学更好的别人,
做更好的自己。
——《微卡智享》
本文长度为3657字,预计阅读8分钟
前言
关于Quartz的使用在《项目实战|C#Socket通讯方式改造(二)--利用Quartz实现定时任务处理》中已经写过一篇,不过那个是在.net framework中的使用,在.net5下使用起来还是有一些差别的,所以这篇主要就是介绍一下Quartz在.net5下的使用。
Quartz在.net5下使用
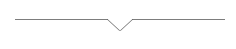
微卡智享
01
安装Quartz的Nuget包
在创建的.net5项目的依赖项中,添加Nuget包,这里要找到Quartz.AspNetCore的包进行安装,(.net framework中使用的话直接先QUartz即可)。
02
创建调度任务
创建了一个JobListenIIS的类,继承IJob接口,在接口的Execute的实现方法中写入我们的任务函数即可。这里和在.net framework中的区别就是Execute的函数返回的是一个Task,而.net framework中这个函数是无返回值的(void),所以输出的是 return Task.Run();
using MedicalPlatform.DAL.Log4Net;
using Quartz;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Text;
using System.Threading.Tasks;
namespace MedicalPaltform.Utils.Quartz
{
public class JobListenIIS : IJob
{
public Task Execute(IJobExecutionContext context)
{
return Task.Run(() =>
{
string url = @"http://localhost:8051/api/test";
try
{
WebClient client = new WebClient();
string desc = client.DownloadString(url);
LogHelper.Info(desc);
}
catch (Exception e)
{
LogHelper.Error(e.Message);
}
});
}
}
}
本项目中写了三个调度任务,所以也创建了三个类,实现的方法在自己的类中写过,这个地方直接调用即可。
03
创建任务调度类
创建了QuartzStartUp的任务调度类,在Start()方法中加入了创建任务,触发器及绑定,这里基本和.net framework的创建及绑定差不多。代码中GetSectionValue的方法就是上篇中说到怎么读取appsettings.json配置的用法。
QuartzStartUp代码
using MedicalPlatform.DAL.Log4Net;
using MedicalPlatform.DAL.Znyg;
using Quartz;
using Quartz.Impl;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace MedicalPaltform.Utils.Quartz
{
public class QuartzStartUp
{
//调度工厂
private ISchedulerFactory _factory;
//调度器
private IScheduler _scheduler;
/// <summary>
/// 初始化参数
/// </summary>
public static void initZnygConfig()
{
//读取SQL的各个参数
string server = ConfigHelper.GetSectionValue("Znyg:SrvName");
string database = ConfigHelper.GetSectionValue("Znyg:DbName");
string uid = ConfigHelper.GetSectionValue("Znyg:UserId");
string pwd = ConfigHelper.GetSectionValue("Znyg:UserPsw");
string connstr = "server=" + server + ";uid=" + uid + ";pwd=" + pwd + ";database=" + database;
//设置药柜数据库参数
YGDbCon.setConnectParam(connstr);
}
/// <summary>
///
/// </summary>
/// <returns></returns>
public async Task<string> Start()
{
initZnygConfig();
//读取医嘱生成间隔时间
int pickInfoInvSec = Convert.ToInt32(ConfigHelper.GetSectionValue("Znyg:PickInfoInvSec"));
int replenishInvSec = Convert.ToInt32(ConfigHelper.GetSectionValue("Znyg:ReplenishInvSec"));
//1、声明一个调度工厂
_factory = new StdSchedulerFactory();
//2、通过调度工厂获得调度器
_scheduler = await _factory.GetScheduler();
//3、开启调度器
await _scheduler.Start();
//4、创建任务
IJobDetail jobpickinfo = JobBuilder.Create<JobPickInfo>().Build();
IJobDetail jobreplenishinfo = JobBuilder.Create<JobReplenishInfo>().Build();
IJobDetail joblisteniis = JobBuilder.Create<JobListenIIS>().Build();
//5、创建一个触发器
ITrigger triggerpickinfo = TriggerBuilder.Create()
.WithIdentity("JobPickInfo", "PickInfo")
.WithSimpleSchedule(x => x.WithIntervalInSeconds(pickInfoInvSec).RepeatForever())
.Build();
ITrigger triggerreplenishinfo = TriggerBuilder.Create()
.WithIdentity("JobReplenishInfo", "ReplenishInfo")
.WithSimpleSchedule(x => x.WithIntervalInSeconds(replenishInvSec).RepeatForever())
.Build();
ITrigger triggerlisteniis = TriggerBuilder.Create()
.WithIdentity("JobListenIIS", "IIS")
.WithSimpleSchedule(t => t.WithIntervalInMinutes(15).RepeatForever())
.Build();
//6、将触发器和任务器绑定到调度器中
await _scheduler.ScheduleJob(jobpickinfo, triggerpickinfo);
await _scheduler.ScheduleJob(jobreplenishinfo, triggerreplenishinfo);
await _scheduler.ScheduleJob(joblisteniis, triggerlisteniis);
return await Task.FromResult("将触发器和任务器绑定到调度器中完成");
}
public void Stop()
{
if(_scheduler != null)
{
_scheduler.Clear();
_scheduler.Shutdown();
}
_scheduler = null;
_factory = null;
}
}
}
04
注册Quartz
这一步是和.net framework中差距最大的,Quartz的注册需要在startup的类中进行实现:
在ConfigureServices中注入调度类
在Configure中启动Quartz
在ConfigureServices中注入调度类
在Configure中启动Quartz
启动调度的函数中用到了appLifetime,默认的Configure的方法中没有IHostApplicationLifetime appLifetime这个参数,需要把这个参数加进来。
startup代码
using MedicalPaltform.Utils.Quartz;
using MedicalPlatform.DAL.Log4Net;
using Microsoft.AspNetCore.Builder;
using Microsoft.AspNetCore.Hosting;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Configuration;
using Microsoft.Extensions.DependencyInjection;
using Microsoft.Extensions.Hosting;
using Microsoft.Extensions.Logging;
using Microsoft.OpenApi.Models;
using Quartz;
using Quartz.Impl;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Threading.Tasks;
namespace MedicalPlatform
{
public class Startup
{
public Startup(IConfiguration configuration)
{
Configuration = configuration;
LogHelper.Configure(); //使用前先配置
}
public IConfiguration Configuration { get; }
// This method gets called by the runtime. Use this method to add services to the container.
public void ConfigureServices(IServiceCollection services)
{
services.AddControllers();
services.AddSwaggerGen(c =>
{
c.SwaggerDoc("v1", new OpenApiInfo { Title = "MedicalPlatform", Version = "v1" });
});
#region 注入 Quartz调度类
services.AddSingleton<QuartzStartUp>();
//注册ISchedulerFactory的实例。
services.AddSingleton<ISchedulerFactory, StdSchedulerFactory>();
#endregion
}
// This method gets called by the runtime. Use this method to configure the HTTP request pipeline.
public void Configure(IApplicationBuilder app, IWebHostEnvironment env, IHostApplicationLifetime appLifetime
, ILoggerFactory loggerFactory)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
app.UseSwagger();
app.UseSwaggerUI(c => c.SwaggerEndpoint("/swagger/v1/swagger.json", "MedicalPlatform v1"));
}
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers();
});
#region Quartz任务调度
//获取前面注入的Quartz调度类
var quartz = app.ApplicationServices.GetRequiredService<QuartzStartUp>();
appLifetime.ApplicationStarted.Register(() =>
{
quartz.Start().Wait();
});
appLifetime.ApplicationStopped.Register(() =>
{
quartz.Stop();
});
#endregion
}
}
}
这样Quartz在.net5下就可以正常使用了。
完
扫描二维码
获取更多精彩
微卡智享
「 往期文章 」
Android CameraX NDK OpenCV(三)-- 人脸贴图替换