12.3 多线程应用
本节中通过实现一个多线程的网络时间服务器,介绍如何综合运用多线程技术编程。每当有客户请求到达时,服务器将启动一个新线程为它返回当前的时间,服务完毕后,这个线程将自动退出。同时,用户界面会显示当前已接收请求的次数。
12.3.1 【实例】:服务器编程
【例】(难度中等)(CH1204)服务器编程。
首先,建立服务器端工程“TimeServer.pro”。文件代码如下:
(1)在头文件“dialog.h”中,定义服务器端界面类Dialog继承自QDialog类,其具体代码如下:
#include <QDialog>
#include <QLabel>
#include <QPushButton>
class Dialog : public QDialog
{
Q_OBJECT
public:
Dialog(QWidget *parent = 0);
~Dialog();
private:
QLabel *Label1; //此标签用于显示监听端口
QLabel *Label2; //此标签用于显示请求次数
QPushButton *quitBtn; //退出按钮
};
(2)在源文件“dialog.cpp”中,Dialog类的构造函数完成了初始化界面,其具体代码如下:
#include "dialog.h"
#include <QHBoxLayout>
#include <QVBoxLayout>
#pragma execution_character_set("utf-8")
Dialog::Dialog(QWidget *parent)
: QDialog(parent)
{
setWindowTitle(tr("多线程时间服务器"));
Label1 = new QLabel(tr("服务器端口:"));
Label2 = new QLabel;
quitBtn = new QPushButton(tr("退出"));
QHBoxLayout *BtnLayout = new QHBoxLayout;
BtnLayout->addStretch(1);
BtnLayout->addWidget(quitBtn);
BtnLayout->addStretch(1);
QVBoxLayout *mainLayout = new QVBoxLayout(this);
mainLayout->addWidget(Label1);
mainLayout->addWidget(Label2);
mainLayout->addLayout(BtnLayout);
connect(quitBtn, SIGNAL(clicked()), this, SLOT(close()));
}
(3)此时运行服务器端工程“TimeServer.pro”,界面显示如图12.5所示。
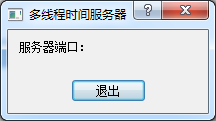
(4)在服务器端工程“TimeServer.pro”中,添加C++ Class文件“timethread.h”及“timethread.cpp”。在头文件“timethread.h”中,工作线程TimeThread类继承自QThread类,实现TCP套接字,其具体代码如下:
#include <QThread>
#include <QtNetwork>
#include <QTcpSocket>
class TimeThread : public QThread
{
Q_OBJECT
public:
TimeThread(int socketDescriptor, QObject *parent = 0);
void run(); //重写此虚函数
signals:
void error(QTcpSocket::SocketError socketError); // 出错信号
private:
int socketDescriptor; //套接字描述符
};
(5)在源文件“timethread.cpp”中,TimeThread类的构造函数只是初始化了套接字描述符,其具体代码如下:
#include "timethread.h"
#include <QDateTime>
#include <QByteArray>
#include <QDataStream>
TimeThread::TimeThread(int socketDescriptor, QObject *parent)
: QThread(parent), socketDescriptor(socketDescriptor)
{
}
TimeThread::run()函数是工作线程(TimeThread)的实质所在,当在TimeServer::incomingConnection()函数中调用了thread->start()函数后,此虚函数开始执行,其具体代码如下:
void TimeThread::run()
{
QTcpSocket tcpSocket; //创建一个QTcpSocket类
if (!tcpSocket.setSocketDescriptor(socketDescriptor))//(a)
{
emit error(tcpSocket.error()); //(b)
return;
}
QByteArray block;
QDataStream out(&block, QIODevice::WriteOnly);
out.setVersion(QDataStream::Qt_5_8);
uint time2u = QDateTime::currentDateTime().toTime_t()(); //(c)
out << time2u;
tcpSocket.write(block); //将获得的当前时间传回客户端
tcpSocket.disconnectFromHost();(); //断开连接
tcpSocket.waitForDisconnected(); //等待返回
}
(a) tcpSocket.setSocketDescriptor(socketDescriptor):将以上创建的QTcpSocket类置以从构造函数中传入的套接字描述符,用于向客户端传回服务器端的当前时间。
(b) emit error(tcpSocket.error()):如果出错,则发出error(tcpSocket.error())信号报告错误。
(c) uint time2u = QDateTime::currentDateTime().toTime_t():如果不出错,则开始获取当前时间。
此处需要注意的是时间数据的传输格式,Qt虽然可以很方便地通过QDateTime类的静态函数currentDateTime()获取一个时间对象,但类结构是无法直接在网络上传输的,此时需要将它转换为一个标准的数据类型后再传输。而QDateTime类提供了uint toTime_t() const函数,这个函数返回当前自1970-01-01 00:00:00(UNIX纪元)经过了多少Time类的void setTime_t(uint seconds)将这个时间还原。
(6)在服务器端工程“TimeServer.pro”中添加了C++ Class文件“timeserver.h”及“timeserver.cpp”。在头文件“timeserver.h”中,实现一个TCP服务器端,类TimeServer继承自QTcpServer类,其具体代码如下:
#include <QTcpServer>
class Dialog; //服务器端的声明
class TimeServer : public QTcpServer
{
Q_OBJECT
public:
TimeServer(QObject *parent = 0);
protected:
void incomingConnection(int socketDescriptor); //(a)
private:
Dialog *dlg; //(b)
};
(a) void incommingConnection(int socketDescriptor):重写此虚函数。这个函数在TCP服务器端有新的连接时被调用,其参数为所接收新连接的套接字描述符。
(b) Dialog *dlg:用于记录创建这个TCP服务器端对象的父类,这里是界面指针,通过这个指针将线程发出的消息关联到界面的槽函数中。
(7)在源文件“timeserver.cpp”中,构造函数只是用传入的父类指针parent初始化私有变量dlg,其具体代码如下:
#include "timeserver.h"
#include "timethread.h"
#include "dialog.h"
TimeServer::TimeServer(QObject *parent)
: QTcpServer(parent)
{
dlg = (Dialog *)parent;
}
重写的虚函数incomingConnection()的具体代码如下:
void TimeServer::incomingConnection(int socketDescriptor)
{
TimeThread *thread = new TimeThread(socketDescriptor, 0); //(a)
connect(thread, SIGNAL(finished()), dlg, SLOT(slotShow())); //(b)
connect(thread, SIGNAL(finished()), thread, SLOT(deleteLater()),
Qt::DirectConnection); //(c)
thread->start(); //(d)
}
(a) TimeThread *thread = new TimeThread(socketDescriptor, 0):以返回的套接字描述符socketDescriptor创建一个工作线程TimeThread。
(b) connect(thread, SIGNAL(finished()), dlg, SLOT(slotShow()):将上述创建的线程结束消息函数finished()关联到槽函数slotShow()用于显示请求计数。此操作中,因为信号是跨线程的,所以使用了排队连接方式。
(c) connect(thread, SIGNAL(finished()), thread, SLOT(deleteLater()), Qt::DirectConnection):将上述创建的线程结束消息函数finished()关联到线程自身的槽函数deleteLater()用于结束线程。此操作中,因为信号是在同一个线程中的,所以使用了直接连接方式,故最后一个参数可以省略而使用Qt的自动连接选择方式。
另外,由于工作线程中存在网络事件,所以不能被外界线程销毁,这里使用了延迟销毁操作deleteLater()保证由工作线程自身销毁。
(d) thread->start():启动上述创建的线程。执行此语句后,工作线程(TimeThread)的虚函数run()开始执行。
(8)在服务器端界面的头文件“dialog.h”中添加的具体代码如下:
class TimeServer;
public slots:
void slotShow(); //此槽函数用于界面上显示的请求次数
private:
TimeServer *timeServer; //TCP服务器端timeServer
int count; //请求次数计数器count
(9)在源文件“dialog.cpp”中,添加的头文件如下:
#include <QMessageBox>
#include "timeserver.h"
其中,在Dialog类的构造函数中添加的内容,用于启动服务器端的网络监听,其具体实现代码如下:
count = 0;
timeServer = new TimeServer(this);
if (!timeServer->listen())
{
QMessageBox::critical(this, tr("多线程时间服务器"),
tr("无法启动服务器:%1.").arg(timeServer->errorString()));
close();
return;
}
Label1->setText(tr("服务器端口:%1.").arg(timeServer->serverPort()));
在源文件“dialog.cpp”中,槽函数slotShow()的具体内容如下:
void Dialog::slotShow()
{
Label2->setText(tr("第%1次请求完毕。").arg(++count));
}
其中,Label2->setText(tr("第%1次请求完毕。").arg(++count))在标签Label2上显示当前的请求次数,并将请求数计数count加1.注意,槽函数slotShow()虽然被多个线程激活,但调用入口只有主线程的事件循环这一个。多个线程的激活信号最终会在主线程的事件循环中排队调用此槽函数,从而保证了count变量的互斥访问。因此,槽函数slotShow()是一个天然的临界区。
(10)在服务器端工程文件“TimeServer.pro”中添加如下代码:
QT += network
(11)最后运行服务器端工程“TimeServer.pro”,结果如图12.5所示。
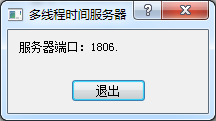
12.3.2 【实例】:客户端编程
【例】(难度中等)(CH1205)客户端编程,界面效果如图12.7所示。
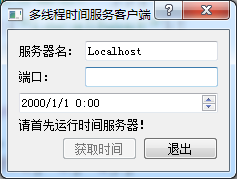
操作步骤如下:
(1)建立客户端工程“TimeClient.pro”。在头文件“timeclient.h”中,定义了客户端界面类TimeClient继承自QDialog类,其具体代码如下:
#include <QDialog>
#include <QLabel>
#include <QLineEdit>
#include <QPushButton>
#include <QDateTimeEdit>
#include <QTcpSocket>
#include <QAbstractSocket>
class TimeClient : public QDialog
{
Q_OBJECT
public:
TimeClient(QWidget *parent = 0);
~TimeClient();
public slots:
void enableGetBtn();
void getTime();
void readTime();
void showError(QAbstractSocket::SocketError socketError);
private:
QLabel *serverNameLabel;
QLineEdit *serverNameLineEdit;
QLabel *portLabel;
QLineEdit *portLineEdit;
QDateTimeEdit *dateTimeEdit;
QLabel *stateLabel;
QPushButton *getBtn;
QPushButton *quitBtn;
uint time2u;
QTcpSocket *tcpSocket;
};
(2)在源文件“timeclient.cpp”中,TimeClient类的构造函数完成了初始化界面,其具体代码如下:
#include "timeclient.h"
#include <QHBoxLayout>
#include <QVBoxLayout>
#include <QGridLayout>
#include <QDataStream>
#include <QMessageBox>
#pragma execution_character_set("utf-8")
TimeClient::TimeClient(QWidget *parent)
: QDialog(parent)
{
setWindowTitle(tr("多线程时间服务客户端"));
serverNameLabel = new QLabel(tr("服务器名:"));
serverNameLineEdit = new QLineEdit("Localhost");
portLabel = new QLabel(tr("端口:"));
portLineEdit = new QLineEdit;
QGridLayout *layout = new QGridLayout;
layout->addWidget(serverNameLabel, 0, 0);
layout->addWidget(serverNameLineEdit, 0, 1);
layout->addWidget(portLabel, 1, 0);
layout->addWidget(portLineEdit, 1, 1);
dateTimeEdit = new QDateTimeEdit(this);
QHBoxLayout *layout1 = new QHBoxLayout;
layout1->addWidget(dateTimeEdit);
stateLabel = new QLabel(tr("请首先运行时间服务器!"));
QHBoxLayout *layout2 = new QHBoxLayout;
layout2->addWidget(stateLabel);
getBtn = new QPushButton(tr("获取时间"));
getBtn->setDefault(true);
getBtn->setEnabled(false);
quitBtn = new QPushButton(tr("退出"));
QHBoxLayout *layout3 = new QHBoxLayout;
layout3->addStretch();
layout3->addWidget(getBtn);
layout3->addWidget(quitBtn);
QVBoxLayout *mainLayout = new QVBoxLayout(this);
mainLayout->addLayout(layout);
mainLayout->addLayout(layout1);
mainLayout->addLayout(layout2);
mainLayout->addLayout(layout3);
connect(serverNameLineEdit, SIGNAL(textChanged(QString)),
this, SLOT(enableGetBtn()));
connect(portLineEdit, SIGNAL(textChanged(QString)),
this, SLOT(enableGetBtn()));
connect(getBtn, SIGNAL(clicked()), this, SLOT(getTime()));
connect(quitBtn, SIGNAL(clicked()), this, SLOT(close()));
tcpSocket = new QTcpSocket(this);
connect(tcpSocket, SIGNAL(readyRead()), this, SLOT(readTime()));
connect(tcpSocket, SIGNAL(error(QAbstractSocket::SocketError)), this,
SLOT(showError(QAbstractSocket::SocketError)));
portLineEdit->setFocus();
}
在源文件“timeclient.cpp”中,enableGetBtn()函数的具体代码如下:
void TimeClient::enableGetBtn()
{
getBtn->setEnabled(!serverNameLineEdit->text().isEmpty() &&
!portLineEdit->text().isEmpty());
}
在源文件“timeclient.cpp”中,getTime()函数的具体代码如下:
void TimeClient::getTime()
{
getBtn->setEnabled(false);
time2u = 0;
tcpSocket->abort();
tcpSocket->connectToHost(serverNameLineEdit->text(),
portLineEdit->text().toInt());
}
在源文件“timeclient.cpp”中,readTime()函数的具体代码如下:
void TimeClient::readTime()
{
QDataStream in(tcpSocket);
in.setVersion(QDataStream::Qt_5_8);
if (time2u == 0)
{
if (tcpSocket->bytesAvailable() < (int)sizeof(uint))
return;
in >> time2u;
}
dateTimeEdit->setDateTime(QDateTime::fromTime_t(time2u));
getBtn->setEnabled(true);
}
在源文件“timeclient.cpp”中,showError()函数的具体代码如下:
void TimeClient::showError(QAbstractSocket::SocketError socketError)
{
switch (socketError)
{
case QAbstractSocket::RemoteHostClosedError:
break;
case QAbstractSocket::HostNotFoundError:
QMessageBox::information(this, tr("时间服务客户端"),
tr("主机不可达!"));
break;
case QAbstractSocket::ConnectionRefusedError:
QMessageBox::information(this, tr("实践服务客户端"),
tr("产生如下错误:%1.").arg(tcpSocket->errorString()));
}
getBtn->setEnabled(true);
}
(3)在客户端工程文件“TimeClient.pro”中,添加如下代码:
QT += network
(4)运行客户端工程“TimeClient.pro”,显示界面如图12.7所示。
最后,同时运行服务器和护短程序,单击客户端“获取时间”按钮,从服务器上获得当前的系统时间,如图12.8所示。
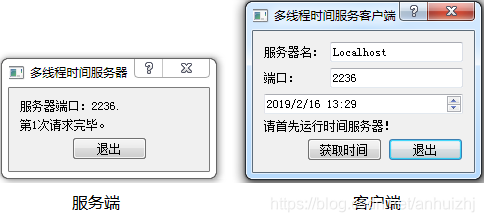
完整代码下载地址:https://download.csdn.net/download/anhuizhj/10960250