- 什么是AOP
AOP即面向切面编程,通过预编译方式和运行期动态代理实现程序功能的统一维护的一种技术。 - AOP可以干什么
利用 AOP 可以对业务逻辑的各个部分进行隔离,从而使得业务逻辑各部分之间的耦合度降低,提高程序的可重用性。 - AOP的常用注解
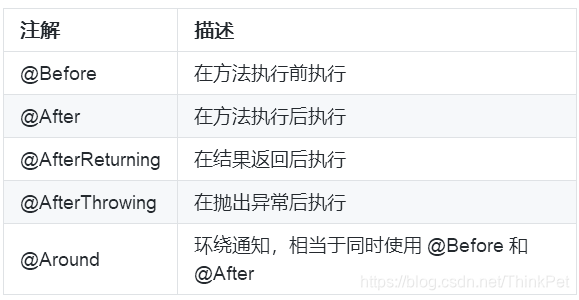
- 使用AOP进行SpringBoot事务控制
package com.xxxx.springboot;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*;
import org.springframework.aop.Advisor;
import org.springframework.aop.aspectj.AspectJExpressionPointcut;
import org.springframework.aop.support.DefaultPointcutAdvisor;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.stereotype.Component;
import org.springframework.transaction.PlatformTransactionManager;
import org.springframework.transaction.interceptor.DefaultTransactionAttribute;
import org.springframework.transaction.interceptor.NameMatchTransactionAttributeSource;
import org.springframework.transaction.interceptor.TransactionInterceptor;
@Aspect
@Component
public class SpringbootAop{
@Autowired
public PlatformTransactionManager platformTransactionManager;
@Bean
public TransactionInterceptor transactionInterceptor(){
DefaultTransactionAttribute defaultTransactionAttribute = new DefaultTransactionAttribute();
defaultTransactionAttribute.setReadOnly(false);
NameMatchTransactionAttributeSource nameMatchTransactionAttributeSource = new NameMatchTransactionAttributeSource();
nameMatchTransactionAttributeSource.addTransactionalMethod("save", defaultTransactionAttribute);
return new TransactionInterceptor(platformTransactionManager, nameMatchTransactionAttributeSource);
}
@Bean
public Advisor advisor(){
AspectJExpressionPointcut aspectJExpressionPointcut = new AspectJExpressionPointcut();
aspectJExpressionPointcut.setExpression("execution(* com.xxx.springboot..*.save(..))");
return new DefaultPointcutAdvisor(aspectJExpressionPointcut, transactionInterceptor());
}
}
- 在save方法里模拟异常,检验aop是否处理了事务
调用以下方法时,会发现除0异常 被AOP拦截处理事务,导致插入的数据被回滚,即没有插入成功。
public User save(User user) {
userRepository.save(user);
int xxaiui = 1 / 0;
user.setPassword("123456");
return userRepository.save(user);
}