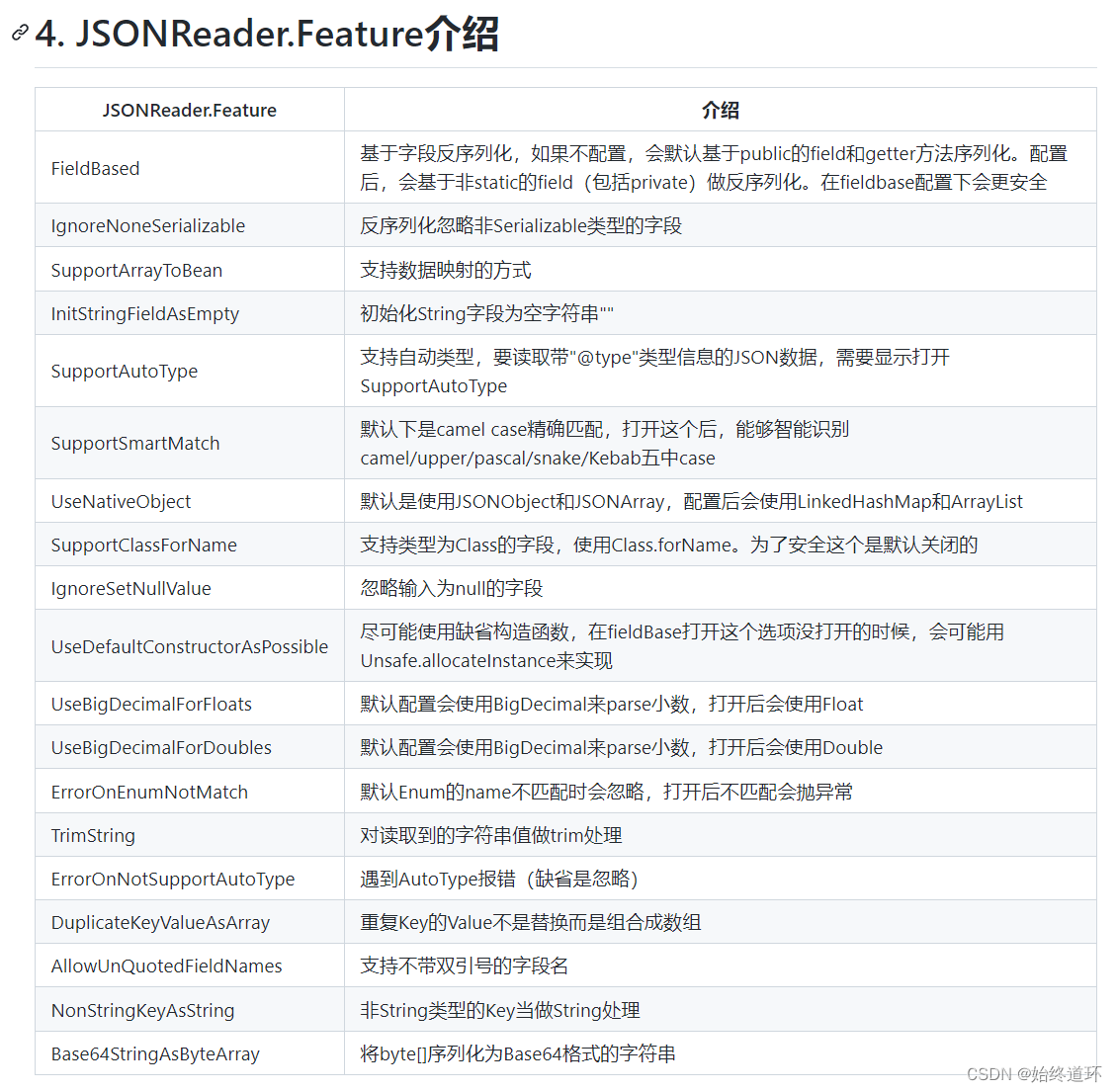
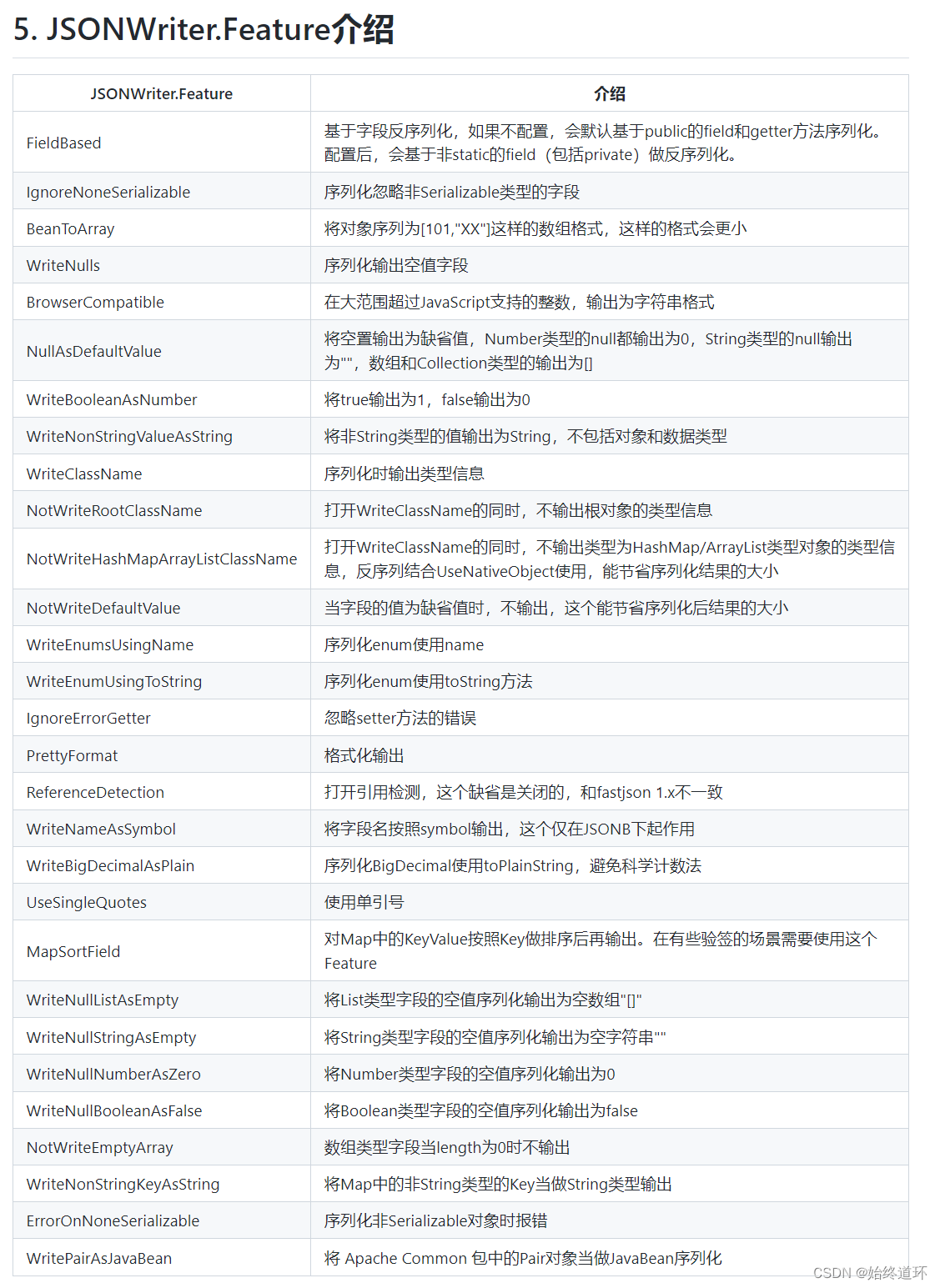
fastjson2 doc
https://github.com/alibaba/fastjson2/wiki/Features_cn
https://appdoc.app/artifact/com.alibaba.fastjson2/fastjson2/2.0.2/com/alibaba/fastjson2/JSONWriter.Feature.html
spring boot3 需要引入的依赖
<dependency>
<groupId>com.alibaba.fastjson2</groupId>
<artifactId>fastjson2-extension-spring6</artifactId>
<version>2.0.25</version>
</dependency>
fastjson2 FastJsonHttpMessageConverter 配置接口的序列化 接口结果的类型转换
package com.jianmu.config;
import com.alibaba.fastjson2.JSONReader;
import com.alibaba.fastjson2.JSONWriter;
import com.alibaba.fastjson2.support.config.FastJsonConfig;
import com.alibaba.fastjson2.support.spring.http.converter.FastJsonHttpMessageConverter;
import org.springframework.context.annotation.Configuration;
import org.springframework.http.converter.HttpMessageConverter;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
import java.nio.charset.StandardCharsets;
import java.util.List;
@Configuration
public class FastJson2Conf implements WebMvcConfigurer {
@Override
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
FastJsonHttpMessageConverter fastJsonHttpMessageConverter = new FastJsonHttpMessageConverter();
FastJsonConfig fastJsonConfig = new FastJsonConfig();
fastJsonConfig.setCharset(StandardCharsets.UTF_8);
fastJsonConfig.setReaderFeatures(
JSONReader.Feature.SupportSmartMatch,
JSONReader.Feature.FieldBased,
JSONReader.Feature.TrimString);
fastJsonConfig.setWriterFeatures(
JSONWriter.Feature.FieldBased,
JSONWriter.Feature.WriteLongAsString,
JSONWriter.Feature.WriteMapNullValue,
JSONWriter.Feature.WriteNullListAsEmpty,
JSONWriter.Feature.WriteNullStringAsEmpty,
JSONWriter.Feature.WriteNullBooleanAsFalse,
JSONWriter.Feature.PrettyFormat,
JSONWriter.Feature.NullAsDefaultValue);
fastJsonHttpMessageConverter.setFastJsonConfig(fastJsonConfig);
converters.add(0, fastJsonHttpMessageConverter);
}
}