圆⚪
import turtle as t
t.circle(100)
t.done()
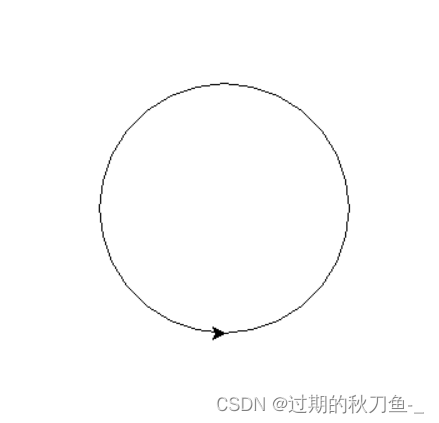
import turtle
turtle.speed(0)
turtle.color('blue')
for i in range(100):
turtle.left(78)
turtle.circle(i )
turtle.mainloop()
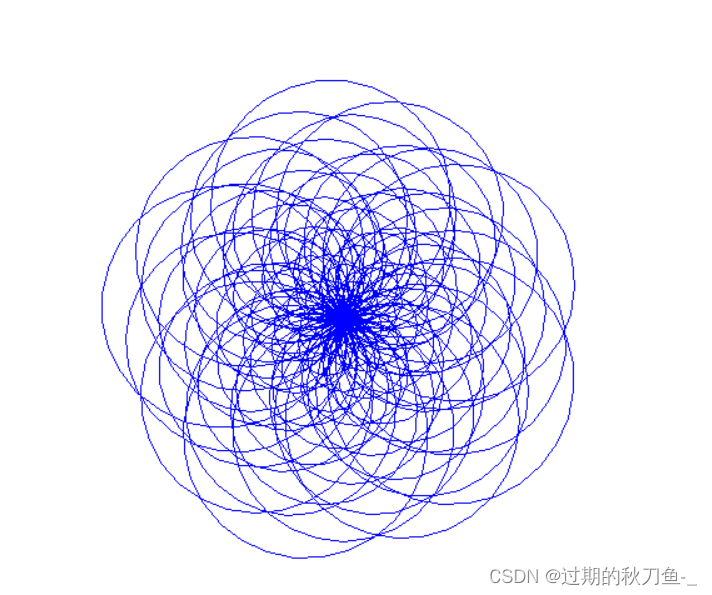
奥运五环
import turtle as t
t.pensize(5)
t.color('black')
t.circle(100)
t.penup()
t.goto(-220,0)
t.pendown()
t.color('blue')
t.circle(100)
t.penup()
t.goto(220,0)
t.pendown()
t.color('red')
t.circle(100)
t.penup()
t.goto(-120,-120)
t.pendown()
t.circle(100)
t.color('yellow')
t.penup()
t.goto(120,-120)
t.pendown()
t.circle(100)
t.color('green')
t.color('red')
t.penup()
t.goto(-150,250)
t.pendown()
t.write('北 京 欢 迎 你',font=('kaiti',32))
t.mainloop()
- 下图可见最后海龟写完北京欢迎你后,还留在画布上,我们可以使用
t.hideturtle()
隐藏海龟, - 隐藏后如果想显示海龟可以使用
t.showturtle()
方法来让海龟出现在画布上
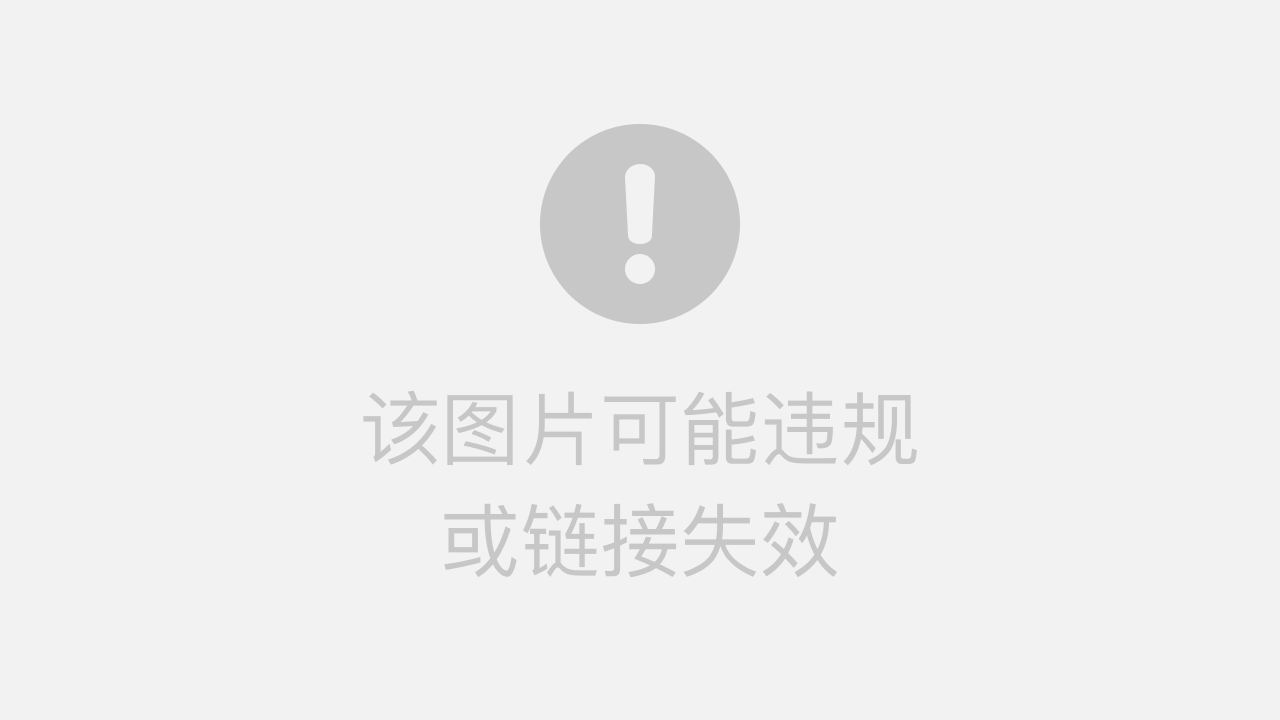
美国盾牌
import turtle as t
t.speed(0)
t.penup()
t.goto(0, -200)
t.pendown()
t.color('red')
t.begin_fill()
t.circle(200)
t.end_fill()
t.penup()
t.goto(0, -150)
t.pendown()
t.color('white')
t.begin_fill()
t.circle(150)
t.end_fill()
t.penup()
t.goto(0, -100)
t.pendown()
t.color('red')
t.begin_fill()
t.circle(100)
t.end_fill()
t.penup()
t.goto(0, -50)
t.pendown()
t.color('blue')
t.begin_fill()
t.circle(50)
t.end_fill()
t.penup()
t.goto(-40, 13)
t.pendown()
t.color('white')
t.begin_fill()
for i in range(5):
t.forward(80)
t.right(144)
t.end_fill()
t.hideturtle()
t.mainloop()
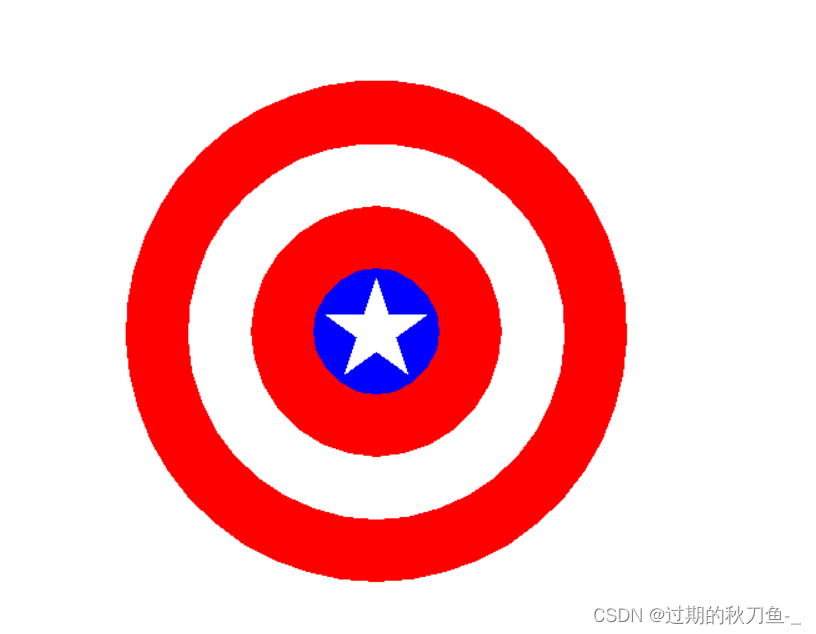
彩旗飘飘
- 引入了
random
模块,让气球出现的位置随机,气球的颜色也随机
"""
1.绘制一个气球(圆圈+直线)
2.填充随机颜色
3.出现随机位置
4.绘制画面中多个彩色气球
"""
import turtle as t
import random
t.colormode(255)
t.speed(0)
for i in range(20):
red = random.randint(0, 255)
green = random.randint(0, 255)
blue = random.randint(0, 255)
x = random.randint(-220, 220)
y = random.randint(-100, 220)
t.penup()
t.goto(x,y)
t.pendown()
t.color(red, green, blue)
t.begin_fill()
t.circle(30)
t.end_fill()
t.right(90)
t.forward(30)
t.left(90)
t.mainloop()
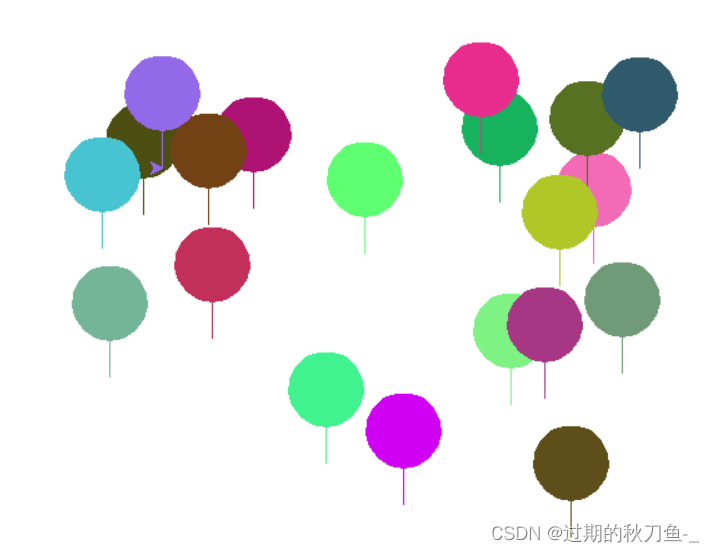
繁星满天
- 为了减少代码冗余,使用循环,减少代码量
- 繁星出现的位置随机,颜色在黄色这个
色域
里面随机
import random
import turtle as t
t.speed(0)
t.colormode(255)
t.bgcolor('black')
t.pensize(100)
color_total=50
position=280
color_add=25
goto_jian=100
for i in range(7):
t.color(color_total,color_total,color_total)
t.penup()
t.goto(-400,position)
t.pendown()
t.forward(900)
color_total += color_add
position-=goto_jian
t.pensize(3)
for i in range(7):
red=random.randint(180,255)
green=random.randint(180,255)
blue=0
t.color(red,green,blue)
x=random.randint(-200,200)
y=random.randint(0,250)
t.penup()
t.goto(x,y)
t.pendown()
l=random.randint(10,20)
t.begin_fill()
for i in range(4):
t.forward(l)
t.left(30)
t.forward(l)
t.right(120)
t.hideturtle()
t.end_fill()
t.left(30)
t.mainloop()
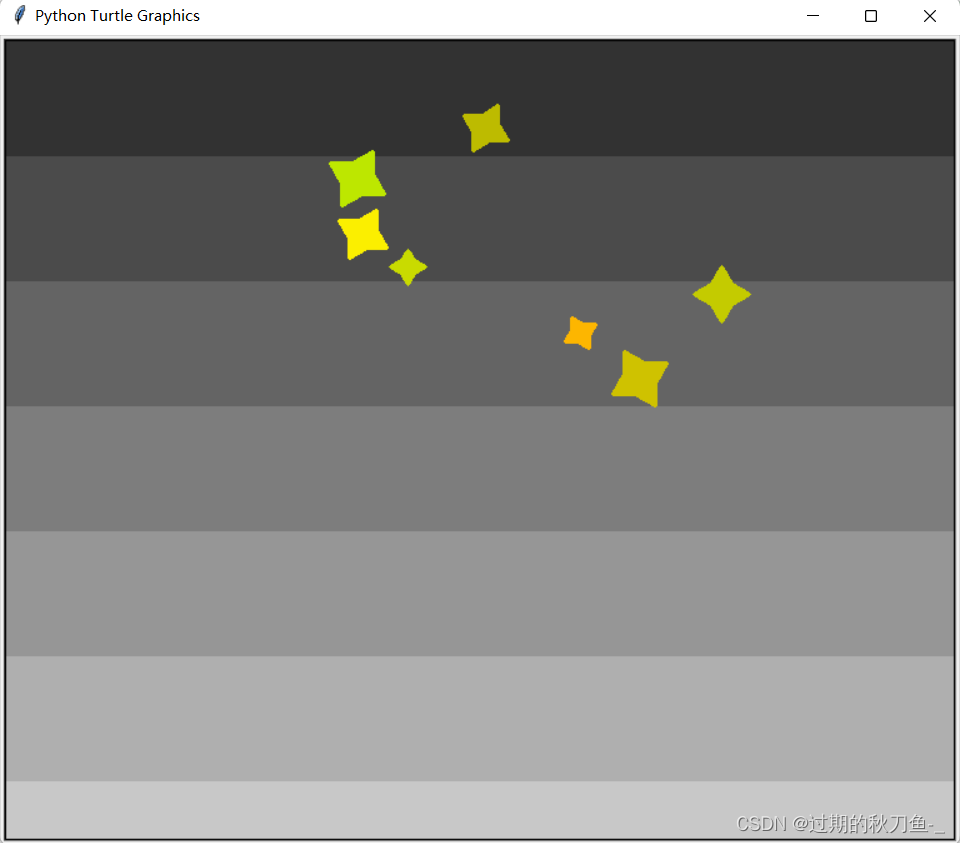
太阳花
import turtle as t
t.penup()
t.goto(-100,0)
t.pendown()
t.color('red','yellow')
t.speed(10)
t.begin_fill()
for i in range(50):
t.fd(200)
t.right(170)
t.end_fill()
t.done()
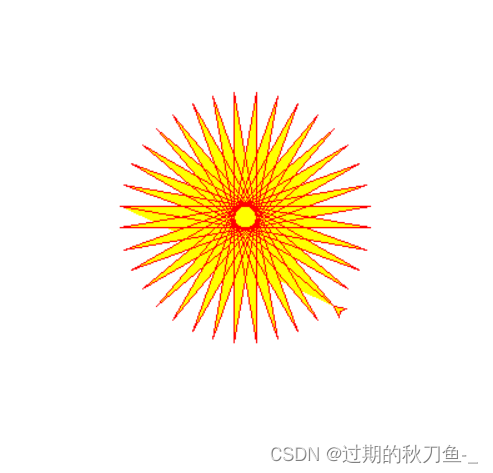