需求说明
-
汽车信息
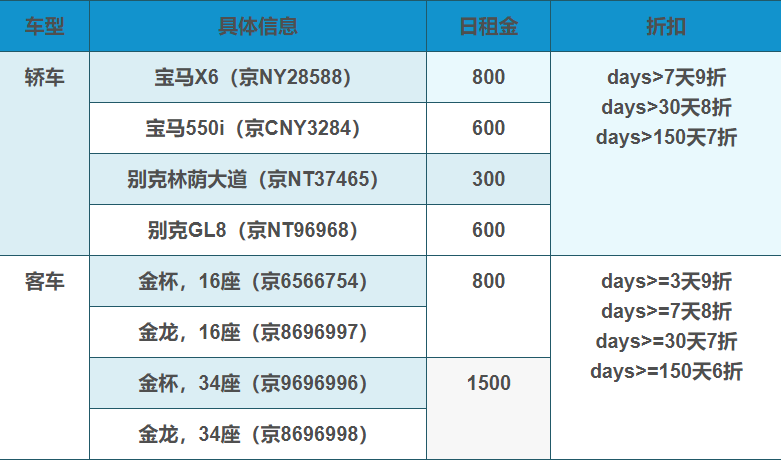
-
方法
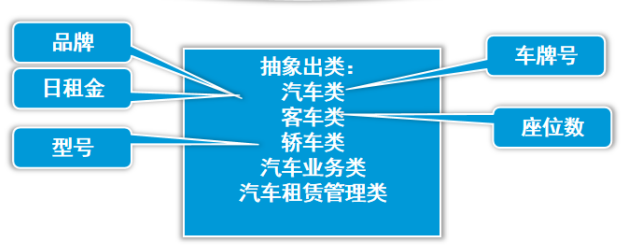
-
类的属性和方法
-
汽车类:车牌号、品牌、日租金
-
客车类:车牌号、品牌、日租金、座位数
-
轿车类:车牌号、品牌、日租金、型号
-
汽车业务类:忽略
-
汽车租赁管理类:忽略
-
呈现效果
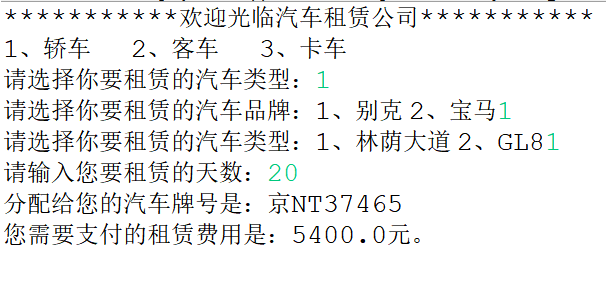
代码实现
-
客车类
package cars.xml;
/**客车类*/
public class Bus extends Automobile{
private int seat;
public Bus() {
super();
}
public Bus(String numberPlate, String brand, double dayRent, int seat) {
super(numberPlate, brand, dayRent);
this.seat = seat;
}
public int getSeat() {
return seat;
}
public void setSeat(int seat) {
this.seat = seat;
}
@Override
public double calRent(int days,String dayRent) {
// System.out.println("bus");
double discount=Integer.valueOf(dayRent)*days;
if(days>150){
discount*=0.6;
}else if(days>30){
discount*=0.7;
}else if(days>7){
discount*=0.8;
}else if(days>3){
discount*=0.9;
}
return discount;
}
}
-
轿车类
package cars.xml;
/** 轿车类*/
public class Car extends Automobile{
// 定义特有属性
private String type;
public Car() {
super();
}
public Car(String numberPlate, String brand, double dayRent, String type) {
super(numberPlate, brand, dayRent);
this.type = type;
}
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
@Override
public double calRent(int days,String dayRent) {
double discount=Integer.valueOf(dayRent)*days;
if(days>150){
discount*=0.7;
}else if(days>30){
discount*=0.8;
}else if(days>7){
discount*=0.9;
}
return discount;
}
}
-
汽车类
package cars.xml;
/**汽车类*/
public abstract class Automobile {
// 定义汽车类的属性(车牌号、品牌、日租金)
private String numberPlate;
private String brand;
private double dayRent;
public Automobile() {
super();
}
public Automobile(String numberPlate, String brand, double dayRent) {
super();
this.numberPlate = numberPlate;
this.brand = brand;
this.dayRent = dayRent;
}
public String getNumberPlate() {
return numberPlate;
}
public void setNumberPlate(String numberPlate) {
this.numberPlate = numberPlate;
}
public String getBrand() {
return brand;
}
public void setBrand(String brand) {
this.brand = brand;
}
public double getDayRent() {
return dayRent;
}
public void setDayRent(double dayRent) {
this.dayRent = dayRent;
}
//定义计算租金的抽象方法
public abstract double calRent(int days,String dayRent);
}
-
XML文件
<?xml version="1.0" encoding="UTF-8" ?>
<Automobiles>
<Automobile numberPlate="京NY28588" brand="宝马" dayRent="800" type="X6" seat="" />
<Automobile numberPlate="京CNY3284" brand="宝马" dayRent="600" type="550i" seat="" />
<Automobile numberPlate="京NT37465" brand="别克" dayRent="300" type="林荫大道" seat="" />
<Automobile numberPlate="京NT96968" brand="别克" dayRent="600" type="GL8" seat="" />
<Automobile numberPlate="京6566754" brand="金杯" dayRent="800" type="" seat="16" />
<Automobile numberPlate="京8696997" brand="金龙" dayRent="800" type="" seat="16" />
<Automobile numberPlate="京9696996" brand="金杯" dayRent="1500" type="" seat="34" />
<Automobile numberPlate="京8696998" brand="金龙" dayRent="1500" type="" seat="34" />
</Automobiles>
-
业务类
package cars.xml;
import java.io.File;
import java.io.IOException;
import java.util.ArrayList;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.parsers.ParserConfigurationException;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.NodeList;
import org.xml.sax.SAXException;
/**汽车业务类*/
public class AutomobileMethod {
Document document;
// 声明方法获得Document对象的
public void getDocument(File file) {
// 获取解析器工厂对象
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
// 从DOM工厂获得DOM解析器
try {
DocumentBuilder db = dbf.newDocumentBuilder();
// 解析XML文档,得到一个Document对象,即DOM树
document = db.parse(file);
} catch (ParserConfigurationException e) {
e.printStackTrace();
} catch (SAXException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
// 租车
public void autoMethod(String brand, String type, int seat,int days) {
Automobile auto = null;
String numStr="";
double money = 0;
NodeList nl = document.getElementsByTagName("Automobile");
for (int i = 0; i < nl.getLength(); i++) {
Element autoXml = (Element) nl.item(i);
if (autoXml.getAttribute("brand").equals(brand)
&& autoXml.getAttribute("seat").equals("")) {
auto=new Car();
money=auto.calRent(days,autoXml.getAttribute("dayRent"));
numStr=autoXml.getAttribute("numberPlate");
break;
}else if(autoXml.getAttribute("brand").equals(brand)
&& autoXml.getAttribute("type").equals("")) {
auto=new Bus();
money=auto.calRent(days,autoXml.getAttribute("dayRent"));
numStr=autoXml.getAttribute("numberPlate");
break;
}
}
System.out.println("租车成功!请按照"+numStr+"车牌号取车!租金为:"+money);
}
}
-
测试类
package cars.xml;
import java.io.File;
import java.util.Scanner;
/**租车测试类*/
public class AutomobileLease {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
String brand=null,type=null;
int seat=0;
AutomobileMethod autoM=new AutomobileMethod();
//获取所有车辆信息
File file=new File("Automobile.xml");
autoM.getDocument(file);
System.out.println("********欢迎光临租赁公司********\n");
System.out.println("请选择汽车类型:1、轿车\t2、客车");
int autoType=sc.nextInt();
if(autoType==1){
System.out.println("请选择轿车品牌:1、宝马\t2、别克");
brand=(sc.nextInt()==1)?"宝马":"别克";
if(brand=="宝马"){
System.out.println("请选择轿车型号:1、X6\t2、550i");
type=(sc.nextInt()==1)?"X6":"550i";
}else if(brand=="别克"){
System.out.println("请选择轿车型号:1、林荫大道\t2、GL8");
type=(sc.nextInt()==1)?"林荫大道":"GL8";
}
}else if(autoType==2){
System.out.println("请选择客车品牌:1、金杯\t2、金龙");
brand=(sc.nextInt()==1)?"金杯":"金龙";
System.out.println("请选择需要的座位数:1、16座\t2、34座");
seat=(sc.nextInt()==1)?16:34;
}
System.out.println("请选择租赁天数:");
int days=sc.nextInt();
autoM.autoMethod(brand, type, seat,days);
}
}