题目描述:
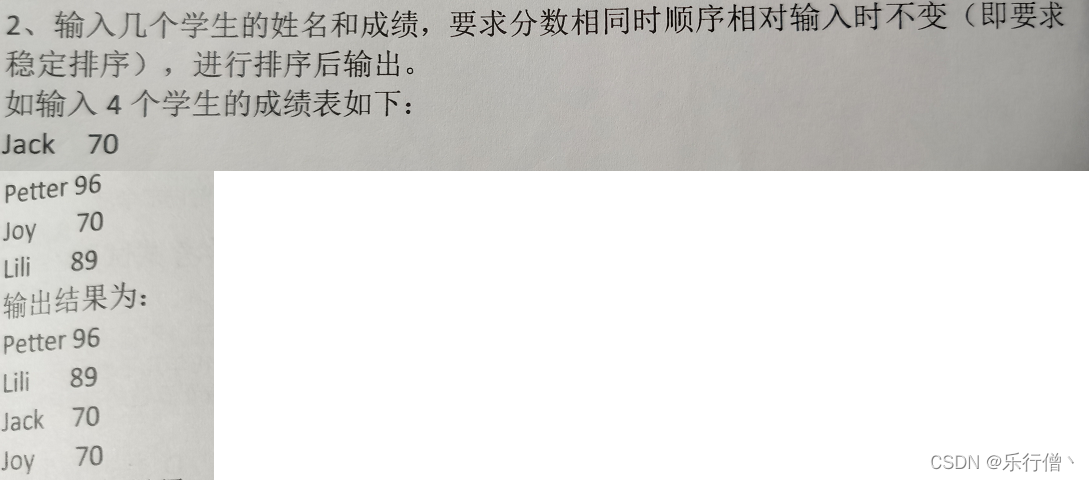
解题思路:
- 选择一种稳定的排序算法,针对成绩关键字进行排序
- 抽象学生类
代码实现:
#include <iostream>
#include <map>
#include <string>
#include <algorithm>
using namespace std;
struct Student {
std::string name;
int score;
};
namespace lsc {
void swap(Student* arr, int i, int j) {
Student t = arr[i];
arr[i] = arr[j];
arr[j] = t;
}
}
const int N = 100;
Student stu[N];
int idx = 0;
void BubbleSort(Student* stu, int n) {
int i = 0;
int j = 0;
bool flag = false;
for (i = 0; i < n - 1; i++) {
flag = true;
for (j = i + 1; j < n - 1 - i; j++) {
if (stu[i].score < stu[j].score) {
lsc::swap(stu, i, j);
flag = false;
}
}
if (flag) {
break;
}
}
}
int main() {
idx = 4;
stu[0] = {"Jack", 70};
stu[1] = {"Petter", 96};
stu[2] = {"Joy", 70};
stu[3] = {"LiLi", 89};
auto f = [] (Student s) {cout << s.name << ":" << s.score << endl;};
for_each(stu, stu + idx, f);
BubbleSort(stu, idx);
cout << endl;
for_each(stu, stu + idx, f);
return 0;
}