ES介绍
Elasticsearch是一款非常强大的开源搜索引擎,能够实现在海量数据中快速检索到满足条件的数据,同时还可以实现分页、高亮显示等功能。
ES实现快速搜索功能的核心概念:倒排索引。将文档的内容通过算法进行分词,得到一个词条列表;将词条列表当作key,包含该词条的文档id列表作为值,形成一张表;词条是唯一的,所以给词条创建索引,提高搜索效率;通过词条查询,得到文档ID,再通过文档ID查询到具体的文档。
MySQL和Elasticsearch概念对比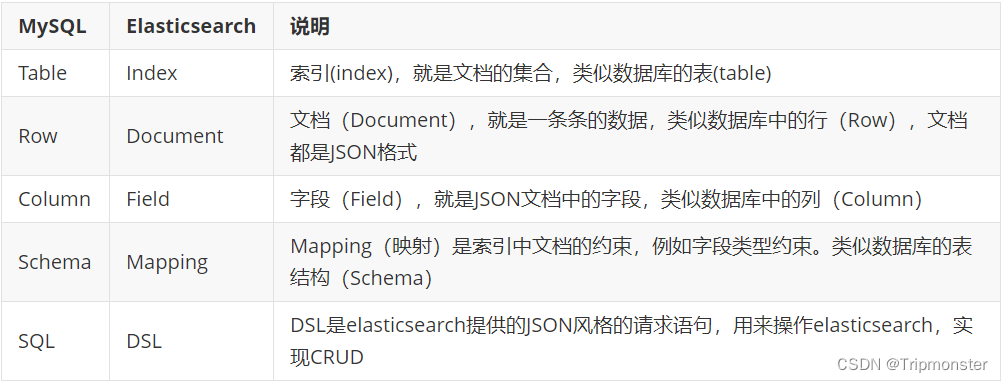
索引库操作
#查询索引库
GET /store
#修改索引库
PUT /heima/_mapping
{
"properties": {
"imageUrl":{
"type": "keyword",
"store": true
}
}
}
#创建索引
PUT /store
{
"mappings": {
"properties": {
"info":{
"type": "text",
"analyzer": "ik_smart",
"index": true,
"store": true
},
"email":{
"type": "text",
"analyzer": "ik_max_word"
},
"brand":{
"type": "keyword"
},
"name":{
"properties": {
"firstName":{
"type":"keyword"
},
"lastName":{
"type":"keyword"
}
}
},
"birth":{
"type": "date",
"format": "yyyy-MM-dd"
}
}
}
}
GET /_analyze
{
"analyzer":"ik_max_word",
"text": "故乡的樱花又开了,奥里给,卷王冲击,法轮功害人不浅"
}
GET _search
{
"query": {
"match_all": {}
}
}
文档操作
#增量修改
POST /store/_update/1001
{
"doc": {
"birth":"2008-11-12"
}
}
#全量修改
PUT /store/_doc/1001
{
"info":"笔记本电脑,方便携带",
"brand":"电子产品",
"email":"comjd@1123.cn",
"birth":"2010-09-18",
"name":{
"firstName":"东",
"lastName":"京"
}
}
#根据id查询文档
GET /store/_doc/1001
#新增文档
POST /store/_doc/1001
{
"info":"笔记本电脑",
"brand":"电子产品",
"email":"comjd@1123.cn",
"birth":"2010-09-18",
"name":{
"firstName":"东",
"lastName":"京"
}
}
JAVA操作ES
1.在kibana分析并创建索引库
PUT /hotel
{
"mappings": {
"properties": {
"id":{
"type": "keyword"
},
"name":{
"type":"text",
"analyzer": "ik_smart",
"copy_to": "all"
},
"address":{
"type": "text",
"analyzer": "ik_smart",
"copy_to": "all"
},
"price":{
"type": "integer"
},
"score":{
"type": "integer"
},
"brand":{
"type": "keyword",
"copy_to": "all"
},
"city":{
"type": "keyword",
"copy_to": "all"
},
"starName":{
"type": "keyword"
},
"business":{
"type": "text",
"analyzer": "ik_smart",
"copy_to": "all"
},
"location":{
"type": "geo_point"
},
"pic":{
"type": "keyword",
"index": false
},
"all":{
"type": "text",
"analyzer": "ik_smart"
}
}
}
}
2.初始化RestClient
2.1导入依赖并修改ES版本(docker中es版本需要和kibana版本一致)
2.2.配置类
2.3. 常量类EsContant
2.4.JAVA代码中进行索引库操作
@SpringBootTest
public class HotelDemoApplicationTests {
@Autowired
RestHighLevelClient restHighLevelClient;
//创建索引库
@Test
public void createIndexTest() throws Exception {
//执行创建索引的对,指定索引名
CreateIndexRequest createIndexRequest = new CreateIndexRequest(EsConstant.HOTEL_INDEX);
//指定映射
createIndexRequest.source(EsConstant.HOTEL_MAPPING, XContentType.JSON);
//创建索引
CreateIndexResponse response = restHighLevelClient.indices().create(createIndexRequest, RequestOptions.DEFAULT);
String index = response.index();
boolean ack = response.isAcknowledged();
System.out.println(index + ":" + ack);
}
//查询索引库
@Test
public void getIndexTest() throws IOException {
GetIndexRequest getIndexRequest = new GetIndexRequest(EsConstant.HOTEL_INDEX);
GetIndexResponse response = restHighLevelClient.indices().get(getIndexRequest, RequestOptions.DEFAULT);
System.out.println(response);
}
//删除索引库
@Test
public void deleteIndexTest() throws IOException {
DeleteIndexRequest deleteIndexRequest = new DeleteIndexRequest(EsConstant.HOTEL_INDEX);
AcknowledgedResponse delete = restHighLevelClient.indices().delete(deleteIndexRequest, RequestOptions.DEFAULT);
System.out.println(delete);
}
//判断索引库是否存在
@Test
public void existIndexTest() throws IOException {
GetIndexRequest getIndexRequest = new GetIndexRequest(EsConstant.HOTEL_INDEX);
boolean b = restHighLevelClient.indices().exists(getIndexRequest, RequestOptions.DEFAULT);
System.out.println(b);
}
}