1.请你尝试编写calloc函数,函数内部使用malloc函数来获取内存。
#include <stdlib.h>
void *calloc(size_t nmemb, size_t size)
{
char *ptr;
int i;
ptr = malloc(nmemb * size);
if(ptr == NULL)
return NULL;
for(i = 0; i < nmemb; i++){
ptr[i] = 0;
}
return ptr;
}
2.编写一个函数,从标准输入读取一列整数,把这些值存储于一个动态分配的数组中并返回这个数组,函数通过观察EOF判断输入是否结束,数组的第一个数是数组包含的值的个数,它的后面就是这些整数值。
#include <stdio.h>
#include <stdlib.h>
#define DELTA 100
int *read()
{
int *array;
int count;
int size;
int value;
size = DELTA;
count = 0;
array = malloc((size + 1) * sizeof(int));
if(array == NULL)
return NULL;
while((scanf("%d",&value)) == 1){
count++;
if(count >= size){
size += DELTA;
array = realloc(array,(size + 1) * sizeof(int));
if(array == NULL)
return NULL;
}
array[count] = value;
}
if(count < size){
array = realloc(array,(count + 1) * sizeof(int));
if(array == NULL)
return NULL;
}
array[0] = count;
return array;
}
3.编写一个函数,从标准输入读取一个字符串,把字符串复制到动态内存分配的内存中,并返回该字符串的拷贝,这个函数不应该对读入字符串的长度作任何限制!
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define SIZE 100
char *getstr(void);
int main()
{
char *string = getstr();
printf("%s\n", string);
free(string);
return EXIT_SUCCESS;
}
char *getstr(void)
{
int ch;
char *strsave;
char *strcopy;
int count = 0;
int size = SIZE + 1;
strsave = malloc(size);
if(strsave == NULL)
return NULL;
while((ch = getchar()) != EOF && ch != '\n'){
strsave[count] = ch;
if((count + 1) > size){
size += SIZE;
strsave = realloc(strsave - count, size);
if(strsave == NULL){
return NULL;
}
strsave = strsave + count;
}
count++;
}
strsave[count] = '\0';
strcopy = malloc(count);
strcpy(strcopy, strsave);
printf("%s\n", strsave);
free(strsave);
return strcopy;
}
4.编写一个程序,按照下图中的样子创建数据结构,最后三个对象都是动态分配的结构。第一个对象则可能是一个静态的指向结构的指针。你不必使这个程序过于全面–我们将在下一章讨论这个结构。
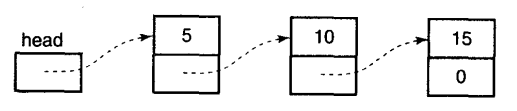
#include <stdio.h>
#include <stdlib.h>
typedef struct NODE {
int value;
struct NODE *link;
} Node;
Node *put_node(int value);
int main()
{
Node *head;
head = put_node(5);
head->link = put_node(10);
head->link->link = put_node(15);
head->link->link->link = NULL;
return EXIT_SUCCESS;
}
Node *put_node(int value)
{
Node *node;
node = (Node *)malloc(sizeof(Node));
if(node != NULL)
node->value = value;
return node;
}