arp协议_arp请求_arp回应
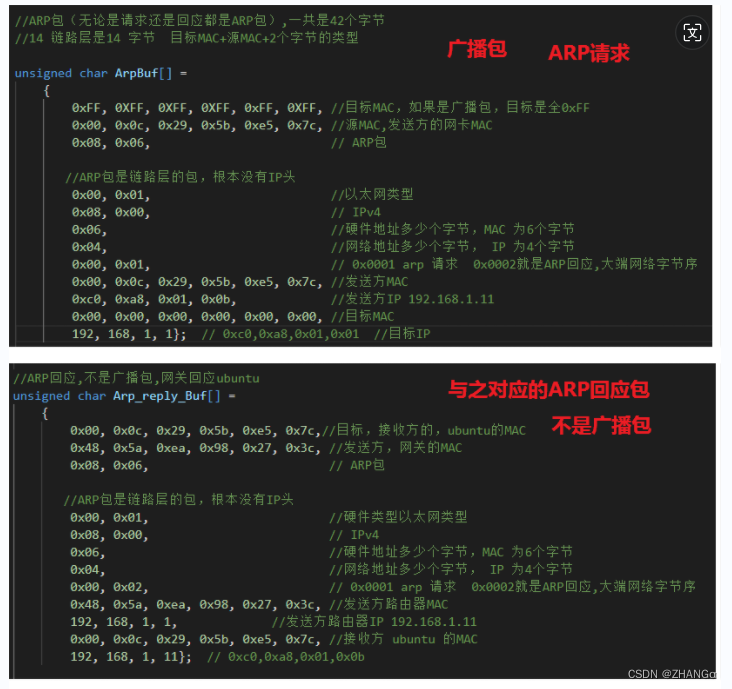
ICMP包构造ping搜狐服务器参考
#include <stdio.h>
#include <sys/types.h> /* See NOTES */
#include <sys/socket.h>
#include <linux/if_packet.h>
#include <linux/if_ether.h>
#include <string.h>
#include <net/if.h>
#include <sys/ioctl.h>
#include <pthread.h>
unsigned short in_cksum(unsigned short *addr, int len)
{
unsigned int sum = 0, nleft = len;
unsigned short answer = 0;
unsigned short *w = addr;
while (nleft > 1) {
sum += *w++;
nleft -= 2;
}
if (nleft == 1) {
*(u_char *) (&answer) = *(u_char *) w;
sum += answer;
}
sum = (sum >> 16) + (sum & 0xffff);//将高16bit与低16bit相加
sum += (sum >> 16);//将进位到高位的16bit与低16bit 再相加
answer = (unsigned short)(~sum);
return (answer);
}
unsigned char ICMPBuf[]=
{
//===链路层
0x48, 0x5a, 0xea, 0x98, 0x27, 0x3c, //目标MAC为网关的MAC
0x00, 0x0c, 0x29, 0x5b, 0xe5, 0x7c, //ubuntu源MAC
0x08, 0x00, // ICMP协议是属于IP包
//===IP层或者说 网络层
0x45,//4代表IPV4 5代表IP头(5*4=20个字节)
0x00,//服务类型,可以反映当前主机一些状态信息,可以直接填0
0,0,//IP包的长度,也不是整个包字节-链路层14个字节?
0x00,0x00,0x40,0x00,//数据包标示编号设置为0,数据包不支持分片功能
64,//默认TTL time to live 64最多可以过64个路由器
1,//ICMP协议
0,0,//IP包头的20个字节校验和
192,168,1,11,//源IP,发送方ubuntu的IP
47,91,20,194,//目标IP,搜狐服务器IP
//ICMP请求内容
0x08,0x00,//为ICMP请求包,如果是0x00,0x00,是回应
0x00,0x00,//ICMP报文校验
0,0,//通常填大端的getpid(),也可以填默认0
0x00,0x2b,//发送的包编号 43,可以随意填,回应包编号和发送包一样
// 0x00,0x00,0x00,0x00,0x00,0x00,0x00,0x00, 时间戳 8个字节可加也可不加
'1','2','3','4','5',//数据内容可以任意
'h','e','l','l','o'
};
192.168.18.10
192.168.59
192.168.18.10
192.168.18+100.10
void *myfun(void *arg)
{
int sockfd = (int)arg;
unsigned char recvbuf[100] = {0};
while (1)
{
int recvlen = recvfrom(sockfd, recvbuf, sizeof(recvbuf), 0, NULL, NULL);
if ((recvbuf[12] == 0x08) && (recvbuf[13] == 0x00))
{//IP包
if (recvbuf[23] == 0x01)
{//ICMP协议
if((recvbuf[34] == 0x00)&&(recvbuf[35] == 0x00))
{//回应
printf("hjs收到搜狐网服务器ICMP回应包:TTL=%d,经过%d个路由器\n",recvbuf[22],64-recvbuf[22]);
}
}
}
}
retursn NULL;
}
int main()
{
unsigned char data[]={0x45,0x00,0x00,0x54,0x00,0x00,0x40,0x00,0x40,0x01,\
0,0,0xc0,0xa8,0x01,0x0b,0x2f,0x5b,0x14,0xc2};
printf("%#x\n",htons(in_cksum(data,20)));
//设置包编识
unsigned short mypid=getpid();
// *(unsigned short *)(ICMPBuf+38)=htons(mypid);
//填入IP包长度
*(unsigned short *)(ICMPBuf+16)=htons(sizeof(ICMPBuf)-14);
//IP包头校验
*(unsigned short *)(ICMPBuf+24)=in_cksum(ICMPBuf+14,20);//htons(in_cksum(ICMPBuf+14,20));
//ICMP部分校验
unsigned short icmplen=sizeof(ICMPBuf)-34;
*(unsigned short *)(ICMPBuf+36)=in_cksum(ICMPBuf+34,sizeof(ICMPBuf)-34);//htons(in_cksum(ICMPBuf+34,sizeof(ICMPBuf)-34));
int myfd = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
struct sockaddr_ll mysocket;
struct ifreq ethreq;
strcpy(ethreq.ifr_ifrn.ifrn_name, "eth0");
ioctl(myfd, SIOCGIFINDEX, ðreq);
memset(&mysocket, 0, sizeof(mysocket));
mysocket.sll_ifindex = ethreq.ifr_ifru.ifru_ivalue; //网卡信息的首地址
pthread_t thread;
pthread_create(&thread, NULL, myfun,(void *)myfd);
pthread_detach(thread);
printf("myfd=%d\n", myfd);
int var = sendto(myfd, ICMPBuf, sizeof(ICMPBuf), 0, (struct sockaddr *)&mysocket, sizeof(mysocket));
sleep(2);
close(myfd);
}
icmp协议_请求和回应 (1)
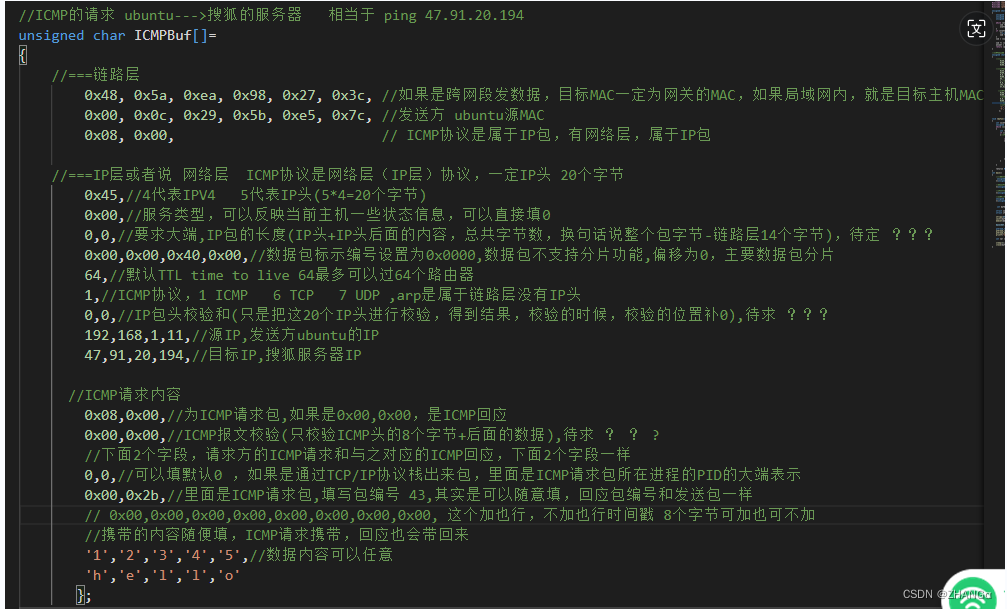
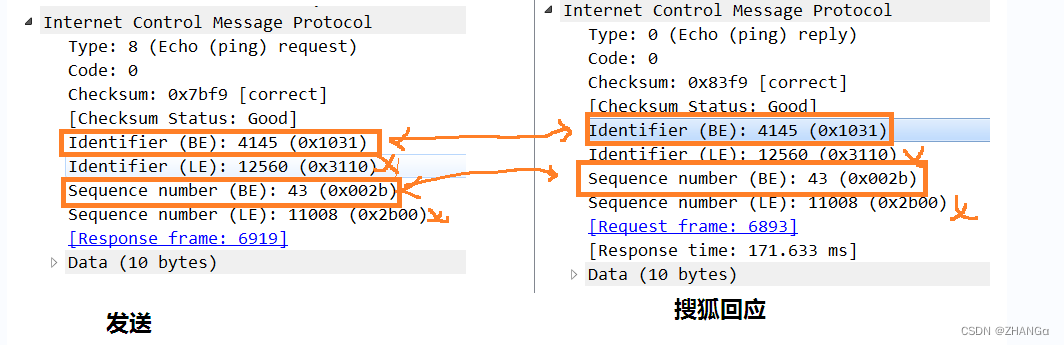
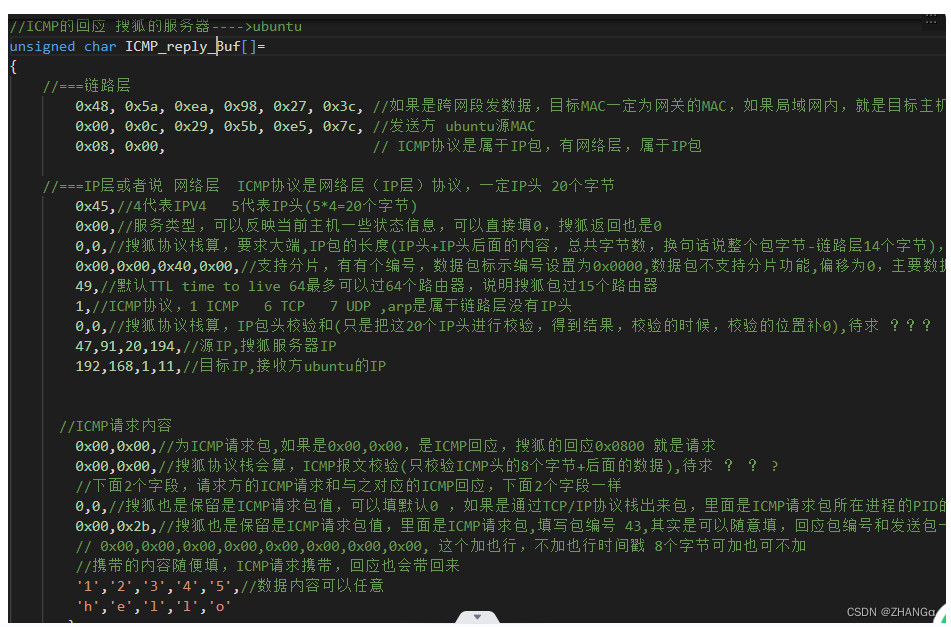
扫描局域网主机MAC和IP参考
#include <stdio.h>
#include <sys/types.h> /* See NOTES */
#include <sys/socket.h>
#include <linux/if_packet.h>
#include <linux/if_ether.h>
#include <string.h>
#include <net/if.h>
#include <sys/ioctl.h>
#include <pthread.h>
unsigned char ArpBuf[] =
{
0xFF, 0XFF, 0XFF, 0XFF, 0xFF, 0XFF, //目标MAC,广播
0x00, 0x0c, 0x29, 0x5b, 0xe5, 0x7c, //源MAC,当前主机ARP请求包发出网卡的MAC
0x08, 0x06, // ARP包
0x00, 0x01, //以太网类型
0x08, 0x00, // IPv4
0x06, //硬件地址MAC 为6个字节
0x04, //网络地址 IP 为4个字节
0x00, 0x01, // arp 请求
0x00, 0x0c, 0x29, 0x5b, 0xe5, 0x7c, //发送方MAC
0xc0, 0xa8, 0x01, 0x0b, //发送方IP 192.168.1.11
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, //目标MAC
192, 168, 1, 0};//最后一个字节待定
void *myfun(void *arg)
{
int sockfd = (int)arg;
unsigned char recvbuf[100] = {0};
while (1)
{
int recvlen = recvfrom(sockfd, recvbuf, sizeof(recvbuf), 0, NULL, NULL);
if ((recvbuf[12] == 0x08) && (recvbuf[13] == 0x06))
{//ARP 包
if ((recvbuf[20] == 0x00) && (recvbuf[21] == 0x02))
{//ARP 回应
printf("MAC: %02x:%02x:%02x:%02x:%02x:%02x===>",
recvbuf[22], recvbuf[23], recvbuf[24], recvbuf[25], recvbuf[26], recvbuf[27]);
printf("IP: %d.%d.%d.%d\n",\
recvbuf[28], recvbuf[29], recvbuf[30], recvbuf[31]);
}
}
// printf("recvlen=%d\n", recvlen);
}
retursn NULL;
}
int main()
{
int myfd = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ALL));//创建一个原始套接字
struct sockaddr_ll mysocket;
struct ifreq ethreq;
strcpy(ethreq.ifr_ifrn.ifrn_name, "eth0");//设置属性,等下sendto就从 eth0网卡发送出去数据包
ioctl(myfd, SIOCGIFINDEX, ðreq);
memset(&mysocket, 0, sizeof(mysocket));
mysocket.sll_ifindex = ethreq.ifr_ifru.ifru_ivalue; //网卡信息的首地址
pthread_t thread;
pthread_create(&thread, NULL, myfun,(void *)myfd);
pthread_detach(thread);
printf("myfd=%d\n", myfd);
int cnt=1;
while(cnt < 255)
{
ArpBuf[41]=cnt;
int var = sendto(myfd, ArpBuf, sizeof(ArpBuf), 0, (struct sockaddr *)&mysocket, sizeof(mysocket));
cnt++;
}
sleep(2);
close(myfd);
}
原始套接字构造ARP请求包获取网关的MAC
//使用原始套接字,拿到当前局域网内,所有主机的IP和MAC
// #include <stdio.h>
// #include <sys/types.h> /* See NOTES */
// #include <sys/socket.h>
// #include <linux/if_ether.h> //ETH_P_ALL
// #include <net/if.h>
// #include <sys/ioctl.h>
// #include <linux/if_packet.h> //struct sockaddr_ll
// #include<string.h>
// #include<stdlib.h>
#include <stdio.h>
#include <sys/types.h> /* See NOTES */
#include <sys/socket.h>
#include <linux/if_packet.h>
#include <linux/if_ether.h>
#include <string.h>
#include <net/if.h>
#include <sys/ioctl.h>
// unsigned char ArpBuf[] = {
// 0xFF, 0XFF, 0XFF, 0XFF, 0XFF, 0XFF, //目标MAC,ARP广播地址
// 0x00, 0x0c, 0x29, 0x5b, 0xe5, 0x7c, //源MAC,虚拟机ubuntu的MAC
// 0x08, 0x06, //是ARP包
// 0x00, 0x01, //是以太网类型帧
// 0x08, 0x00, //是IPV4协议
// 0x06, //硬件地址MAC是6个字节
// 0x04, //网络地址IP是4个字节
// 0x00, 0x01, //这是一个ARP请求包
// 0x00, 0x0c, 0x29, 0x5b, 0xe5, 0x7c, //源MAC
// 192, 168, 1, 11, //源IP
// 0x00, 0x00, 0x00, 0x00, 0x00, 0x00,
// 192, 168, 1, 1 //目标的IP
// }; //其实一个ARP包是48个字节
// // 17 9 7 8 1
unsigned char ArpBuf[] =
{
0xFF, 0XFF, 0XFF, 0XFF, 0xFF, 0XFF, //目标MAC
0x00, 0x0c, 0x29, 0x5b, 0xe5, 0x7c, //源MAC
0x08, 0x06, // ARP包
0x00, 0x01, //以太网类型
0x08, 0x00, // IPv4
0x06, //硬件地址MAC 为6个字节
0x04, //网络地址 IP 为4个字节
0x00, 0x01, // arp 请求
0x00, 0x0c, 0x29, 0x5b, 0xe5, 0x7c, //发送方MAC
0xc0, 0xa8, 0x01, 0x0b, //发送方IP 192.168.1.11
0x00, 0x00, 0x00, 0x00, 0x00, 0x00, //目标MAC
// 0xc0,0xa8,0x01,0x09 //目标IP
192, 168, 1, 1};
int main()
{
// 192.168.1.0 组网,主机IP 192.168.1.1~192.168.1.254
//手动构造192.168.1.1~192.168.1.254 的ARP请求包,在子网发送,拿到所有的
// ARP回应包。回应包 IP和MAC提取出来打印出来。
//经过扫描就可以拿到当前局域里所有的主机IP和MAC
//创建原始套接字,补充协议需要主机字节序转网络字节序
// int myfd = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
// //指定从哪一个网卡发送出去?
// //要获取一个个网卡 eth0
// struct ifreq myeth;
// memset(&myeth,0,sizeof(myeth));
// strcpy(myeth.ifr_ifrn.ifrn_name, "eth0"); //填入网卡名字
// ioctl(myfd, SIOCGIFINDEX, &myeth); //获取网卡名为 eth0的信息,并填入到myeth
// struct sockaddr_ll sll;
// bzero(&sll,sizeof(sll));
// sll.sll_ifindex = myeth.ifr_ifru.ifru_ivalue; //网卡信息的首地址
// int ret = sendto(myfd, ArpBuf, sizeof(ArpBuf), 0, (struct sockaddr *)&sll, sizeof(struct sockaddr)); //把ARP封包发送出去
int myfd = socket(AF_PACKET, SOCK_RAW, htons(ETH_P_ALL));
struct sockaddr_ll mysocket;
struct ifreq ethreq;
// IFNAMSIZ
// # define ifr_name ifr_ifrn.ifrn_name
// strcpy(ethreq.ifr_name,"eth0");仅仅是一个宏定义
strcpy(ethreq.ifr_ifrn.ifrn_name, "eth0");
ioctl(myfd, SIOCGIFINDEX, ðreq);
memset(&mysocket, 0, sizeof(mysocket));
mysocket.sll_ifindex = ethreq.ifr_ifru.ifru_ivalue; //网卡信息的首地址
int var = sendto(myfd, ArpBuf, sizeof(ArpBuf), 0, (struct sockaddr *)&mysocket, sizeof(mysocket));
printf("myfd=%d,ret=%d\n", myfd, var);
unsigned char recvbuf[100] = {0};
int recvlen = recvfrom(myfd, recvbuf, sizeof(recvbuf), 0, NULL, NULL);
printf("recvlen=%d\n", recvlen);
int i = 0;
recvlen = 42;
while (i < recvlen)
{
if ((i % 10 == 0) && (i != 0))
{ //一行如果有10个字节,就换行打印
printf("\n");
}
printf("%x ", recvbuf[i]);
i++;
}
if ((recvbuf[12] == 0x08) && (recvbuf[13] == 0x06))
{
printf("这是ARP包!!\n");
if ((recvbuf[20] == 0x00) && (recvbuf[21] == 0x02))
{
printf("这是回应包!!\n");
printf("网关的MAC: %02x:%02x:%02x:%02x:%02x:%02x\n",\
recvbuf[6], recvbuf[7], recvbuf[8], recvbuf[9], recvbuf[10], recvbuf[11]);
}
}
close(myfd);
}