栈的特点是后进先出。
【头文件linkstack.h】
template<class T>class Linkstack;
template<class T>
class stacknode
{
friend class Linkstack<T>;
private:
T data;
stacknode<T> *next;
};
template<class T>
class Linkstack
{
public:
Linkstack():top(0){};
~Linkstack();
bool Isempty()const;
void push(T item);
void pop();
T Top()const;
void show();
private:
stacknode<T> *top;
};
template<class T>
bool Linkstack<T>::Isempty()const
{
return top==0;
}
template<class T>
void Linkstack<T>::push(T item)
{
stacknode<T> *p=new stacknode<T>();
cout<<item<<"进栈"<<endl;
p->data=item;
p->next=top;
top=p;
}
template<class T>
void Linkstack<T>::pop()
{
if(Isempty())
{
cout<<"栈为空"<<endl;
}
else
{
stacknode<T> *p=top;
cout<<top->data<<"出栈"<<endl;
top=top->next;
delete p;
}
}
template<class T>
T Linkstack<T>::Top()const
{
if(Isempty())
{
throw "栈为空";
}
else
{
return top->data;
}
}
template<class T>
void Linkstack<T>::show()
{
stacknode<T> *p=top;
if(Isempty())
{
cout<<"栈为空"<<endl;
}
else
{
cout<<"此时栈中元素为:";
while(p)
{
cout<<p->data<<" ";
p=p->next;
}
cout<<endl;
}
}
template<class T>
Linkstack<T>::~Linkstack()
{
while(!Isempty())
{
pop();
}
}
#endif
【主程序】
//main.cpp代码
#include "linkstack.h"
#include<iostream>
using namespace std
int main()
{
Linkstack<int> L
L.push(1)
L.push(2)
L.push(3)
L.push(4)
L.show()
cout<<"栈顶元素为:"<<L.Top()<<endl
L.pop()
cout<<"栈顶元素为:"<<L.Top()<<endl
L.push(5)
cout<<"栈顶元素为:"<<L.Top()<<endl
system("pause")
return 0
}
【结果图】
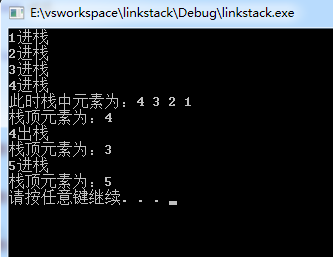