需求说明
-
呈现效果图
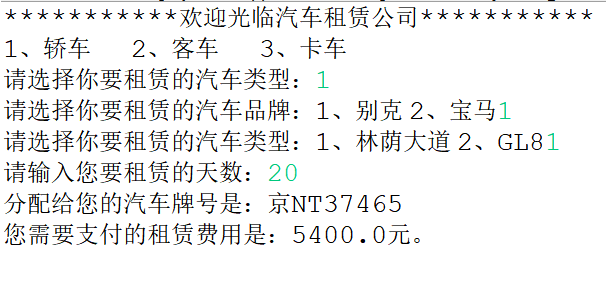
-
车型信息
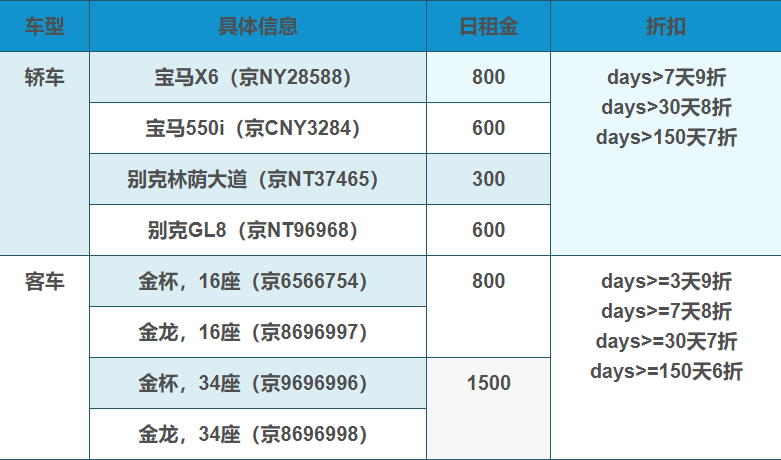
-
方法
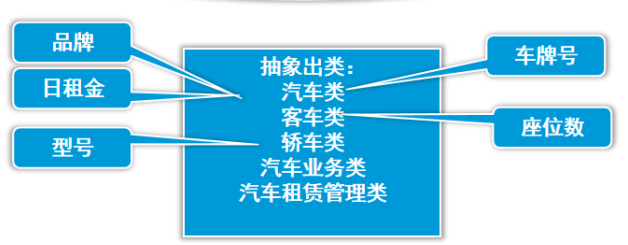
-
类的属性和方法
-
汽车类:车牌号、品牌、日租金
-
客车类:车牌号、品牌、日租金、座位数
-
轿车类:车牌号、品牌、日租金、型号
-
汽车业务类:忽略
-
汽车租赁管理类:忽略
代码实现
-
客车类
package cars.array;
/**客车类*/
public class Bus extends MotoVehicle{
private int seatCount;//座位数
public int getSeatCount() {
return seatCount;
}
public void setSeatCount(int seatCount) {
this.seatCount = seatCount;
}
public Bus(){
}
public Bus(String vehicleId, String brand,int seatCount,int perRent){
super(vehicleId, brand,perRent);
this.seatCount=seatCount;
}
/**
* 重写计算租金
*/
public float calRent(int days) {
float price=this.getPerRent()*days;
if(days>=3 && days<7){
price *= 0.9f;
}else if(days>=7 && days<30){
price *= 0.8f;
}else if(days>=30 && days<150){
price *= 0.7f;
}else if(days>=150){
price*= 0.6f;
}
return price;
}
}
-
轿车类
package cars.array;
/**轿车类*/
public class Car extends MotoVehicle{
private String type;//型号
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public Car(){
}
public Car(String vehicleId, String brand, String type,int perRent){
super(vehicleId, brand,perRent);
this.type=type;
}
/**
* 重写计算租金
*/
public float calRent(int days) {
float price=this.getPerRent()*days;
if(days>7 && days<=30){
price *= 0.9f;
}else if(days>30 && days<=150){
price *= 0.8f;
}else if(days>150){
price *= 0.7f;
}
return price;
}
}
-
卡车类
package cars.array;
public class Truck extends MotoVehicle {
private int tonnage;
public int getTonnage() {
return tonnage;
}
public void setTonnage(int tonnage) {
this.tonnage = tonnage;
}
public Truck(int tonnage) {
super();
this.tonnage = tonnage;
}
@Override
public float calRent(int days) {
// TODO Auto-generated method stub
return 0;
}
public Truck() {
super();
// TODO Auto-generated constructor stub
}
public Truck(String vehicleId, String brand, int perRent,int tonnage) {
super(vehicleId, brand, perRent);
// TODO Auto-generated constructor stub
}
}
-
汽车类
package cars.array;
/**汽车类*/
public abstract class MotoVehicle {
private String vehicleId;//车牌号
private String brand;//品牌
private int perRent;//日租金
public abstract float calRent(int days);//计算租金
public MotoVehicle(){
}
public MotoVehicle(String vehicleId, String brand,int perRent) {
this.vehicleId = vehicleId;
this.brand = brand;
this.perRent=perRent;
}
public String getVehicleId() {
return vehicleId;
}
public void setVehicleId(String vehicleId) {
this.vehicleId = vehicleId;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public int getPerRent() {
return perRent;
}
public void setPerRent(int perRent) {
this.perRent = perRent;
}
}
-
汽车业务类
package cars.array;
/**汽车业务类*/
public class MotoOperation {
public MotoVehicle motos[] = new MotoVehicle[12];
public void init(){
motos[0] = new Car("京NY28588", "宝马", "X6",800);
motos[1] = new Car("京CNY3284", "宝马", "550i",600);
motos[2] = new Car("京NT37465", "别克", "林荫大道",300);
motos[3] = new Car("京NT96968", "别克", "GL8",600);
motos[4] = new Bus("京6566754", "金杯", 16,800);
motos[5] = new Bus("京8696997", "金龙", 16,800);
motos[6] = new Bus("京9696996", "金杯", 34,1500);
motos[7] = new Bus("京8696998", "金龙", 34,1500);
motos[8] = new Truck("京MH98725", "一汽解放", 50,2);
motos[9] = new Truck("京L593216", "重庆红岩", 50,1);
motos[10] = new Truck("京NU98631", "一汽解放", 50,1);
motos[11] = new Truck("京CY56312", "重庆红岩", 50,2);
}
/**
* 租赁汽车
* @return 汽车
*/
public MotoVehicle motoLeaseOut(String brand,String type,int seat,int tonnage){
MotoVehicle moto=null;
for (MotoVehicle mymoto : motos) {
if(mymoto instanceof Car){
Car car=(Car)mymoto;
if(car.getBrand().equals(brand)&&car.getType().equals(type)){
moto=car;
break;
}
}else if(mymoto instanceof Bus){
Bus bus=(Bus)mymoto;
if(bus.getBrand().equals(brand)&&bus.getSeatCount()==seat){
moto=bus;
break;
}
}else{
Truck truck=(Truck)mymoto;
if(truck.getBrand().equals(brand)&&truck.getTonnage()==tonnage){
moto=truck;
break;
}
}
}
return moto;//返回一个汽车对象
}
}
-
测试类
package cars.array;
import java.util.Scanner;
/**租车测试类*/
public class RentMgrSys {
public static void main(String[] args) {
Scanner input=new Scanner(System.in);
MotoOperation motoMgr=new MotoOperation();
motoMgr.init();
System.out.println("***********欢迎光临汽车租赁公司***********");
System.out.println("1、轿车 \t2、客车\t3、卡车");
System.out.print("请选择你要租赁的汽车类型:");
int motoType=input.nextInt();
String brand="";
String type="";
int seat=0;
int tonnage=0;
if(motoType==1){
System.out.print("请选择你要租赁的汽车品牌:1、别克 2、宝马");
int choose2=input.nextInt();
if(choose2==1){
brand="别克";
System.out.print("请选择你要租赁的汽车类型:1、林荫大道 2、GL8");
type=(input.nextInt()==1)?"林荫大道":"GL8";
}else if(choose2==2){
brand="宝马";
System.out.print("请选择你要租赁的汽车类型:1、X6 2、550i");
type=(input.nextInt()==1)?"X6":"550i";
}
}else if(motoType==2){
type="";
System.out.print("请选择你要租赁的汽车品牌:1、金龙 2、金杯");
brand=(input.nextInt()==1)?"金龙":"金杯";
System.out.print("请选择你要租赁的汽车座位数:1、16座 2、34座");
seat=(input.nextInt()==1)?16:34;
}else if(motoType==3){
type="";
System.out.print("请选择你要租赁的汽车品牌:1、一汽解放 2、重庆红岩");
brand=(input.nextInt()==1)?"一汽解放":"重庆红岩";
System.out.print("请选择你要租赁的汽车吨位:1、1吨 2、2吨");
tonnage=(input.nextInt()==1)?1:2;
}
MotoVehicle moto=null;//汽车
moto=motoMgr.motoLeaseOut(brand,type,seat,tonnage);//获得
System.out.print("请输入您要租赁的天数:");
int days=input.nextInt();
double money=moto.calRent(days);//租赁费用
System.out.println("分配给您的汽车牌号是:"+moto.getVehicleId());
System.out.println("您需要支付的租赁费用是:"+money+"元。");
}
}