加载布局、数据库(增删改查)、load下载、图片加载
依赖
implementation 'org.xutils:xutils:3.5.0'
清单文件
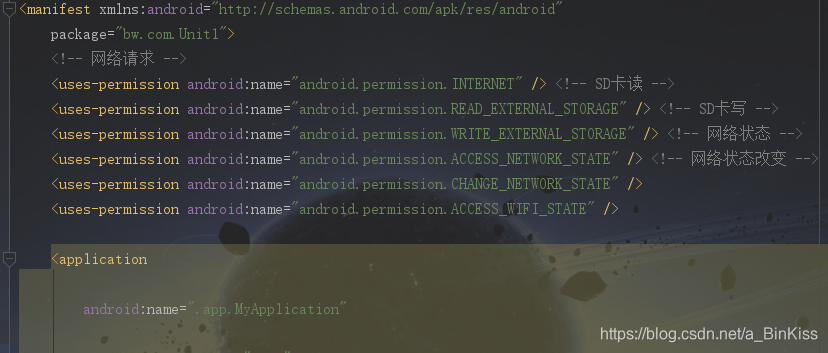
效果图
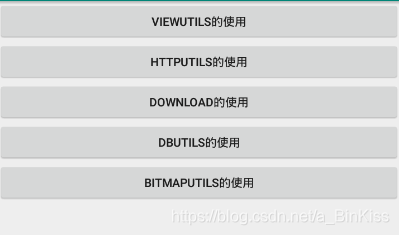
Application初始化
package bw.com.Unit1.app;
import android.app.Application;
import org.xutils.x;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.SSLSession;
public class MyApplication extends Application {
@Override
public void onCreate() {
super.onCreate();
x.Ext.init(this);
x.Ext.setDebug(true);
x.Ext.setDefaultHostnameVerifier(new HostnameVerifier() {
@Override
public boolean verify(String hostname, SSLSession session) {
return true;
}
});
}
}
主布局代码
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".MainActivity">
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="ViewUtils的使用"
/>
<Button
android:id="@+id/button2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="HttpUtils的使用"
/>
<Button
android:id="@+id/button3"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="DownLoad的使用"
/>
<Button
android:id="@+id/button4"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="DBUtils的使用"
/>
<Button
android:id="@+id/button5"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="BitmapUtils的使用"
/>
<ImageView
android:id="@+id/imageview"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</LinearLayout>
主类java代码
package bw.com.Unit1;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import org.xutils.view.annotation.ContentView;
import org.xutils.view.annotation.Event;
import org.xutils.view.annotation.ViewInject;
import org.xutils.x;
import bw.com.Unit1.app.HttpActivity;
@ContentView(R.layout.activity_main)
public class MainActivity extends AppCompatActivity {
@ViewInject(R.id.imageview)
private ImageView imageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
x.view().inject(this);
}
@Event({R.id.button1,R.id.button2,R.id.button3,R.id.button4})
private void click(View view){
Intent intent = new Intent();
switch (view.getId()){
case R.id.button1:
intent.setClass(MainActivity.this,ViewActivity.class);
break;
case R.id.button2:
intent.setClass(MainActivity.this, HttpActivity.class);
break;
case R.id.button3:
intent.setClass(MainActivity.this, DownLoadActivity.class);
break;
case R.id.button4:
intent.setClass(MainActivity.this, DBActivity.class);
break;
}
startActivity(intent);
}
@Event(R.id.button5)
private void getImageview(View view){
x.image().bind(imageView,"https://images0.cnblogs.com/blog/651487/201501/281616176915467.jpg");
}
}
ViewUtils
布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
tools:context=".ViewActivity">
<Button
android:id="@+id/btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="单击返回!"
/>
<RadioButton
android:id="@+id/rb"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="长按"
/>
<CheckBox
android:id="@+id/cb"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="选中与取消"
/>
</LinearLayout>
java代码
package bw.com.Unit1;
import android.app.Activity;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.CompoundButton;
import android.widget.Toast;
import org.xutils.view.annotation.ContentView;
import org.xutils.view.annotation.Event;
import org.xutils.view.annotation.ViewInject;
import org.xutils.x;
@ContentView(R.layout.activity_view)
public class ViewActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
x.view().inject(ViewActivity.this);
}
@Event(R.id.btn)
private void click(View view){
switch (view.getId()){
case R.id.btn:
Toast.makeText(this, "点击成功!", Toast.LENGTH_SHORT).show();
finish();
break;
}
}
@Event(value = {R.id.rb,},type = View.OnLongClickListener.class)
private boolean longclick(View view){
Toast.makeText(this, "单选长按成功!", Toast.LENGTH_SHORT).show();
return true;
}
@Event(value = {R.id.cb,},type = CompoundButton.OnCheckedChangeListener.class)
private void checkclick(CompoundButton compoundButton,boolean b){
if (b){
Toast.makeText(this, "选中了!", Toast.LENGTH_SHORT).show();
}else{
Toast.makeText(this, "取消了", Toast.LENGTH_SHORT).show();
}
}
}
HttpUtils
布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".app.HttpActivity">
<Button
android:id="@+id/getBtn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="GET请求"
/>
<Button
android:id="@+id/postBtn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="POST请求"
/>
</LinearLayout>
Java代码
package bw.com.Unit1.app;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import org.xutils.common.Callback;
import org.xutils.http.RequestParams;
import org.xutils.view.annotation.ContentView;
import org.xutils.view.annotation.Event;
import org.xutils.x;
import bw.com.Unit1.R;
@ContentView(R.layout.activity_http)
public class HttpActivity extends AppCompatActivity {
String url = "http://www.qubaobei.com/ios/cf/dish_list.php?stage_id=1&limit=10&page=1";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
x.view().inject(this);
}
@Event(R.id.getBtn)
private void GETHttp(View view){
x.http().get(new RequestParams(url), new Callback.CommonCallback<String>() {
@Override
public void onSuccess(String result) {
Log.e("###",result);
}
@Override
public void onError(Throwable ex, boolean isOnCallback) {
}
@Override
public void onCancelled(CancelledException cex) {
}
@Override
public void onFinished() {
}
});
}
@Event(R.id.postBtn)
private void POSTHttp(View view){
RequestParams requestParams = new RequestParams("https://www.apiopen.top/createUser?key=00d91e8e0cca2b76f515926a36db68f5&");
requestParams.addQueryStringParameter("phone","zzz");
requestParams.addQueryStringParameter("passwd","123");
x.http().post(requestParams, new Callback.CommonCallback<String>() {
@Override
public void onSuccess(String result) {
Log.e("@@@",result);
}
@Override
public void onError(Throwable ex, boolean isOnCallback) {
}
@Override
public void onCancelled(CancelledException cex) {
}
@Override
public void onFinished() {
}
});
}
}
DownLoad
布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".DownLoadActivity">
<Button
android:id="@+id/downBtn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="DownLoad下载"
/>
</LinearLayout>
Java代码
package bw.com.Unit1;
import android.app.ProgressDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.net.Uri;
import android.os.Environment;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Toast;
import org.xutils.common.Callback;
import org.xutils.http.RequestParams;
import org.xutils.view.annotation.ContentView;
import org.xutils.view.annotation.Event;
import org.xutils.x;
import java.io.File;
@ContentView(R.layout.activity_down_load)
public class DownLoadActivity extends AppCompatActivity {
private static final String SAVEPATH = Environment.getExternalStorageDirectory()+"/qq.apk";
private ProgressDialog progressDialog;
private Callback.Cancelable cancelable;
private String url = "http://softfile.3g.qq.com:8080/msoft/179/24659/43549/qq_hd_mini_1.4.apk";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
x.view().inject(this);
initProgressDialog();
}
private void initProgressDialog() {
progressDialog = new ProgressDialog(DownLoadActivity.this);
progressDialog.setTitle("下载APK");
progressDialog.setMessage("下载中……");
progressDialog.setProgressStyle(ProgressDialog.STYLE_HORIZONTAL);
progressDialog.setCanceledOnTouchOutside(false);
progressDialog.setButton(ProgressDialog.BUTTON_NEGATIVE, "暂停", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
cancelable.cancel();
}
});
}
@Event(R.id.downBtn)
private void DownLoad(View view){
downloadApk();
}
private void downloadApk() {
RequestParams requestParams = new RequestParams();
requestParams.setUri(url);
requestParams.setAutoRename(true);
requestParams.setCancelFast(true);
requestParams.setSaveFilePath(SAVEPATH);
cancelable = x.http().get(requestParams, new Callback.ProgressCallback<File>() {
@Override
public void onSuccess(File result) {
Toast.makeText(DownLoadActivity.this, "下载完成!路径:"+result.getAbsolutePath(), Toast.LENGTH_SHORT).show();
Intent intent = new Intent();
intent.setAction(Intent.ACTION_VIEW);
intent.setDataAndType(Uri.fromFile(result),"application/vnd.android.package-archive");
intent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
DownLoadActivity.this.startActivity(intent);
}
@Override
public void onError(Throwable ex, boolean isOnCallback) {
ex.getStackTrace();
}
@Override
public void onCancelled(CancelledException cex) {
}
@Override
public void onFinished() {
progressDialog.cancel();
}
@Override
public void onWaiting() {
}
@Override
public void onStarted() {
progressDialog.show();
}
@Override
public void onLoading(long total, long current, boolean isDownloading) {
progressDialog.setProgress((int) (current*100/total));
}
});
}
}
DBUtils
布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context=".DBActivity">
<Button
android:id="@+id/write_btn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="写入"
/>
<Button
android:id="@+id/read_btn"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="读取"
/>
<TextView
android:id="@+id/text_show"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
数据库类
package bw.com.Unit1.db;
import org.xutils.db.annotation.Column;
import org.xutils.db.annotation.Table;
@Table(name = "UserDButils")
public class UserDButils {
@Column(name = "id",isId = true)
private int id;
@Column(name = "name")
private String name;
@Column(name = "age")
private int age;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
@Override
public String toString() {
return "UserDButils{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
'}';
}
}
Java代码
package bw.com.Unit1;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import org.xutils.DbManager;
import org.xutils.ex.DbException;
import org.xutils.view.annotation.ContentView;
import org.xutils.view.annotation.Event;
import org.xutils.view.annotation.ViewInject;
import org.xutils.x;
import bw.com.Unit1.db.UserDButils;
@ContentView(R.layout.activity_db)
public class DBActivity extends AppCompatActivity {
private DbManager.DaoConfig daoConfig;
private DbManager dbManager;
@ViewInject(R.id.text_show)
private TextView textView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
x.view().inject(this);
initDB();
}
private void initDB() {
daoConfig = new DbManager.DaoConfig();
daoConfig.setDbName("UserDButils");
daoConfig.setDbVersion(1);
dbManager = x.getDb(daoConfig);
}
@Event({R.id.read_btn,R.id.write_btn})
private void readTable(View view){
switch (view.getId()){
case R.id.read_btn:
readDB();
break;
case R.id.write_btn:
writeDB();
break;
}
}
private void writeDB() {
UserDButils userDButils = new UserDButils();
userDButils.setId(1);
userDButils.setName("张三");
userDButils.setAge(21);
try {
dbManager.save(userDButils);
} catch (DbException e) {
e.printStackTrace();
}
}
private void readDB() {
try {
UserDButils first = dbManager.selector(UserDButils.class).findFirst();
textView.setText(first.toString());
} catch (DbException e) {
e.printStackTrace();
}
}
private void update() {
WhereBuilder whereBuilder = WhereBuilder.b();
whereBuilder.and("name","=","李四");
KeyValue keyValue = new KeyValue("name","钱七");
try {
dbManager.update(UserDb.class, whereBuilder,keyValue);
Log.e("修改","修改成功!");
} catch (DbException e) {
e.printStackTrace();
}
}
private void delete() {
WhereBuilder whereBuilder = WhereBuilder.b();
whereBuilder.and("name","=","张三");
try {
dbManager.delete(UserDb.class,whereBuilder);
Log.e("删除","删除成功!");
} catch (DbException e) {
e.printStackTrace();
}
}
BitmapUtils
Java代码
x.image().bind(imageView,"https://images0.cnblogs.com/blog/651487/201501/281616176915467.jpg");