以下题目是从菜鸟教程网站挑选出的。C 语言经典100例 | 菜鸟教程 (runoob.com)
第4题(判断这一天是一年的第几天)
#include <stdio.h>
void main()
{
int Year,Month,Day,Sum,LeapFlag;
printf("Input the Year,Month,Day(数据之间用回车或空格隔开)\n");
scanf("%d %d %d",&Year,&Month,&Day);
switch(Month-1)
{
case 0: Sum = 0;break;
case 1: Sum = 31;break;
case 2: Sum = 59;break;
case 3: Sum = 90;break;
case 4: Sum = 120;break;
case 5: Sum = 151;break;
case 6: Sum = 181;break;
case 7: Sum = 212;break;
case 8: Sum = 243;break;
case 9: Sum = 273;break;
case 10: Sum = 304;break;
case 11: Sum = 334;break;
default:printf("input error");break;
}
if((Year%400 == 0) || ((Year%4 == 0)&&(Year%100 != 0))) //判断闰年
{
LeapFlag = 1;
}
else
{
LeapFlag = 0;
}
Sum += Day;
if(LeapFlag == 1 && Month > 2) //如果是闰年且月份大于2,总天数加1
{
Sum += 1;
}
printf("The result is:%d\n",Sum);
}
第29题(将数分解)
我的做法,用递归函数。
#include<stdio.h>
int digui(int);
int main()
{
int a;
printf("input:\n");
scanf("%d",&a);
digui(a);
printf("\n");
}
int count = 1;
int digui(int a)
{
if(a/10!=0)
{
printf("%d",a%10);
count++;
digui(a/10);
}
else{
printf("%d\nthe size is:%\n",a%10,count);
}
}
可以处理9位数以内的数字。
第31题(需要注意getchar()的作用:吃掉换行符)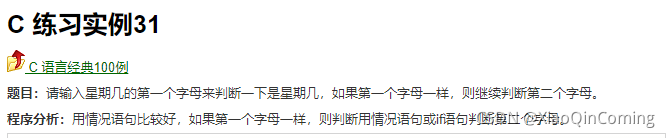
我的代码:
#include<stdio.h>
int main()
{
char *a,str[10];
a = str;
printf("Please input the Weekend:\n");
scanf("%s",a);
if( *a == 'M'){
printf("Monday\n");
}
else if( *a == 'S'){
if( *(a + 1) == 'u'){
printf("Sunday\n");
}
else if( *(a + 1) == 'a'){
printf("Saturday\n");
}
}
//此处省略其他周
else{
printf("error\n");
}
}
第32题(注意给字符数组先定义后赋值 和 直接初始化是不同的。)
由于此题给的答案只针对固定数组,本人的改进代码如下,可针对任意手动输入的数组(只随机测试几组):
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
char *delete(char c, char *a);
int main()
{
char *a,str[100],c;
a = str;
printf("input the string:\n");
scanf("%s",a);
getchar();
printf("input the delete char:\n");
scanf("%c",&c);
printf("%s\n",delete(c,a));
return 0;
}
char *delete(char c, char *a)
{
char *b = a;
if('\0' == c)
return a;
char str1[100] = {0};
int i,j,k = strlen(a);
for(i=0,j=0; j < k; a++,j++)
{
if(*a != c){
str1[i] = *a;
i++;
}
}
strcpy(b,str1);
return b;
}
此题需要注意的细节:
传送门:赋值给数组_理解字符串赋值给字符指针和字符数组的不同点_艾格吃饱了的博客-CSDN博客
第33题(质数判断)
我的做法,通过判断除数是否增加到 sqrt(被除数),即可。
#include<stdio.h>
#include<math.h>
int main()
{
int a,i;
scanf("%d",&a);
int limit = sqrt((double)a);
if(a <= 1)
printf("not\n");
else if(a == 2)
printf("Yes\n");
else if(a % 2 == 0)
printf("Not\n");
else {
for( i = 3; i <= limit ; i+=2)
{
if(a % i == 0){
printf("Not\n");
break;
}
}
if( i > limit)
printf("Yes\n");
}
}
我的分析:一个数可以拆成俩个因数相乘,可以看成是一个小数x大数。
例如:48
2x24
3x16
4x12
6x8
倘若我们要找48的因数,将小数从2递增 看有哪些数能否被整除,而当小数判断到6的时候就不需要再判断了,因为再增加就成了大数8,12,16...也可以看出在判断小数的时候 所有的大数也同时筛选完了,而所有的小数又都<=sqrt(被除数),所以判断范围就是<=sqrt(被除数)。
第55题(按位取反)
这题需要理解正数、负数的原码、反码、补码的概念。
原码,反码和补码概念可参考:二进制 原码 反码 补码_cinling123的专栏-CSDN博客
int 在dev编译器占4个字节(传送门:(38条消息) 一个字节多少位_weixin_30687051的博客-CSDN博客)
- 1字节(byte) = 8位(bit)
在16位的系统中(比如8086微机) 1字 (word)= 2字节(byte)= 16(bit)
在32位的系统中(比如win32) 1字(word)= 4字节(byte)=32(bit)
在64位的系统中(比如win64)1字(word)= 8字节(byte)=64(bit)
我的理解:对于这题,
a=234(0000 0000 0000 0000 0000 0000 1110 1010)
b=~a=(1111 1111 1111 1111 1111 1111 0001 0101)
取反后b是负数的补码形式。
要想知道b的十进制形式的值,就得转为十进制的原码,(负数的原码转补码、补码转原码的规则):
(1)负数原码转补码的过程:原码除符号位外 取反 +1。
(2)负数补码转原码的过程:(补码-1)除符号位外 再取反。
则求b的原码形式为:1000 0000 0000 0000 0000 0000 1110 1011,最左边那个1是符号位,所以换算成十进制为-235。
要想知道b的十六进制形式的值,直接将负数的补码形式换算(四个1相当于1个f):ffff ff15。
随机拿另外一个数验证,a=126(0000 0000 0000 0000 0000 0000 0111 1110)
b=~a=(1111 1111 1111 1111 1111 1111 1000 0001)(负数,补码形式)
要得出b的十进制值:b(将补码转原码再换成算十进制)=(1000 0000 0000 0000 0000 0000 0111 1111)= -127。
要得出b的十六进制值:b(将补码直接换算)=ffff ff81。
第32、35、66题(记住指针传递的书写形式)
我的代码:
#include<stdio.h>
//#include<stdlib.h>
#include<string.h>
void reverse(char *);
int main()
{
char s[]="www.i love u.com";
printf("%s\n",s);
reverse(s);
printf("%s\n",s);
return 0;
}
void reverse(char *s)
{
char c;
int i , len = strlen(s), len_half = strlen(s) / 2;
for(i = 0; i < len_half; i++)
{
c = *(s + i);
*(s + i) = *(s + len - i - 1);
*(s + len - i - 1) = c;
}
}
我的代码:
#include<stdio.h>
#include<string.h>
char *delete(char *,char );
int main()
{
char *str,str1[100],charone;
printf("Input the string:\n");
str = str1;
scanf("%s",str);
getchar();
printf("Input the need be delete char£º\n");
scanf("%c",&charone);
printf("The result:%s\n",delete(str,charone));
return 0;
}
char *delete(char *str, char charset)
{
int i ,temp[256];
if('\0' == charset)
return str;
int len = strlen(str);
for(i = 0; i < 256; i++)
temp[i] = 0;
temp[charset] = 1;
int current = 0;
for(i = 0; i < len; i++)
{
if(!temp[str[i]])
str[current++] = str[i];
}
str[current] = '\0';
return str;
}
第68、69(独立训练)
我的代码:
#include<stdio.h>
#include<string.h>
void move_arr(int arr[],int offset,int number);
void print_arr(int arr[],int number);
int main()
{
int number,offset,arr[20];
printf("Input the number of digits:\n");
scanf("%d",&number);
int i;
printf("Input the digits:\n");
for(i = 0; i < number; i++)
scanf("%d",&arr[i]);
printf("Input the offset:\n");
scanf("%d",&offset);
printf("Before:\n");
print_arr(arr,number);
move_arr(arr,offset,number);
printf("After:\n");
print_arr(arr,number);
return 0;
}
void print_arr(int arr[],int number)
{
int i;
for(i = 0; i < number; i++)
printf("%d ",arr[i]);
printf("\n");
}
void move_arr(int arr[],int offset,int number)
{
int *p, *last = arr + number;
while(offset--)
{
for(p = last; p != arr; p--){
*p = *(p-1);
}
arr[0] = *last;
}
}
第71、72、74题(有关结构体的独立训练)
相对比可发现,用typedef定义出来的 Stu 等价于struct student。
我的代码:
#include<stdio.h>
#include<stdlib.h>
typedef struct{
char name[20];
char sex;
int age;
}Stu;
void input(Stu *);
void output(Stu *);
int main()
{
Stu student[5];
printf("Input the student information\n");
input(student);
printf("Output the student information:\n");
output(student);
return 0;
}
void input(Stu *student)
{
int i;
for(i = 0; i < 5; i++)
{
//注意性别定义为单个字符,若定位为数组则去掉"&"
//注意下行%s %c %d之间需要有空格
scanf("%s %c %d",student[i].name,&(student[i].sex),&(student[i].age));
}
}
void output(Stu *student)
{
int i;
for(i = 0; i < 5; i++)
{
printf("%s %c %d\n",student[i].name,student[i].sex,student[i].age);
}
}
第二种写法:
#include<stdio.h>
struct student{
char name[20];
char sex;
int age;
}stu[5];
void input(struct student *);
void output(struct student *);
int main()
{
printf("Input the information:\n");
input(stu);
printf("output the information:\n");
output(stu);
return 0;
}
void input(struct student *stu)
{
int i;
for(i = 0; i < 5; i++)
scanf("%s %c %d",stu[i].name,&stu[i].sex,&stu[i].age);
}
void output(struct student *stu)
{
int i;
for(i = 0; i < 5; i++)
printf("%s %c %d\n",stu[i].name,stu[i].sex,stu[i].age);
}
typedef 定义的*LinkList 使得 LinkList CreateList() 相当于:
struct LNode *CreateList();
我的代码:
#include<stdio.h>
#include<malloc.h>
#include<stdlib.h>
typedef struct LNode{
int Data;
struct LNode *next;
}LNode1,*List;
List CreateList(int );
void PrintList(List );
int main()
{
List Head = NULL;
int n;
printf("input the n:");
scanf("%d",&n);
Head = CreateList(n);
PrintList(Head);
return 0;
}
List CreateList(int n)
{
List q,p,L;
int i;
L = malloc(sizeof(LNode1));
if(!L)
return NULL;
q = L;
L->next = NULL;
for(i = 1; i <= n; i++)
{
p = malloc(sizeof(LNode1));
if(!p)
return NULL;
printf("请输入第%d个元素的值:",i);
scanf("%d",&(p->Data));
p->next = NULL;
q->next = p;
q = p;
}
return L;
}
void PrintList(List Head)
{
List p = Head->next;
while(p != NULL)
{
printf("The number is:%d\n",p->Data);
p = p->next;
}
}
typedef struct list node
等价于
struct list{
....
}node;
typedef node *list :表示把list定义为与node同类型的结构体,不同之处:list是结构体指针。
所以 link delete_node(link pointer,link tmp) 可等价于
struct list *delete_node(struct list *pointer,struct list *tmp)
第77题(注意三者的区别)
const char *ptr;
char const *ptr;
char * const ptr;
传送门:const char * 、char const *、 char * const 三者的区别_SilentOB的博客-CSDN博客
第79题(fgets函数用法)
fgets(line,(sizeof line / sizeof line[0]),stdin);
将stdin,也就是键盘输入的内容读取到line变量,缓冲区数组长度是(sizeof line / sizeof line[0]),也就是整个数组的字节数除以一个字符的字节数。
第87题(关于结构体做参数传递)
把一个完整的结构体变量作为参数传递,要将全部成员值一个一个传递,费时间又费空间,开销大。如果结构体类型中的成员很多,或有一些成员是数组,则程序运行效率会大大降低。在这种情况下,用指针做函数参数比较好,能提高运行效率。