Springboot连接远程服务器执行linux命令
1.在pom文件中添加依赖,我这里用的是com.jcraft,还有一个是ch.ethz.ganymed,可以百度自行研究
<dependency>
<groupId>com.jcraft</groupId>
<artifactId>jsch</artifactId>
<version>0.1.54</version>
</dependency>
2.添加工具类
@Component
public class SSHClient {
private String host = "localhost";
private Integer port = 22;
private String username = "";
private String password = "";
private String pubKeyPath = "C:/Users/zhangyanhe/.ssh/id_rsa";
private JSch jsch = null;
private Session session = null;
private Channel channel = null;
private final Integer SESSION_TIMEOUT = 60000;
private final Integer CHANNEL_TIMEOUT = 60000;
private final Integer CYCLE_TIME = 100;
public SSHClient() throws JSchException {
jsch = new JSch();
jsch.addIdentity(pubKeyPath);
}
public SSHClient setHost(String host) {
this.host = host;
return this;
}
public SSHClient setPort(Integer port) {
this.port = port;
return this;
}
public SSHClient setUsername(String username) {
this.username = username;
return this;
}
public SSHClient setPassword(String password) {
this.password = password;
return this;
}
public Session getSession() {
return this.session;
}
public Channel getChannel() {
return this.channel;
}
public void login(String username,String host,Integer port) {
this.username = username;
this.host = host;
this.port = port;
try {
if (null == session) {
session = jsch.getSession(this.username, this.host, this.port);
session.setConfig("StrictHostKeyChecking", "no");
}
session.connect(SESSION_TIMEOUT);
} catch (JSchException e) {
this.logout();
}
}
public void login() {
this.login(this.username,this.host,this.port);
}
public void logout() {
this.session.disconnect();
}
public synchronized String sendCmd(String command) {
if (!session.isConnected()) {
this.login();
}
if (this.session == null)
return null;
Channel channel = null;
BufferedReader bufferedReader = null;
String resp = "";
try {
channel = this.session.openChannel("exec");
((ChannelExec) channel).setCommand(command);
channel.setInputStream(null);
((ChannelExec) channel).setErrStream(System.err);
channel.connect();
bufferedReader = new BufferedReader(new InputStreamReader(channel.getInputStream()));
String line = null;
while ((line = bufferedReader.readLine()) != null) {
resp += line + "\n";
}
if (resp != null && !resp.equals("")) {
resp = resp.substring(0, resp.length() - 1);
}
} catch (JSchException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (bufferedReader != null) {
try {
bufferedReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (channel != null) {
channel.disconnect();
}
}
return resp;
}
public static void main(String[] args) throws JSchException {
SSHClient sshClient=new SSHClient();
sshClient.setHost("192.168.31.250").setPort(22).setUsername("root");
sshClient.login();
String commond5 = "sar 1 1";
String ret5 = sshClient.sendCmd(commond5).trim();
ret5=ret5.replaceAll("\n",",");
String[] split5 = ret5.split(",");
if(split5.length>0){
ret5=split5[split5.length-1];
ret5 = ret5.replaceAll("[平均时间:|Average:|all]", "").trim();
ret5 = ret5.replaceAll("\\s{1,}", ",");
String[] split6 = ret5.split(",");
System.out.println(Arrays.toString(split6));
}
System.out.println("******************************");
System.out.println(ret5);
System.out.println("******************************");
sshClient.logout();
}
3.在controller中添加接口
我是在类初始话的时候就将连接建立好了,不然每次都要建立连接,然后关闭连接,耗费资源,因为我的接口是要定时的获取信息。如果发现有服务挂了,再次连接就可以了。
@Scope("session")
@RestController
@RequestMapping("/tool/ssh")
public class SSHController extends BaseController {
@Autowired
private ISysOuturlConfigService sysOuturlConfigService;
private static Map<String, SSHClient> sshPool;
@PostConstruct
public void init() {
try {
System.out.println("初始化ssh连接池");
sshPool = new HashMap<>();
List<SysOuturlConfig> sysOuturlConfigs = sysOuturlConfigService.selectSysOuturlConfigList(null);
for (SysOuturlConfig sysOuturlConfig : sysOuturlConfigs) {
SSHClient sshClient = new SSHClient();
sshClient.setHost(sysOuturlConfig.getServiceIp()).setPort(22).setUsername(sysOuturlConfig.getRootUser());
sshClient.login();
sshPool.put(sysOuturlConfig.getCode(), sshClient);
}
} catch (JSchException e) {
e.printStackTrace();
}
}
@GetMapping("/dynamicInfo/{code}")
public AjaxResult dynamicInfo(@PathVariable("code") String code) throws JSchException {
Map<String, Object> map = new HashMap<>();
SSHClient sshClient = sshPool.get(code);
if (!sshClient.getSession().isConnected()) {
sshClient.login();
sshPool.put(code,sshClient);
}
String commond5 = "sar 1 1";
String ret5 = sshClient.sendCmd(commond5).trim();
ret5=ret5.replaceAll("\n",",");
String[] split5 = ret5.split(",");
if (split5.length > 0) {
ret5=split5[split5.length-1];
ret5 = ret5.replaceAll("[平均时间:|Average:|all]", "").trim();
ret5 = ret5.replaceAll("\\s{1,}", ",");
String[] split6 = ret5.split(",");
map.put("cpuUserUsage", split6[0]);
map.put("cpuSysUsage", split6[2]);
map.put("cpuIdleUsage", split6[5]);
} else {
map.put("cpuUserUsage", 0);
map.put("cpuSysUsage", 0);
map.put("cpuIdleUsage", 0);
}
double memtotal = 1.0;
double memfree = 0.0;
String commond6 = "cat /proc/meminfo | awk '$1 ~/MemTotal/' |awk '{print $2}'";
String ret6 = sshClient.sendCmd(commond6).trim();
if (StringUtils.isNotEmpty(ret6)) {
memtotal = Double.valueOf(ret6);
}
String commond7 = "cat /proc/meminfo | awk '$1 ~/MemFree/' |awk '{print $2}'";
String ret7 = sshClient.sendCmd(commond7).trim();
if (StringUtils.isNotEmpty(ret7)) {
memfree = Double.valueOf(ret7);
}
if (memtotal > 0 && memfree > 0 && (memtotal - memfree) > 0) {
map.put("memUsage", (memtotal - memfree) / memtotal * 100);
} else {
map.put("memUsage", 0.0);
}
SysOuturlConfig sysOuturlConfig = sysOuturlConfigService.selectSysOuturlConfigByCode(code);
map.put("appName", sysOuturlConfig.getName());
map.put("appUrl", sysOuturlConfig.getUrl());
map.put("appIcon", sysOuturlConfig.getIcon());
List<Map<String, Object>> list = new ArrayList<>();
String retc = sshClient.sendCmd("ps aux|grep" + " " + code + " " + "|grep -v \"grep\"|awk '{print $2,$9,$10,$8}'");
if (StringUtils.isNotEmpty(retc)) {
retc = retc.replaceAll("\n", ",");
String[] split = retc.split(",");
for (String s : split) {
Map<String, Object> mp = new HashMap<>();
String[] sp = s.split("\\s{1,1}");
mp.put("appPid", sp[0]);
mp.put("appStartTime", sp[1] + " " + sp[2]);
mp.put("appStatus", sp[3]);
list.add(mp);
}
}
map.put("appProcess", list);
double appCpuUsage = 0.0;
String commond8 = "ps aux|grep" + " " + code + " " + "|grep -v \"grep\"|awk '{print $3}'";
String ret8 = sshClient.sendCmd(commond8);
ret8 = ret8.replaceAll("\n", ",");
ret8 = ret8 + "0";
String[] split1 = ret8.split(",");
for (String s : split1) {
appCpuUsage += Double.valueOf(s);
}
map.put("appCpuUsage", appCpuUsage);
double memoryUsage = 0.0;
String commond9 = "ps aux|grep" + " " + code + " " + "|grep -v \"grep\"|awk '{print $4}'";
String ret9 = sshClient.sendCmd(commond9);
ret9 = ret9.replaceAll("\n", ",");
ret9 = ret9 + "0";
String[] split9 = ret9.split(",");
for (String s : split9) {
memoryUsage += Double.valueOf(s);
}
map.put("appMemoryUsage", memoryUsage);
return AjaxResult.success(map);
}
@GetMapping("/sysInfo/{code}")
public AjaxResult sysInfo(@PathVariable("code") String code) throws JSchException {
Map<String, Object> map = new HashMap<>();
SSHClient sshClient = sshPool.get(code);
if (!sshClient.getSession().isConnected()) {
sshClient.login();
}
String commond1 = "hostname";
String ret1 = sshClient.sendCmd(commond1).replaceAll("[\\s*\\t\\n\\r]", "");
map.put("hostName", ret1);
String commond2 = "cat /etc/redhat-release";
String ret2 = sshClient.sendCmd(commond2).replaceAll("[\\s*\\t\\n\\r]", "");
map.put("operSystem", ret2);
String commond3 = "ifconfig |head -2 |grep inet |awk '{print $2}'";
String ret3 = sshClient.sendCmd(commond3).replaceAll("[\\s*\\t\\n\\r]", "");
map.put("serviceIp", ret3);
String commond4 = "arch";
String ret4 = sshClient.sendCmd(commond4).replaceAll("[\\s*\\t\\n\\r]", "");
map.put("systemArch", ret4);
String cmdPhysicalNum = "cat /proc/cpuinfo |grep \"physical id\"|sort|uniq|wc -l";
String physicalNum = sshClient.sendCmd(cmdPhysicalNum).replaceAll("[\\s*\\t\\n\\r]", "");
map.put("physicalNum", physicalNum);
String cmdCoresNUm = "cat /proc/cpuinfo |grep \"cpu cores\"|wc -l";
String coresNUm = sshClient.sendCmd(cmdCoresNUm).replaceAll("[\\s*\\t\\n\\r]", "");
map.put("coresNUm", coresNUm);
return AjaxResult.success(map);
}
}
4.前端展示
下面是我弄的前端页面。毕竟不是搞前端的,凑合看吧!!!
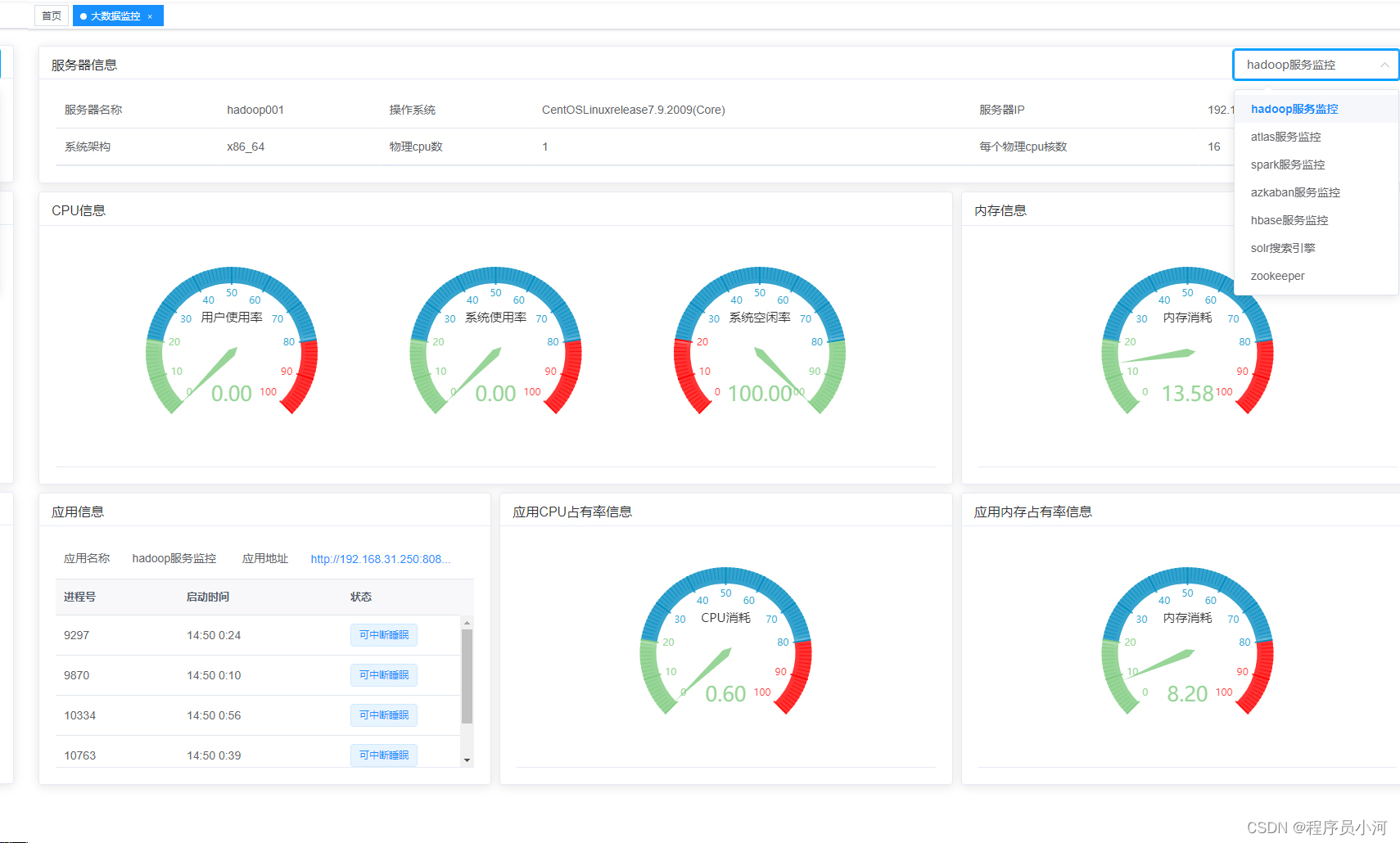
代码写的不好,还请各位大神批评指正!!!