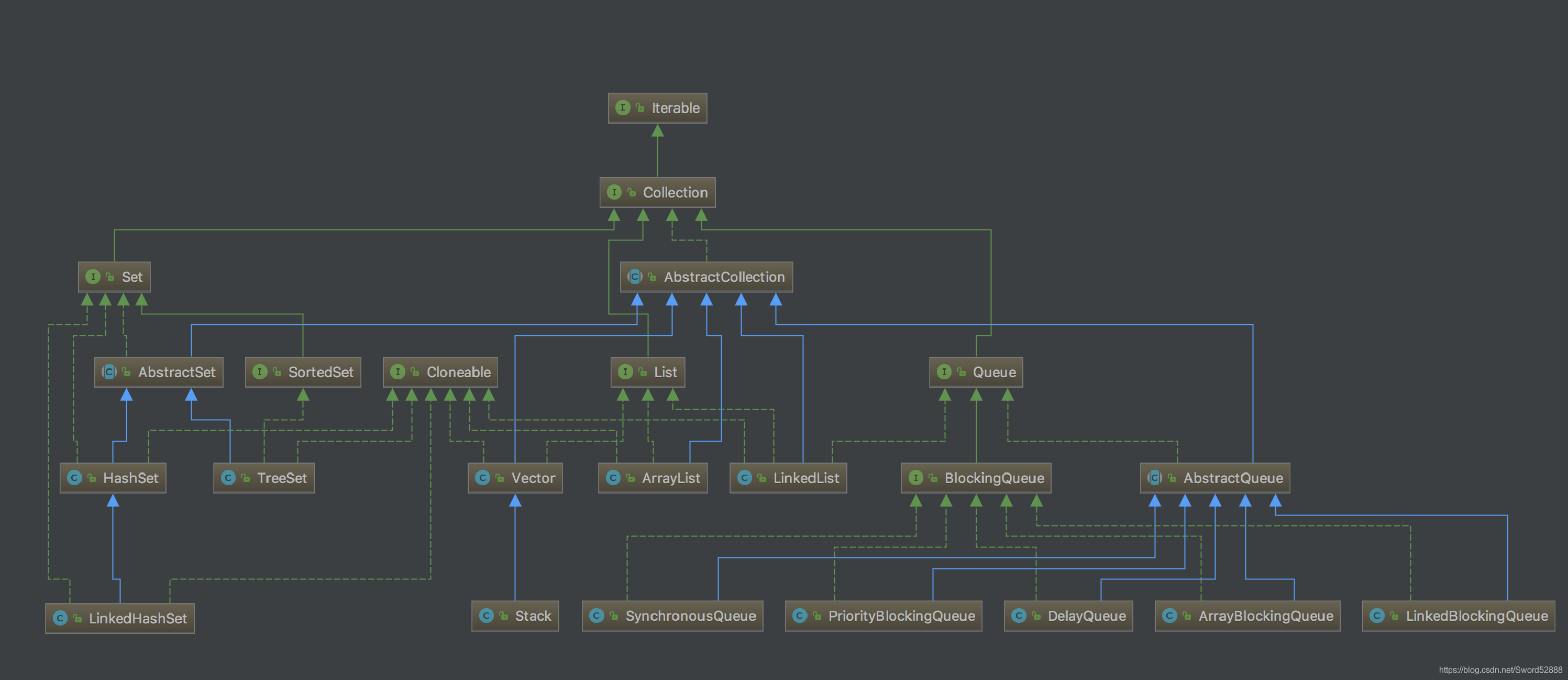
- 这里要注意,Map跟Collection没有直接关系,更不是 Collection 的子类。
=== 点击查看top目录 ===
二、Iterable 接口
2.1 源码
public interface Iterable<T> {
Iterator<T> iterator();
default void forEach(Consumer<? super T> action) {
Objects.requireNonNull(action);
for (T t : this) {
action.accept(t);
}
}
default Spliterator<T> spliterator() {
return Spliterators.spliteratorUnknownSize(iterator(), 0);
}
}
=== 点击查看top目录 ===
2.2 方法解析
方法 | 描述 |
---|
iterator | 返回迭代类 Iterator,可以对Collection 内的元素进行迭代处理 |
forEach | default 方法,传入消费类Consumer,对全体元素进行一波消费。jdk1.8之后,允许interface 定义default 方法与 static 方法 |
spliterator | 返回 IteratorSpliterator 类 |
=== 点击查看top目录 ===
三、 Iterator 接口
3.1 源码
public interface Iterator<E> {
boolean hasNext();
E next();
default void remove() {
throw new UnsupportedOperationException("remove");
}
default void forEachRemaining(Consumer<? super E> action) {
Objects.requireNonNull(action);
while (hasNext())
action.accept(next());
}
}
=== 点击查看top目录 ===
3.2 方法解析
方法 | 描述 |
---|
hasNext | 后面是否还有元素 |
remove | 移除元素 ,default 方法,需要被子类重写 |
forEachRemaining | 传入 Consumer,处理集合中剩下的所有元素,default 方法 |
=== 点击查看top目录 ===
四、Collection 接口
- Collection 接口,底下有一堆实现类,比如:List,Set ,Queue等等
4.1 源码剖析
public interface Collection<E> extends Iterable<E> {
int size();
boolean isEmpty();
boolean contains(Object o);
Iterator<E> iterator();
Object[] toArray();
<T> T[] toArray(T[] a);
boolean add(E e);
boolean remove(Object o);
boolean containsAll(Collection<?> c);
boolean addAll(Collection<? extends E> c);
boolean removeAll(Collection<?> c);
default boolean removeIf(Predicate<? super E> filter) {
Objects.requireNonNull(filter);
boolean removed = false;
final Iterator<E> each = iterator();
while (each.hasNext()) {
if (filter.test(each.next())) {
each.remove();
removed = true;
}
}
return removed;
}
boolean retainAll(Collection<?> c);
void clear();
boolean equals(Object o);
int hashCode();
@Override
default Spliterator<E> spliterator() {
return Spliterators.spliterator(this, 0);
}
default Stream<E> stream() {
return StreamSupport.stream(spliterator(), false);
}
default Stream<E> parallelStream() {
return StreamSupport.stream(spliterator(), true);
}
}
=== 点击查看top目录 ===
4.2 方法列表
方法 | 描述 |
---|
int size | 查询 Collection 内 element 数量 |
boolean isEmpty | 检查 Collection 内是否是空的 |
boolean contains(Object o); | 检查 Collection 内是否包含某个元素 |
Iterator iterator(); | 返回Iterator 接口 |
Object[] toArray(); | Collection 转 数组Array |
T[] toArray(T[] a); | Collection 转 数组Array |
boolean add(E e); | Collection 插入元素 |
boolean remove(Object o); | Collection 移除元素 |
boolean containsAll(Collection<?> c); | Collection 是否包含 Collection c内的全部元素在 |
boolean addAll(Collection<? extends E> c); | 一个Collection 塞入另一个 Collection |
boolean removeAll(Collection<?> c); | Collection 把 Collection c内的全部元素移除 |
default boolean removeIf(Predicate<? super E> filter) | 传入判断条件Predicate,按条件删除 |
boolean retainAll(Collection<?> c); | 取两个Collection 的交集,原Collection - Collection c |
void clear(); | 清掉 Collection ,往往是为了加快 GC |
boolean equals(Object o); | 判断两个Collection是否equals,需要重写该方法 |
int hashCode(); | hashCode |
default Spliterator spliterator() | default 方法,返回 Spliterator |
default Stream stream() | default 方法,拿到Stream 流 |
default Stream parallelStream() | default 方法,拿到 parallelStream 流 |
=== 点击查看top目录 ===
4.3 常用 Collection 子类
接口 | 子类 |
---|
Set | HashSet 、TreeSet |
List | Vector 、ArrayList 、LinkedList |
Queue | SynchronousQueue 、PriorityBlockingQueue 、 DelayQueue 、ArrayBlockingQueue、LinkedBlockingQueue |
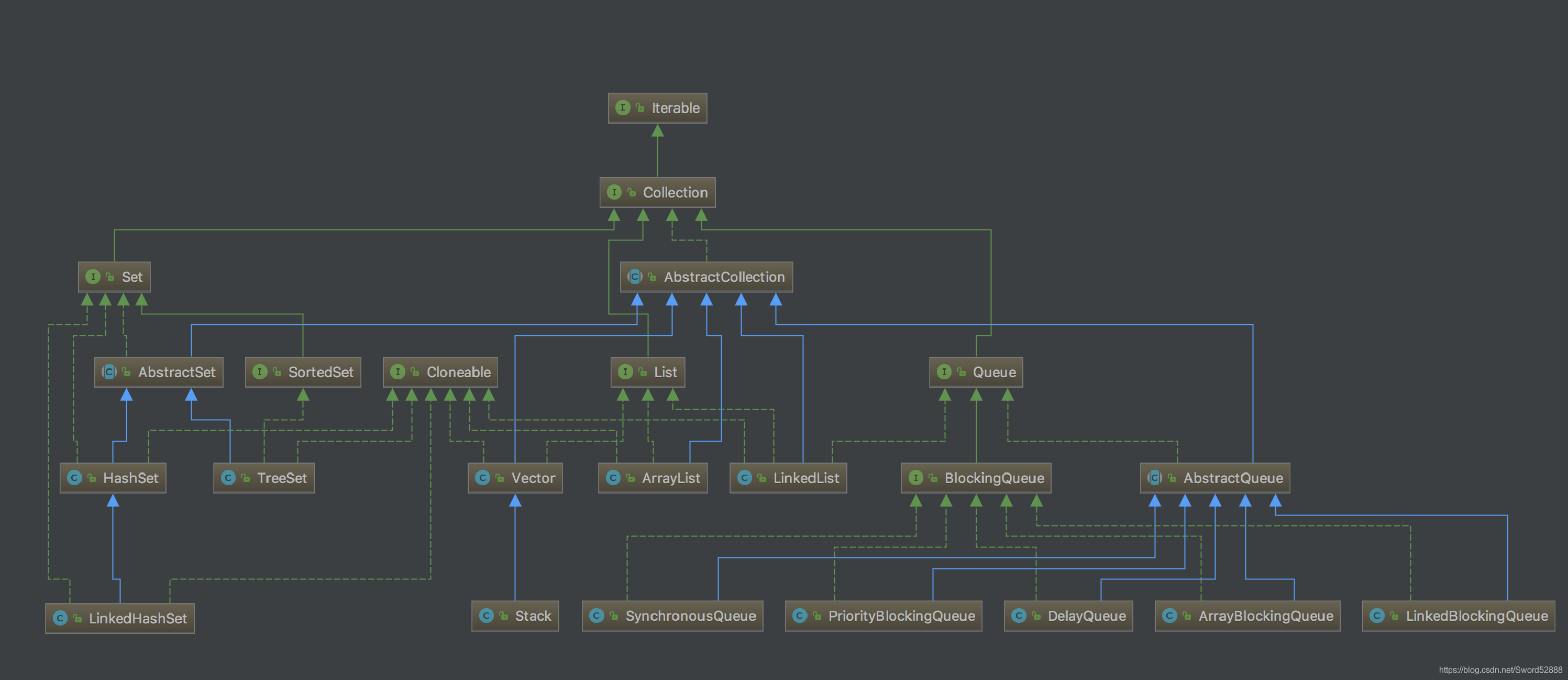
=== 点击查看top目录 ===
五、AbstractCollection 抽象类
- 相比 Collection 接口,实现了 N 若干个方法
相比 Collection 接口实现的方法 |
---|
isEmpty() |
contains(Object o) |
toArray() |
toArray(T[] a) |
remove(Object o) |
containsAll(Collection<?> c) |
addAll(Collection<? extends E> c) |
removeAll(Collection<?> c) |
retainAll(Collection<?> c) |
clear() |
toString() |
六、List 接口
public interface List<E> extends Collection<E> {}
6.1 特点
- 集合内元素有序(排列顺序自己定)
- 元素可以重复
- 可以有N个null 元素
6.2 List 方法列表
6.2.1 重写 Collection 方法
方法 | 描述 |
---|
int size(); | 同 Collection |
boolean isEmpty(); | 同 Collection |
boolean contains(Object o); | 同 Collection |
Iterator iterator(); | 同 Collection |
Object[] toArray(); | 同 Collection |
T[] toArray(T[] a); | 同 Collection |
boolean add(E e); | 同 Collection |
boolean remove(Object o); | 同 Collection |
boolean containsAll(Collection<?> c); | 同 Collection |
boolean addAll(Collection<? extends E> c); | 同 Collection |
boolean removeAll(Collection<?> c); | 同 Collection |
boolean retainAll(Collection<?> c); | 同 Collection |
void clear(); | 同 Collection |
boolean equals(Object o); | 同 Collection |
int hashCode(); | 同 Collection |
default Spliterator spliterator() | 同 Collection // 重写,Spliterator.ORDERED |
6.2.2 相比 Collection 增加的方法 (12个)
方法 | 描述 |
---|
boolean addAll(int index, Collection<? extends E> c); | 比老的allAll多了一个下标index |
E get(int index); | 获取某个 index 下 Element |
E set(int index, E element); | 设置某个 index 下的 Element |
void add(int index, E element); | 在某个 index 后插入Element |
E remove(int index); | 移除某个 index 的Element |
int indexOf(Object o); | 从0 到 N,查看某个Element的下标 |
int lastIndexOf(Object o); | 从 N 到0 ,查看某个Element的下标 |
ListIterator listIterator(); | 返回一个双向 ListIterator |
ListIterator listIterator(int index); | 从某个 index 往后截取全部元素,组成双向 ListIterator |
List subList(int fromIndex, int toIndex); | 切割List,组成双向 ListIterator |
default void replaceAll(UnaryOperator operator) | 输出操作函数,对list内全部元素进行处理 |
default void sort(Comparator<? super E> c) | 输入 Comparator 排列器,对list内全部元素进行排队 |
6.2.3 相比 Collection 少了的方法 (3个)
方法 | 描述 |
---|
default boolean removeIf(Predicate<? super E> filter) | 传入判断条件Predicate,按条件删除 |
default Stream stream() | default 方法,拿到Stream 流 |
default Stream parallelStream() | default 方法,拿到 parallelStream 流 |
=== 点击查看top目录 ===
七、Queue 接口
public interface Queue<E> extends Collection<E> {}
7.1 特点
- 先进先出
- 元素可以重复
7.2 Queue 方法列表
7.2.1 重写 Collection 方法(无)
无
7.2.2 相比 Collection 增加的方法 (6个)
方法 | 描述 |
---|
boolean add(E e); | 添加元素,失败抛 IllegalArgumentException |
boolean offer(E e); | 添加元素,失败返回 false |
E remove(); | 移除元素,队列内没元素抛 NoSuchElementException |
E poll(); | 移除元素,队列内没元素返回 null |
E element(); | 取出队头元素,队列内没元素抛 NoSuchElementException |
E peek(); | 取出队头元素,队列内没元素返回 false |
7.2.3 相比 Collection 少了全部方法
=== 点击查看top目录 ===
八、Set 接口
public interface Set<E> extends Collection<E> {}
8.1 特点
- 集合内元素无序
- 元素不可以重复
- 可以有一个null 元素
8.2 Set 方法列表
8.2.1 重写 Collection 方法
方法 | 描述 |
---|
int size(); | 同 Collection |
boolean isEmpty(); | 同 Collection |
boolean contains(Object o); | 同 Collection |
Iterator iterator(); | 同 Collection |
Object[] toArray(); | 同 Collection |
T[] toArray(T[] a); | 同 Collection |
boolean add(E e); | 同 Collection |
boolean remove(Object o); | 同 Collection |
boolean containsAll(Collection<?> c); | 同 Collection |
boolean addAll(Collection<? extends E> c); | 同 Collection |
boolean retainAll(Collection<?> c); | 同 Collection |
boolean removeAll(Collection<?> c); | 同 Collection |
void clear(); | 同 Collection |
boolean equals(Object o); | 同 Collection |
int hashCode(); | 同 Collection |
8.2.2 新增 Collection 方法 (无)
无
8.2.3 相比 Collection 少了的方法(4个)
方法 | 描述 |
---|
default boolean removeIf(Predicate<? super E> filter) | 传入判断条件Predicate,按条件删除 |
default Spliterator spliterator() | default 方法,返回 Spliterator |
default Stream stream() | default 方法,拿到Stream 流 |
default Stream parallelStream() | default 方法,拿到 parallelStream 流 |
=== 点击查看top目录 ===
九、番外篇
下一章节:【Java Collection】List 剖析(二)