Drawable Animation(Drawable动画)
效果图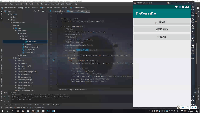
Java代码(XML实现)
package bw.com.Unit2;
import android.graphics.drawable.AnimationDrawable;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.ImageView;
import org.xutils.view.annotation.ContentView;
import org.xutils.view.annotation.Event;
import org.xutils.view.annotation.ViewInject;
import org.xutils.x;
import bw.com.Unit1.R;
@ContentView(R.layout.activity_frame_xml)
public class FrameXMLActivity extends AppCompatActivity {
private AnimationDrawable animationDrawable;
@ViewInject(R.id.iv_xml)
ImageView imageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
x.view().inject(this);
animationDrawable = (AnimationDrawable) imageView.getBackground();
}
@Event({R.id.start_btn,R.id.stop_btn})
private void click(View view){
switch (view.getId()){
case R.id.start_btn:
startFrame();
break;
case R.id.stop_btn:
stopFrame();
break;
}
}
private void stopFrame() {
animationDrawable.stop();
}
private void startFrame() {
animationDrawable.start();
}
}
布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="bw.com.Unit2.FrameXMLActivity">
<Button
android:id="@+id/start_btn"
android:text="开启帧动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/stop_btn"
android:text="停止帧动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ImageView
android:id="@+id/iv_xml"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/frame_xml"
/>
</LinearLayout>
Java代码(java实现)
package bw.com.Unit2;
import android.graphics.drawable.AnimationDrawable;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import org.xutils.view.annotation.ContentView;
import org.xutils.view.annotation.Event;
import org.xutils.view.annotation.ViewInject;
import org.xutils.x;
import bw.com.Unit1.R;
@ContentView(R.layout.activity_frame_java)
public class FrameJavaActivity extends AppCompatActivity {
@ViewInject(R.id.iv_java)
private ImageView imageView;
private AnimationDrawable animationDrawable;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
x.view().inject(this);
initAnimationDrawable();
}
private void initAnimationDrawable() {
animationDrawable = new AnimationDrawable();
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._1),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._2),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._3),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._4),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._5),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._6),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._7),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._8),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._9),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._10),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._11),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._12),500);
animationDrawable.addFrame(getResources().getDrawable(R.mipmap._13),500);
imageView.setImageDrawable(animationDrawable);
}
@Event({R.id.start_btn,R.id.stop_btn})
private void click(View v){
switch (v.getId()){
case R.id.start_btn:
animationDrawable.start();
break;
case R.id.stop_btn:
animationDrawable.stop();
break;
}
}
}
布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="bw.com.Unit2.FrameJavaActivity">
<Button
android:id="@+id/start_btn"
android:text="开启帧动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/stop_btn"
android:text="停止帧动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ImageView
android:id="@+id/iv_java"
android:layout_width="match_parent"
android:layout_height="match_parent"
/>
</LinearLayout>
View Animation(视图动画)
效果图
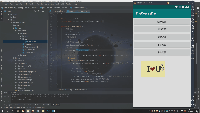
Java代码
package bw.com.Unit2;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.view.animation.BounceInterpolator;
import android.view.animation.ScaleAnimation;
import android.widget.ImageView;
import android.widget.Toast;
import org.xutils.view.annotation.ContentView;
import org.xutils.view.annotation.Event;
import org.xutils.view.annotation.ViewInject;
import org.xutils.x;
import bw.com.Unit1.R;
@ContentView(R.layout.activity_tween_frame)
public class TweenFrameActivity extends AppCompatActivity {
private AnimationUtils animationUtils;
@ViewInject(R.id.iv_Tween)
private ImageView imageView;
private int count = 1;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
x.view().inject(this);
animationUtils = new AnimationUtils();
}
@Event({R.id.alpha_btn,R.id.rotate_btn,R.id.scale_btn,R.id.tranScale_btn,R.id.set_btn})
private void click(View v){
switch (v.getId()){
case R.id.alpha_btn:
setAlphe();
break;
case R.id.rotate_btn:
setRotate();
break;
case R.id.scale_btn:
setScale();
break;
case R.id.tranScale_btn:
settranScale();
break;
case R.id.set_btn:
setAnimation();
break;
}
}
private void setAnimation() {
imageView.startAnimation(AnimationUtils.loadAnimation(this,R.anim.set));
}
private void settranScale() {
imageView.startAnimation(AnimationUtils.loadAnimation(this,R.anim.traslate));
}
private void setScale() {
ScaleAnimation scaleAnimation= new ScaleAnimation(1.0f,3.0f,1.0f,4.0f,
ScaleAnimation.ABSOLUTE,100,ScaleAnimation.ABSOLUTE,100);
scaleAnimation.setFillBefore(true);
scaleAnimation.setDuration(5000);
scaleAnimation.setRepeatCount(3);
scaleAnimation.setRepeatMode(ScaleAnimation.REVERSE);
scaleAnimation.setInterpolator(new BounceInterpolator());
scaleAnimation.setAnimationListener(new Animation.AnimationListener() {
@Override
public void onAnimationStart(Animation animation) {
Toast.makeText(TweenFrameActivity.this, "动画开始", Toast.LENGTH_SHORT).show();
}
@Override
public void onAnimationEnd(Animation animation) {
Toast.makeText(TweenFrameActivity.this, "动画结束", Toast.LENGTH_SHORT).show();
}
@Override
public void onAnimationRepeat(Animation animation) {
Toast.makeText(TweenFrameActivity.this, "重复次数为"+count++, Toast.LENGTH_SHORT).show();
}
});
imageView.startAnimation(scaleAnimation);
}
private void setRotate() {
imageView.startAnimation(AnimationUtils.loadAnimation(this,R.anim.rotate));
}
private void setAlphe() {
Animation animation = AnimationUtils.loadAnimation(this, R.anim.alpha);
imageView.startAnimation(animation);
}
}
布局文件
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="bw.com.Unit2.TweenFrameActivity">
<Button
android:id="@+id/alpha_btn"
android:text="透明动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/rotate_btn"
android:text="旋转动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/scale_btn"
android:text="缩放动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/tranScale_btn"
android:text="平移动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<Button
android:id="@+id/set_btn"
android:text="集合动画"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<ImageView
android:id="@+id/iv_Tween"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@mipmap/_13"
android:layout_marginLeft="50dp"
android:layout_marginTop="30dp"
/>
</LinearLayout>
anim
<?xml version="1.0" encoding="utf-8"?>
<alpha xmlns:android="http://schemas.android.com/apk/res/android"
android:fromAlpha="1.0"
android:toAlpha="0.0"
android:duration="5000"
android:fillBefore ="true"
>
</alpha>
<?xml version="1.0" encoding="utf-8"?>
<rotate xmlns:android="http://schemas.android.com/apk/res/android"
android:fromDegrees="0"
android:toDegrees="720"
android:pivotX="200"
android:pivotY="200"
android:fillBefore="true"
android:duration="5000"
>
</rotate>
<?xml version="1.0" encoding="utf-8"?>
<scale xmlns:android="http://schemas.android.com/apk/res/android"
android:fromXScale="1.0"
android:fromYScale="1.0"
android:toXScale="3.0"
android:toYScale="4.0"
android:pivotY="100"
android:pivotX="100"
android:fillBefore="true"
android:duration="5000"
>
</scale>
<?xml version="1.0" encoding="utf-8"?>
<translate xmlns:android="http://schemas.android.com/apk/res/android"
android:fromXDelta="0"
android:fromYDelta="0"
android:toXDelta="300"
android:toYDelta="300"
android:fillBefore="true"
android:duration="5000"
>
</translate>
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android"
>
<alpha
android:fromAlpha="1.0"
android:toAlpha="0.0"
android:duration="5000"
android:fillBefore ="true"
></alpha>
<translate
android:fromXDelta="0"
android:fromYDelta="0"
android:toXDelta="300"
android:toYDelta="300"
android:fillBefore="true"
android:duration="5000"
></translate>
<scale
android:fromXScale="1.0"
android:fromYScale="1.0"
android:toXScale="3.0"
android:toYScale="4.0"
android:pivotY="100"
android:pivotX="100"
android:fillBefore="true"
android:duration="5000"
></scale>
<rotate
android:fromDegrees="0"
android:toDegrees="720"
android:pivotX="200"
android:pivotY="200"
android:fillBefore="true"
android:duration="5000"
></rotate>
</set>