2.如何把地图的key引入项目
在public目录下找到index.html
<script src="https://webapi.luokuang.com/maps?ak=您申请的ak"></script>
3.添加地图容器
<div id="map"></div>
4.初始化数据
data() {
return {
map: null,
inforWindow: null,
lnglats: [
{
id: 1,
name: "动物园",
position: [116.33731593367531, 39.941387955338996],
},
{
id: 2,
name: "玉渊谭公园",
position: [116.31959602683907, 39.916069734293075],
},
{
id: 3,
name: "北京天安门",
position: [116.3984929272525, 39.90787656038206],
},
{
id: 4,
name: "日坛公园",
position: [116.44389016057424, 39.914487034587154],
},
],
};
},
5.添加标记到地图
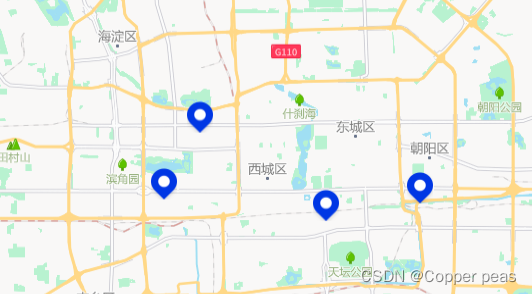
addMarkers() {
let position = new LKMap.LngLat(116.39751541617153, 39.90768542687843);
let markers = [];
this.map = new LKMap.Map("map", {
center: position,
zoom: 10.5,
});
for (let i = 0; i < this.lnglats.length; i++) {
markers[i] = new LKMap.Marker({
map: this.map,
position: new LKMap.LngLat(
this.lnglats[i].position[0],
this.lnglats[i].position[1]
),
anchor: "top",
extData: {
id: i,
name: this.lnglats[i].name,
},
});
markers[i].on("click", this.markerClick);
}
},
6.点击弹窗事件
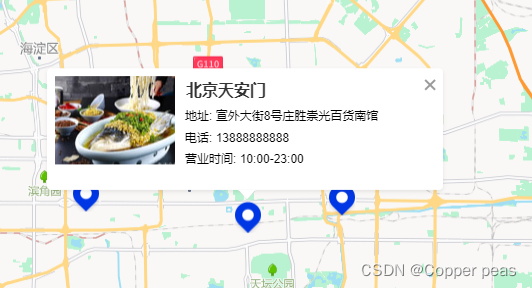
markerClick(e) {
const extData = e.layer.getExtData();
const title = "";
const content = [
`<img src='https://lkimgyt.luokuang.com/1587364867424.jpg'><span>${extData.name}</span>`,
`<label>地址: 宣外大街8号庄胜崇光百货南馆</label>`,
`<label>电话: 13888888888</label>`,
`<label>营业时间: 10:00-23:00</label>`
].join("<br/>");
this.inforWindow = new LKMap.InforWindow({
anchor: "bottom",
className: "customClassName",
content: this.createInfoWindow(title, content),
isCustom: true,
offset: new LKMap.Pixel(0, -16),
});
const markerPosition = e.layer.getPosition();
this.inforWindow.open(this.map, markerPosition);
},
createInfoWindow(title, content) {
const info = document.createElement("div");
info.className = "custom-info content-window-card";
const top = document.createElement("div");
const titleD = document.createElement("div");
const closeX = document.createElement("img");
top.className = "info-top";
titleD.innerHTML = title;
closeX.src = "https://lkimgyt.luokuang.com/1587366541654.png";
closeX.onclick = this.closeInfoWindow.bind(this);
top.appendChild(titleD);
top.appendChild(closeX);
info.appendChild(top);
const middle = document.createElement("div");
middle.className = "info-middle";
middle.style.backgroundColor = "white";
middle.innerHTML = content;
info.appendChild(middle);
const bottom = document.createElement("div");
bottom.className = "info-bottom";
bottom.style.position = "relative";
bottom.style.top = "-3px";
bottom.style.margin = "0 auto";
const sharp = document.createElement("img");
sharp.src = "https://lkimgyt.luokuang.com/1587367651134.png";
bottom.appendChild(sharp);
info.appendChild(bottom);
return info;
},
closeInfoWindow() {
this.map.clearInfoWindow();
},
7.弹窗相关样式
<style>
#map {
width: 100%;
height: 100%;
}
.content-window-card {
position: relative;
bottom: 0;
left: 0;
width: 400px;
height: 120px;
padding: 0;
border: 0;
background: 0;
}
.content-window-card p {
height: 2rem;
}
div.info-top {
position: relative;
height: 0;
}
div.info-top div {
display: inline-block;
color: #333333;
font-size: 14px;
font-weight: bold;
line-height: 31px;
padding: 0 10px;
}
div.info-top img {
position: absolute;
top: 10px;
right: 10px;
transition-duration: 0.25s;
}
div.info-top img:hover {
box-shadow: 0px 0px 5px #000;
}
div.info-middle {
font-size: 12px;
padding: 8px;
line-height: 20px;
background: rgba(255, 255, 255, 1);
box-shadow: 0px 2px 4px 0px rgba(0, 0, 0, 0.08);
border-radius: 4px;
-webkit-border-radius: 4px;
width: 380px;
height: 108px;
}
div.info-middle span {
font-size: 16px;
font-weight: bold;
color: rgba(51, 51, 51, 1);
display: inline-block;
line-height: 30px;
}
div.info-middle label {
display: inline-block;
line-height: 22px;
padding-left: 4px;
margin-bottom: 0;
}
div.info-middle label.price {
color: rgba(186, 51, 48, 1);
}
div.info-middle a {
float: right;
color: #0037e4;
}
div.info-bottom {
height: 0px;
width: 100%;
clear: both;
text-align: center;
background: 0;
}
div.info-bottom img {
position: relative;
z-index: 104;
}
span {
margin-left: 5px;
font-size: 11px;
}
.info-middle img {
float: left;
margin-right: 6px;
}
</style>