生命周期汇总图
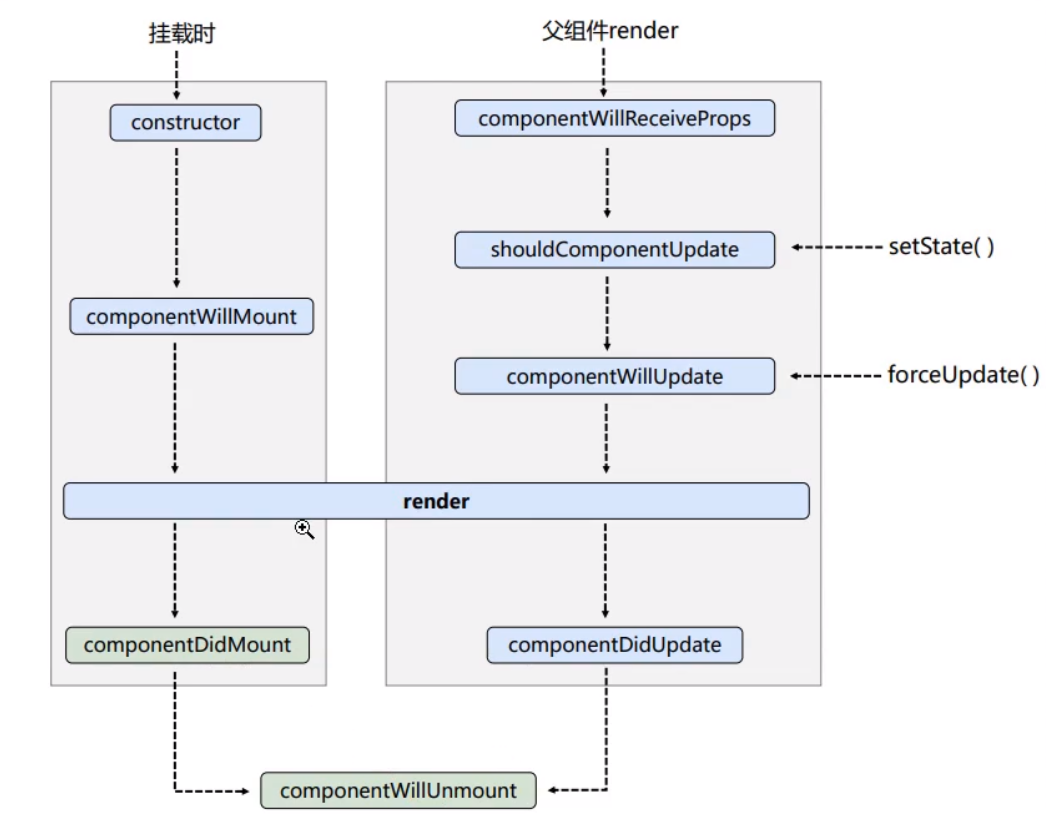
线路一
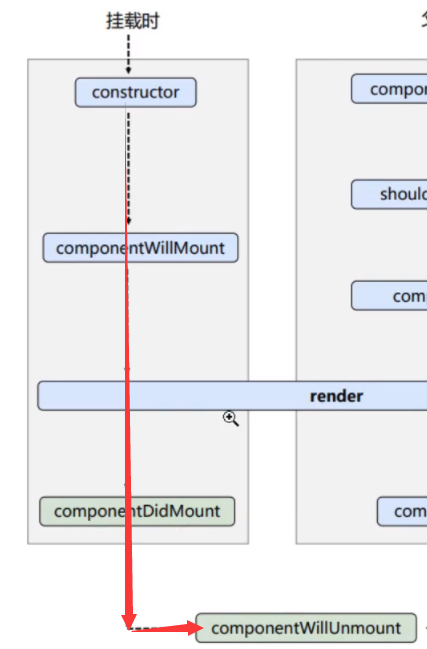
class Demo extends React.Component {
constructor () {
super()
this.state = {
count: 0
}
console.log('constructor')
}
handleAdd = () => {
this.setState({
count: this.state.count + 1
})
}
handleUnmounted = () => {
ReactDOM.unmountComponentAtNode(document.getElementById('app'))
}
componentWillMount () {
console.log('componentWillMount')
}
render () {
console.log('render')
return (
<div>
<div>{this.state.count}</div>
<button onClick={this.handleAdd}>+1</button>
<button onClick={this.handleUnmounted}>卸载</button>
</div>
)
}
componentDidMount () {
console.log('componentDidMount')
}
componentWillUnmount () {
console.log('componentWillUnmount')
}
}
ReactDOM.render(<Demo />, document.getElementById('app'))
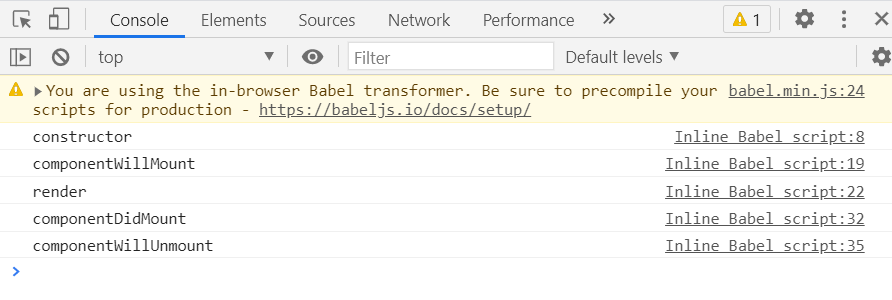
线路二
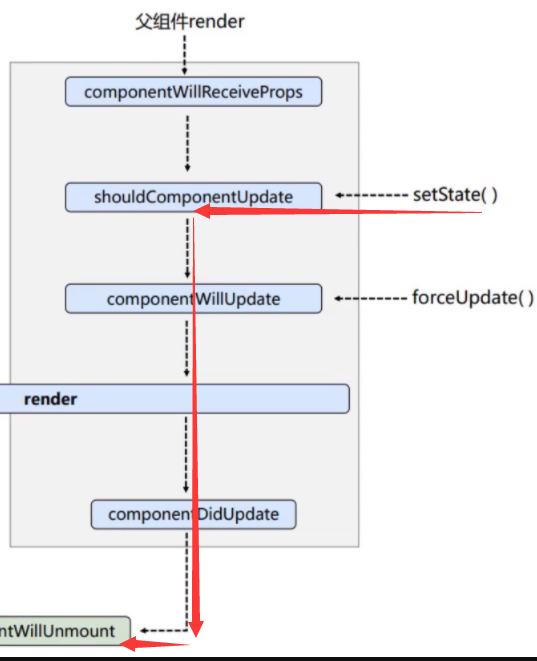
class Demo extends React.Component {
constructor () {
...
}
handleAdd = () => {
...
}
handleUnmounted = () => {
...
}
shouldComponentUpdate() {
console.log('shouldComponentUpdate')
return true
}
componentWillMount () {
console.log('componentWillMount')
}
componentWillUpdate () {
console.log('componentWillUpdate')
}
render () {
...
}
componentDidUpdate () {
console.log('componentDidUpdate')
}
componentDidMount () {
console.log('componentDidMount')
}
componentWillUnmount () {
console.log('componentWillUnmount')
}
}
ReactDOM.render(<Demo />, document.getElementById('app'))
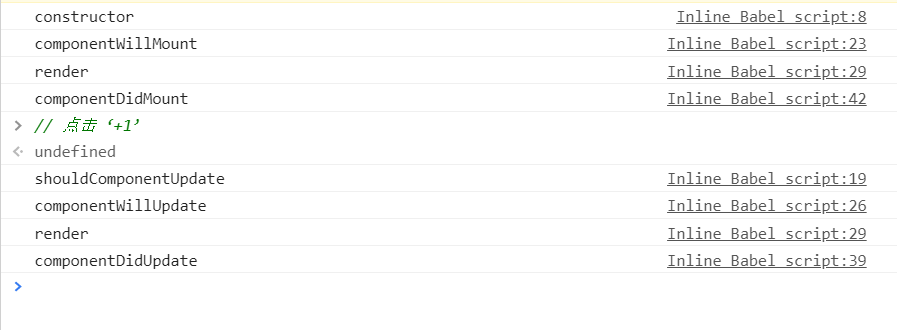
线路三
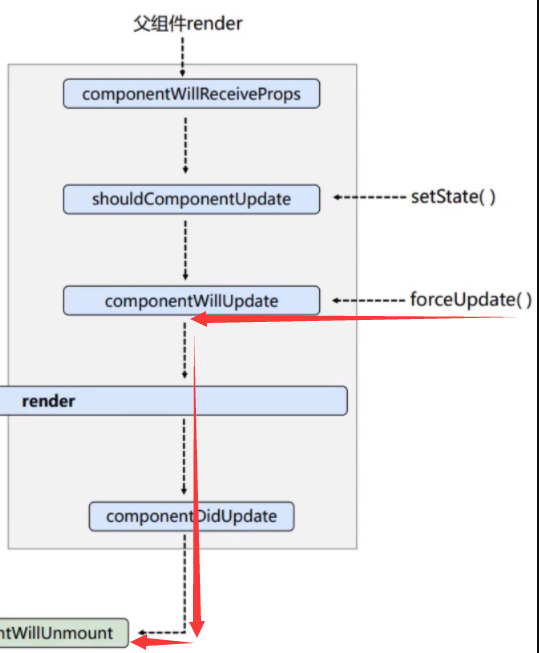
class Demo extends React.Component {
constructor () {
...
}
handleAdd = () => {
...
}
handleUnmounted = () => {
...
}
shouldComponentUpdate() {
...
}
componentWillMount () {
console.log('componentWillMount')
}
componentWillUpdate () {
console.log('componentWillUpdate')
}
render () {
console.log('render')
return (
<div>
...
<button onClick={()=>this.forceUpdate()}>强制更新</button>
</div>
)
}
componentDidUpdate () {
console.log('componentDidUpdate')
}
componentDidMount () {
console.log('componentDidMount')
}
componentWillUnmount () {
console.log('componentWillUnmount')
}
}
ReactDOM.render(<Demo />, document.getElementById('app'))
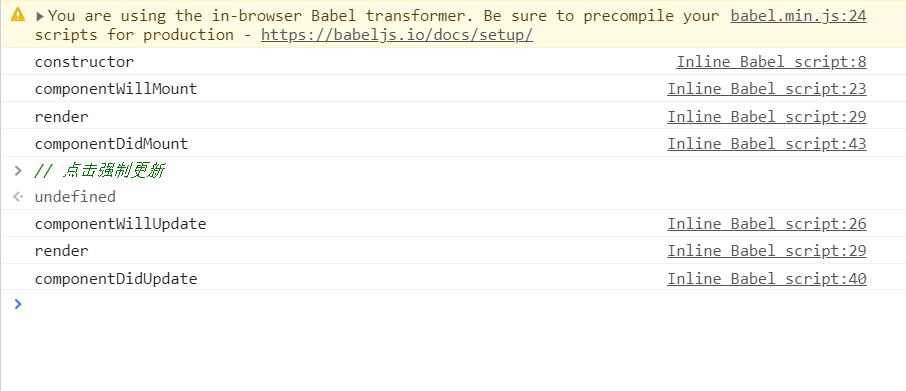
线路四
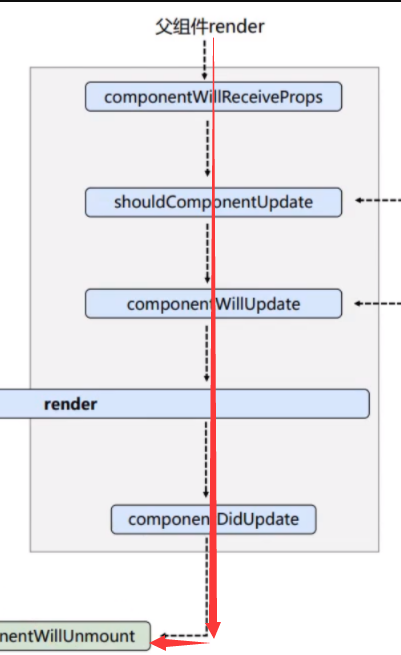
class Parent extends React.Component{
state = {
count: 0
}
handleAdd = () => {
this.setState({
count: this.state.count + 1
})
}
render () {
return (
<div>
<Child count={this.state.count}/>
<button onClick={this.handleAdd}>+1</button>
</div>
)
}
}
class Child extends React.Component {
componentWillReceiveProps () {
console.log('componentWillReceiveProps')
}
shouldComponentUpdate() {
console.log('shouldComponentUpdate')
return true
}
componentWillUpdate () {
console.log('componentWillUpdate')
}
render () {
return (
<div>
{this.props.count}
</div>
)
}
componentDidUpdate () {
console.log('componentDidUpdate')
}
}
ReactDOM.render(<Parent />, document.getElementById('app'))
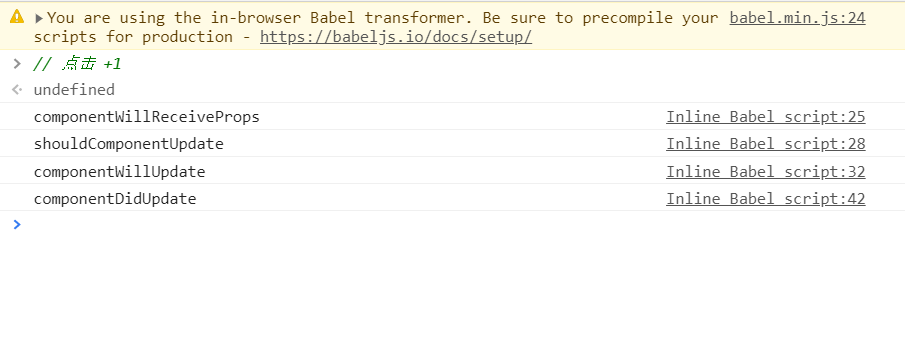
17版本的生命周期变动
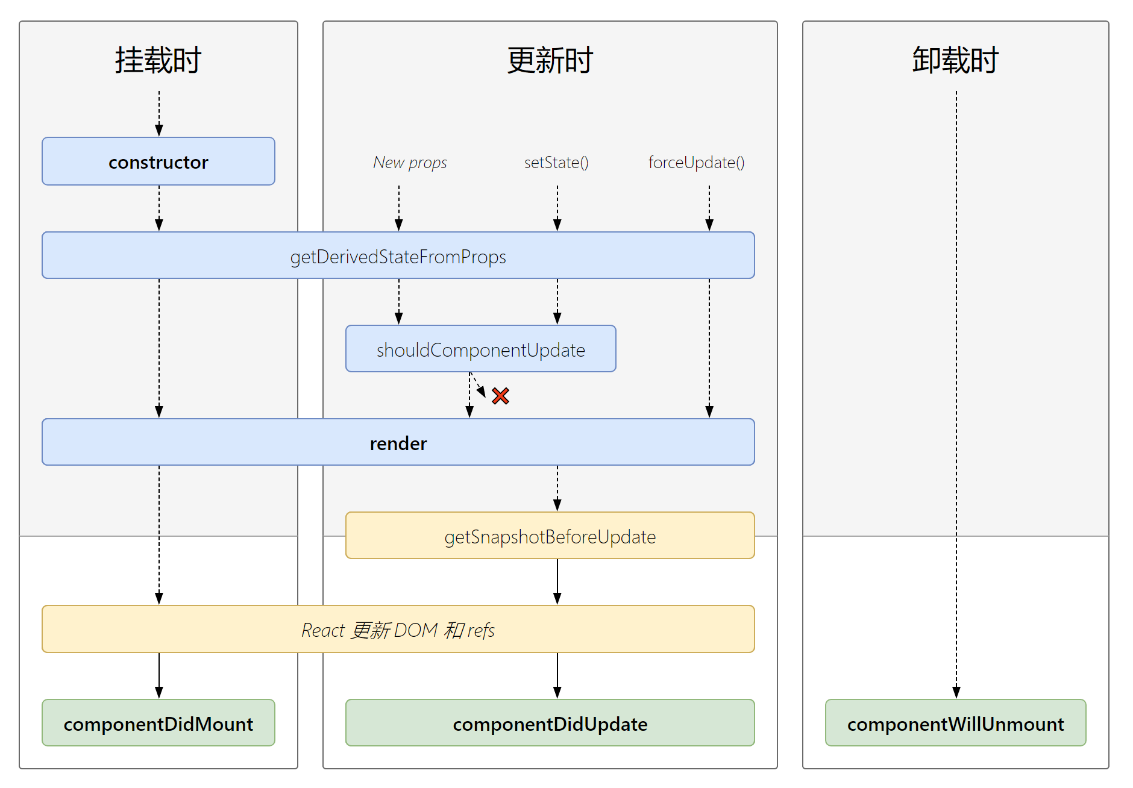
- React 预计废弃这三个钩子:
componentWillReceiveProps、componentWillMount、componentWillUpdate
- 在17版本中,这三个待废弃的钩子被重命名为:
UNSAFE_componentWillReceiveProps、UNSAFE_componentWillMount、UNSAFE_componentWillUpdate
- 在17版本中,新增了两个钩子:
getDerivedStateFromProps、getSnapshotBeforeUpdate
getDerivedStateFromProps
- 该方法意为
从props中获取派生的state
- 该方法为
静态方法
- 该方法接收两个参数,参数1为
props
、参数2为state
- 该方法返回
null
,或者派生的state
,页面渲染时,该派生的state
优先级高于 this.state
- 该方法横跨
挂载
和更新
,可以在任何时候决定用于渲染视图的state
- 该方法用法罕见,仅当需要由
props
来决定state
时,可能有用
class Demo extends React.Component {
constructor () {
super()
this.state = {
count: 0
}
console.log('constructor')
}
handleAdd = () => {
...
}
handleUnmounted = () => {
ReactDOM.unmountComponentAtNode(document.getElementById('app'))
}
static getDerivedStateFromProps (props, state) {
console.log('getDerivedStateFromProps')
return null
}
render () {
console.log('render')
...
}
componentDidMount () {
console.log('componentDidMount')
}
}
ReactDOM.render(<Demo />, document.getElementById('app'))
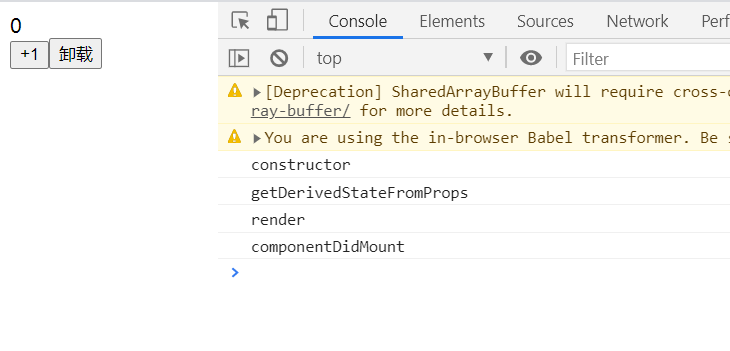
static getDerivedStateFromProps (props, state) {
console.log('getDerivedStateFromProps')
return {count: 100}
}
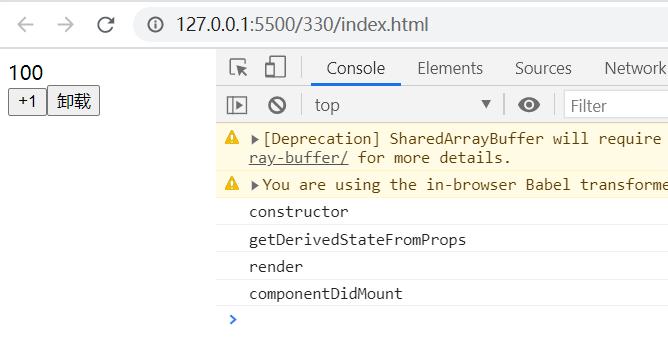
getSnapshotBeforeUpdate
- 该方法意为
在更新之前获取快照
- 该方法接收两个参数,参数1
prevProps
:之前的props,参数2prevState
:之前的state - 该方法的返回值,会传递给
componentDidMount
第三个参数 componentDidMount
的三个参数:(prevProps
, prevState
, snapshot
)- 通过该钩子,可以捕获一些更新前的数据,并传递给
componentDidMount
- 同样是用法罕见的钩子