同样的,在
按键驱动程序(中断方式)
的基础之上添加互斥阻塞机制。当设备被一个程序打开时,存在被另一个程序打开的可能,
如果两个或多个程序同时对设备文件进行写操作,或者读设备文件都会出现同步的问题
。本程序是通过
获取信号量“锁住”打开程序,直到释放了信号量,另外的程序才能打开程序
。(另外的方法还有用
原子操作
维护设备被打开的计数等)。获得信号量有可以用int
down_trylock
(struct semaphore *sem)尝试获得信号量,立即返回。void
down
(struct semaphore *sem)获得信号量,
获得不到就休眠等待直到可以获得信号量
。可以在驱动程序的
open函数中调用获得信号量
,
根据文件打开标志O_NONBLOCK是否存在来决定是否是非阻塞或阻塞方式打开
,如果是
非阻塞
则应用
down_trylock()
获得信号量,
一旦获取不到立马返回错误值
,
否则应用down()
或其它如int
down_interruptible
(struct semaphore *sem)等。
在设备文件被关闭时
,
即在close函数中应该释放掉信号量,以便其它程序使用
,用到的函数是
void up
(struct semaphore *sem)。
详细驱动程序如下:
点击(此处)折叠或打开
- #include <linux/module.h>
- #include <linux/kernel.h>
- #include <linux/fs.h>
- #include <linux/init.h>
- #include <linux/delay.h>
- #include <linux/irq.h>
- #include <asm/uaccess.h>
- #include <linux/cdev.h>
- #include <linux/interrupt.h>
- #include <linux/device.h>
- #include <linux/sched.h>
- #include <linux/gpio.h>
-
- #define SIXDEV MKDEV(250, 0)
- struct cdev sixdrv_cdev;
-
- static struct class *sixdrv_class;
-
- struct button {
- int irq;
- char *name;
- int pin;
- int val;
- };
-
- static volatile int pressed = 0;
- static unsigned char key_val;
-
- struct fasync_struct *button_async;
-
- DECLARE_WAIT_QUEUE_HEAD(button_wqh);
-
- /* 六个按键的相关定义整合到结构体 */
- static struct button buttons[6] = {
- {IRQ_EINT8, "K1", S3C2410_GPG(0), 0x1},
- {IRQ_EINT11, "K2", S3C2410_GPG(3), 0x2},
- {IRQ_EINT13, "K3", S3C2410_GPG(5), 0x3},
- {IRQ_EINT14, "K4", S3C2410_GPG(6), 0x4},
- {IRQ_EINT15, "K5", S3C2410_GPG(7), 0x5},
- {IRQ_EINT19, "K6", S3C2410_GPG(11),0x6},
- };
-
-
- static DECLARE_MUTEX(button_lock);
-
- /* 中断处理函数 */
- static irqreturn_t sixdrv_intr(int irq, void *data)
- {
- struct button *buttonp;
- int val;
-
- buttonp = (struct button*)data;
-
- val = s3c2410_gpio_getpin(buttonp->pin);
-
-
- if (!val) {/* 按下按键*/
- key_val = buttonp->val;
- } else { /* 释放按键*/
- key_val = buttonp->val | 0x10; //将第4位置1,做标记
- }
-
- pressed = 1; //此处改变按下标志,以使队列不继续睡眠
- wake_up_interruptible(&button_wqh);
-
- return IRQ_RETVAL(IRQ_HANDLED);
- }
-
- static int sixdrv_open(struct inode * inode, struct file * file)
- {
- int i=6;
- if (file->f_flags & O_NONBLOCK) {
- if(down_trylock(&button_lock))
- return -EBUSY;
- } else {
- down(&button_lock);
- }
- while(i--){
- request_irq(buttons[i].irq, &sixdrv_intr, IRQ_TYPE_EDGE_BOTH,
- buttons[i].name, &buttons[i]);
- }
- return 0;
-
- }
-
- static ssize_t sixdrv_read(struct file *file, char __user *user, size_t size,loff_t*o)
- {
- int sz = sizeof(key_val) ;
-
- if (sz != size) {
- return -EINVAL;
- }
-
- if (file->f_flags & O_NONBLOCK)
- {
- if (!pressed)
- return -EAGAIN;
- }
- else
- {
- /* 如果没有按键动作, 休眠 */
- wait_event_interruptible(button_wqh, pressed);
- }
- copy_to_user(user, &key_val, sz);
-
- /* 重新清除按下标志 */
- pressed = 0;
-
- return sz;
- }
-
- static int sixdrv_close(struct inode *inode, struct file *file)
- {
- int i=6;
-
- while(i--) {
- free_irq(buttons[i].irq, &buttons[i]);
- }
- up(&button_lock);
- return 0;
- }
-
- static struct file_operations sixdrv_ops = {
- .owner = THIS_MODULE,
- .open = sixdrv_open,
- .read = sixdrv_read,
- .release = sixdrv_close,
- };
- static int sixdrv_init(void)
- {
- int ret;
- int devt = SIXDEV;
-
- ret = register_chrdev_region(devt, 1, "sixdrv");
- if (ret) {
- printk(KERN_ERR "Unable to register minors for sixdrv\n");
- goto fail;
- }
- sixdrv_class = class_create(THIS_MODULE, "sixdrv_class");
- if (IS_ERR(sixdrv_class)) {
- printk(KERN_ERR "can't register device class\n");
- return PTR_ERR(sixdrv_class);
- }
- device_create(sixdrv_class, NULL, devt, NULL, "buttons");
- cdev_init(&sixdrv_cdev, &sixdrv_ops);
- ret = cdev_add(&sixdrv_cdev, devt, 1);
- if (ret < 0)
- goto fail_cdev;
- return 0;
-
- fail_cdev:
- class_unregister(sixdrv_class);
- device_destroy(sixdrv_class, devt);
- cdev_del(&sixdrv_cdev);
- fail:
- unregister_chrdev_region(devt, 1);
-
- return 0;
- }
-
- static void sixdrv_exit(void)
- {
- class_unregister(sixdrv_class);
- device_destroy(sixdrv_class, SIXDEV);
- cdev_del(&sixdrv_cdev);
- unregister_chrdev_region(SIXDEV, 1);
- }
-
- module_init(sixdrv_init);
- module_exit(sixdrv_exit);
- MODULE_LICENSE("GPL");
应用程序如下:
点击(此处)折叠或打开
- #include <sys/types.h>
- #include <sys/stat.h>
- #include <fcntl.h>
- #include <stdio.h>
- #include <poll.h>
- #include <signal.h>
-
- int main(int argc, char *argv[])
- {
- int fd, cnt=1;
- unsigned char val;
-
- fd = open("/dev/buttons", O_RDWR);
- //fd = open("/dev/buttons", O_RDWR|O_NONBLOCK);
- if (fd < 0)
- {
- printf("Can not open device\n");
- return -1;
- }
-
- while(1)
- {
- read(fd, &val, sizeof(unsigned char));
- printf("cnt:%d, val:0x%x\n", cnt++, val);
- }
-
- return 0;
- }
运行情况:
1.执行./six_app &命令后台运行应用程序时,用ps查看进程号为724的进程处于S状态,即休眠状态,在等待中断发生。
2.再次运行一个./six_app &,发现新的进程为726,处于D状态,即不可中断状态,在等待724释放信号量。
3.如果运行kill -9 726发现该进程是杀不死的,因为此时./six_app处于不可中断状态,即阻塞状态,阻塞状态虽然保护了文件同时不被好几个程序读写,但也会经常发生锁死状况,因为如果进程724的./six_app如果一直不释放锁或者信号量的话,那么进程726的./six_app将一直阻塞下去。
4.当用kill -9 724杀死724进程时,726处于S状态,等待中断发生。
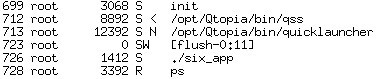
应用程序说明:上面的运行程序基于阻塞方式打开open("/dev/buttons", O_RDWR);
当调用非阻塞方式打开时open("/dev/buttons", O_RDWR|O_NONBLOCK);获得信号量并且在read后,if (!pressed)如果没有按键按下,会立即返回,所以会在while(1)里面打印出很多信息。这个实验不上图。
更多同步互斥阻塞知识请在互联网或者相关书籍中搜索,定会找到更详细内容。