实例代码下载
学习SCSF 有写日子了,对该框架有一些了解,于是自己脑子发热写了个假SCSF 虽然不成熟,但是是对自己学习的一个总结。
主要框架示意图(解决方案):
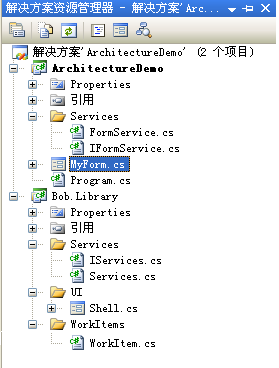
概念:
1.整个系统共用一个WorkItem(工作单元).
2.WorkItem中有 Service集合.
3.初始默认使用ShellForm.
WorkItem:
WorkItem 是自定义的静态类,在程序启动时加载默认设置,当前是代码以后会使用XML配置。
WorkItem代码:
WorkItem
using System;
using System.Collections.Generic;
using System.Text;
using System.Windows.Forms;
using Bob.Library.UI;
using Bob.Library.Services;

namespace Bob.Library.WorkItems


{
public static class WorkItem

{
private static Shell _ShellForm;
private static IServices _Services;

public static void InitWorkItem()

{
InitServices();
InitShellForm();
}


public static Shell ShellForm

{
get

{
if (_ShellForm == null)

{
InitShellForm();
}
return _ShellForm;
}
}

private static void InitShellForm()

{
_ShellForm = new Shell();
}


public static Bob.Library.Services.IServices Services

{
get

{
if (_Services == null)

{
InitServices();
}
return _Services;
}
}

private static void InitServices()

{
_Services = new Services.Services();
}

}
}
WorkItem 中有一个 IServices 类型的属性 Services,该属性用于保存全局的Service,
IService 有 AddService<TService>、GetServiceByKey<TService>、Clear 三个方法:
实现 添加、获取、清空Service操作。
代码:
IServices Services
//接口
using System;
using System.Collections.Generic;
using System.Text;

namespace Bob.Library.Services


{
public interface IServices

{
TService AddService<TService>(string key,TService service) where TService : class;

TService GetServiceByKey<TService>(string key) where TService : class;

void Clear();
}
}



//实现
using System;
using System.Collections.Generic;
using System.Text;

namespace Bob.Library.Services


{
public class Services :IServices

{
IDictionary<string, object> _Services;

public Services()

{
InitServices();
}

private void InitServices()

{
_Services = new Dictionary<string, object>();
}

IServices#region IServices

public TService AddService<TService>(string key, TService service) where TService : class

{
_Services[key] = service;
return _Services[key] as TService;
}

public TService GetServiceByKey<TService>(string key) where TService : class

{
object service = _Services[key];
return service is TService ? service as TService : null;
}

public void Clear()

{
InitServices();
}

#endregion
}
}
WorkItem 中还有一个 Shell 类型的ShellForm 属性:该属性是一个MDI窗口的实例,作为系统的父容器。
设计图:
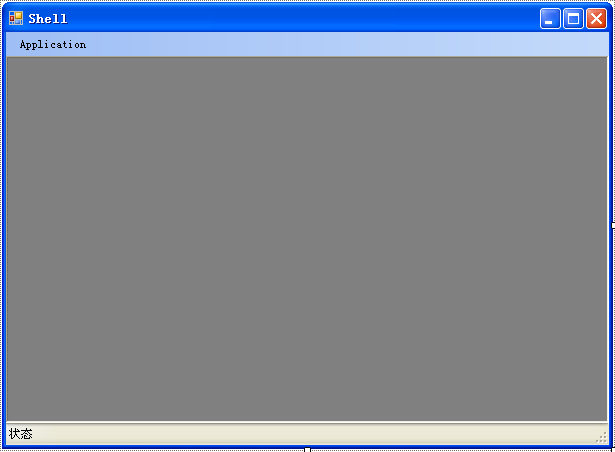
代码:
Shell
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;

namespace Bob.Library.UI


{
public partial class Shell : Form

{
public Shell()

{
InitializeComponent();
_AppMenu.Text = AppMenuName;
_ExitMenu.Text = ExitString;
}

public string FormName

{
get

{
return this.ParentForm.Text;
}
set

{
this.ParentForm.Text = value;
}
}

public void StatusUpdate(string message)

{
_ShellStatus.Text = message;
}

private void _ExitAppMenu_Click(object sender, EventArgs e)

{
if (MessageBox.Show("Exit ?", "Question", MessageBoxButtons.YesNo, MessageBoxIcon.Question) == DialogResult.Yes)

{
Environment.Exit(0);
}
}



MenuItemString#region MenuItemString
private string _AppName = "Application";
private string _ExitName = "Exit";
public string AppMenuName

{

get
{ return _AppName; }
set

{
_AppName = value;
_AppMenu.Text = _AppName;
}
}

public string ExitString

{

get
{ return _ExitName; }
set

{
_ExitName = value;
_ExitMenu.Text = _ExitName;
}
}

#endregion

public MenuStrip ShellMenu

{
get

{
return _ShellMenu;
}
}

}
}
Shell 中有 一个菜单控件,一个状态栏控件,将两个控件作为属性发布。
初始加载了一个菜单项 _AppMenu ,将菜单项的Text属性布.
然后为_AppMenu 添加一个子菜单项 _ExitMenu 同时将他的Text属性发布。
为_ExitMenu 添加事件 _ExitAppMenu_Click;
然后发布一个方法 StatusUpdate(string message) 在状态栏显示提示消息。
准备工作完成,开始项目开发:首先创建一个普通的winform项目,将 Bob.Library 应用进来,
在系统开始类 Program.cs 中添加 WorkItem的加载 代码如下:
Program
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
using Bob.Library.WorkItems;
using ArchitectureDemo.Services;

namespace ArchitectureDemo


{
static class Program

{
[STAThread]
static void Main()

{
Application.EnableVisualStyles();

Application.SetCompatibleTextRenderingDefault(false);

InitWorkItem();

Application.Run(WorkItem.ShellForm);
}

private static void InitWorkItem()

{
WorkItem.InitWorkItem();

AddServices();

AddModules();
}



private static void AddModules()

{
WorkItem.ShellForm.AppMenuName = "程序";

WorkItem.ShellForm.ExitString = "退出";

AddCustomModule();
}

private static void AddCustomModule()

{
ToolStripMenuItem _btnCutomMenuItem = new ToolStripMenuItem("自定义模块");

ToolStripItem _btnShowMyForm = new ToolStripButton("弹出");

_btnShowMyForm.Click += new EventHandler(ShowMyForm_Click);

_btnCutomMenuItem.DropDownItems.Add(_btnShowMyForm);

WorkItem.ShellForm.ShellMenu.Items.Add(_btnCutomMenuItem);
}

private static void ShowMyForm_Click(object sender, EventArgs e)

{
MyForm mForm = new MyForm();

mForm.MdiParent = WorkItem.ShellForm;

mForm.Show();
}

private static void AddServices()

{
IFormService service = WorkItem.Services.AddService<IFormService>("FormService", new FormService());
}
}
}
首先: 加载WorkItem 添加InitWorkItem() 方法,将Bob.Library 中的ShellForm 实例化。
然后加载 Service 和 模块
AddServices() 添加一个 Key 为 FormService 的 IFormService 实例,该实例在MyForm中有用到。
GetService
private void btnGetGUID_Click(object sender, EventArgs e)

{
IFormService service = WorkItem.Services.GetServiceByKey<IFormService>("FormService");
txtGUID.Text = service.GetGuidString();
}
AddModules() ,模拟的添加一个自定义模块,AddCustomModule(),为该模块添加独享的菜单,为该模块添加子菜单,
为子菜单绑定事件.
然后我们让程序开始Run 我们的 Shell Application.Run(WorkItem.ShellForm);