//头尾相连的单链表
#include<iostream>
using namespace std;
typedef int DataType;
struct LinkNode
{
DataType _data;
LinkNode *_next;
LinkNode(const DataType &x)
:_data(x)
, _next(NULL)
{}
};
class sList
{
public:
sList()
:_head(NULL)
,_tail(NULL)
{}
~sList()
{
Destory();
}
sList(const sList &s) :_head(NULL), _tail(NULL)
{
LinkNode *begin = s._head;
do
{
this->PushBack(begin->_data);
begin = begin->_next;
} while (begin != s._head);
}
//优化
void Swap(sList &s)
{
swap(_head, s._head);
swap(_tail, s._tail);
}
sList &operator=(const sList &s)
{
if (this != &s)
{
this->Destory();
/*LinkNode *begin = s._head;
do
{
this->PushBack(begin->_data);
begin = begin->_next;
} while (begin != s._head);*/
sList tmp(s);
Swap(tmp);
}
return *this;
}
void PushBack(const DataType &x)
{
//1.没有节点(空链表)
if (_head == NULL)
{
_head = new LinkNode(x);
_tail = _head;
_tail->_next = _head;
return;
}
_tail->_next = new LinkNode(x);
_tail = _tail->_next;
_tail->_next = _head;
}
void PopBack()
{
if (_head == NULL)
{
return;
}
if (_head == _tail)
{
delete _head;
_head = NULL;
_tail = NULL;
return;
}
LinkNode *begin = _head;
while (begin->_next != _tail)
{
begin=begin->_next;
}
begin->_next = _head;
delete _tail;
_tail = begin;
}
void PushFront(const DataType &x)
{
if (_head == NULL)
{
_head = new LinkNode(x);
_tail = _head;
_tail->_next = _head;
return;
}
_tail->_next = new LinkNode(x);
_tail->_next->_next = _head;
_head=_tail->_next;
}
void PopFront()
{
if (_head == NULL)
{
return;
}
if (_head == _tail)
{
delete _head;
_head = NULL;
_tail = NULL;
return;
}
_tail->_next = _head->_next;
delete _head;
_head = _tail->_next;
}
LinkNode *Find(const DataType &x)
{
LinkNode *begin = _head;
//1.空链表
if (_head == NULL)
{
return NULL;
}
do
{
if (begin->_data == x)
{
return begin;
}
begin = begin->_next;
} while (begin != _head);
return NULL; //没找到该节点
}
//插到n后
void Insert(LinkNode *n,const DataType &x)
{
//1.空链表
if (_head == NULL)
{
_head = new LinkNode(x);
_tail = _head;
_tail->_next = _head;
}
//2.尾结点后,更新尾
else if (_tail == n)
{
_tail->_next = new LinkNode(x);
_tail = _tail->_next;
_tail->_next = _head;
}
//3.插入到链表间
else
{
LinkNode *tmp = n->_next;
n->_next = new LinkNode(x);
n->_next->_next = tmp;
}
}
//删除n结点
void Remove(LinkNode *n)
{
//1.空链表
if (_head == NULL)
{
return;
}
//2.删除的是头结点
else if (_head == n)
{
PopFront();
}
else
{
LinkNode *prev = _head;
while (prev->_next != n)//删除的是中间任意一个结点
{
prev = prev->_next;
}
prev->_next = n->_next;
if (n == _tail)
{
_tail = prev;//删除的是尾结点,更新尾
}
delete n;
}
}
//链表逆序
void Reverse()
{
if (_head == NULL || _head == _tail)
{
return;
}
LinkNode *begin = _head->_next;
LinkNode *NewHead = _head;
LinkNode *NewTail = _head;
NewHead->_next = NULL;
while (begin!=_head)
{
LinkNode *tmp = begin;
begin = begin->_next;
tmp->_next = NewHead;
NewHead = tmp;
}
_head = NewHead;
_tail= NewTail;
_tail->_next = _head;
}
void Destory()
{
while (_head)
{
PopBack();
}
}
void Print()
{
if (_head == NULL)
{
return;
}
LinkNode *begin = _head;
while (begin != _tail)
{
cout << begin->_data<<" ";
begin = begin->_next;
}
cout << begin->_data << endl;;
}
private:
LinkNode *_head;
LinkNode *_tail;
};
int main()
{
sList op1;
op1.PushBack(1);
op1.PushBack(2);
op1.PushBack(3);
op1.PushBack(4);
op1.PushBack(5);
op1.Print();
op1.Reverse();
op1.Print();
/*op1.PopBack();
op1.PopBack();
op1.PopBack();*/
//op1.PopBack();
//op1.PopBack();
/*op1.PushFront(100);
op1.PushFront(200);
op1.PushFront(300);
op1.PushFront(400);
op1.Print();
op1.PopFront();
op1.PopFront();
op1.PopFront();
op1.PopFront();
op1.Print();*/
/*LinkNode *tmp =op1. Find(5);
cout << "tmp->_data=" << tmp << endl;
op1.Remove(tmp);
op1.Print();*/
//op1.Destory();
/*op1.Print();
sList op2 = op1;
cout << "op2= " << endl;
op2.Print();
sList op3;
op3 = op2;
cout << "op3=";
op3.Print();*/
/*op1.Insert(tmp,6);
op1.Print();*/
system("pause");
return 0;
}
【C++】单链表的基本操作
最新推荐文章于 2023-01-03 20:06:47 发布
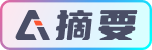