参考:http://www.cnblogs.com/codingmengmeng/p/5871254.html
1、首先是返回对象的情况:
#include<iostream>
#include<string.h>
using namespace std;
class String
{
private:
char *str;
int len;
public:
String(const char* s);//构造函数声明
String operator=(const String& another);//运算符重载,此时返回的是对象
void show()
{
cout << "value = " << str << endl;
}
/*copy construct*/
String(const String& other)
{
len = other.len;
str = new char[len + 1];
strcpy(str, other.str);
cout << "copy construct" << endl;
}
~String()
{
cout << "deconstruct" <<str<<endl;
delete[] str;
}
};
String::String(const char* s)//构造函数定义
{
len = strlen(s);
str = new char[len + 1];
strcpy(str, s);
cout<<"Constructor called"<<endl;
}
String String::operator=(const String &other)//运算符重载
{
if (this == &other)
return *this;
// return;
delete[] str;
len = other.len;
str = new char[len + 1];
strcpy(str, other.str);
return *this;
// return;
}
int main()
{
String str1("abc");
String str2("123");
String str3("456");
str1.show();
str2.show();
str3.show();
str3 = str1 = str2;//str3.operator=(str1.operator=str2)
str3.show();
str1.show();
return 0;
}
执行结果:
当运算符重载返回的是对象时,会在赋值运算过程的返回途中调用两次拷贝构造函数和析构函数(因为return的是个新的对象)。
2、下面是返回引用的情况(String& operator+(const String& str)),直接贴运行结果: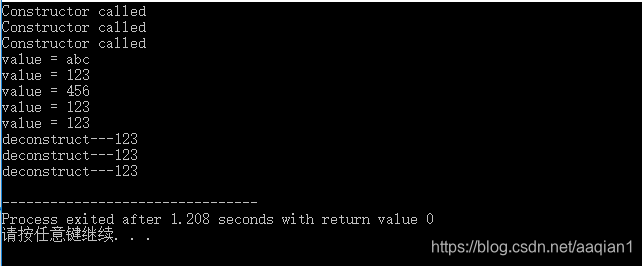
如果采用 String& operator+(const String& str) 这样就不会有多余的调用(因为这里直接return一个已经存在的对象的引用)。
3、把 = 重载中的程序改成返回对象的情况:
#include<iostream>
#include<string.h>
using namespace std;
class STRING{
private:
char *ptr;
public:
STRING(char *s){ //构造函数
ptr=new char[strlen(s)+1];
strcpy(ptr,s);
cout<<"Constructor called..."<<ptr<<endl;
}
STRING(const STRING &p){
ptr=new char[strlen(p.ptr)+1];
strcpy(ptr,p.ptr);
cout<<"copy constructor"<<endl;
}
~STRING(){
cout<<"Destructor called.---"<<ptr<<endl;
delete ptr;
}
STRING operator=(const STRING &); //声明赋值运算符重载函数 属于成员运算符重载函数
//STRING &operator属于使用引用返回函数值,返回函数的值类型为 STRING
//const STRING & 属于使用常引用作为函数参数 学习笔记30
};
STRING STRING::operator=(const STRING &s){ //定义赋值运算符重载函数
if(this==&s) return *this; //这里的 &s 表示 s 的地址
delete ptr;
ptr=new char[strlen(s.ptr)+1];
strcpy(ptr,s.ptr);
return *this;
}
int main(){
STRING p1("book");
STRING p2("junp");
STRING p3("r");
p1=p2;
return 0;
}
执行结果: