#include<iostream>
#define SIZE 50
using namespace std;
class Matrix
{
int a,b;
int **p = NULL;
public:
Matrix();
Matrix(int a,int b);
Matrix(const Matrix &m);//添加复制构造函数 实现深复制
~Matrix();
int getRows();
int getColumns();
int* operator[](int i);
const int* operator[](int i)const;//定义常成员函数 防止常对象不能访问数组元素
friend ostream &operator<<(ostream& out,const Matrix &m);
friend Matrix operator+(const Matrix &A,const Matrix &B);
friend Matrix operator*( Matrix &A, Matrix &B);
};
//必须以全局函数形式重载该符号
ostream &operator<<(ostream& out,const Matrix &m)
{
for(int i = 0;i < m.a ;i++)
{
for(int j = 0;j < m.b ;j++)
out<<m.p[i][j]<<" ";
cout<<endl;}
return out;
}
Matrix::Matrix()
{
a = 0;b = 0;
}
Matrix::Matrix(int a,int b)
{
this->a = a;
this->b = b;
p = new int *[50];//注意:二维数组动态内存分配 一维指针数组
for(int i = 0;i < a;i++)
{
p[i] = new int [b];
}
}
Matrix::Matrix(const Matrix &m)
{
this->b = m.b ;
this->a = m.a ;
p = new int *[a];//注意:一维指针数组
for(int i = 0;i < a;i++)
{
p[i] = new int [b];
}
}
Matrix::~Matrix()
{
for(int i = 0;i < a;i++)
delete []p[i];
delete p;//赋空
p = NULL;
}
int Matrix::getColumns()
{
return b;
}
int Matrix::getRows()
{
return a;
}
int* Matrix::operator[](int i)//注意形式
{
return p[i]; //注意here 二维数组
}
const int* Matrix::operator[](int i) const
{
return p[i];
}
Matrix operator+(const Matrix &A,const Matrix &B)
{
Matrix M(A.a,A.b);
for(int i = 0;i < A.a;i++ )
for(int j = 0;j < A.b;j++ )
M.p[i][j] = A.p[i][j] + B.p[i][j];
return M;
}
Matrix operator*( Matrix &A, Matrix &B)
{
Matrix M(A.a,B.b);
for(int i = 0;i < A.a; i++)
for(int j = 0;j < B.b;j++)
{
for(int k = 0; k < A.b;k++)
M.p[i][j] += A.p[i][k]* B.p[k][j];
}
return M;
}
int main(){
int a,b;
cin>>a>>b;
Matrix m1(a,b),m2(a,b),m3(b,a);
int i,j;
for(i=0;i<m1.getRows();i++)
for(j=0;j<m1.getColumns();j++)
cin>>m1[i][j];
for(i=0;i<m2.getRows();i++)
for(j=0;j<m2.getColumns();j++)
cin>>m2[i][j];
for(i=0;i<m3.getRows();i++)
for(j=0;j<m3.getColumns();j++)
cin>>m3[i][j];
cout<<"Matrix M1:"<<endl;
cout<<m1;
cout<<"Matrix M2:"<<endl;
cout<<m2;
cout<<"Matrix M3:"<<endl;
cout<<m3;
cout<<"Result of Matrix Addition:"<<endl;
cout<<m1+m2;
cout<<"Result of Matrix Multiplication:"<<endl;
cout<<m1*m3;
return 0;
}
运算符重载,数组内存动态分配,重载实现深复制
最新推荐文章于 2025-03-11 23:44:00 发布
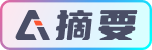