#include <stdio.h>
using namespace std;
#include <stack>
struct location
{
int x;//行
int y;//列
};
//定义一个地图,墙 = -1,路 = 0,走过的点 = 1, 已走过的回退的无效点 = 2
int MAP[10][11]={
{ 0, -1, -1, -1, -1, -1, -1, -1, -1, -1, -1},
{ 0, 0, 0, -1, -1, 0, 0, 0, 0, -1, -1},
{-1, 0, -1, 0, -1, -1, 0, -1, 0, -1, -1},
{-1, 0, -1, 0, -1, -1, 0, -1, 0, 0, 0},
{-1, 0, -1, 0, 0, -1, 0, -1, -1, 0, -1},
{-1, 0, -1, -1, 0, -1, 0, 0, -1, 0, -1},
{-1, 0, -1, 0, 0, 0, -1, 0, 0, 0, 0},
{-1, 0, -1, 0, -1, 0, -1, 0, -1, 0, -1},
{-1, 0, -0, 0, -1, 0, 0, 0, -1, 0, 0},
{-1, -1, -1, 0, -1, -1, -1, 0, -1, -1, 0},
};
void print_map()
{
for(int i = 0; i < 10; i++)
{
for(int j = 0; j < 11; j++)
{
printf("%3d",MAP[i][j]);
}
printf("\n");
}
}
//尝试下一个位置是否能走,顺序依次为,右、下、左、上
int isok(location current_loc)
{
//如果下一个位置未被走过,即地图值为0,而且不会超过地图范围,则说明此位置可走,返回方向数字
if(MAP[current_loc.x][current_loc.y+1] == 0 && current_loc.y+1 < 11)
{
return 1;
}
else if(MAP[current_loc.x+1][current_loc.y] == 0 && current_loc.x+1 < 10)
{
return 2;
}
else if(MAP[current_loc.x][current_loc.y-1] == 0 && current_loc.y-1 >= 0)
{
return 3;
}
else if(MAP[current_loc.x-1][current_loc.y] == 0 && current_loc.x-1 >=0)
{
return 4;
}
else
{
return 0;
}
}
int main()
{
stack<location> qs;
int flag = -1;//标记是否已无路可走,初始值-1,0表示成功,1表示无解
location start_loc;
start_loc.x = 0;
start_loc.y = 0;
location end_loc;
end_loc.x = 9;
end_loc.y = 10;
location current_loc;
current_loc.x = start_loc.x;
current_loc.y = start_loc.y;
qs.push(current_loc);//将走过的结点压栈,以便与走到死胡同时的回溯
while(1)
{
//将当前位置在地图上标记
MAP[current_loc.x][current_loc.y] = 1;
//如果当前位置和终点位置相同,则退出循环
if(current_loc.x == end_loc.x && current_loc.y == end_loc.y)
{
flag == 0;
break;
}
//尝试下一个位置
int ret = isok(current_loc);
switch (ret) {
case 1:
current_loc.y += 1;
qs.push(current_loc);
break;
case 2:
current_loc.x += 1;
qs.push(current_loc);
break;
case 3:
current_loc.y -= 1;
qs.push(current_loc);
break;
case 4:
current_loc.x -= 1;
qs.push(current_loc);
break;
default:
//若当前位置没有可走的下一步,且栈内没有可回溯的点,说明无法走到终点
if(qs.empty())
{
flag = 1;
break;
}
else
{
MAP[current_loc.x][current_loc.y] = 2;
current_loc = qs.top();
qs.pop();
}
break;
}
}
if(flag == 0)
{
printf("success to solve the maze!\n");
print_map();
}
else if(flag == 1)
{
printf("there is no way!\n");
print_map();
}
else
{
printf("the application error!\n");
print_map();
}
return 0;
}
一直没弄明白的迷宫问题,今天竟然突然开窍啦。记录一下,好像也没有那么难。。。
最新推荐文章于 2022-09-16 15:46:39 发布
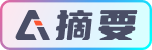