✨✨小新课堂开课了,欢迎欢迎~✨✨
🎈🎈养成好习惯,先赞后看哦~🎈🎈
所属专栏:C++:由浅入深篇
小新的主页:编程版小新-CSDN博客
前言:我们今天主要介绍的是类的六个默认成员函数,在学习完这六个默认成员函数之后,我们还将会用所学知识着手实现一个日期类。
1.类的默认成员函数
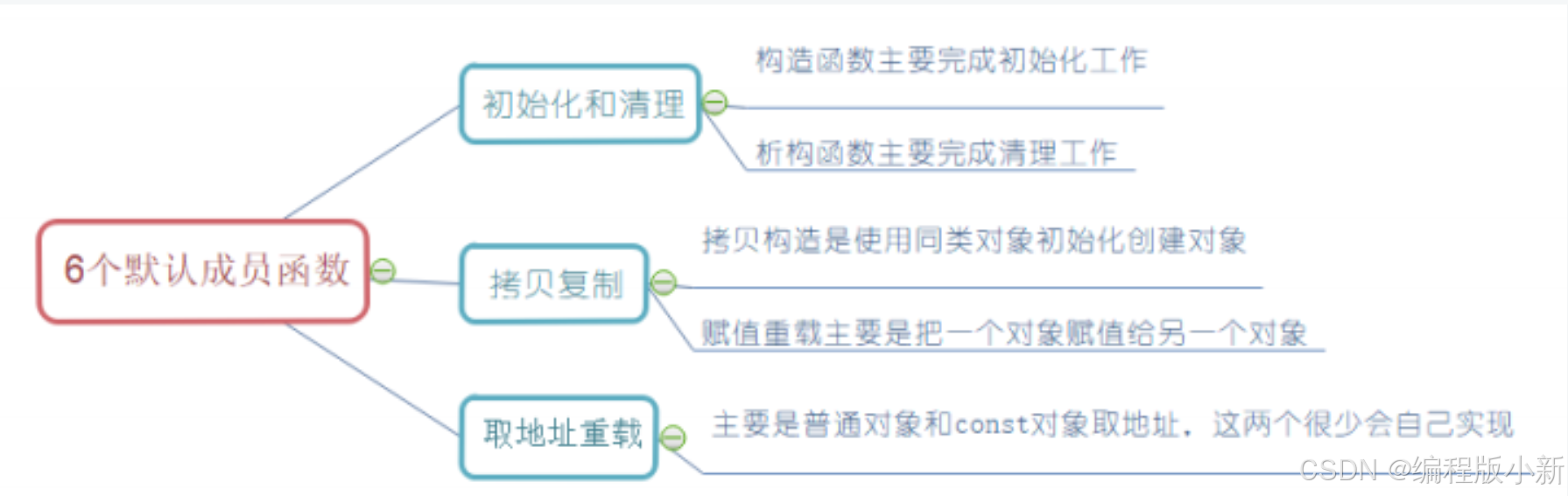
2.构造函数
2.1定义
构造函数:构造函数的名称必须与类名相同,创建类类型对象时,编译器会自动调用,以保证每一个数据成员都有一个合适的初始值,并在对象的整个生命周期中只调用一次。
2.2特点
构造函数的特点:1. 函数名与类名相同。2. 无返回值。 (返回值啥都不需要给,也不需要写void,不要纠结,C++规定如此)3. 对象实例化时系统会自动调用对应的构造函数。4. 构造函数可以重载。
注意:构造函数虽然名称叫构造,但是构造函数的主要任务并不是开空间创建对象(我们常使用的局部对象是栈帧创建时,空间就开好了),而是对象实例化时初始化对象。
2.3小细节
下面我们实现一个日期类的构造函数
#include<iostream>
using namespace std;
class Date
{
public:
//我们自己实现的构造函数
Date(int year, int month, int day)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "-" << _month << "-" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2014, 7, 27);//自动调用
d1.Print();
return 0;
}
运行结果:
构造函数的本质是要替代我们以前Stack和Date类中写的Init函数的功能,构造函数的自动调用特点就完美的替代的了Init,代码的容错率也大大提高(我们有时可能会忘记初始化变量),后面这一点会更有体现。
如果类中没有显式定义构造函数,则C++编译器会自动生成一个无参的默认构造函数,一旦用户显式定义编译器将不再生成。
#include<iostream>
using namespace std;
class Date
{
public:
//我们自己实现的构造函数
/*Date(int year, int month, int day)
{
_year = year;
_month = month;
_day = day;
}*/
//编译器会默认生成一个构造函数
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
//无参的不能加() Data d1() ,区分函数的声明与实例化
Date d1;//自动调用
d1.Print();
return 0;
}
运行结果:
d1调用了编译器默认生成的构造函数进行初始化,从运行结果来看,这里都是随机值,这是为什么呢?
编译器自动生成的构造函数对内置类型不做处理,对于自定义类型,编译器会调用他们自己的默认构造函数(有三个默认构造函数)。
#include<iostream>
using namespace std;
class test
{
public:
test()
{
cout << "test" << endl;
}
};
class Date
{
public:
//编译器会默认生成一个构造函数
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
private:
test t;
int _year;
int _month;
int _day;
};
int main()
{
Date d1;//自动调用
d1.Print();
return 0;
}
运行结果:
说明:C++把类型分成内置类型(基本类型)和自定义类型。内置类型就是语言提供的原生数据类型, 如:int/char/double/指针等,自定义类型就是我们使用class/struct等关键字自己定义的类型。
#include<iostream>
using namespace std;
class Date
{
public:
// 1.无参构造函数
Date()
{
_year = 1;
_month = 1;
_day = 1;
}
// 2.全缺省构造函数
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1; // 调用默认构造函数
d1.Print();
return 0;
}
运行结果:
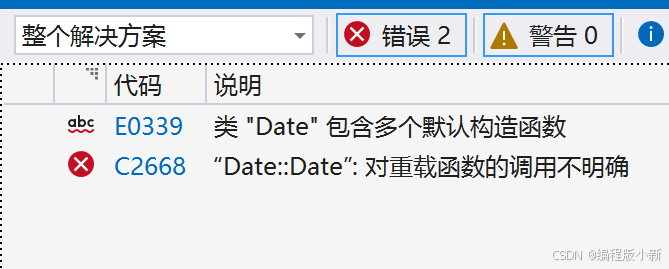
3.析构函数
3.1定义
我们知道当一个类对象销毁时,局部对象是存在栈帧的,函数结束栈帧销毁,他就释放了,不需要我们管,例如,我们用日期类创建了一个对象d1,当d1被销毁时,对象d1当中的局部对象__year/_month/_day_也会被编译器销毁。所以严格说Date是不需要析构函数的。
但是这并不意味着析构函数没有什么意义。像栈(Stack)这样的类对象,当该对象被销毁时,其中动态开辟的空间并不会随之被销毁,需要我们对其进行空间释放,这时析构函数的意义就体现了。
3.2特点
析构函数的特点:1. 析构函数名是在类名前加上字符 ~。2. 无参数无返回值。 (这里跟构造类似,也不需要加void)3. ⼀个类只能有一个析构函数。若未显式定义,系统会自动生成默认的析构函数。4. 对象生命周期结束时,系统会自动调用析构函数。
3.3小细节
下面我们实现一个日期类的析构函数
#include<iostream>
using namespace std;
class Date
{
public:
//构造函数
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
//析构函数
~Date()
{
_year = _month = _day = 0;
}
private:
int _year;
int _month;
int _day;
};
析构函数就相当我们之前实现的Destroy函数,用于销毁动态开辟的空间,析构函数自动调用的特点,也大大的提高了代码的容错率。(我们有时可能会忘记释放空间)
如果类中没有显式写析构函数,则C++编译器会自动生成一个默认的析构函数,一旦用户显式定义编译器将不再生成。
class Date
{
public:
//构造函数
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
//编译器会自动生成默认的析构函数
private:
int _year;
int _month;
int _day;
};
#include<iostream>
using namespace std;
class test
{
public:
~test()
{
cout << "~test" << endl;
}
};
class Date
{
public:
//构造函数
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
//默认生成
private:
test t;
int _year;
int _month;
int _day;
};
int main()
{
Date d1;
return 0;
}
运行结果:
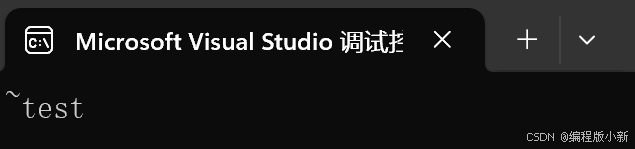
#include<iostream>
using namespace std;
class test
{
public:
~test()
{
cout << "~test" << endl;
}
};
class Date
{
public:
//构造函数
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
//析构函数
~Date()
{
_year = _month = _day = 0;
}
private:
test t;
int _year;
int _month;
int _day;
};
int main()
{
Date d1;
return 0;
}
运行结果:
#include<iostream>
using namespace std;
typedef int STDataType;
class Stack
{
public:
Stack(int n = 4)
{
_a = (STDataType*)malloc(sizeof(STDataType) * n);
if (nullptr == _a)
{
perror("malloc申请空间失败");
return;
}
_capacity = n;
_top = 0;
}
~Stack()
{
cout << "~Stack()" << endl;
free(_a);
_a = nullptr;
_top = _capacity = 0;
}
private:
STDataType* _a;
size_t _capacity;
size_t _top;
};
// 两个Stack实现队列
class MyQueue
{
public:
//编译器默认生成MyQueue的析构函数调用了Stack的析构,释放的Stack内部的资源
// 显示写析构,也会自动Stack的析构
/*~MyQueue()
{
}*/
private:
Stack pushst;
Stack popst;
};
int main()
{
Stack st;
MyQueue mq;
return 0;
}
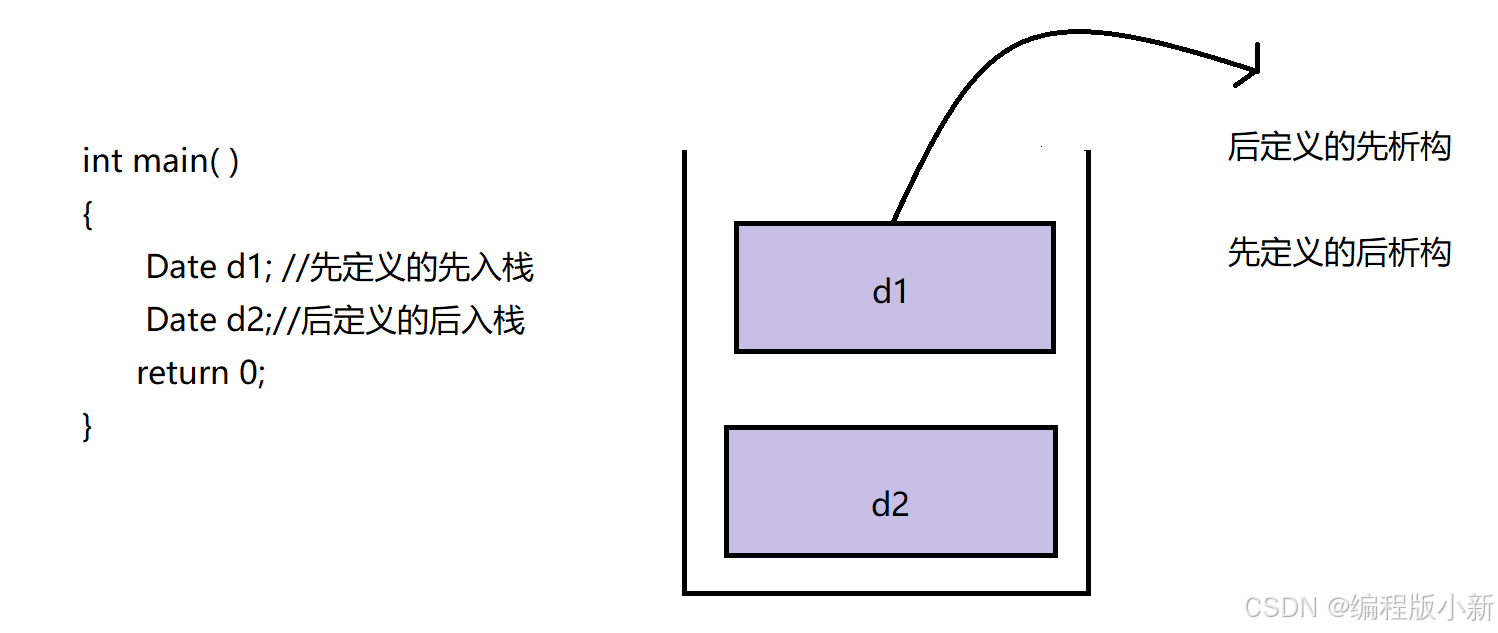
4.拷贝构造函数
4.1定义
4.2特点及小细节
#include<iostream>
using namespace std;
class Date
{
public:
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
//拷贝构造
Date(const Date& d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2024, 7, 27);
//两种写法
Date d2(d1);//拷贝构造
Date d3 = d1;//拷贝构造
d1.Print();
d2.Print();
d3.Print();
return 0;
}
运行结果:
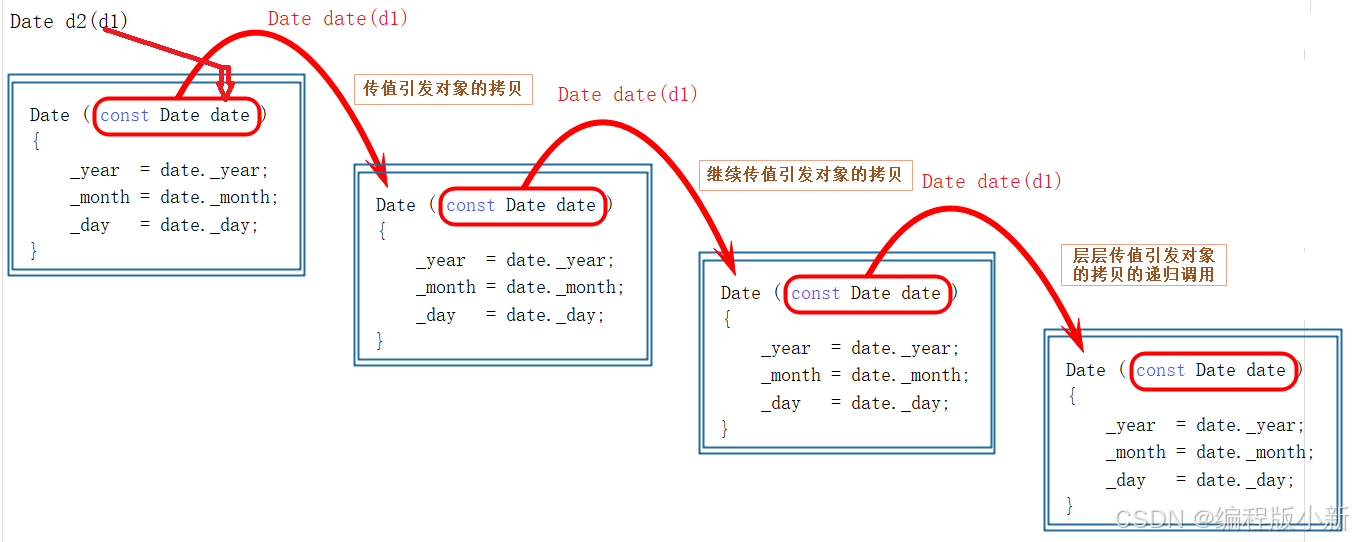
#include<iostream>
using namespace std;
class Date
{
public:
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2024, 7, 27);
//两种写法
Date d2(d1);//拷贝构造
Date d3 = d1;//拷贝构造
d1.Print();
d2.Print();
d3.Print();
return 0;
}
运行结果:
#include<iostream>
using namespace std;
typedef int STDataType;
class Stack
{
public:
Stack(int n = 4)
{
_a = (STDataType*)malloc(sizeof(STDataType) * n);
if (nullptr == _a)
{
perror("malloc申请空间失败");
return;
}
_capacity = n;
_top = 0;
}
Stack(const Stack& st)
{
// 需要对_a指向资源创建同样大的资源再拷⻉值
_a = (STDataType*)malloc(sizeof(STDataType) * st._capacity);
if (nullptr == _a)
{
perror("malloc申请空间失败!!!");
return;
}
memcpy(_a, st._a, sizeof(STDataType) * st._top);
_top = st._top;
_capacity = st._capacity;
}
void Push(STDataType x)
{
if (_top == _capacity)
{
int newcapacity = _capacity * 2;
STDataType* tmp = (STDataType*)realloc(_a, newcapacity *
sizeof(STDataType));
if (tmp == NULL)
{
perror("realloc fail");
return;
}
_a = tmp;
_capacity = newcapacity;
}
_a[_top++] = x;
}
~Stack()
{
cout << "~Stack()" << endl;
free(_a);
_a = nullptr;
_top = _capacity = 0;
}
private:
STDataType* _a;
size_t _capacity;
size_t _top;
};
// 两个Stack实现队列
class MyQueue
{
private:
Stack pushst;
Stack popst;
};
int main()
{
MyQueue mq1;
// MyQueue自动生成的拷⻉构造,会自动调用Stack拷⻉构造完成pushst/popst
MyQueue mq2 = mq1;
return 0;
}
#include<iostream>
using namespace std;
typedef int STDataType;
class Stack
{
public:
Stack(int n = 4)
{
_a = (STDataType*)malloc(sizeof(STDataType) * n);
if (nullptr == _a)
{
perror("malloc申请空间失败");
return;
}
_capacity = n;
_top = 0;
}
//Stack(const Stack& st)
//{
// // 需要对_a指向资源创建同样大的资源再拷⻉值
// _a = (STDataType*)malloc(sizeof(STDataType) * st._capacity);
// if (nullptr == _a)
// {
// perror("malloc申请空间失败!!!");
// return;
// }
// memcpy(_a, st._a, sizeof(STDataType) * st._top);
// _top = st._top;
// _capacity = st._capacity;
//}
void Push(STDataType x)
{
if (_top == _capacity)
{
int newcapacity = _capacity * 2;
STDataType* tmp = (STDataType*)realloc(_a, newcapacity *
sizeof(STDataType));
if (tmp == NULL)
{
perror("realloc fail");
return;
}
_a = tmp;
_capacity = newcapacity;
}
_a[_top++] = x;
}
~Stack()
{
cout << "~Stack()" << endl;
free(_a);
_a = nullptr;
_top = _capacity = 0;
}
private:
STDataType* _a;
size_t _capacity;
size_t _top;
};
int main()
{
Stack st1;
st1.Push(1);
st1.Push(2);
// Stack不显示实现拷⻉构造,用自动生成的拷⻉构造完成浅拷⻉
// 会导致st1和st2里面的_a指针指向同一块资源,析构时会析构两次,程序崩溃
Stack st2 = st1;
return 0;
}
5.运算符重载
5.1定义
5.2特点
运算符重载的特点
1• 运算符重载以后,其优先级和结合性与对应的内置类型运算符保持⼀致。2• 不能通过连接语法中没有的符号来创建新的操作符:比如operator@。3• .* , ::, sizeof, ? : , . 注意以上5个运算符不能重载。4• 重载操作符至少有⼀个类类型参数,不能通过运算符重载改变内置类型对象的含义,如: intoperator+(int x, int y)5• 重载运算符函数的参数个数和该运算符作用的运算对象数量一样多。一元运算符有一个参数,二元运算符有两个参数,二元运算符的左侧运算对象传给第一个参数,右侧运算对象传给第二个参数。6• 作为成员函数重载时,它的第一个参数默认传给隐式的this指针,因此参数看起来比操作数数目少一个。
#include<iostream>
using namespace std;
class Date
{
public:
Date(int year, int month, int day)
{
_year = year;
_month = month;
_day = day;
}
bool operator == ( const Date& d)
{
return _year == d._year
&& _month == d._month
&& _day == d._day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2024, 7, 27);
Date d2(2024, 7, 28);
if (d1 == d2)//这里也可以显示调用operator==(d1,d2);
{
cout << "日期相等" << endl;
}
else
{
cout << "日期不相等" << endl;
}
return 0;
}
5.3小细节
上面实现的运算符重载函数是在类里面实现的,我们也可以将运算符重载函数放在类外面,但此时类外部无法访问类中的成员变量,这时我们可以将类中的成员变量设置为共有(public),这样外部就可以访问该类的成员变量了(也可以用友元函数解决该问题)。并且在类外没有this指针,所以此时函数的形参我们必须显示的设置两个。
#include<iostream>
using namespace std;
class Date
{
public:
Date(int year, int month, int day)
{
_year = year;
_month = month;
_day = day;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
int _year;
int _month;
int _day;
};
bool operator == (const Date& d1, const Date& d2)
{
return d1._year == d2._year
&& d1._month == d2._month
&& d1._day == d2._day;
}
int main()
{
Date d1(2024, 7, 27);
Date d2(2024, 7, 28);
if (d1 == d2)//这里也可以显示调用operator==(d1,d2);
{
cout << "日期相等" << endl;
}
else
{
cout << "日期不相等" << endl;
}
return 0;
}
这里还有一些知识点我们会在下面实现的日期类中补充说明。
6.赋值运算符重载
6.1定义
6.2特点及小细节
下面我们简单实现一个赋值运算符重载
#include<iostream>
using namespace std;
class Date
{
public:
// 构造函数
Date(int year = 1, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
// 赋值运算符重载函数
Date& operator=(const Date& d)
{
if (this != &d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
return *this;
}
void Print()
{
cout << _year << "/" << _month << "/" << _day << endl;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2014, 7, 27);
Date d2(2024, 7, 28);
d2 = d1;
d2.Print();
return 0;
}
赋值操作进行完毕时,我们应该返回赋值运算符的左操作数,而在函数体内我们只能通过this指针访问到左操作数,所以要返回左操作数就只能返回*this。
int main()
{
Date d1(2024, 7, 27);
Date d2(d1);
Date d3(2024, 7, 28);
d1 = d3;//赋值运算符重载
// 需要注意这里是拷⻉构造,不是赋值重载
// 请牢牢记住赋值重载完成两个已经存在的对象直接的拷⻉赋值
// 而拷⻉构造用于⼀个对象拷⻉初始化给另⼀个要创建的对象
Date d4 = d1;
return 0;
}
7.const成员函数
// void Print(const Date* const this) const
void Print() const
{
cout << _year << "/" << _month << "/" << _day << endl;
}
8. 取地址运算符重载
class Date
{
public:
//普通取地址运算符重载
Date* operator&()
{
return this;
// return nullptr;
}
//const取地址运算符重载
const Date* operator&()const
{
return this;
// return nullptr;
}
private:
int _year;
int _month;
int _day;
};
9.日期类的实现
到这里我们已经学完了类的六个成员函数,现在我们来完整的实现一个日期类,对前面的知识进行简单的复盘。
#pragma once
#include<iostream>
using namespace std;
#include<assert.h>
class Date
{
//友元函数
friend ostream& operator<<(ostream& out, const Date& d);
friend istream& operator>>(istream& in, Date& d);
public:
bool CheckDate() const;
Date(int year = 1000, int month = 1, int day = 1);
void Print() const;
bool operator<(const Date& d) const;
bool operator<=(const Date& d) const;
bool operator>(const Date& d) const;
bool operator>=(const Date& d) const;
bool operator==(const Date& d) const;
bool operator!=(const Date& d) const;
Date operator+(int day) const;
Date& operator+=(int day);
Date operator-(int day) const;
Date& operator-=(int day);
// d1++;
// d1.operator++(0);
Date operator++(int);
// ++d1;
// d1.operator++();
Date& operator++();
// d1--;
// d1.operator--(0);
Date operator--(int);
// --d1;
// d1.operator--();
Date& operator--();
// d1 - d2
int operator-(const Date& d) const;
//void operator<<(ostream& out);
private:
int _year;
int _month;
int _day;
};
ostream& operator<<(ostream& out, const Date& d);
istream& operator>>(istream& in, Date& d);
构造函数
inline int GetMonthDay(int year, int month)
{
assert(month > 0 && month < 13);
static int monthDayArray[13] = { -1, 31, 28, 31, 30, 31, 30,
31, 31, 30, 31, 30, 31 };
if (month == 2 && ((year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)))
{
return 29;
}
return monthDayArray[month];
}
//检查日期的合法性
bool Date::CheckDate() const
{
if (_month < 1 || _month > 12 ||
_day < 1 || _day > GetMonthDay(_year, _month))
{
return false;
}
else
{
return true;
}
}
Date::Date(int year , int month , int day )
{
_year = year;
_month = month;
_day = day;
if (!CheckDate())
{
cout << "非法日期:";
Print();
}
}
这里我们来看一下GetMonthDay( )函数的小细节
首先,该函数会被频繁调用,所以我们将其设置为内联函数,提升执行效率。
其次,函数中存储每月天数的数组最好是用static修饰,存储在静态区,避免每次调用该函数都需要重新开辟数组。
最后,我们在判断时是先判断是否是二月在判断是否是闰年,提高了效率,因为如果不是二月就没必要判断是不是闰年。
注意:当函数声明和定义分开时,在声明时注明缺省参数,定义时不标出缺省参数。
打印函数
void Date::Print() const
{
cout << "_year" << '/' << "_month" << '/' << "_day" << endl;
}
日期+=天数
如果给定的天数是负数,加上一个负数就等效于减去一个正数,我们直接复用-=的运算符重载。
我们先将要加的天数加到日上面,然后判断日期是否合法,若不合法,则通过不断调整,直到日期合法为止。
调整日期的思路:
1.若日已满,则日减去当前月的天数,月加一。
2.若月已满,则将年加一,月置为1。
重复上述操作,直到日期合法为止。
Date& Date::operator+=(int day)
{
if (day < 0)
{
return *this -= (-day);
}
_day += day;
while (_day > GetMonthDay(_year,_month))
{
_day -= GetMonthDay(_year, _month);
_month++;
if (_month == 13)
{
_year++;
_month = 1;
}
}
return *this;
}
日期+天数
我们知道a = b+1,a的结果是b+1的返回值,但是b的值不会变,这也是+=的区别,我们返回tmp,但是不改变this指针指向的对象,因此我们还可以用const对该函数进行修饰,防止函数内部改变了this指针指向的对象。
Date Date::operator+(int day) const
{
Date tmp = *this;
tmp += day;
return tmp;
}
日期-=天数
如果给定的天数是负数,减去一个负数就等效于加上一个正数,我们直接复用+=的运算符重载。
们先将要天数减到日上面,然后判断日期是否合法,若不合法,则通过不断调整,直到日期合法为止。
调整日期的思路:
1.若日为非正,则月减一。
2.若月为0,则年减一,月置为12。
3.日加上当前月的天数。
重复上述操作,直到日期合法为止。
Date& Date::operator-=(int day)
{
if (day < 0)
{
return *this += (-day);
}
_day -= day;
while (_day <= 0)
{
_month--;
if (_month == 0)
{
_year--;
_month = 12;
}
_day += GetMonthDay(_year, _month);
}
return *this;
}
日期-天数
和日期+天数的思路一致。
Date Date::operator-(int day) const
{
Date tmp = *this;
tmp -= day;
return tmp;
}
前置++
前置++,我们可以复用+=运算符的重载函数。
// ++d1;
// d1.operator++();
Date& Date::operator++()
{
*this += 1;
return *this;
}
后置++
重载++运算符时,有前置++和后置++,运算符重载函数名都是operator++,无法很好的区分,C++规定,后置++重载时,增加一个int形参,跟前置++构成函数重载,方便区分。
后置++,我们也可以复用+=运算符的重载函数,这里要注意的是this指针指向的对象不改变
// d1++;
// d1.operator++(0);
Date Date::operator++(int)
{
Date tmp = *this;
tmp += 1;
return tmp;
}
前置--
前置--和后置--和前面的前置++和后置++的思路想法是一致的,不做过多的解释。
// --d1;
// d1.operator--();
Date& Date::operator--()
{
*this -= 1;
return *this;
}
后置--
// d1--;
// d1.operator--(0);
Date Date::operator--(int)
{
Date tmp = *this;
tmp -= 1;
return tmp;
}
日期类的大小关系比较
==运算符的重载
bool Date::operator==(const Date& d) const
{
return _year == d._year &&
_month == d._month &&
_day == d._day;
}
<运算符的重载
年大则大,年相等比月,月大则大,月相等比天,天大则大。
bool Date::operator<(const Date& d) const
{
if (_year > d._year)
{
return true;
}
else if (_year == d._year)
{
if (_month > d._month)
{
return true;
}
else if (_month == d._month)
{
return _day > d._day;
}
}
else
{
return false;
}
}
<=运算符的重载
下面这些的思路都是一样的,对前面实现的函数进行服用,以及!的伟大妙用。
bool Date::operator<=(const Date& d) const
{
return *this < d || *this == d;
}
>运算符的重载
bool Date::operator>(const Date& d) const
{
return !(*this <= d);
}
>=运算符的重载
bool Date::operator>=(const Date& d) const
{
return !(*this < d);
}
!=运算符的重载
bool Date::operator!=(const Date& d) const
{
return !(*this == d);
}
日期-日期
日期 - 日期,是在计算给定的两个日期相差的天数。我们只需要让较小的日期的天数一直加一,直到最后和较大的日期相等即可,这个过程中较小日期所加的总天数便是这两个日期之间差值的绝对值。若是第一个日期大于第二个日期,则返回这个差值的正值,若第一个日期小于第二个日期,则返回这个差值的负值。
代码中flag的妙用在于标记返回值的正负,flag为1代表返回的是正值,flag为-1代表返回的是负值,最后返回总天数与flag相乘之后的值即可。
// d1 - d2
int Date::operator-(const Date& d) const
{
int flag = 1;
Date max = *this;
Date min = d;
if (*this < d)
{
max = d;
min = *this;
flag = -1;
}
int n = 0;
while (max != max)
{
min++;
n++;
}
return n * flag;
}
自行输入
istream& operator>>(istream& in, Date& d)
{
while (1)
{
cout << "请依次输入年月日:>";
in >> d._year >> d._month >> d._day;
if (!d.CheckDate())
{
cout << "输入日期非法:";
d.Print();
cout << "请重新输入!!!" << endl;
}
else
{
break;
}
}
return in;
}
输出
ostream& operator<<(ostream& out, const Date& d)
{
out << d._year << "年" << d._month << "月" << d._day << "日" << endl;
return out;
}