一.文件上传
1.1 Java Web的文件上传
public void uploadFile(HttpServletRequest request,
HttpServletResponse response) throws ServletException, IOException {
response.setContentType("text/html;charset=GBK");
request.setCharacterEncoding("GBK");
HttpSession session = request.getSession();
session.setAttribute("progressBar", 0); // 定义指定上传进度的Session变量
String error = "";
int maxSize = 50 * 1024 * 1024; // 单个上传文件大小的上限
DiskFileItemFactory factory = new DiskFileItemFactory(); // 基于磁盘文件项目创建一个工厂对象
ServletFileUpload upload = new ServletFileUpload(factory); // 创建一个新的文件上传对象
try {
List items = upload.parseRequest(request);// 解析上传请求
Iterator itr = items.iterator();// 枚举方法
while (itr.hasNext()) {
FileItem item = (FileItem) itr.next(); // 获取FileItem对象
if (!item.isFormField()) {// 判断是否为文件域
if (item.getName() != null && !item.getName().equals("")) {// 判断是否选择了文件
long upFileSize = item.getSize(); // 上传文件的大小
String fileName = item.getName(); // 获取文件名
// System.out.println("上传文件的大小:" + item.getSize());
if (upFileSize > maxSize) {
error = "您上传的文件太大,请选择不超过50M的文件";
break;
}
// 此时文件暂存在服务器的内存中
File tempFile = new File(fileName);// 构造临时对象
// String savePath=tempFile.getName();
// //返回上传文件在客户端的完整路径名称
// request.setAttribute("filename", savePath);
@SuppressWarnings("deprecation")
File file = new File(request.getRealPath("/upload"),
tempFile.getName()); // 获取根目录对应的真实物理路径
InputStream is = item.getInputStream();
int buffer = 1024; // 定义缓冲区的大小
int length = 0;
byte[] b = new byte[buffer];
double percent = 0;
FileOutputStream fos = new FileOutputStream(file);
while ((length = is.read(b)) != -1) {
percent += length / (double) upFileSize * 100D; // 计算上传文件的百分比
fos.write(b, 0, length); // 向文件输出流写读取的数据
session.setAttribute("progressBar", Math
.round(percent)); // 将上传百分比保存到Session中
}
fos.close();
Thread.sleep(1000); // 线程休眠1秒
} else {
error = "没有选择上传文件!";
}
}
}
} catch (Exception e) {
e.printStackTrace();
error = "上传文件出现错误:" + e.getMessage();
}
if (!"".equals(error)) {
request.setAttribute("error", error);
request.getRequestDispatcher("error.jsp")
.forward(request, response);
} else {
request.setAttribute("result", "文件上传成功!");
request.getRequestDispatcher("upFile_deal.jsp").forward(request,
response);
}
}
1.2 SpringMVC的文件上传
1.2.1 MultipartResolver
JavaWeb的文件上传过于繁琐了。
SpringMVC为文件上传提供了直接的支持,这种支持是通过即插即用的MultipartResolver实现的。Spring 用 Jakarta Commons FileUpload 技术实现了一个MultipartResolver实现类:CommonMultipartResolver。
SpringMVC上下文中默认没有装配MultipartResolver,因此默认情况下不能处理文件的上传工作,要想使用SpringMVC的文件上传功能,需要在上下文中配置MultipartResolver。
配置 MultipartResolver:defaultEncoding: 必须和用户 JSP 的 pageEncoding 属性一致,以便正确解析表单的内容。为了让 CommonsMultipartResovler 正确工作,必须先将 Jakarta Commons FileUpload 及 Jakarta Commons io 的类包添加到类路径下。
1.2.2 文件上传步骤
1.导入jar包 :
commons-fileupload-1.2.1.jar
commons-io-2.0.jar
2.文件上传表单准备 :
form action="${ctp }/upload" enctype="multipart/form-data" method="post">
3.在SpringMVC配置文件中,配置文件上传解析器
<!-- 配置文件上传解析器 -->
<bean class="org.springframework.web.multipart.commons.CommonsMultipartResolver" id="multipartResolver">
<property name="maxInMemorySize" value="#{1024*1024*20}"></property>
<!-- 设置编码 -->
<property name="defaultEncoding" value="utf-8"></property>
</bean>
4.文件上传请求处理: 在处理器方法上写一个@RequestParam("headerimg")MultipartFile file 封装了当前文件信息,可以直接保存
public String upload(@RequestParam(value="username",required=false)String username,
@RequestParam("headerimg")MultipartFile file,
Model model) {
//文件保存
file.transferTo(new File("G:\\haha\\"+file.getOriginalFilename()));
...
}
二.实验代码
2.1单文件上传代码
页面上传表单:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<%
pageContext.setAttribute("ctp",request.getContextPath());
%>
</head>
<body>
${msg }
<form action="${ctp }/upload" enctype="multipart/form-data" method="post">
用户图像:<input type="file" name="headerimg"/>
用户名:<input type="text" name="username"/>
<input type="submit"/>
</form>
</body>
</html>
配置文件:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<context:component-scan base-package="com.test"></context:component-scan>
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/pages/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
<mvc:default-servlet-handler/>
<mvc:annotation-driven ></mvc:annotation-driven>
<!-- 配置文件上传解析器 -->
<bean class="org.springframework.web.multipart.commons.CommonsMultipartResolver" id="multipartResolver">
<property name="maxInMemorySize" value="#{1024*1024*20}"></property>
<!-- 设置编码 -->
<property name="defaultEncoding" value="utf-8"></property>
</bean>
</beans>
处理器方法:
@RequestMapping("/upload")
public String upload(@RequestParam(value="username",required=false)String username,
@RequestParam("headerimg")MultipartFile file,
Model model) {
System.out.println("上传文件的信息");
System.out.println("文件项的姓名"+file.getName());
System.out.println("文件的姓名"+file.getOriginalFilename());
//文件保存
try {
file.transferTo(new File("G:\\haha\\"+file.getOriginalFilename()));
model.addAttribute("msg", "文件上传成功了!");
} catch (Exception e) {
// TODO Auto-generated catch block
model.addAttribute("msg", "文件上传成功了!"+e.getMessage());
}
return "forward:/index.jsp";
}
2.2 多文件上传:
页面表单:
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
<%
pageContext.setAttribute("ctp",request.getContextPath());
%>
</head>
<body>
<!--
1.文件上传
1)文件上传表单准备 :enctype="multipart/form-data"
2)导入jar包
commons-fileupload-1.2.1.jar
commons-io-2.0.jar
3)只要在SpringMVC配置文件中,配置文件上传解析器
4)文件上传请求处理
在处理器方法上写一个@RequestParam("headerimg")MultipartFile file 封装了当前文件信息,可以直接保存
-->
${msg }
<form action="${ctp }/upload" enctype="multipart/form-data" method="post">
用户图像:<input type="file" name="headerimg"/><br/>
用户图像:<input type="file" name="headerimg"/><br/>
用户图像:<input type="file" name="headerimg"/><br/>
用户图像:<input type="file" name="headerimg"/><br/>
用户名:<input type="text" name="username"/><br/>
<input type="submit"/>
</form>
</body>
</html>
处理器方法:
/*
* 测试多文件
*/
@RequestMapping("/upload")
public String upload(@RequestParam(value="username",required=false)String username,
@RequestParam("headerimg")MultipartFile[] file,
Model model) {
System.out.println("上传文件的信息");
for(MultipartFile multipartFile:file){
if(!multipartFile.isEmpty()){
try {
multipartFile.transferTo(new File("G:\\haha\\"+multipartFile.getOriginalFilename()));
model.addAttribute("msg", "文件上传成功了!");
} catch (Exception e) {
model.addAttribute("msg", "文件上传失败!"+e.getMessage());
}
}
}
return "forward:/index.jsp";
}
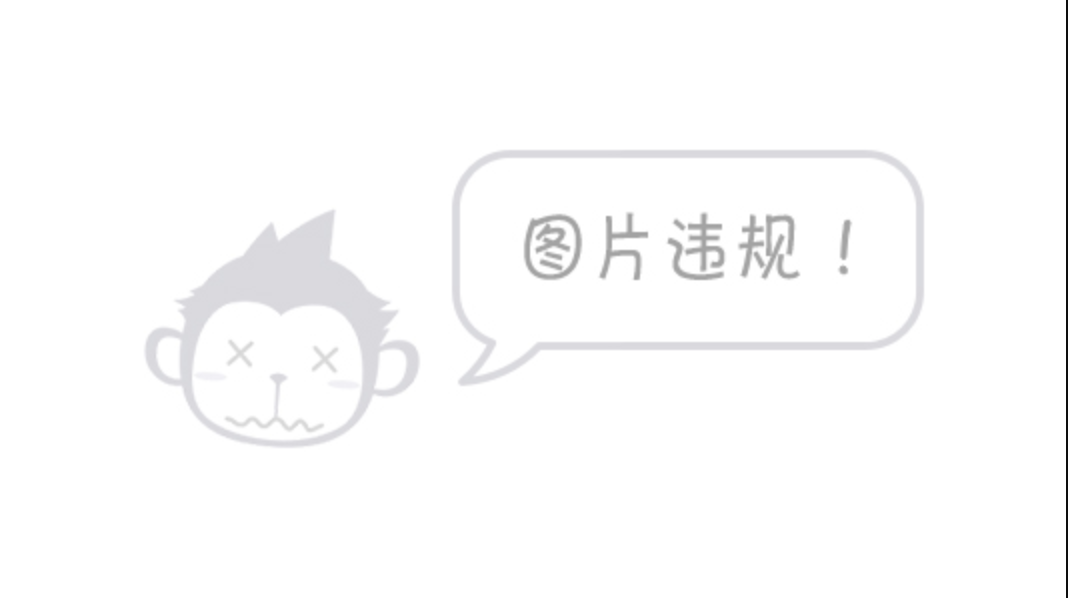
配置文件:
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-4.0.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd">
<context:component-scan base-package="com.test"></context:component-scan>
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="/WEB-INF/pages/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
<mvc:default-servlet-handler/>
<mvc:annotation-driven ></mvc:annotation-driven>
<!-- 配置文件上传解析器 -->
<bean class="org.springframework.web.multipart.commons.CommonsMultipartResolver" id="multipartResolver">
<property name="maxInMemorySize" value="#{1024*1024*20}"></property>
<!-- 设置编码 -->
<property name="defaultEncoding" value="utf-8"></property>
</bean>
</beans>