#include"list.h"
//初始化
zt_p initia()
{
zt_p T=(zt_p)malloc(sizeof(zt));
if(T==NULL)
{
printf("空间申请失败\n");
return NULL;
}
T->front=NULL;
T->rear=NULL;
return T;
}
//创建节点
node_p create_node(int data)
{
node_p new=(node_p)malloc(sizeof(node));
if(new==NULL)
{
printf("空间申请失败\n");
return NULL;
}
new->data=data;
return new;
}
//判空
int empty_que(zt_p T)
{
if(T==NULL)
{
printf("入参为空\n");
return -1;
}
return T->front==NULL;
}
//入队
void push_que(zt_p T,int data)
{
if(T==NULL)
{
printf("入参为空\n");
return;
}
node_p new=(node_p)malloc(sizeof(node));
if(new==NULL)
{
printf("空间申请失败\n");
return;
}
new->data=data;
new->next=NULL;
if(empty_que(T))
{
T->front=T->rear=new;
}
else
{
T->rear->next=new;
T->rear=new;
}
}
//出队
int pop_que(zt_p T)
{
if(T==NULL)
{
printf("入参为空\n");
return -1;
}
if(empty_que(T))
{
printf("链式列为空\n");
return -2;
}
node_p p=T->front;
int data=p->data;
T->front=T->front->next;
if(T->front==NULL)
{
T->rear=NULL;
}
free(p);
return data;
}
//输出链式列
void show_que(zt_p T)
{
if(T==NULL)
{
printf("入参为空\n");
return;
}
if(empty_que(T))
{
printf("链式列为空\n");
return;
}
node_p p=T->front;
for(;p!=NULL;p=p->next)
{
printf("%-4d",p->data);
}
putchar(10);
}
//销毁链式列
void des_que(zt_p *T)
{
if(empty_que(*T))
{
printf("入参为空\n");
}
if(empty_que(*T))
{
pop_que(*T);
}
free(*T);
*T=NULL;
}
04.19
最新推荐文章于 2024-11-15 18:38:47 发布
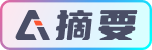