功能介绍:
当鼠标点击某张图片,所有图片以相同速度向某定位点进行移动,最后使点击图片完全展露,实现图片展开效果。
效果预览:
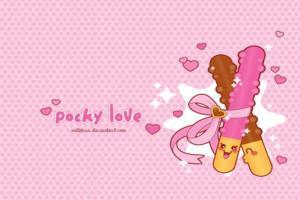
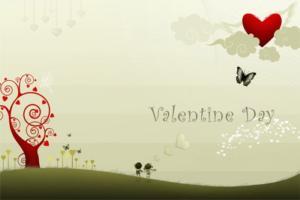
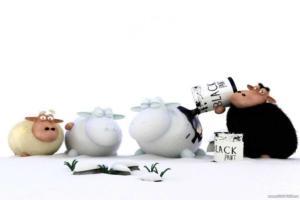
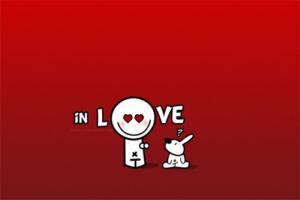
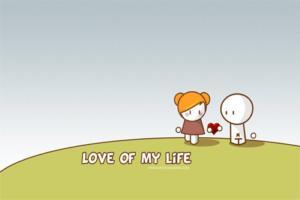
创建构造函数,并在里面调用初始化函数,传入参数稍后介绍;
1
function
gallery()
2 {
3 this .init.apply( this ,arguments);
4 }
2 {
3 this .init.apply( this ,arguments);
4 }
初始化方法init()接受4个参数,分别为:id:包含图片容器id,size:包含图片容器大小,showSize:展开图片大小,speed:折叠/展开速度,默认值为10;
1
init:
function
(id,size,showSize,speed){
2 var container = document.getElementById(id),
3 that = this ;
4 container.style.height = size + " px " ;
5 container.style.overflow = " hidden " ;
6 container.style.position = " relative " ;
7 this .span = []; // 保存文本节点
8 this .speed = speed || 10 ;
9 this .showHeight = showSize;
10 this .div = container.getElementsByTagName( " div " );
11 var len = this .div.length;
12 this .closeHeight = (size - this .showHeight) / (len-1);
13 this .count = len;
14 this .handle = null ;
15 this .img = container.getElementsByTagName( " img " );
16 this .each( function (i){
17 if (i === 0 ){
18 this .top = 0 ; // 保存目标位置
19 } else {
20 this .top = that.showHeight + that.closeHeight * (i - 1 );
21 }
22 this .style.position = " absolute " ;
23 this .style.top = this .top + " px " ;
24 addEventHandler( this , " click " , function (){
25 that.set.call(that,i);
26 });
27 that.createTitle( this ,i);
28 });
29 this .span[ 0 ].style.display = " none " ;
30 },
2 var container = document.getElementById(id),
3 that = this ;
4 container.style.height = size + " px " ;
5 container.style.overflow = " hidden " ;
6 container.style.position = " relative " ;
7 this .span = []; // 保存文本节点
8 this .speed = speed || 10 ;
9 this .showHeight = showSize;
10 this .div = container.getElementsByTagName( " div " );
11 var len = this .div.length;
12 this .closeHeight = (size - this .showHeight) / (len-1);
13 this .count = len;
14 this .handle = null ;
15 this .img = container.getElementsByTagName( " img " );
16 this .each( function (i){
17 if (i === 0 ){
18 this .top = 0 ; // 保存目标位置
19 } else {
20 this .top = that.showHeight + that.closeHeight * (i - 1 );
21 }
22 this .style.position = " absolute " ;
23 this .style.top = this .top + " px " ;
24 addEventHandler( this , " click " , function (){
25 that.set.call(that,i);
26 });
27 that.createTitle( this ,i);
28 });
29 this .span[ 0 ].style.display = " none " ;
30 },
在init()中计算出折叠后的图片高度和展开后的图片高度,获取所有图片调用each()方法设置绝对定位初始位置;
1
each:
function
(fn){
2 for ( var i = 0 ;i < this .count;i ++ )
3 fn.call( this .div[i],i);
4 },
2 for ( var i = 0 ;i < this .count;i ++ )
3 fn.call( this .div[i],i);
4 },
获取图片的title属性,创建新节点添加到图片左上角,假如不存在title属性,默认字符串“点击展开”;
1
createTitle:
function
(element,i){
2 var text = this .img[i].title ? this .img[i].title: " 点击展开 " ;
3 var span = document.createElement( " span " );
4 span.innerHTML = text;
5 element.appendChild(span);
6 span.style.position = " absolute " ;
7 span.style.top = " 5px " ;
8 span.style.right = " 5px " ;
9 span.style.border = " 1px dotted #333 "
10 this .span.push(span);
11 },
2 var text = this .img[i].title ? this .img[i].title: " 点击展开 " ;
3 var span = document.createElement( " span " );
4 span.innerHTML = text;
5 element.appendChild(span);
6 span.style.position = " absolute " ;
7 span.style.top = " 5px " ;
8 span.style.right = " 5px " ;
9 span.style.border = " 1px dotted #333 "
10 this .span.push(span);
11 },
添加点击事件处理程序set(),根据点击,重新设置图片的目标定位位置,并使点击图片指示标签隐藏,其它恢复;
1
set:
function
(index){
2 var that = this ;
3 this .each( function (i){
4 this .top = (i <= index) ? that.closeHeight * i:(that.closeHeight * (i - 1 ) + that.showHeight);
5 if (i == index){
6 that.span[i].style.display = " none " ;
7 } else {
8 that.span[i].style.display = " inline " ;
9 }
10 })
11 clearTimeout( this .handler);
12 that.run();
13 },
2 var that = this ;
3 this .each( function (i){
4 this .top = (i <= index) ? that.closeHeight * i:(that.closeHeight * (i - 1 ) + that.showHeight);
5 if (i == index){
6 that.span[i].style.display = " none " ;
7 } else {
8 that.span[i].style.display = " inline " ;
9 }
10 })
11 clearTimeout( this .handler);
12 that.run();
13 },
在set()中调用run(),根据当前位置与目标位置的差值,得出图片该向上移动还是向下移动,并设置定时器,使图片根据速度设置每次移动一定距离,形成动画;
1
run:
function
(){
2 var finish = true ,
3 that = this ;
4 this .each( function (){
5 var currentheight = parseInt( this .style.top, 10 );
6 if ( this .top - currentheight === 0 ) return ;
7 var step = ( this .top - currentheight > 0 ) ? that.speed: - that.speed;
8 if (Math.abs( this .top - currentheight) < that.speed) step = this .top - currentheight;
9 this .style.top = currentheight + step + " px " ;
10 finish = false ;
11 })
12 if ( ! finish){
13 that.handle = setTimeout( function (){
14 that.run();
15 }, 40 );
16 }
17 }
2 var finish = true ,
3 that = this ;
4 this .each( function (){
5 var currentheight = parseInt( this .style.top, 10 );
6 if ( this .top - currentheight === 0 ) return ;
7 var step = ( this .top - currentheight > 0 ) ? that.speed: - that.speed;
8 if (Math.abs( this .top - currentheight) < that.speed) step = this .top - currentheight;
9 this .style.top = currentheight + step + " px " ;
10 finish = false ;
11 })
12 if ( ! finish){
13 that.handle = setTimeout( function (){
14 that.run();
15 }, 40 );
16 }
17 }
创建实例,传入适当参数,完成。
1
new
gallery(
"
idGlideView2
"
,
400
,
200
,
10
);
完整代码:
html:


1
<
div
id
="gallery"
>
2 < div >< img src ="images/1.jpg" alt ="1.jpg" title ="图1" /></ div >
3 < div >< img src ="images/2.jpg" alt ="2.jpg" title ="图2" /></ div >
4 < div >< img src ="images/3.jpg" alt ="3.jpg" title ="图3" /></ div >
5 < div >< img src ="images/4.jpg" alt ="4.jpg" title ="图4" /></ div >
6 < div >< img src ="images/5.jpg" alt ="5.jpg" title ="图5" /></ div >
7 </ div >
2 < div >< img src ="images/1.jpg" alt ="1.jpg" title ="图1" /></ div >
3 < div >< img src ="images/2.jpg" alt ="2.jpg" title ="图2" /></ div >
4 < div >< img src ="images/3.jpg" alt ="3.jpg" title ="图3" /></ div >
5 < div >< img src ="images/4.jpg" alt ="4.jpg" title ="图4" /></ div >
6 < div >< img src ="images/5.jpg" alt ="5.jpg" title ="图5" /></ div >
7 </ div >


1
#gallery
{
2 height : 400px ;
3 width : 300px ;
4 border : 1px solid #666 ;
5 }
6 #gallery div {
7 width : 300px ;
8 height : 200px ;
9 border-top : 1px solid #000 ;
10 }
2 height : 400px ;
3 width : 300px ;
4 border : 1px solid #666 ;
5 }
6 #gallery div {
7 width : 300px ;
8 height : 200px ;
9 border-top : 1px solid #000 ;
10 }
js:


1
function
addEventHandler(element,type,handler){
2 if (element.addEventListener){
3 element.addEventListener(type,handler, false );
4 } else if (element.attachEvent){
5 element.attachEvent( " on " + type,handler)
6 } else {
7 element[ " on " + type] = handler;
8 }
9 }
10
11 function gallery(){
12 this .init.apply( this ,arguments);
13 }
14
15 gallery.prototype = {
16 /* 实现图片折叠/展开效果
17 * id:包含图片容器id
18 * size:包含图片容器大小
19 * showSize:展开图片大小
20 * speed:折叠/展开速度,默认值为10
21 */
22 init: function (id,size,showSize,speed){
23 var container = document.getElementById(id),
24 that = this ;
25 container.style.height = size + " px " ;
26 container.style.overflow = " hidden " ;
27 container.style.position = " relative " ;
28 this .span = []; // 保存文本节点
29 this .speed = speed || 10 ;
30 this .showHeight = showSize;
31 this .div = container.getElementsByTagName( " div " );
32 var len = this .div.length;
33 this .closeHeight = (size - this .showHeight) / (len-1);
34 this .count = len;
35 this .handle = null ;
36 this .img = container.getElementsByTagName( " img " );
37 this .each( function (i){
38 if (i === 0 ){
39 this .top = 0 ; // 保存目标位置
40 } else {
41 this .top = that.showHeight + that.closeHeight * (i - 1 );
42 }
43 this .style.position = " absolute " ;
44 this .style.top = this .top + " px " ;
45 addEventHandler( this , " click " , function (){
46 that.set.call(that,i);
47 });
48 that.createTitle( this ,i);
49 });
50 this .span[ 0 ].style.display = " none " ;
51 },
52 createTitle: function (element,i){
53 var text = this .img[i].title ? this .img[i].title: " 点击展开 " ;
54 var span = document.createElement( " span " );
55 span.innerHTML = text;
56 element.appendChild(span);
57 span.style.position = " absolute " ;
58 span.style.top = " 5px " ;
59 span.style.right = " 5px " ;
60 span.style.border = " 1px dotted #333 "
61 this .span.push(span);
62 },
63 each: function (fn){
64 for ( var i = 0 ;i < this .count;i ++ )
65 fn.call( this .div[i],i);
66 },
67 set: function (index){
68 var that = this ;
69 this .each( function (i){
70 this .top = (i <= index) ? that.closeHeight * i:(that.closeHeight * (i - 1 ) + that.showHeight);
71 if (i == index){
72 that.span[i].style.display = " none " ;
73 } else {
74 that.span[i].style.display = " inline " ;
75 }
76 })
77 clearTimeout( this .handler);
78 that.run();
79 },
80 run: function (){
81 var finish = true ,
82 that = this ;
83 this .each( function (){
84 var currentheight = parseInt( this .style.top, 10 );
85 if ( this .top - currentheight === 0 ) return ;
86 var step = ( this .top - currentheight > 0 ) ? that.speed: - that.speed;
87 if (Math.abs( this .top - currentheight) < that.speed) step = this .top - currentheight;
88 this .style.top = currentheight + step + " px " ;
89 finish = false ;
90 })
91 if ( ! finish){
92 that.handle = setTimeout( function (){
93 that.run();
94 }, 40 );
95 }
96 }
97 }
98 new gallery( " gallery " , 400 , 200 , 10 );
2 if (element.addEventListener){
3 element.addEventListener(type,handler, false );
4 } else if (element.attachEvent){
5 element.attachEvent( " on " + type,handler)
6 } else {
7 element[ " on " + type] = handler;
8 }
9 }
10
11 function gallery(){
12 this .init.apply( this ,arguments);
13 }
14
15 gallery.prototype = {
16 /* 实现图片折叠/展开效果
17 * id:包含图片容器id
18 * size:包含图片容器大小
19 * showSize:展开图片大小
20 * speed:折叠/展开速度,默认值为10
21 */
22 init: function (id,size,showSize,speed){
23 var container = document.getElementById(id),
24 that = this ;
25 container.style.height = size + " px " ;
26 container.style.overflow = " hidden " ;
27 container.style.position = " relative " ;
28 this .span = []; // 保存文本节点
29 this .speed = speed || 10 ;
30 this .showHeight = showSize;
31 this .div = container.getElementsByTagName( " div " );
32 var len = this .div.length;
33 this .closeHeight = (size - this .showHeight) / (len-1);
34 this .count = len;
35 this .handle = null ;
36 this .img = container.getElementsByTagName( " img " );
37 this .each( function (i){
38 if (i === 0 ){
39 this .top = 0 ; // 保存目标位置
40 } else {
41 this .top = that.showHeight + that.closeHeight * (i - 1 );
42 }
43 this .style.position = " absolute " ;
44 this .style.top = this .top + " px " ;
45 addEventHandler( this , " click " , function (){
46 that.set.call(that,i);
47 });
48 that.createTitle( this ,i);
49 });
50 this .span[ 0 ].style.display = " none " ;
51 },
52 createTitle: function (element,i){
53 var text = this .img[i].title ? this .img[i].title: " 点击展开 " ;
54 var span = document.createElement( " span " );
55 span.innerHTML = text;
56 element.appendChild(span);
57 span.style.position = " absolute " ;
58 span.style.top = " 5px " ;
59 span.style.right = " 5px " ;
60 span.style.border = " 1px dotted #333 "
61 this .span.push(span);
62 },
63 each: function (fn){
64 for ( var i = 0 ;i < this .count;i ++ )
65 fn.call( this .div[i],i);
66 },
67 set: function (index){
68 var that = this ;
69 this .each( function (i){
70 this .top = (i <= index) ? that.closeHeight * i:(that.closeHeight * (i - 1 ) + that.showHeight);
71 if (i == index){
72 that.span[i].style.display = " none " ;
73 } else {
74 that.span[i].style.display = " inline " ;
75 }
76 })
77 clearTimeout( this .handler);
78 that.run();
79 },
80 run: function (){
81 var finish = true ,
82 that = this ;
83 this .each( function (){
84 var currentheight = parseInt( this .style.top, 10 );
85 if ( this .top - currentheight === 0 ) return ;
86 var step = ( this .top - currentheight > 0 ) ? that.speed: - that.speed;
87 if (Math.abs( this .top - currentheight) < that.speed) step = this .top - currentheight;
88 this .style.top = currentheight + step + " px " ;
89 finish = false ;
90 })
91 if ( ! finish){
92 that.handle = setTimeout( function (){
93 that.run();
94 }, 40 );
95 }
96 }
97 }
98 new gallery( " gallery " , 400 , 200 , 10 );