package com.js;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringJsCrudApplication {
public static void main(String[] args) {
SpringApplication.run(SpringJsCrudApplication.class, args);
}
}
package com.js;
import java.util.List;
import javax.servlet.http.HttpSession;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.jpa.repository.query.Procedure;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.CrossOrigin;
import org.springframework.web.bind.annotation.DeleteMapping;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PathVariable;
//import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.PutMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.RestController;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.js.beans.NoteTaker;
import com.js.service.NoteDao;
@RestController
public class HomeJsController {
@Autowired
private NoteDao dao;
// @RequestMapping(path = "/add", method = RequestMethod.POST, consumes = {"application/json"})
@CrossOrigin
@PostMapping("/add")
public void save(@RequestBody NoteTaker note) {
System.out.println("Inserting");
dao.saveNote(note);
}
@CrossOrigin
@GetMapping("/show")
public List<NoteTaker> showAll(){
List<NoteTaker> list = dao.getAllNote();
// System.out.println(list);
return list;
}
@CrossOrigin
@DeleteMapping("/delete/{id}")
public void deleteData(@PathVariable("id") long id) {
String row = dao.deleteRow(id);
// System.out.println(row);
}
@CrossOrigin
@GetMapping("/user/{id}")
public NoteTaker getSingleNote(@PathVariable("id") long id) {
NoteTaker noteTaker = dao.getNoteById(id);
// System.out.println(noteTaker);
return noteTaker;
}
@CrossOrigin
@PutMapping("/update")
public void updateUser(@RequestBody NoteTaker note) {
System.out.println("Updating"+note.getnId());
NoteTaker newNote = new NoteTaker(note.getnId(), note.getTopicName(), note.getNote());
dao.updateNote(newNote);
}
}
package com.js.beans;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import com.fasterxml.jackson.annotation.JsonFormat;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
@Entity
public class NoteTaker {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private long nId;
private String topicName;
@Column(columnDefinition = "TEXT(3000)")
private String note;
public long getnId() {
return nId;
}
public void setnId(long nId) {
this.nId = nId;
}
public String getTopicName() {
return topicName;
}
public void setTopicName(String topicName) {
this.topicName = topicName;
}
public String getNote() {
return note;
}
public void setNote(String note) {
this.note = note;
}
public NoteTaker(long nId, String topicName, String note) {
super();
this.nId = nId;
this.topicName = topicName;
this.note = note;
}
public NoteTaker(String topicName, String note) {
super();
this.topicName = topicName;
this.note = note;
}
public NoteTaker() {
super();
// TODO Auto-generated constructor stub
}
@Override
public String toString() {
return "NoteTaker [nId=" + nId + ", topicName=" + topicName + ", note=" + note + "]";
}
}
package com.js.repo;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import com.js.beans.NoteTaker;
@Repository
public interface NoteRepo extends JpaRepository<NoteTaker, Long> {
}
package com.js.service;
import java.util.List;
import java.util.Optional;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import com.js.beans.NoteTaker;
import com.js.repo.NoteRepo;
@Service
public class NoteDao {
@Autowired
private NoteRepo repo;
public int saveNote(NoteTaker note) {
repo.save(note);
return 1;
}
public int updateNote(NoteTaker note) {
repo.save(note);
return 2;
}
public List<NoteTaker> getAllNote() {
List<NoteTaker> list = repo.findAll();
return list;
}
public String deleteRow(long id) {
repo.deleteById(id);
return "Success";
}
public NoteTaker getNoteById(long id) {
Optional<NoteTaker> optional = repo.findById(id);
NoteTaker noteTaker = optional.get();
return noteTaker;
}
}
spring.jpa.hibernate.ddl-auto=update
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.3</version>
<relativePath /> <!-- lookup parent from repository -->
</parent>
<groupId>com.spring.js</groupId>
<artifactId>Spring-Js-CRUD</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>Spring-Js-CRUD</name>
<description>spring boot and and bus experiment</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Spring-Js Crud</title>
<link rel="stylesheet" href="/css/bootstrap.css">
<link rel="stylesheet" href="/css/all.css">
<link rel="stylesheet" href="/css/Js-Spring-CRUD.css">
</head>
<body>
<header class="bg-primary">
<h1 class="text-white">CRUD</h1>
<button type="button" class="btn btn-danger" data-bs-toggle="modal" data-bs-target="#exampleModal"
id="open-model" onclick="clearField()">
Create note
</button>
</header>
<main class="pt-3">
<div class="container">
<div class="row">
<div class="col-sm">
<!-- Modal -->
<div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel"
aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Notes</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal"
aria-label="Close"></button>
</div>
<div class="modal-body">
<form id="myForm" class="insert">
<div class="mb-3">
<label class="form-label">Topic name</label>
<input type="text" class="form-control" id="form-name"
aria-describedby="emailHelp" name="topicName">
</div>
<div>
<label class="form-label">write notes</label>
<textarea class="form-control" placeholder="Leave a comment here"
style="height: 100px" name="note" id="form-note"></textarea>
</div>
<br>
<button type="submit" class="btn btn-primary" id="submit-form">Submit</button>
</form>
</div>
</div>
</div>
</div>
<div class="modal fade" id="exampleModals" tabindex="-1" aria-labelledby="exampleModalLabel"
aria-hidden="true">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title" id="exampleModalLabel">Update</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal"
aria-label="Close"></button>
</div>
<div class="modal-body">
<form id="myForm" class="update">
<input type="text" class="form-control" id="form-ids" hidden="hidden"
name="nId">
<div class="mb-3">
<label class="form-label">Topic name</label>
<input type="text" class="form-control" id="form-names"
aria-describedby="emailHelp" name="topicName">
</div>
<div>
<label class="form-label">write notes</label>
<textarea class="form-control" placeholder="Leave a comment here"
style="height: 100px" name="note" id="form-notes"></textarea>
</div>
<br>
<button type="submit" class="btn btn-primary" id="submit-form">Submit</button>
</form>
</div>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="container">
<button class="btn btn-success" id="load-data">refresh</button>
<div class="row" id="put-row">
</div>
</div>
</main>
<script src="/js/bootstrap.bundle.js"></script>
<script src="/js/Js-Spring-CRUD.js"></script>
<!-- <script src="https://code.jquery.com/jquery-3.6.0.min.js"
integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous"></script> -->
<script>
</script>
</body>
</html>
// Show all Data========================================
const cardRow = document.getElementById("put-row");
function showAllCards() {
var xhr = new XMLHttpRequest();
xhr.open('GET', 'http://localhost:8080/show', true);
xhr.onload = function () {
if (this.status == 200) {
let allData = JSON.parse(this.responseText);
var row = "";
for (var i = 0; i < allData.length; i++) {
row += '<div class="col-12 col-md-6 col-lg-3 mb-3"><div class="card">'
+ '<div class="card-body">'
+ '<h5 class="card-title" style="border-bottom: 1px solid gray">' + allData[i].topicName + '</h5>'
+ '<p class="card-text" onload="minimizeText()" id="words" style="height: 120px; overflow: hidden;">'
+ allData[i].note
+ '</p>'
+ '<button class="btn btn-primary" value="' + allData[i].nId + '" id="edit-btn" onclick="getUser(this.value)" data-bs-toggle="modal" data-bs-target="#exampleModals"><i class="fa-solid fa-pen-to-square"></i></button> '
+ '<button class="btn btn-danger delete-btn" value="' + allData[i].nId + '" id="delete-btn" onclick="deleteUser(this.value)"><i class="fa-solid fa-trash"></i></button>'
+ '</div>'
+ '</div></div>'
cardRow.innerHTML = row;
}
}
}
xhr.send();
};
// setInterval(showAllCards, 2000);
showAllCards();
const refresh = document.getElementById("load-data");
refresh.onclick = function () {
showAllCards();
};
// Insert Data in Page===========================================================
document.querySelector(".insert").addEventListener('submit', (e) => {
e.preventDefault();
var xhr = new XMLHttpRequest();
var name = document.getElementById('form-name').value;
var notes = document.getElementById('form-note').value;
xhr.open('POST', 'http://localhost:8080/add', true);
xhr.setRequestHeader('Content-type', 'application/json');
xhr.onload = function () {
if (this.status == 200) {
showAllCards();
}
}
var param = {
topicName: name,
note: notes
}
var params = JSON.stringify(param);
xhr.send(params);
clearField();
});
function clearField() {
var name = document.getElementById('form-name').value = '';
var notes = document.getElementById('form-note').value = '';
}
function clearField() {
var name = document.getElementById('form-names').value = '';
var notes = document.getElementById('form-notes').value = '';
var ids = document.getElementById('form-ids').value = '';
}
// Delete User operation=====================================================
function deleteUser(el) {
var order = confirm("Are you sure!!!");
if (order == true) {
var xhr = new XMLHttpRequest();
xhr.open('DELETE', 'http://localhost:8080/delete/' + el, true);
xhr.onload = function () {
if (this.status == 200) {
showAllCards();
}
}
xhr.send();
}
}
// Get single User By Id===========================================
function getUser(elId) {
var xhr = new XMLHttpRequest();
xhr.open('GET', 'http://localhost:8080/user/' + elId, true);
xhr.onload = function () {
if (this.status == 200) {
var jsObject = JSON.parse(this.responseText);
console.log(jsObject);
var myName = document.getElementById('form-names').value = jsObject.topicName;
var myNotes = document.getElementById('form-notes').value = jsObject.note;
var myIds = document.getElementById('form-ids').value = jsObject.nId;
}
}
xhr.send();
}
// Update Single single User By Id===========================================
document.querySelector(".update").addEventListener('submit', (e) => {
e.preventDefault();
var xhr = new XMLHttpRequest();
var updateName = document.getElementById('form-names').value;
var updateNotes = document.getElementById('form-notes').value;
var updateId = document.getElementById('form-ids').value
xhr.open('PUT', 'http://localhost:8080/update', true);
xhr.setRequestHeader('Content-type', 'application/json');
xhr.onload = function () {
if (this.status == 200) {
showAllCards();
}
}
var param = {
nId: updateId,
topicName: updateName,
note: updateNotes
}
var params = JSON.stringify(param);
console.log(params);
xhr.send(params);
clearField();
});
// Minimize Text area===================================
function minimizeText(){
const para = document.querySelectorAll("#words")
for (var i = 0; i < para.length; i++) {
var strings = para[i].innerText;
// console.log(strings);
var result = add3Dots(strings, 13);
para[i].innerText = result;
}
}
function add3Dots(string, limit) {
var dots = "...";
if (string.length > limit) {
// you can also use substr instead of substring
string = string.substring(0, limit) + dots;
}
return string;
}
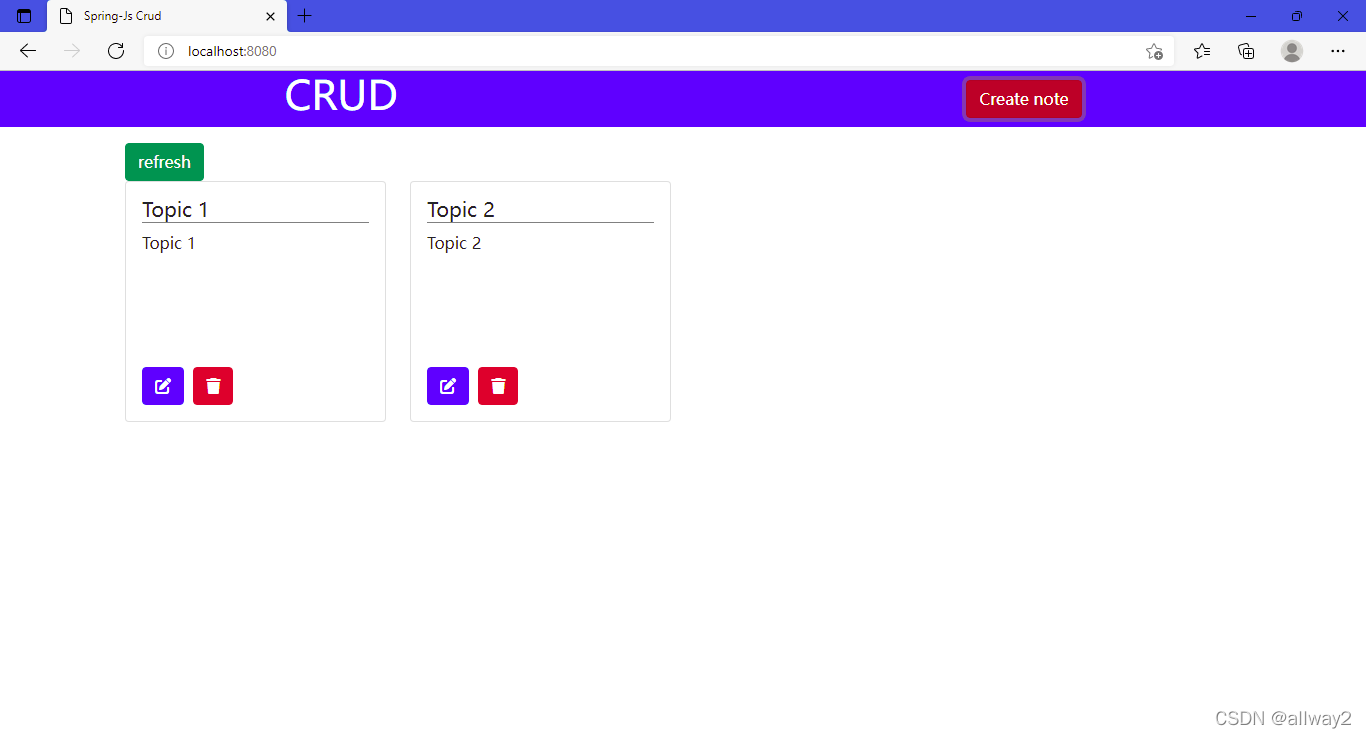
GitHub - vishal1605/Spring-boot-Vanilla-JavaScript-Ajax-CRUD: Spring boot as Backend and Vanilla JavaScript Ajax as Frontend CRUD operation without jQuery