前言
上一篇简单的阐述了 spring-cloud-thrift-starter
这个插件的配置和使用,并引入了一个calculator
的项目。本文将基于一个银行存款、取款的业务场景,给出一套thrift
在生产环境的应用案例。
首先设计如下几张简单的数据库表:银行(bank
)、分支(branch
)、银行卡(deposit_card
)、客户(customer
)、存款历史纪录(deposit_history
)、取款历史纪录(withdraw_history
)。
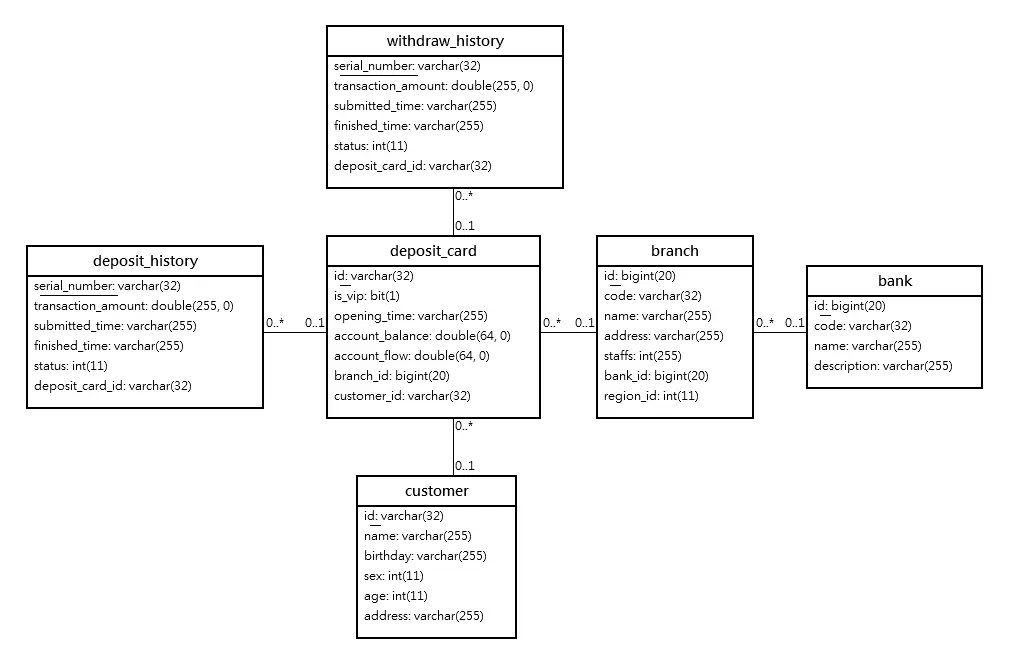
image
正文
项目结构如下,依然是由三个模块组成:
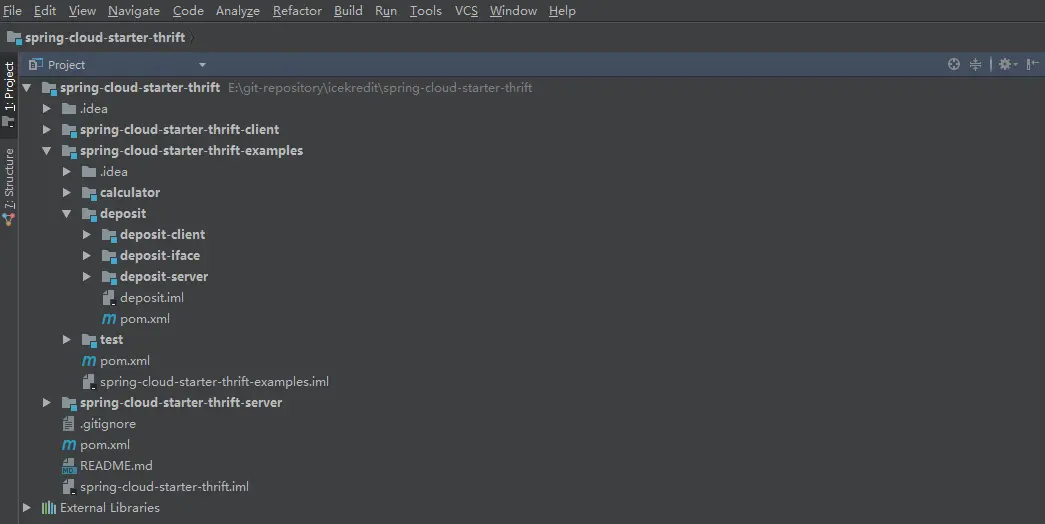
image
- deposit
- deposit-client
- deposit-iface
- deposit-server
Thrift IDL编写
关于 thrift
更复杂的用法可以参考Apache Thrift
基础学习系列,根据数据库表的设计编写 deposit.thrift
。
deposit.thrift
定义了以下四个部分:命名空间 (namespace
)、枚举类型 (enum
)、结构类型 (struct
)和服务类型 (service
)。
(a). 命名空间 (namespace
)
// 指定编译生成的源代码的包路径名称
namespace java com.icekredit.rpc.thrift.examples.thrift
(b). 枚举类型 (enum
)
// 通过枚举定义银行分支所属区域
enum ThriftRegion {
NORTH = 1,
CENTRAL = 2,
SOUTH = 3,
EAST = 4,
SOUTHWEST = 5,
NORTHWEST = 6,
NORTHEAST = 7
}
// 存款完成状态
enum ThriftDepositStatus {
FINISHED = 1,
PROCCEDING = 2,
FAILED = 3
}
// 取款完成状态
enum ThriftWithdrawStatus {
FINISHED = 1,
PROCCEDING = 2,
FAILED = 3
}
(c). 结构类型 (struct
)
// 银行
struct ThriftBank {
1: required i64 id,
2: required string code,
3: required string name,
4: optional string description,
5: optional map<ThriftRegion, list<ThriftBranch>> branches
}
// 银行分支
struct ThriftBranch {
1: required i64 id,
2: required string code,
3: required string name,
4: required string address,
5: optional i32 staffs,
6: optional ThriftBank bank,
7: optional ThriftRegion region
}
// 客户
struct ThriftCustomer {
1: required string IDNumber,
2: required string name,
3: required string birthday,
4: required i32 sex = 0,
5: required i32 age,
6: optional list<string> address,
7: optional set<ThriftDepositCard> depositCards
}
// 银行卡
struct ThriftDepositCard {
1: required string id,
2: required bool isVip,
3: required string openingTime,
4: required double accountBalance,
5: optional double accountFlow,
6: optional ThriftBranch branch,
7: optional ThriftCustomer customer,
8: optional list<ThriftDeposit> depositHistory,
9: optional list<ThriftWithdraw> WithdrawHistory
}
// 存款历史纪录
struct ThriftDeposit {
1: required string serialNumber,
2: required double transactionAmount,
3: required string submittedTime,
4: optional string finishedTime,
5: optional ThriftDepositStatus status,
6: optional ThriftDepositCard depositCard
}
// 取款历史纪录
struct ThriftWithdraw {
1: required string serialNumber,
2: required double transactionAmount,
3: required string submittedTime,
4: optional string finishedTime,
5: optional ThriftWithdrawStatus status,
6: optional ThriftDepositCard depositCard
}
(d). 服务类型 (service
)
// 银行 - 业务服务定义
service ThriftBankService {
void registerNewBank(ThriftBank bank);
list<ThriftBank> queryAllBanks();
ThriftBank getBankById(i64 bankId);
map<ThriftRegion, list<ThriftBranch>> queryAllBranchesByRegion(i64 bankId);
}
// 银行分支 - 业务服务定义
service ThriftBranchService {
void addNewBranch(i64 bankId, ThriftBranch branch);
list<ThriftBranch> queryAllBranches(i64 bankId);
ThriftBranch getBranchById(i64 branchId);
}
// 客户 - 业务服务定义
service ThriftCustomerService {
ThriftCustomer getCustomerById(string customerId);
list<ThriftCustomer> queryAllCustomers();
void addNewUser(ThriftCustomer customer);
void modifyUserById(string customerId, ThriftCustomer customer);
i32 getTotalDepositCard(string customerId);
}
// 银行卡 - 业务服务定义
service ThriftDepositCardService {
set<ThriftDepositCard> queryAllDepositCards(string customerId);
void addNewDepositCard(string customerId, ThriftDepositCard depositCard);
ThriftDepositStatus depositMoney(string depositCardId, double money);
ThriftWithdrawStatus withdrawMoney(string depositCardId, double money);
list<ThriftDeposit> queryDepositHistorys(string depositCardId);
list<ThriftWithdraw> queryWithdrawHistorys(string depositCardId);
}
进入src/main/thrift
目录,编译生成所需的枚举类、结构类和业务服务类的源文件。
thrift -gen java ./deposit.thrift
所有生成的源文件都位于同一个命名空间(包)下面:com.icekredit.rpc.thrift.examples.thrift
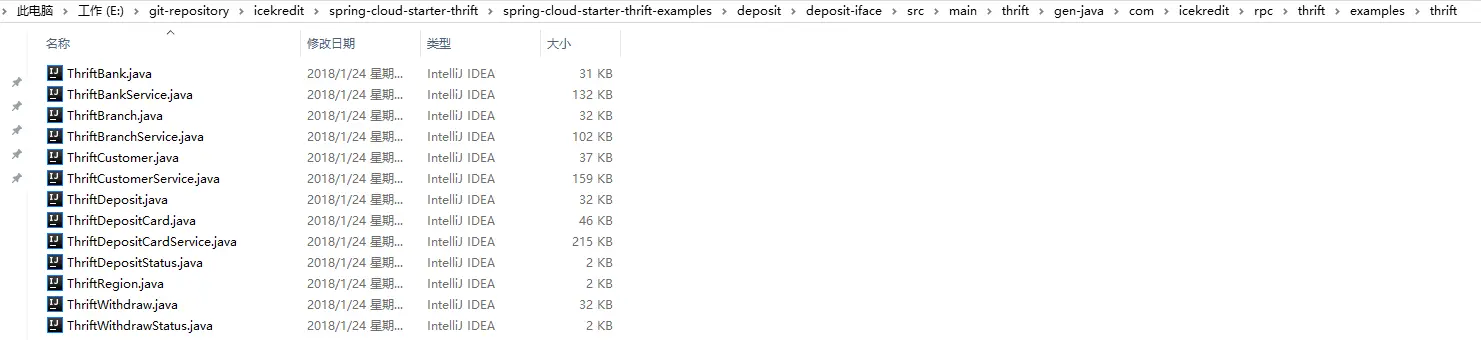
image
中间契约(deposit-iface)
将上述源文件拷贝到 deposit-iface
模块中。
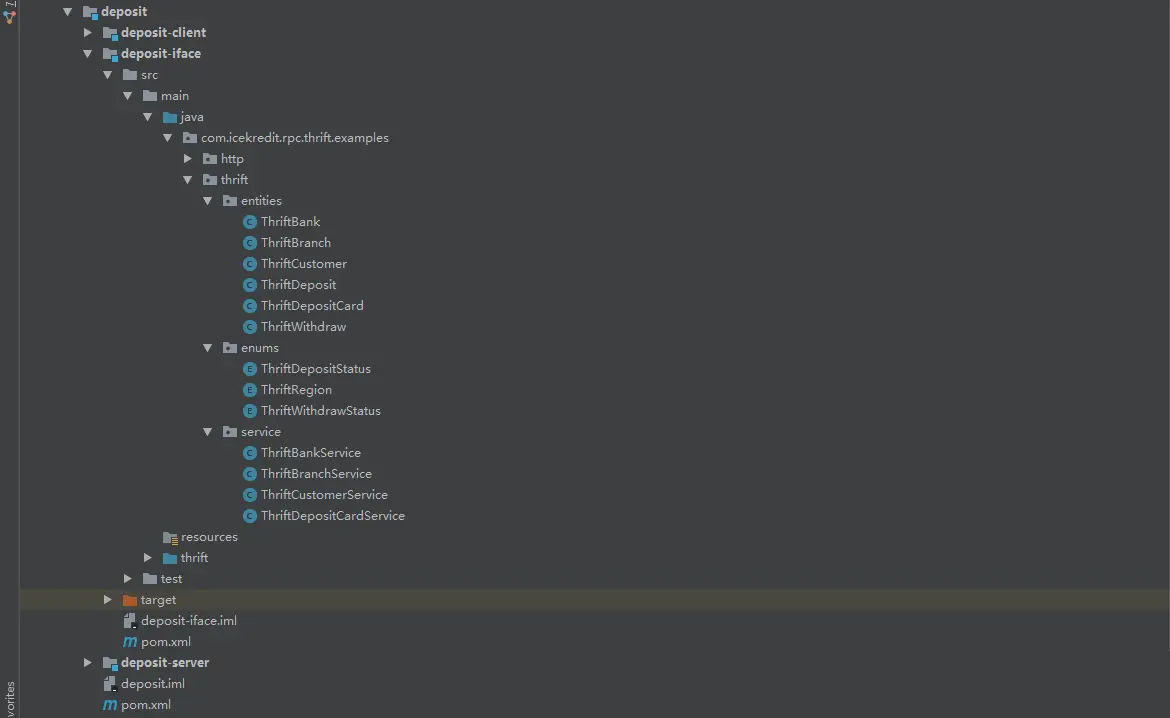
image