IO
一、缓冲流
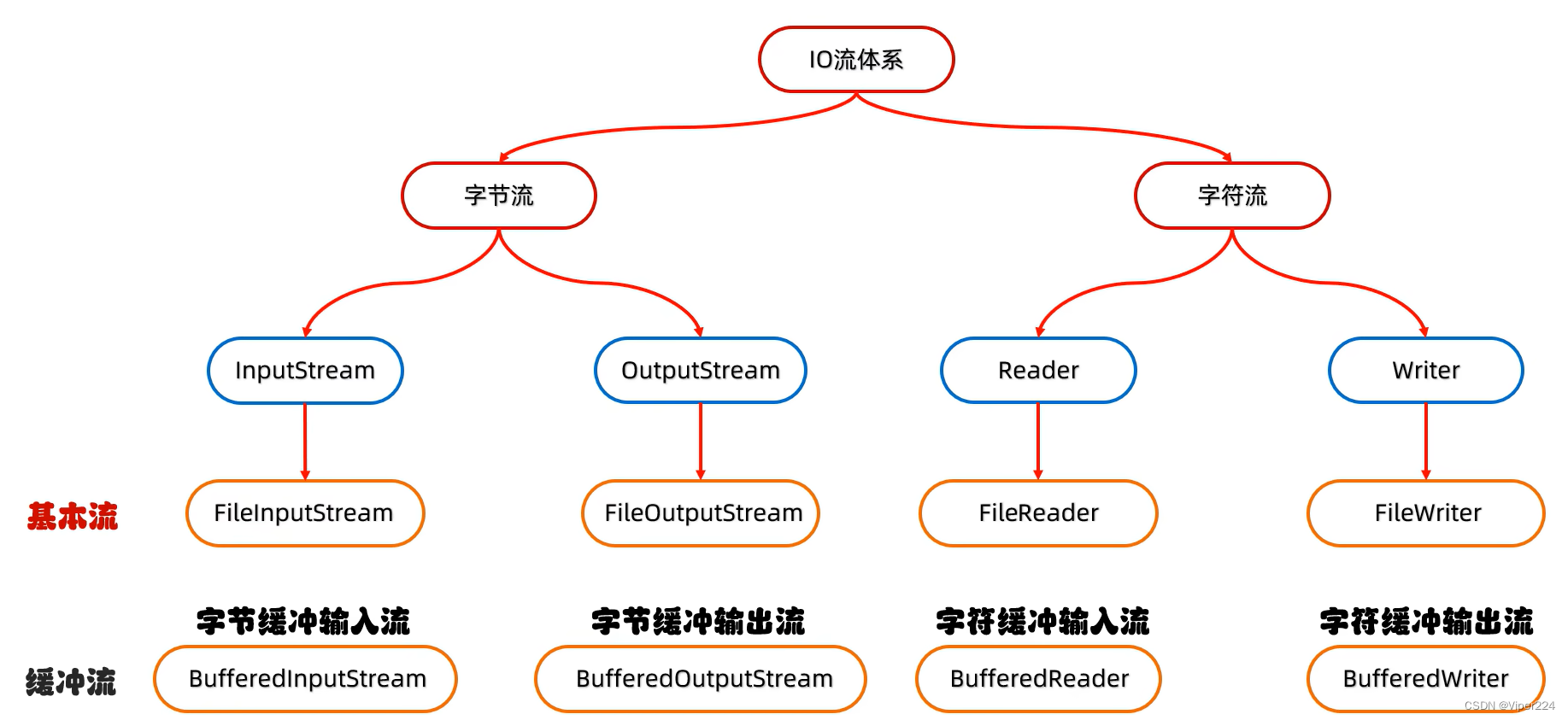
1、字节缓冲流
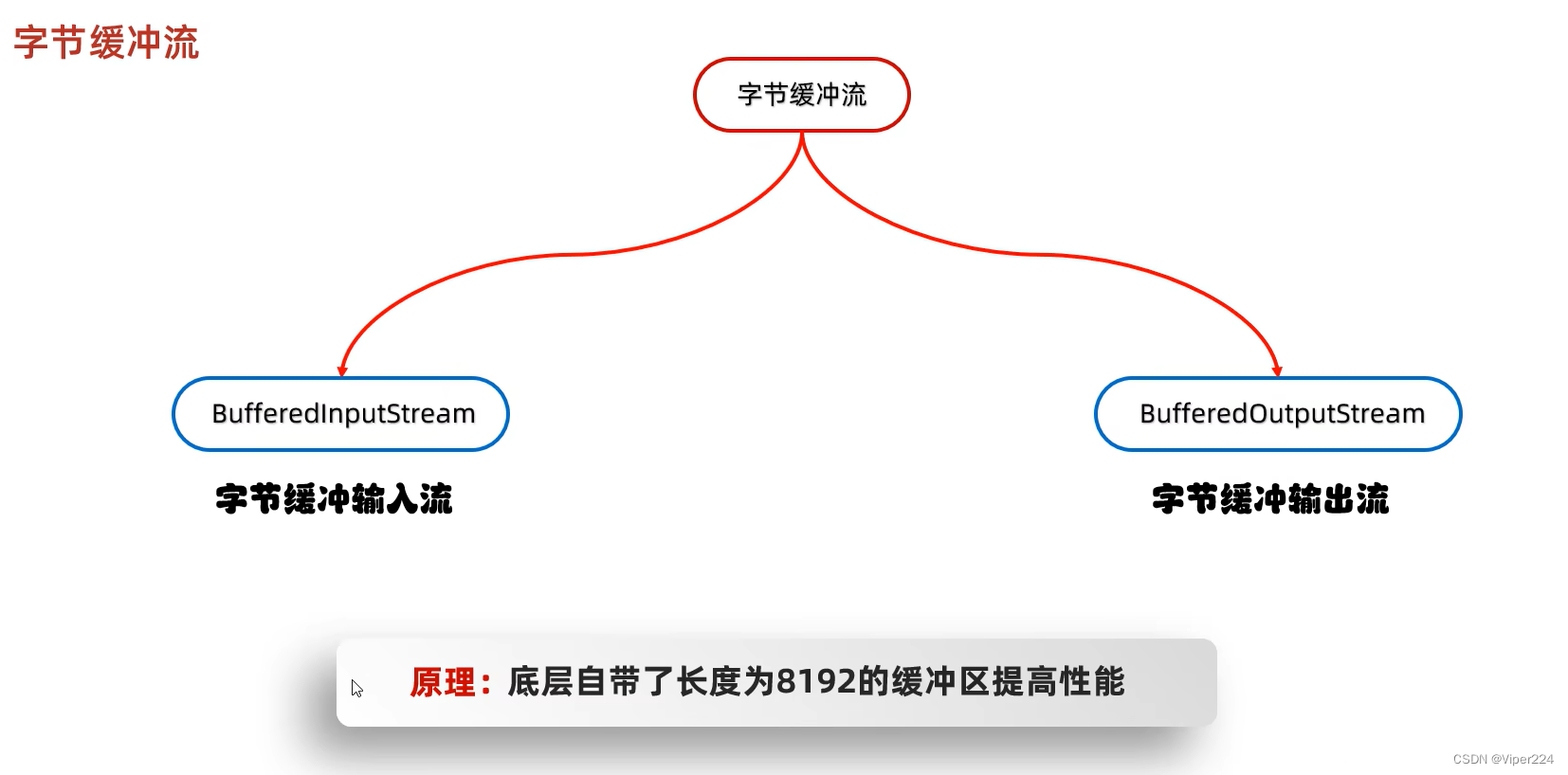
①字节缓冲流拷贝
public class Test1 {
public static void main(String[] args) throws IOException {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("day26-code\\aaa\\b.txt"));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("day26-code\\aaa\\c.txt"));
int b;
while ((b = bis.read()) != -1) {
bos.write(b);
}
bos.close();
bis.close();
}
}
public class Test1 {
public static void main(String[] args) throws IOException {
BufferedInputStream bis = new BufferedInputStream(new FileInputStream("day26-code\\aaa\\b.txt"));
BufferedOutputStream bos = new BufferedOutputStream(new FileOutputStream("day26-code\\aaa\\d.txt"));
int len;
byte[] bytes = new byte[1024];
while ((len = bis.read(bytes)) != -1) {
bos.write(bytes, 0, len);
}
bos.close();
bis.close();
}
}
②缓冲区原理
通过在内存中创建缓冲区,降低了IO次数,由于内存的速度比IO快很多,这样就加快了传输的速度,属于空间换时间的思想
2、字符缓冲流
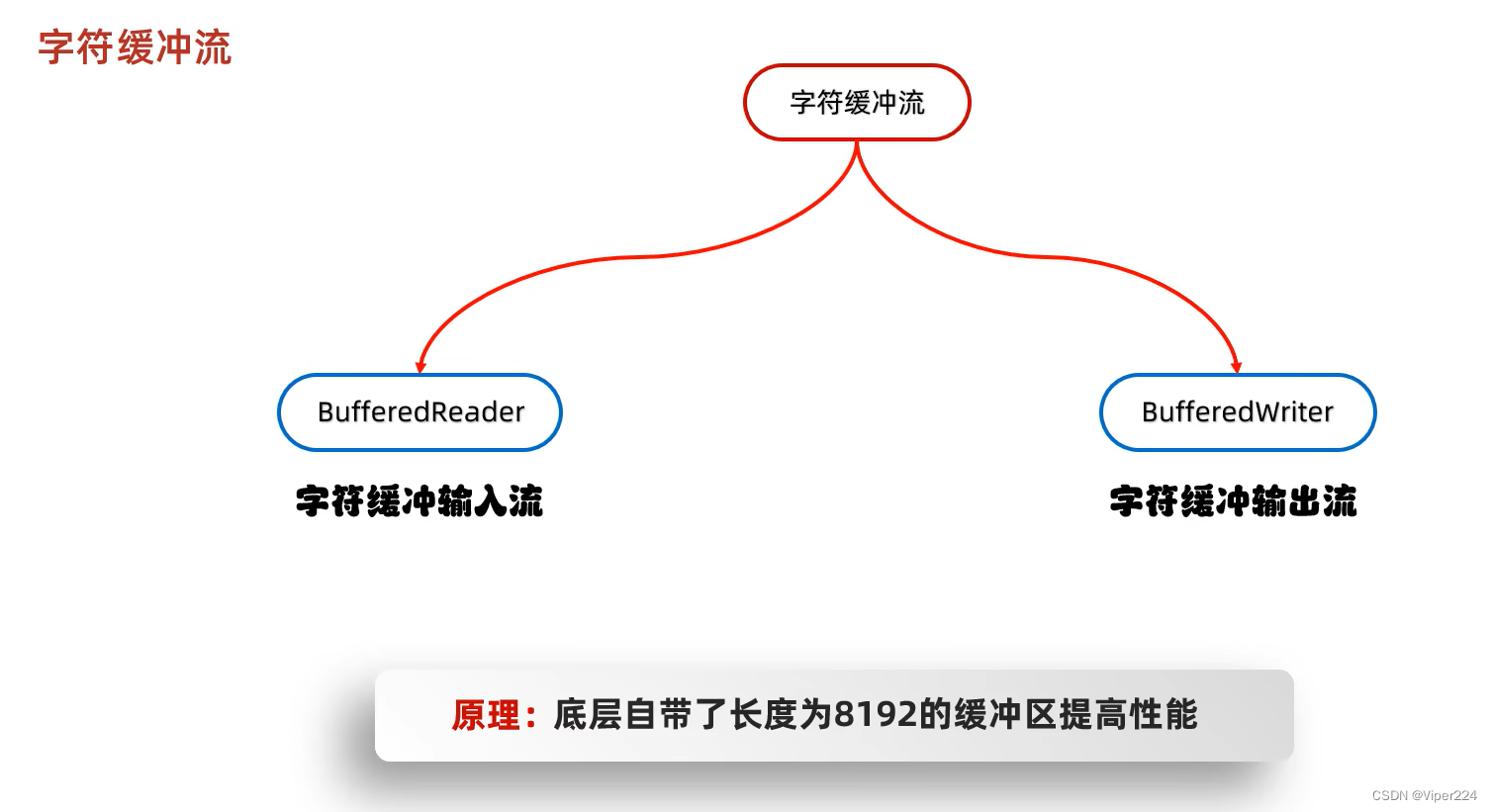
①字符流本来就带有缓冲池,所以字符缓冲流主要用处是使用其特有的一些方法,
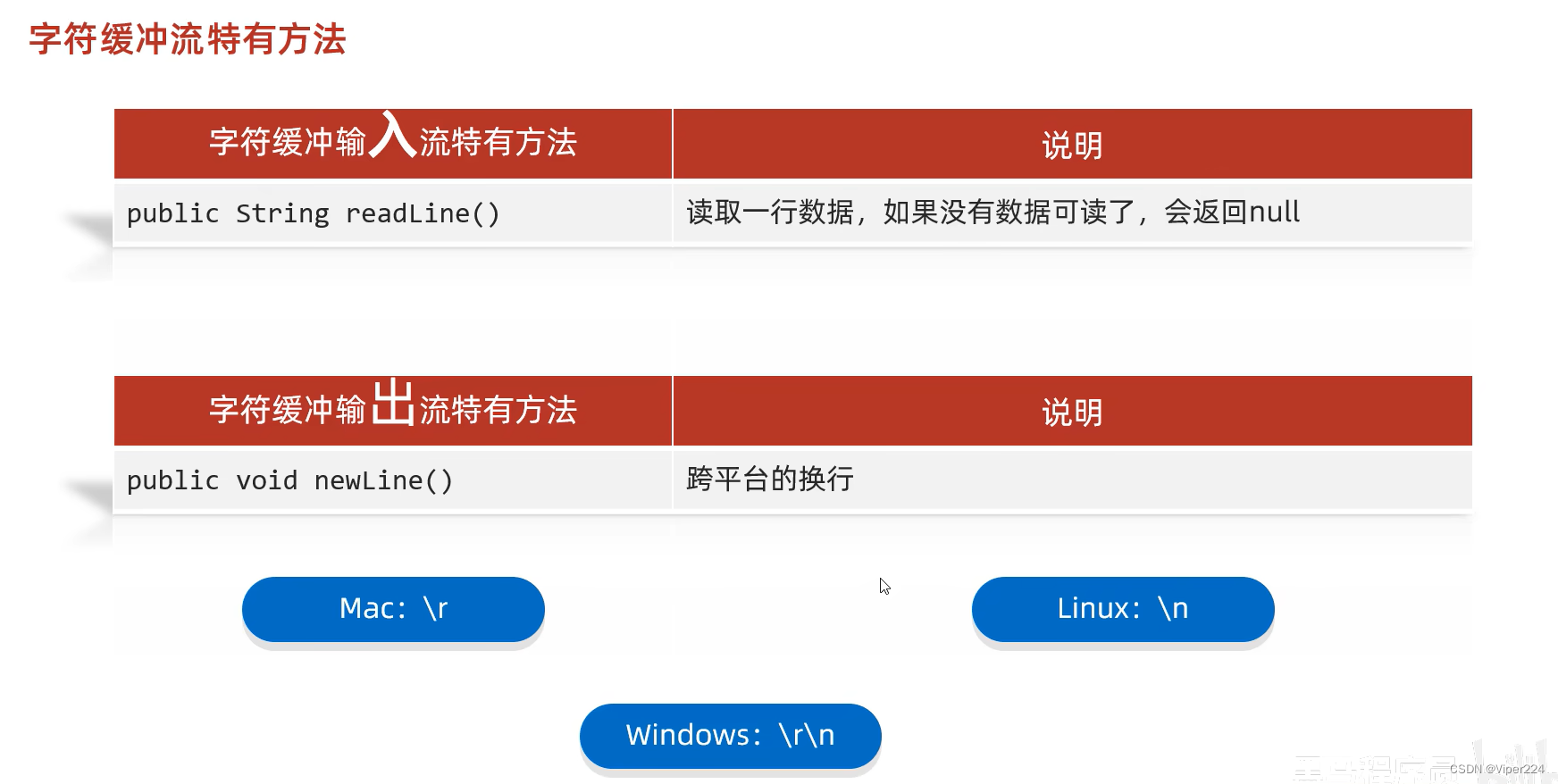
public class Test2 {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("day26-code\\aaa\\b.txt"));
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
br.close();
}
}
public class Test2 {
public static void main(String[] args) throws IOException {
BufferedWriter bw = new BufferedWriter(new FileWriter("day26-code\\aaa\\e.txt"));
bw.write("你嘴角上扬的样子");
bw.newLine();
bw.write("百度搜索不到");
bw.newLine();
bw.close();
}
}
②注意加上续写参数的时候,参数是加在FileWriter初始化当中的,不要写到BufferedWriter中
③缓冲区的大小,对于字节流而言是8192个字节也就是8KB,对于字符流而言是8192个字符,注意严谨
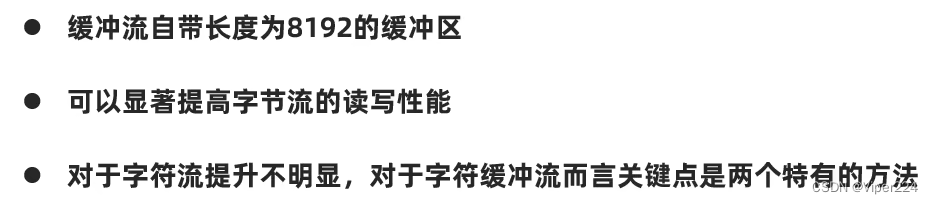
④练习:给出师表排序
public class Test3 {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new FileReader("day26-code\\aaa\\csb.txt"));
TreeMap<Integer, String> tm = new TreeMap<>();
String line;
while ((line = br.readLine()) != null) {
String[] arr = line.split("\\.");
tm.put(Integer.parseInt(arr[0]), arr[1]);
}
br.close();
BufferedWriter bw = new BufferedWriter(new FileWriter("day26-code\\aaa\\csb1.txt"));
for (Map.Entry<Integer, String> entry : tm.entrySet()) {
bw.write(entry.getKey() + "." + entry.getValue());
bw.newLine();
}
bw.close();
}
}
二、转换流
用于连接字节流和字符流
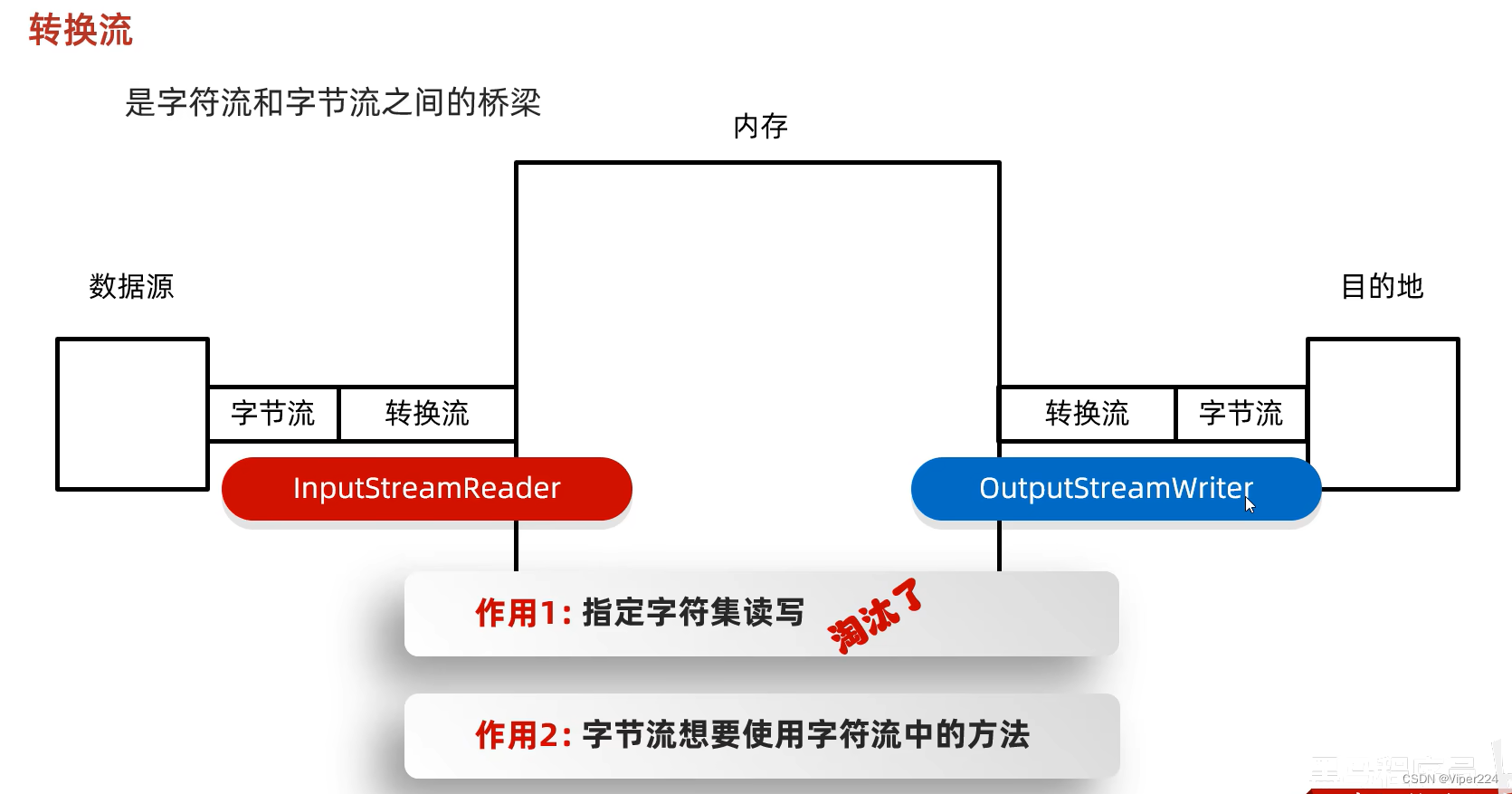
1、指定字符集读写
public class Test1 {
public static void main(String[] args) throws IOException {
InputStreamReader isr = new InputStreamReader(new FileInputStream("day26-code\\aaa\\gbkfile.txt"), "GBK");
int ch;
while ((ch = isr.read()) != -1) {
System.out.print((char) ch);
}
isr.close();
}
}
public class Test2 {
public static void main(String[] args) throws IOException {
InputStreamReader isr = new InputStreamReader(new FileInputStream("day26-code\\aaa\\gbkfile.txt"), "GBK");
OutputStreamWriter osw = new OutputStreamWriter(new FileOutputStream("day26-code\\aaa\\utffile.txt"), "UTF-8");
int ch;
while ((ch = isr.read()) != -1) {
osw.write(ch);
}
osw.close();
isr.close();
}
}
但是这种情况下可以使用字符流更加简单
public class Test1 {
public static void main(String[] args) throws IOException {
FileReader fr = new FileReader("day26-code\\aaa\\gbkfile.txt", Charset.forName("GBK"));
int ch;
while ((ch = fr.read()) != -1) {
System.out.print((char) ch);
}
fr.close();
}
}
2、字节流想要使用字符流中的方法
例如想要用字节流每次读取一行数据,而且不能出现乱码
public class Test3 {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(new FileInputStream("day26-code\\aaa\\utffile.txt")));
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
br.close();
}
}
三、序列化流和反序列化流
1、序列化流
①标记性接口
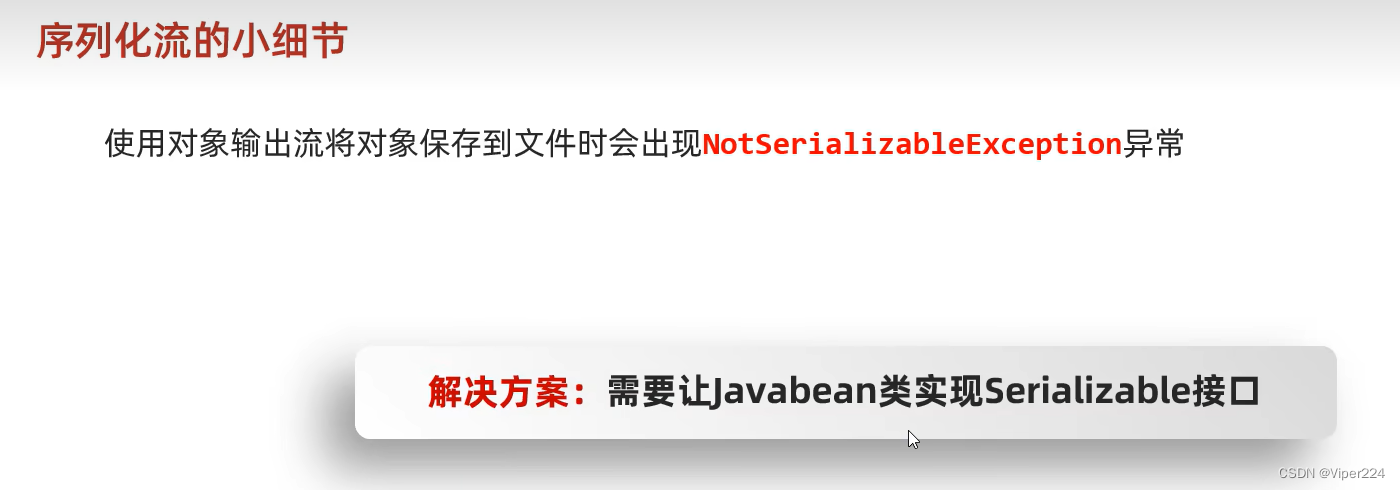
②定义序列版本号
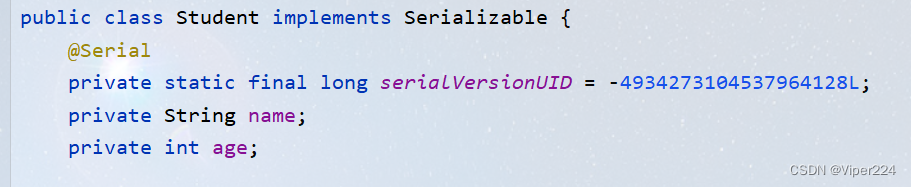
public class Test1 {
public static void main(String[] args) throws IOException {
Student st = new Student("zhangsan", 23);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("day26-code\\object.txt"));
oos.writeObject(st);
oos.close();
}
}
2、反序列化流
public class Test1 {
public static void main(String[] args) throws IOException, ClassNotFoundException {
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("day26-code\\object.txt"));
Object o = ois.readObject();
ois.close();
System.out.println(o);
}
}
①瞬态关键字 transient
瞬态关键字修饰的变量,不会被序列化
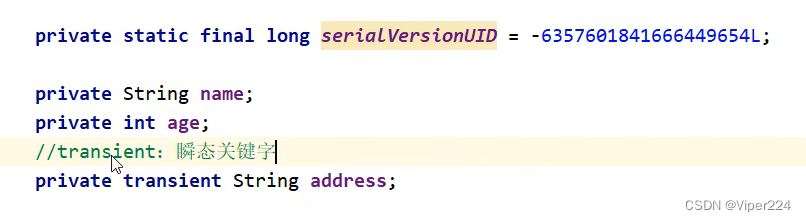
3、总结
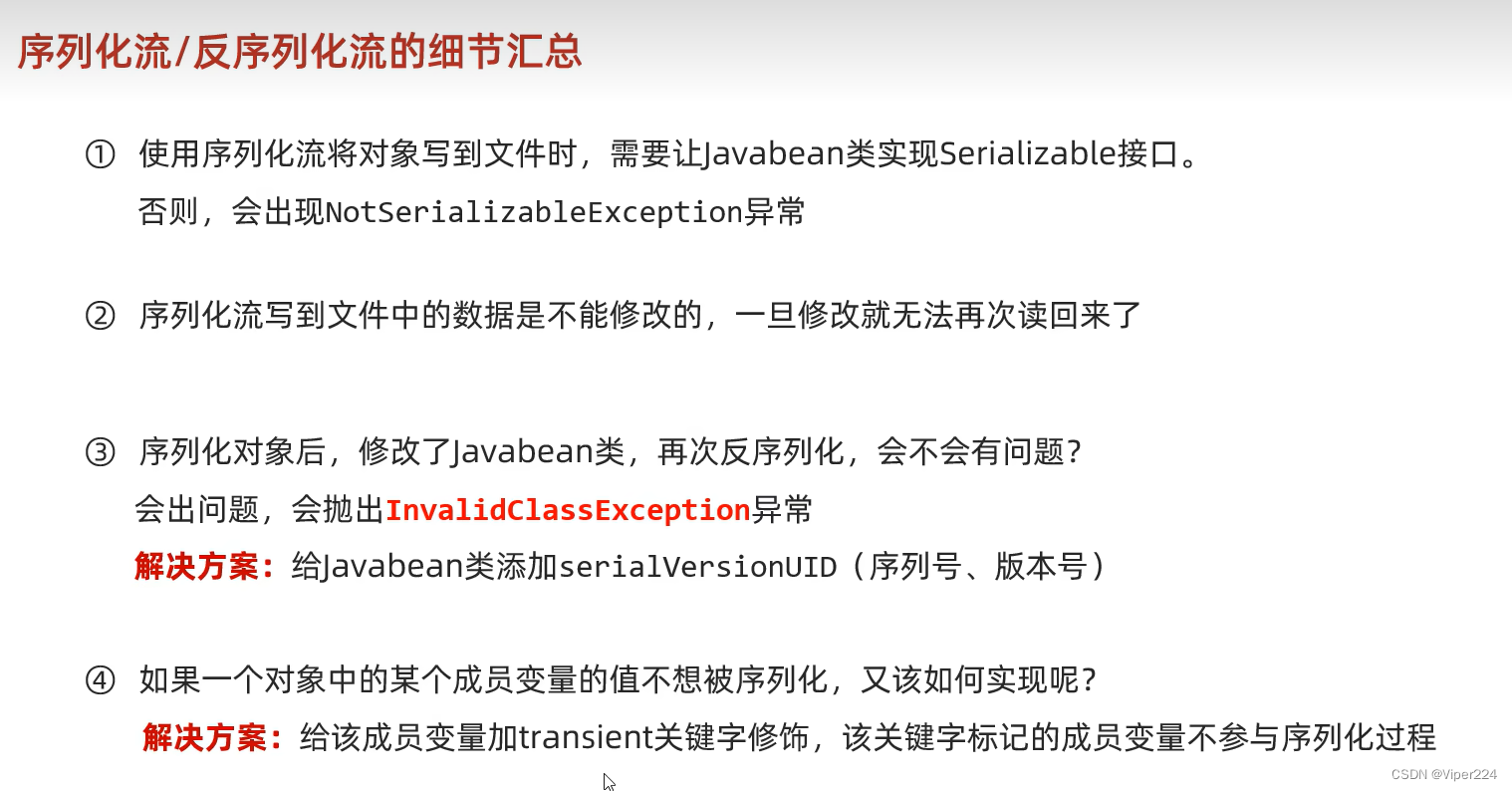
4、批量序列化和反序列化
public class Test2 {
public static void main(String[] args) throws IOException, ClassNotFoundException {
Teacher t1 = new Teacher("zhangsan", 23, "南京");
Teacher t2 = new Teacher("lisi", 25, "江西");
Teacher t3 = new Teacher("wangwu", 26, "河北");
ArrayList<Teacher> list = new ArrayList<>();
list.add(t1);
list.add(t2);
list.add(t3);
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("day26-code\\teacher.txt"));
oos.writeObject(list);
oos.close();
ObjectInputStream ois = new ObjectInputStream(new FileInputStream("day26-code\\teacher.txt"));
ArrayList<Teacher> listResult = (ArrayList<Teacher>) ois.readObject();
System.out.println(listResult);
}
}
四、字节打印流和字符打印流
打印流只能写不能读
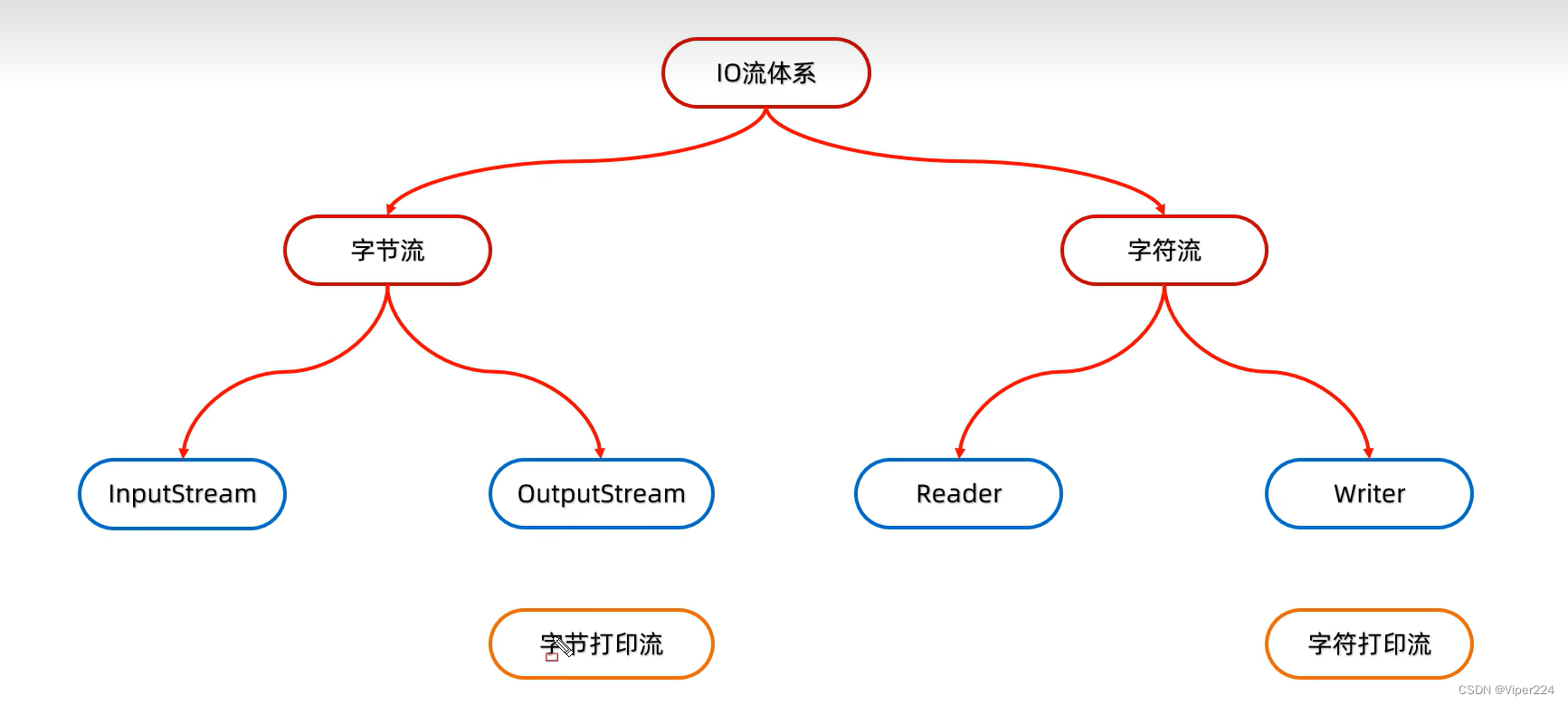
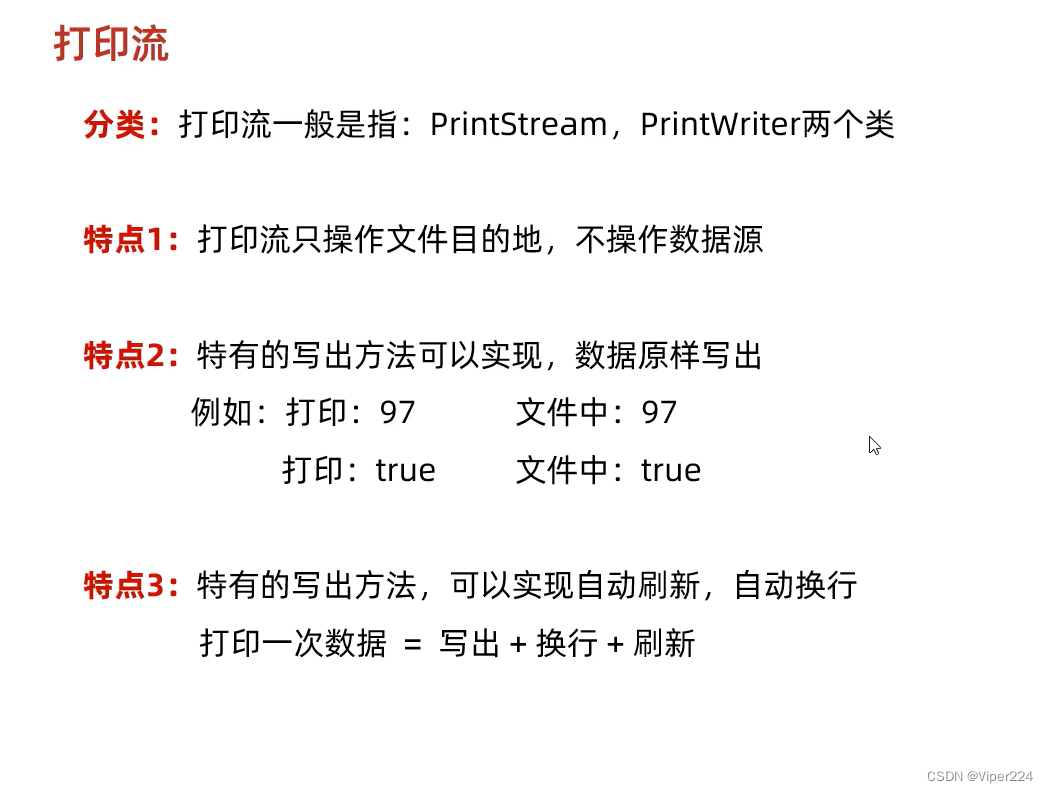
1、字节打印流
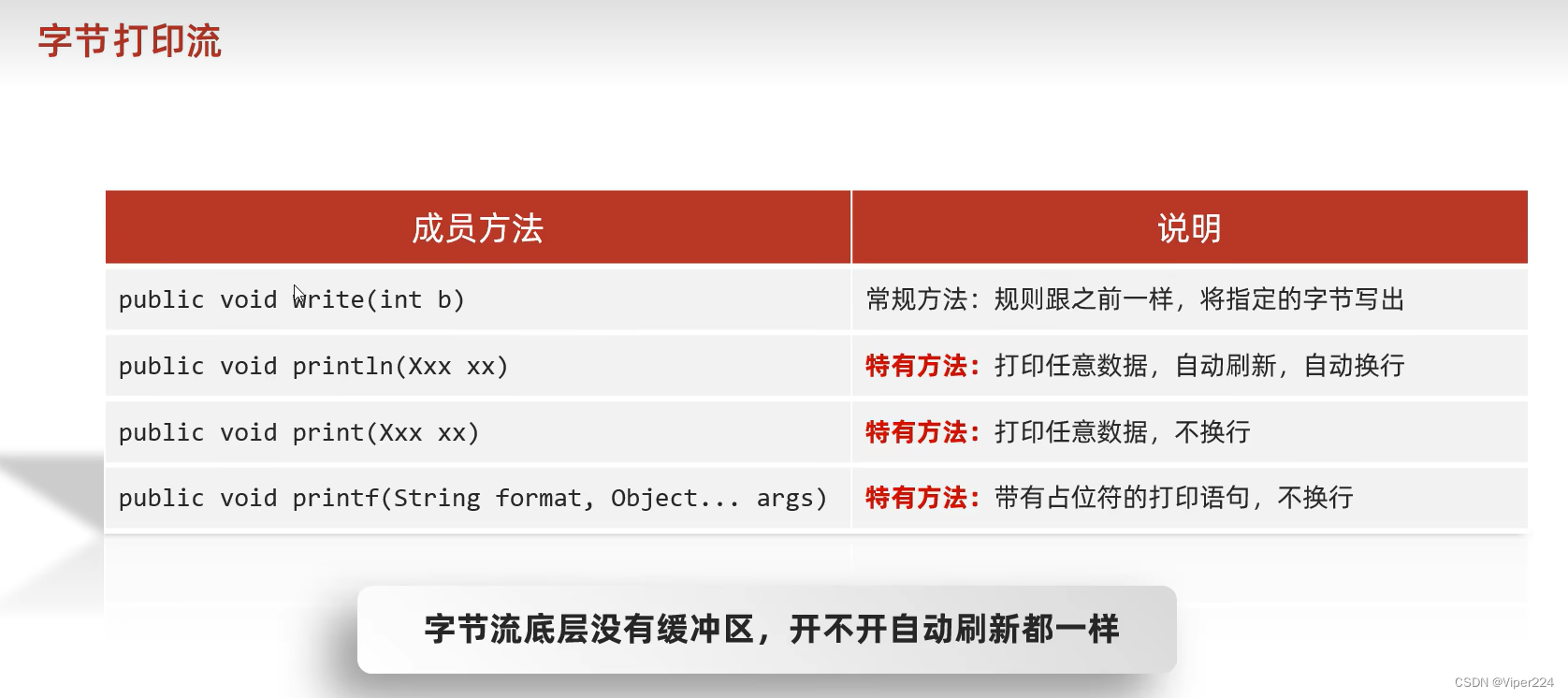
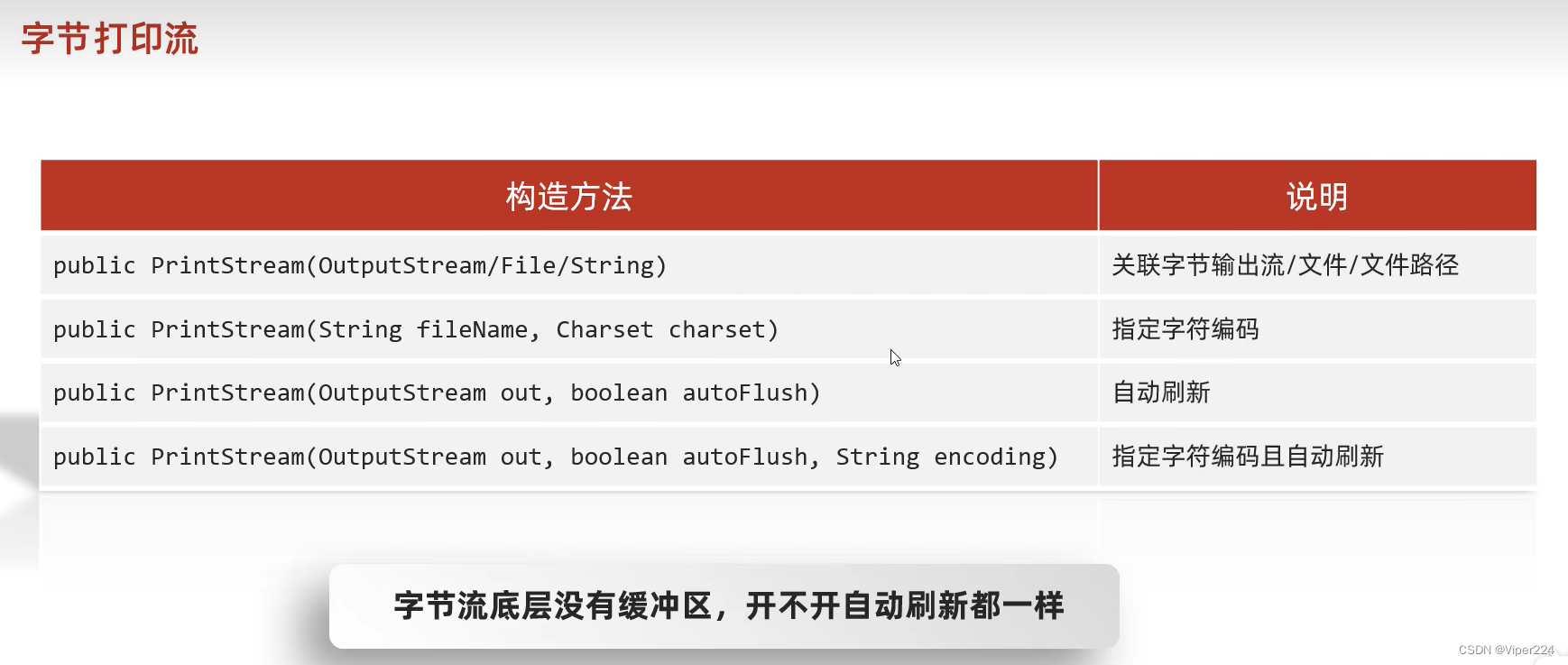
2、字符打印流
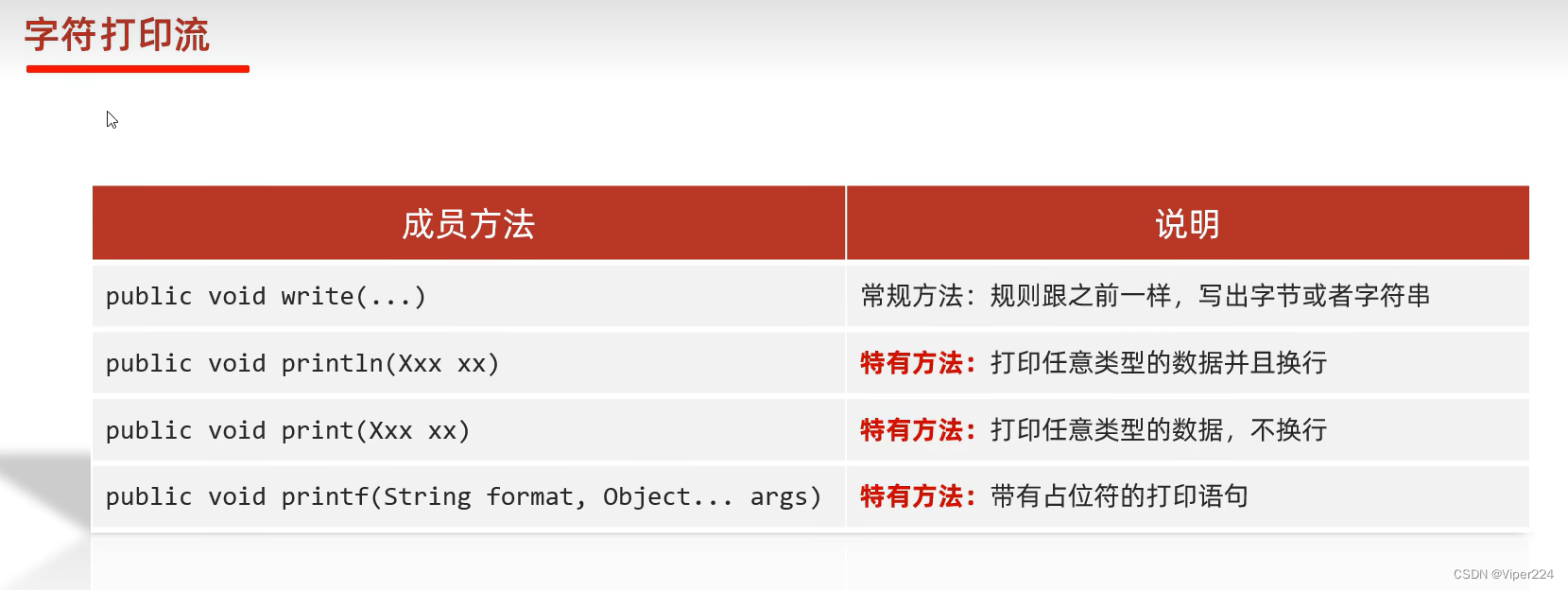
3、标准输出流(系统打印流)PrintStream
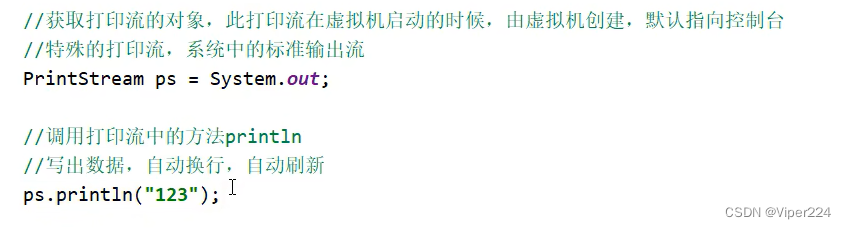
五、解压缩流和压缩流
1、解压缩流
public class Test1 {
public static void main(String[] args) throws IOException {
File f1 = new File("D:\\src.zip");
File f2 = new File("D:\\mysrc");
unzip(f1, f2);
}
private static void unzip(File src, File dest) throws IOException {
ZipInputStream zis = new ZipInputStream(new FileInputStream(src));
ZipEntry zipEntry;
while ((zipEntry = zis.getNextEntry()) != null) {
if (zipEntry.isDirectory()) {
File file = new File(dest, zipEntry.toString());
file.mkdirs();
} else {
FileOutputStream fos = new FileOutputStream(new File(dest, zipEntry.toString()));
int b;
while ((b = zis.read()) != -1) {
fos.write(b);
}
fos.close();
zis.closeEntry();
}
}
zis.close();
}
}
2、压缩流
public class Test2 {
public static void main(String[] args) throws IOException {
File src = new File("D:\\dest\\a.txt");
File zip = new File("D:\\");
tozip(src, zip);
}
private static void tozip(File src, File zip) throws IOException {
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(new File(zip, "a.zip")));
ZipEntry entry = new ZipEntry("a.txt");
zos.putNextEntry(entry);
FileInputStream fis = new FileInputStream(src);
int b;
while ((b = fis.read()) != -1) {
zos.write(b);
}
fis.close();
zos.closeEntry();
zos.close();
}
}
3、压缩文件夹
public class Test5 {
public static void main(String[] args) throws IOException {
File src = new File("D:\\src");
File destParent = src.getParentFile();
File dest = new File(destParent, src.getName() + ".zip");
ZipOutputStream zos = new ZipOutputStream(new FileOutputStream(dest));
zipDic(src, zos, src.getName());
zos.close();
}
private static void zipDic(File src, ZipOutputStream zos, String name) throws IOException {
File[] files = src.listFiles();
if (files != null) {
for (File file : files) {
if (file.isFile()) {
ZipEntry entry = new ZipEntry(name + "\\" + file.getName());
zos.putNextEntry(entry);
FileInputStream fis = new FileInputStream(file);
int b;
while ((b = fis.read()) != -1) {
zos.write(b);
}
fis.close();
zos.closeEntry();
} else {
zipDic(file, zos, name + "\\" + file.getName());
}
}
}
}
}
六、Commons-io
工具包,前面学的全都有,经典白学,嘻嘻^^
七、Hotool
工具包,前面学的全都有,经典白学,嘻嘻^^